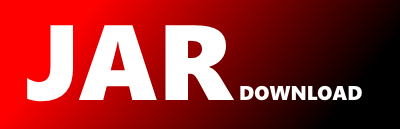
org.jboss.weld.injection.producer.BasicInjectionTarget Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source
* Copyright 2008, Red Hat, Inc., and individual contributors
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jboss.weld.injection.producer;
import java.util.HashSet;
import java.util.Set;
import javax.enterprise.context.spi.CreationalContext;
import javax.enterprise.inject.spi.AnnotatedType;
import javax.enterprise.inject.spi.Bean;
import javax.enterprise.inject.spi.InjectionPoint;
import javax.enterprise.inject.spi.InjectionTarget;
import javax.enterprise.inject.spi.Interceptor;
import javax.interceptor.Interceptors;
import org.jboss.weld.annotated.enhanced.EnhancedAnnotatedType;
import org.jboss.weld.annotated.slim.SlimAnnotatedType;
import org.jboss.weld.logging.BeanLogger;
import org.jboss.weld.manager.BeanManagerImpl;
import org.jboss.weld.manager.api.WeldInjectionTarget;
import org.jboss.weld.util.collections.WeldCollections;
import org.jboss.weld.util.reflection.Reflections;
/**
* Basic {@link InjectionTarget} implementation. The implementation supports:
*
* - @Inject injection + initializers
* - @PostConstruct/@PreDestroy callbacks
*
*
* Interception and decoration is not supported but can be added using extension points.
*
* @author Pete Muir
* @author Jozef Hartinger
*/
public class BasicInjectionTarget extends AbstractProducer implements WeldInjectionTarget {
public static BasicInjectionTarget create(EnhancedAnnotatedType type, Bean bean, BeanManagerImpl beanManager, Injector injector, LifecycleCallbackInvoker invoker) {
return new BasicInjectionTarget(type, bean, beanManager, injector, invoker);
}
public static BasicInjectionTarget createDefault(EnhancedAnnotatedType type, Bean bean, BeanManagerImpl beanManager) {
return new BasicInjectionTarget(type, bean, beanManager, null);
}
public static BasicInjectionTarget createDefault(EnhancedAnnotatedType type, Bean bean, BeanManagerImpl beanManager, Instantiator instantiator) {
return new BasicInjectionTarget(type, bean, beanManager, instantiator);
}
/**
* Creates {@link InjectionTarget} for interceptors which do not have associated {@link Interceptor}. These interceptors are a
* result of using {@link Interceptors} annotation directly on the target class.
*/
public static BasicInjectionTarget createNonCdiInterceptor(EnhancedAnnotatedType type, BeanManagerImpl beanManager) {
return new BasicInjectionTarget(type, null, beanManager, DefaultInjector.of(type, null, beanManager), NoopLifecycleCallbackInvoker.getInstance());
}
protected final BeanManagerImpl beanManager;
private final SlimAnnotatedType type;
private final Set injectionPoints;
// Instantiation
private Instantiator instantiator;
private final Injector injector;
private final LifecycleCallbackInvoker invoker;
protected BasicInjectionTarget(EnhancedAnnotatedType type, Bean bean, BeanManagerImpl beanManager, Injector injector, LifecycleCallbackInvoker invoker) {
this(type, bean, beanManager, injector, invoker, null);
}
protected BasicInjectionTarget(EnhancedAnnotatedType type, Bean bean, BeanManagerImpl beanManager, Injector injector, LifecycleCallbackInvoker invoker, Instantiator instantiator) {
this.beanManager = beanManager;
this.type = type.slim();
this.injector = injector;
this.invoker = invoker;
final Set injectionPoints = new HashSet();
checkType(type);
this.injector.registerInjectionPoints(injectionPoints);
if (instantiator != null) {
this.instantiator = instantiator;
} else {
this.instantiator = initInstantiator(type, bean, beanManager, injectionPoints);
}
this.injectionPoints = WeldCollections.immutableGuavaSet(injectionPoints);
checkDelegateInjectionPoints();
}
protected BasicInjectionTarget(EnhancedAnnotatedType type, Bean bean, BeanManagerImpl beanManager, Instantiator instantiator) {
this(type, bean, beanManager, DefaultInjector.of(type, bean, beanManager), DefaultLifecycleCallbackInvoker.of(type), instantiator);
}
protected void checkType(EnhancedAnnotatedType type) {
if (Reflections.isNonStaticInnerClass(type.getJavaClass())) {
throw BeanLogger.LOG.simpleBeanAsNonStaticInnerClassNotAllowed(type);
}
}
@Override
public T produce(CreationalContext ctx) {
return instantiator.newInstance(ctx, beanManager);
}
@Override
public void inject(T instance, CreationalContext ctx) {
injector.inject(instance, ctx, beanManager, type, this);
}
@Override
public void postConstruct(T instance) {
invoker.postConstruct(instance, instantiator);
}
@Override
public void preDestroy(T instance) {
invoker.preDestroy(instance, instantiator);
}
@Override
public void dispose(T instance) {
// No-op
}
@Override
public Set getInjectionPoints() {
return injectionPoints;
}
protected SlimAnnotatedType getType() {
return type;
}
public BeanManagerImpl getBeanManager() {
return beanManager;
}
public Instantiator getInstantiator() {
return instantiator;
}
public void setInstantiator(Instantiator instantiator) {
this.instantiator = instantiator;
}
public boolean hasInterceptors() {
return instantiator.hasInterceptorSupport();
}
public boolean hasDecorators() {
return instantiator.hasDecoratorSupport();
}
protected void initializeAfterBeanDiscovery(EnhancedAnnotatedType annotatedType) {
}
/**
* Returns an instantiator that will be used to create a new instance of a given component. If the instantiator uses a
* constructor with injection points, the implementation of the
* {@link #initInstantiator(EnhancedAnnotatedType, Bean, BeanManagerImpl, Set)} method is supposed to register all these
* injection points within the injectionPoints set passed in as a parameter.
*/
protected Instantiator initInstantiator(EnhancedAnnotatedType type, Bean bean, BeanManagerImpl beanManager, Set injectionPoints) {
DefaultInstantiator instantiator = new DefaultInstantiator(type, bean, beanManager);
injectionPoints.addAll(instantiator.getParameterInjectionPoints());
return instantiator;
}
@Override
public AnnotatedType getAnnotated() {
return type;
}
@Override
public AnnotatedType getAnnotatedType() {
return getAnnotated();
}
public Injector getInjector() {
return injector;
}
public LifecycleCallbackInvoker getLifecycleCallbackInvoker() {
return invoker;
}
@Override
public String toString() {
StringBuilder result = new StringBuilder("InjectionTarget for ");
if (getBean() == null) {
result.append(getAnnotated());
} else {
result.append(getBean());
}
return result.toString();
}
@Override
public Bean getBean() {
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy