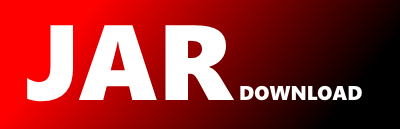
org.jboss.weld.util.collections.ImmutableSet Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source
* Copyright 2014, Red Hat, Inc., and individual contributors
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jboss.weld.util.collections;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.Set;
import org.jboss.weld.util.Preconditions;
/**
* Weld's immutable set implementation. Instances returned from methods of this class may use different strategies to achieve good performance / memory
* consumption balance.
*
* These strategies include:
*
* - A single shared {@link Set} implementation instance representing an empty list
* - An optimized implementation for holding one, two or three references.
* - An immutable {@link Set} implementation based on hashing
*
*
*
* @author Jozef Hartinger
* @ee WELD-1753
*
* @param the type of elements
*/
public abstract class ImmutableSet extends AbstractImmutableSet {
ImmutableSet() {
}
/**
* Creates a new immutable set that consists of the elements in the given collection. If the given collection is already an instance created by
* {@link ImmutableSet}, the instance is re-used.
*
* @param collection the given collection
* @return a new immutable set that consists of the elements in the given collection
*/
@SuppressWarnings("unchecked")
public static Set copyOf(Collection extends T> collection) {
Preconditions.checkNotNull(collection);
if (collection instanceof AbstractImmutableSet>) {
return (Set) collection;
}
if (collection.isEmpty()) {
return Collections.emptySet();
}
if (collection instanceof Set) {
return from((Set) collection);
}
return ImmutableSet. builder().addAll(collection).build();
}
@SuppressWarnings("unchecked")
public static Set copyOf(Iterable extends T> iterable) {
Preconditions.checkNotNull(iterable);
if (iterable instanceof AbstractImmutableSet>) {
return (Set) iterable;
}
if (!iterable.iterator().hasNext()) {
return Collections.emptySet();
}
return ImmutableSet. builder().addAll(iterable).build();
}
/**
* Creates a new immutable set that consists of given elements.
*
* @param elements the given elements
* @return a new immutable set that consists of given elements
*/
@SafeVarargs
public static Set of(T... elements) {
Preconditions.checkNotNull(elements);
return ImmutableSet. builder().addAll(elements).build();
}
/**
* Creates a new empty builder for building immutable sets.
*
* @return a new empty builder
*/
public static Builder builder() {
return new BuilderImpl();
}
/**
* Builder for building immutable sets. The builder may be re-used after build() is called.
*
* @author Jozef Hartinger
*
* @param the type of elements
*/
public interface Builder {
Builder add(T item);
Builder addAll(Iterable extends T> items);
Builder addAll(@SuppressWarnings("unchecked") T... items);
/**
* Create a new immutable set.
*
* @return a new immutable set
*/
Set build();
}
private static class BuilderImpl implements Builder {
private Set set;
private BuilderImpl() {
this.set = new LinkedHashSet<>();
}
@Override
public Builder add(T item) {
if (item == null) {
throw new IllegalArgumentException("This collection does not support null values");
}
set.add(item);
return this;
}
@Override
public Builder addAll(@SuppressWarnings("unchecked") T... items) {
for (T item : items) {
add(item);
}
return this;
}
@Override
public Builder addAll(Iterable extends T> items) {
for (T item : items) {
add(item);
}
return this;
}
@Override
public Set build() {
return from(set);
}
}
private static Set from(Set set) {
switch (set.size()) {
case 0:
return Collections.emptySet();
case 1:
return new ImmutableTinySet.Singleton(set);
case 2:
return new ImmutableTinySet.Doubleton(set);
case 3:
return new ImmutableTinySet.Tripleton(set);
default:
return new ImmutableHashSet<>(set);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy