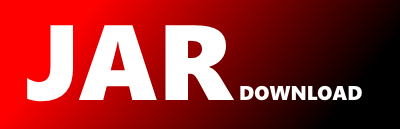
org.jboss.weld.invokable.CleanupActions Maven / Gradle / Ivy
package org.jboss.weld.invokable;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
import jakarta.enterprise.context.Dependent;
import jakarta.enterprise.inject.Instance;
class CleanupActions implements Consumer {
private final List cleanupTasks = new ArrayList<>();
private final List> dependentInstances = new ArrayList<>();
@Override
public void accept(Runnable runnable) {
cleanupTasks.add(runnable);
}
public void addInstanceHandle(Instance.Handle> handle) {
if (handle.getBean().getScope().equals(Dependent.class)) {
dependentInstances.add(handle);
}
}
public void cleanup() {
// run all registered tasks
for (Runnable r : cleanupTasks) {
r.run();
}
cleanupTasks.clear();
// destroy dependent beans we created
for (Instance.Handle> handle : dependentInstances) {
handle.destroy();
}
dependentInstances.clear();
}
// this signature fits into the `MethodHandles.tryFinally()` combinator in case of non-`void` methods
public static R run(Throwable cause, R returnValue, CleanupActions cleanupActions) {
cleanupActions.cleanup();
return returnValue;
}
// this signature fits into the `MethodHandles.tryFinally()` combinator in case of `void` methods
public static void run(Throwable cause, CleanupActions cleanupActions) {
cleanupActions.cleanup();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy