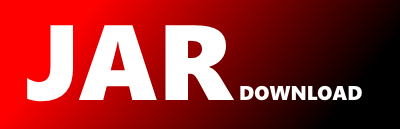
org.jboss.weld.environment.logging.CommonLogger_$logger Maven / Gradle / Ivy
package org.jboss.weld.environment.logging;
import org.jboss.weld.environment.logging.WeldEnvironmentLogger;
import java.util.Locale;
import java.lang.IllegalStateException;
import java.io.Serializable;
import org.jboss.weld.resources.spi.ClassFileInfoException;
import org.jboss.logging.DelegatingBasicLogger;
import jakarta.enterprise.inject.UnsatisfiedResolutionException;
import java.lang.String;
import org.jboss.logging.Logger;
import org.jboss.logging.BasicLogger;
import java.lang.Throwable;
import java.lang.Object;
import java.util.Arrays;
import static org.jboss.logging.Logger.Level.TRACE;
import static org.jboss.logging.Logger.Level.INFO;
import static org.jboss.logging.Logger.Level.DEBUG;
import static org.jboss.logging.Logger.Level.WARN;
/**
* Warning this class consists of generated code.
*/
public class CommonLogger_$logger extends DelegatingBasicLogger implements CommonLogger, org.jboss.weld.environment.logging.WeldEnvironmentLogger, BasicLogger, Serializable {
private static final long serialVersionUID = 1L;
private static final String FQCN = CommonLogger_$logger.class.getName();
public CommonLogger_$logger(final Logger log) {
super(log);
}
private static final Locale LOCALE = Locale.ROOT;
protected Locale getLoggingLocale() {
return LOCALE;
}
@Override
public final void couldNotReadResource(final Object param1, final Throwable cause) {
super.log.logv(FQCN, WARN, cause, couldNotReadResource$str(), param1);
}
protected String couldNotReadResource$str() {
return "WELD-ENV-000002: Could not read resource with name: {0}";
}
@Override
public final void unexpectedClassLoader(final Throwable cause) {
super.log.logv(FQCN, WARN, cause, unexpectedClassLoader$str());
}
protected String unexpectedClassLoader$str() {
return "WELD-ENV-000004: Could not invoke JNLPClassLoader#getJarFile(URL) on context class loader, expecting Web Start class loader";
}
@Override
public final void jnlpClassLoaderInternalException(final Throwable cause) {
super.log.logv(FQCN, WARN, cause, jnlpClassLoaderInternalException$str());
}
protected String jnlpClassLoaderInternalException$str() {
return "WELD-ENV-000005: JNLPClassLoader#getJarFile(URL) threw exception";
}
@Override
public final void jnlpClassLoaderInvocationException(final Throwable cause) {
super.log.logv(FQCN, WARN, cause, jnlpClassLoaderInvocationException$str());
}
protected String jnlpClassLoaderInvocationException$str() {
return "WELD-ENV-000006: Could not invoke JNLPClassLoader#getJarFile(URL) on context class loader";
}
@Override
public final void cannotHandleFilePath(final Object file, final Object path, final Throwable cause) {
super.log.logv(FQCN, WARN, cause, cannotHandleFilePath$str(), file, path);
}
protected String cannotHandleFilePath$str() {
return "WELD-ENV-000007: Error handling file path\n File: {0}\n Path: {1}";
}
@Override
public final void couldNotOpenStreamForURL(final Object param1, final Throwable cause) {
super.log.logv(FQCN, WARN, cause, couldNotOpenStreamForURL$str(), param1);
}
protected String couldNotOpenStreamForURL$str() {
return "WELD-ENV-000010: Could not open the stream on the url {0} when adding to the jandex index.";
}
@Override
public final void couldNotCloseStreamForURL(final Object param1, final Throwable cause) {
super.log.logv(FQCN, WARN, cause, couldNotCloseStreamForURL$str(), param1);
}
protected String couldNotCloseStreamForURL$str() {
return "WELD-ENV-000011: Could not close the stream on the url {0} when adding to the jandex index.";
}
protected String unableToLoadClass$str() {
return "WELD-ENV-000012: Unable to load class {0}";
}
@Override
public final ClassFileInfoException unableToLoadClass(final Object param1) {
final ClassFileInfoException result = new ClassFileInfoException(_formatMessage(unableToLoadClass$str(), param1));
_copyStackTraceMinusOne(result);
return result;
}
private String _formatMessage(final String format, final Object... args) {
final java.text.MessageFormat formatter = new java.text.MessageFormat(format, getLoggingLocale());
return formatter.format(args, new StringBuffer(), new java.text.FieldPosition(0)).toString();
}
private static void _copyStackTraceMinusOne(final Throwable e) {
final StackTraceElement[] st = e.getStackTrace();
e.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
}
protected String undefinedBeanDiscoveryValue$str() {
return "WELD-ENV-000013: beans.xml defines unrecognized bean-discovery-mode value: {0}";
}
@Override
public final IllegalStateException undefinedBeanDiscoveryValue(final Object param1) {
final IllegalStateException result = new IllegalStateException(_formatMessage(undefinedBeanDiscoveryValue$str(), param1));
_copyStackTraceMinusOne(result);
return result;
}
@Override
public final void reflectionFallback() {
super.log.logv(FQCN, INFO, null, reflectionFallback$str());
}
protected String reflectionFallback$str() {
return "WELD-ENV-000014: Falling back to Java Reflection for bean-discovery-mode=\"annotated\" discovery. Add io.smallrye:jandex to the classpath to speed-up startup.";
}
protected String unableToLoadAnnotation$str() {
return "WELD-ENV-000015: Unable to load annotation: {0}";
}
@Override
public final ClassFileInfoException unableToLoadAnnotation(final Object param1) {
final ClassFileInfoException result = new ClassFileInfoException(_formatMessage(unableToLoadAnnotation$str(), param1));
_copyStackTraceMinusOne(result);
return result;
}
protected String missingBeansXml$str() {
return "WELD-ENV-000016: Missing beans.xml file in META-INF";
}
@Override
public final IllegalStateException missingBeansXml() {
final IllegalStateException result = new IllegalStateException(missingBeansXml$str());
_copyStackTraceMinusOne(result);
return result;
}
protected String unableToResolveBean$str() {
return "WELD-ENV-000018: Unable to resolve a bean for {0} with bindings {1}";
}
@Override
public final UnsatisfiedResolutionException unableToResolveBean(final Object param1, final Object param2) {
final UnsatisfiedResolutionException result = new UnsatisfiedResolutionException(_formatMessage(unableToResolveBean$str(), param1, param2));
_copyStackTraceMinusOne(result);
return result;
}
protected String jandexIndexNotCreated$str() {
return "WELD-ENV-000019: Jandex index is null in the constructor of class: {0}";
}
@Override
public final IllegalStateException jandexIndexNotCreated(final Object param1) {
final IllegalStateException result = new IllegalStateException(_formatMessage(jandexIndexNotCreated$str(), param1));
_copyStackTraceMinusOne(result);
return result;
}
@Override
public final void usingJandex() {
super.log.logv(FQCN, INFO, null, usingJandex$str());
}
protected String usingJandex$str() {
return "WELD-ENV-000020: Using jandex for bean discovery";
}
@Override
public final void archiveIsolationDisabled() {
super.log.logv(FQCN, DEBUG, null, archiveIsolationDisabled$str());
}
protected String archiveIsolationDisabled$str() {
return "WELD-ENV-000023: Archive isolation disabled - only one bean archive will be created";
}
@Override
public final void archiveIsolationEnabled() {
super.log.logv(FQCN, DEBUG, null, archiveIsolationEnabled$str());
}
protected String archiveIsolationEnabled$str() {
return "WELD-ENV-000024: Archive isolation enabled - creating multiple isolated bean archives if needed";
}
protected String indexForNameNotFound$str() {
return "WELD-ENV-000025: Index for name: {0} not found.";
}
@Override
public final ClassFileInfoException indexForNameNotFound(final Object param1) {
final ClassFileInfoException result = new ClassFileInfoException(_formatMessage(indexForNameNotFound$str(), param1));
_copyStackTraceMinusOne(result);
return result;
}
protected String unableToInstantiate$str() {
return "WELD-ENV-000026: Unable to instantiate {0} using parameters: {1}.";
}
@Override
public final IllegalStateException unableToInstantiate(final Object param1, final Object param2, final Throwable cause) {
final IllegalStateException result = new IllegalStateException(_formatMessage(unableToInstantiate$str(), param1, param2), cause);
_copyStackTraceMinusOne(result);
return result;
}
@Override
public final void initSkippedNoBeanArchiveFound() {
super.log.logf(FQCN, WARN, null, initSkippedNoBeanArchiveFound$str());
}
protected String initSkippedNoBeanArchiveFound$str() {
return "WELD-ENV-000028: Weld initialization skipped - no bean archive found";
}
protected String cannotLoadClass$str() {
return "WELD-ENV-000029: Cannot load class for {0}.";
}
@Override
public final IllegalStateException cannotLoadClass(final Object param1, final Throwable cause) {
final IllegalStateException result = new IllegalStateException(_formatMessage(cannotLoadClass$str(), param1), cause);
_copyStackTraceMinusOne(result);
return result;
}
@Override
public final void cannotLoadClassUsingResourceLoader(final String className) {
super.log.logv(FQCN, DEBUG, null, cannotLoadClassUsingResourceLoader$str(), className);
}
protected String cannotLoadClassUsingResourceLoader$str() {
return "WELD-ENV-000030: Cannot load class using the ResourceLoader: {0}";
}
@Override
public final void beanArchiveReferenceCannotBeHandled(final Object beanArchiveRef, final Object handlers) {
super.log.logv(FQCN, WARN, null, beanArchiveReferenceCannotBeHandled$str(), beanArchiveRef, handlers);
}
protected String beanArchiveReferenceCannotBeHandled$str() {
return "WELD-ENV-000031: The bean archive reference {0} cannot be handled by any BeanArchiveHandler: {1}";
}
@Override
public final void processingBeanArchiveReference(final Object beanArchiveRef) {
super.log.logv(FQCN, DEBUG, null, processingBeanArchiveReference$str(), beanArchiveRef);
}
protected String processingBeanArchiveReference$str() {
return "WELD-ENV-000032: Processing bean archive reference: {0}";
}
protected String invalidScanningResult$str() {
return "WELD-ENV-000033: Invalid bean archive scanning result - found multiple results with the same reference: {0}";
}
@Override
public final IllegalStateException invalidScanningResult(final Object beanArchiveRef) {
final IllegalStateException result = new IllegalStateException(_formatMessage(invalidScanningResult$str(), beanArchiveRef));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotScanClassPathEntry$str() {
return "WELD-ENV-000034: Cannot scan class path entry: {0}";
}
@Override
public final IllegalStateException cannotScanClassPathEntry(final Object entry, final Throwable cause) {
final IllegalStateException result = new IllegalStateException(_formatMessage(cannotScanClassPathEntry$str(), entry), cause);
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotReadClassPathEntry$str() {
return "WELD-ENV-000035: Class path entry cannot be read: {0}";
}
@Override
public final IllegalStateException cannotReadClassPathEntry(final Object entry) {
final IllegalStateException result = new IllegalStateException(_formatMessage(cannotReadClassPathEntry$str(), entry));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotReadJavaClassPathSystemProperty$str() {
return "WELD-ENV-000036: Weld cannot read the java class path system property!";
}
@Override
public final IllegalStateException cannotReadJavaClassPathSystemProperty() {
final IllegalStateException result = new IllegalStateException(cannotReadJavaClassPathSystemProperty$str());
_copyStackTraceMinusOne(result);
return result;
}
@Override
public final void beanArchiveReferenceHandled(final Object beanArchiveRef, final Object handler) {
super.log.logv(FQCN, DEBUG, null, beanArchiveReferenceHandled$str(), beanArchiveRef, handler);
}
protected String beanArchiveReferenceHandled$str() {
return "WELD-ENV-000039: Bean archive reference {0} handled by {1}";
}
@Override
public final void jandexDiscoveryStrategyDisabled() {
super.log.logv(FQCN, INFO, null, jandexDiscoveryStrategyDisabled$str());
}
protected String jandexDiscoveryStrategyDisabled$str() {
return "WELD-ENV-000040: Jandex discovery strategy was disabled.";
}
@Override
public final void usingServiceLoaderSourcedDiscoveryStrategy(final Object discoveryStrategy) {
super.log.logv(FQCN, INFO, null, usingServiceLoaderSourcedDiscoveryStrategy$str(), discoveryStrategy);
}
protected String usingServiceLoaderSourcedDiscoveryStrategy$str() {
return "WELD-ENV-000041: Using {0} for bean discovery";
}
@Override
public final void classPathEntryDoesNotExist(final Object entry) {
super.log.logv(FQCN, WARN, null, classPathEntryDoesNotExist$str(), entry);
}
protected String classPathEntryDoesNotExist$str() {
return "WELD-ENV-000042: Class path entry does not exist: {0}";
}
@Override
public final void catchingDebug(final Throwable throwable) {
super.log.logf(FQCN, DEBUG, throwable, catchingDebug$str());
}
protected String catchingDebug$str() {
return "Catching";
}
@Override
public final void catchingTrace(final Throwable throwable) {
super.log.logf(FQCN, TRACE, throwable, catchingTrace$str());
}
protected String catchingTrace$str() {
return "Catching";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy