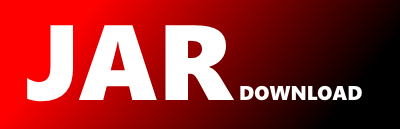
javax.security.jacc.URLPattern Maven / Gradle / Ivy
Show all versions of jboss-javaee-all-8.0
package javax.security.jacc;
/**
*
* The representation of a URLPattern in the {@code WebResourcePermission} and {@code WebUserDataPermission}
* URLPatternSpecs.
*
*
* @author Scott Stark
* @author Stefan Guilhen
* @see WebResourcePermission
* @see WebUserDataPermission
*/
class URLPattern
{
private enum PatternType {
/** the '/' pattern */
DEFAULT,
/** the '/*' pattern */
THE_PATH_PREFIX,
/** a '/.../*' pattern */
PATH_PREFIX,
/** a '*.xxx' pattern */
EXTENSION,
/** an exact pattern */
EXACT
};
private String pattern;
private String ext;
private int length;
private PatternType type;
/**
*
* Creates a {@code URLPattern} instance from the specified pattern {@code String}.
*
*
* @param pattern
* the pattern {@code String}.
*/
URLPattern(String pattern)
{
this.pattern = pattern;
length = pattern.length();
if (pattern.equals("/"))
type = PatternType.DEFAULT;
else if (pattern.startsWith("/*"))
type = PatternType.THE_PATH_PREFIX;
else if (length > 0 && pattern.charAt(0) == '/' && pattern.endsWith("/*"))
type = PatternType.PATH_PREFIX;
else if (pattern.startsWith("*."))
{
type = PatternType.EXTENSION;
ext = pattern.substring(1);
}
else
type = PatternType.EXACT;
}
/**
*
* Checks if this pattern matches the specified {@code URLPattern}.
*
*
*
* The matching rules from the {@code WebResourcePermission#implies}:
*
* - their pattern values are {@code String} equivalent, or
* - this pattern is the path-prefix pattern "/*", or
* - this pattern is a path-prefix pattern (that is, it starts with "/" and ends with "/*") and the argument
* pattern starts with the substring of this pattern, minus its last 2 characters, and the next character of the
* argument pattern, if there is one, is "/", or
* - this pattern is an extension pattern (that is, it starts with "*.") and the argument pattern ends with this
* pattern, or 5. the reference pattern is the special default pattern, "/", which matches all argument patterns.
*
*
*
* @param url
* the {@code URLPattern} instance to which this pattern is to be matched.
* @return {@code true} if this pattern matches the specified {@code URLPattern}; {@code false} otherwise.
*/
boolean matches(URLPattern url)
{
return matches(url.pattern);
}
/**
*
* Checks if this pattern matches the specified pattern String.
*
*
*
* The matching rules from the {@code WebResourcePermission#implies}:
*
* - their pattern values are {@code String} equivalent, or
* - this pattern is the path-prefix pattern "/*", or
* - this pattern is a path-prefix pattern (that is, it starts with "/" and ends with "/*") and the argument
* pattern starts with the substring of this pattern, minus its last 2 characters, and the next character of the
* argument pattern, if there is one, is "/", or
* - this pattern is an extension pattern (that is, it starts with "*.") and the argument pattern ends with this
* pattern, or 5. the reference pattern is the special default pattern, "/", which matches all argument patterns.
*
*
*
* @param urlPattern
* a {@code String} representing the pattern to which this pattern is to be matched.
* @return {@code true} if this pattern matches the specified {@code URLPattern}; {@code false} otherwise.
*/
boolean matches(String urlPattern)
{
// 2 or 5
if (type == PatternType.DEFAULT || type == PatternType.THE_PATH_PREFIX)
return true;
// 4, extension pattern
if (type == PatternType.EXTENSION && urlPattern.endsWith(ext))
return true;
// 3. a path-prefix pattern
if (type == PatternType.PATH_PREFIX)
{
if (urlPattern.regionMatches(0, pattern, 0, length - 2))
{
int last = length - 2;
if (urlPattern.length() > last && urlPattern.charAt(last) != '/')
return false;
return true;
}
return false;
}
// 1. pattern values are String equivalent for exact pattern
if (pattern.equals(urlPattern))
return true;
return false;
}
/**
*
* Obtains the {@code String} representation of this pattern.
*
*
* @return this pattern's {@code String} representation.
*/
String getPattern()
{
return this.pattern;
}
/**
*
* Checks if this pattern is a default (i.e. '/') pattern.
*
*
* @return {@code true} if this is a default pattern; {@code false} otherwise.
*/
boolean isDefault()
{
return this.type == PatternType.DEFAULT;
}
/**
*
* Checks if this pattern is an exact pattern.
*
*
* @return {@code true} if this is an exact pattern; {@code false} otherwise.
*/
boolean isExact()
{
return this.type == PatternType.EXACT;
}
/**
*
* Checks if this pattern is an extension (i.e. '*.xxx') pattern.
*
*
* @return {@code true} if this is an extension pattern; {@code false} otherwise.
*/
boolean isExtension()
{
return this.type == PatternType.EXTENSION;
}
/**
*
* Checks if this pattern is a prefix (i.e. '/*' or '/.../*') pattern.
*
*
* @return {@code true} if this is a prefix pattern; {@code false} otherwise.
*/
boolean isPrefix()
{
return this.type == PatternType.THE_PATH_PREFIX || this.type == PatternType.PATH_PREFIX;
}
/*
* (non-Javadoc)
*
* @see java.lang.Object#hashCode()
*/
@Override
public int hashCode()
{
return this.pattern.hashCode();
}
/*
* (non-Javadoc)
*
* @see java.lang.Object#equals(java.lang.Object)
*/
@Override
public boolean equals(Object o)
{
if (o instanceof URLPattern == false)
return false;
URLPattern pattern = (URLPattern) o;
boolean equals = this.type == pattern.type;
if (equals)
{
equals = this.pattern.equals(pattern.pattern);
}
return equals;
}
}