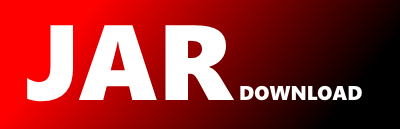
org.jboss.weld.injection.producer.WeldInjectionTargetBuilderImpl Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source
* Copyright 2014, Red Hat, Inc., and individual contributors
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jboss.weld.injection.producer;
import java.security.AccessController;
import java.security.PrivilegedAction;
import javax.enterprise.inject.spi.AnnotatedType;
import javax.enterprise.inject.spi.Bean;
import org.jboss.weld.annotated.enhanced.EnhancedAnnotatedType;
import org.jboss.weld.manager.BeanManagerImpl;
import org.jboss.weld.manager.api.WeldInjectionTarget;
import org.jboss.weld.manager.api.WeldInjectionTargetBuilder;
import org.jboss.weld.resources.ClassTransformer;
import org.jboss.weld.util.InjectionTargets;
/**
* Default {@link WeldInjectionTargetBuilder} implementation. The builder runs in a privileged context.
*
* @author Jozef Hartinger
*
* @param
*/
public class WeldInjectionTargetBuilderImpl implements WeldInjectionTargetBuilder, PrivilegedAction> {
private final InjectionTargetService injectionTargetService;
private boolean resourceInjectionEnabled = true;
private boolean targetClassLifecycleCallbacksEnabled = true;
private boolean interceptorsEnabled = true;
private boolean decorationEnabled = true;
private Bean bean;
private final EnhancedAnnotatedType type;
private final BeanManagerImpl manager;
public WeldInjectionTargetBuilderImpl(AnnotatedType type, BeanManagerImpl manager) {
this.manager = manager;
this.type = manager.getServices().get(ClassTransformer.class).getEnhancedAnnotatedType(type, manager.getId());
this.injectionTargetService = manager.getServices().get(InjectionTargetService.class);
}
@Override
public WeldInjectionTargetBuilder setResourceInjectionEnabled(boolean value) {
this.resourceInjectionEnabled = value;
return this;
}
@Override
public WeldInjectionTargetBuilder setTargetClassLifecycleCallbacksEnabled(boolean value) {
this.targetClassLifecycleCallbacksEnabled = value;
return this;
}
@Override
public WeldInjectionTargetBuilder setInterceptionEnabled(boolean value) {
this.interceptorsEnabled = value;
return this;
}
@Override
public WeldInjectionTargetBuilder setDecorationEnabled(boolean value) {
this.decorationEnabled = value;
return this;
}
@Override
public WeldInjectionTargetBuilder setBean(Bean bean) {
this.bean = bean;
return this;
}
@Override
public WeldInjectionTarget build() {
if (System.getSecurityManager() != null) {
return AccessController.doPrivileged(this);
}
return run();
}
@Override
public BasicInjectionTarget run() {
BasicInjectionTarget injectionTarget = buildInternal();
injectionTargetService.addInjectionTargetToBeInitialized(type, injectionTarget);
injectionTargetService.validateProducer(injectionTarget);
return injectionTarget;
}
private BasicInjectionTarget buildInternal() {
final Injector injector = buildInjector();
final LifecycleCallbackInvoker invoker = buildInvoker();
NonProducibleInjectionTarget nonProducible = InjectionTargets.createNonProducibleInjectionTarget(type, bean, injector, invoker, manager);
if (nonProducible != null) {
return nonProducible;
}
if (!interceptorsEnabled && !decorationEnabled) {
return BasicInjectionTarget.create(type, bean, manager, injector, invoker);
} else if (interceptorsEnabled && decorationEnabled) {
return new BeanInjectionTarget(type, bean, manager, injector, invoker);
}
throw new IllegalStateException(
"Unsupported combination: [interceptorsEnabled=" + interceptorsEnabled + ", decorationEnabled=" + decorationEnabled + "]");
}
private Injector buildInjector() {
if (resourceInjectionEnabled) {
return ResourceInjector.of(type, bean, manager);
} else {
return DefaultInjector.of(type, bean, manager);
}
}
private LifecycleCallbackInvoker buildInvoker() {
if (targetClassLifecycleCallbacksEnabled) {
return DefaultLifecycleCallbackInvoker.of(type);
} else {
return NoopLifecycleCallbackInvoker.getInstance();
}
}
@Override
public String toString() {
return "WeldInjectionTargetBuilderImpl for " + type;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy