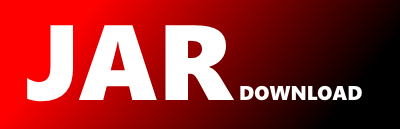
com.tinkerpop.frames.modules.javahandler.JavaHandlerContext Maven / Gradle / Ivy
Go to download
Windup Frames is an extension of the upstream Frames project, with tools to ease debugging and integration within windup.
package com.tinkerpop.frames.modules.javahandler;
import com.tinkerpop.blueprints.Direction;
import com.tinkerpop.blueprints.Edge;
import com.tinkerpop.blueprints.Element;
import com.tinkerpop.blueprints.Vertex;
import com.tinkerpop.frames.FramedGraph;
import com.tinkerpop.gremlin.java.GremlinPipeline;
/**
* By implementing this interface your Java handler implementation can access the underlying graph and the framed vertex/edge.
*
* @author Bryn Cooke
*
* @param
*/
public interface JavaHandlerContext {
/**
* @return The framed graph
*/
public FramedGraph> g();
/**
* @return The element that was framed
*/
public C it();
/**
* @return A gremlin pipeline at the context element
*/
public GremlinPipeline gremlin();
/**
* Start a gremlin pipeline
*
* @param starts
* @return Start a gremlin pipeline at an element
*/
public GremlinPipeline gremlin(Object starts);
/**
* Frame a vertex using the return type of the method
*
* @param vertex The vertex to frame
* @return The framed vertex
*/
public T frame(Vertex vertex);
/**
* Frame a vertex using an explicit kind of frame
*
* @param vertex The vertex to frame
* @param kind The type of frame
* @return The framed vertex
*/
public T frame(Vertex vertex, Class kind);
/**
* Frame an edge using the return type of the method
*
* @param edge The edge to frame
* @return The framed edge
*/
public T frame(Edge edge);
/**
* Frame an edge using an explicit kind of frame
*
* @param edge The edge to frame
* @param kind The type of frame
* @return The framed edge
*/
public T frame(Edge edge, Class kind);
/**
* Frame an edge using the return type of the method
*
* @param edge The edge to frame
* @param direction The direction of the edge
* @return The framed edge
*/
public T frame(Edge edge, Direction direction);
/**
* Frame an edge using an explicit kind of frame
*
* @param edge The edge to frame
* @param direction The direction of the edge
* @param kind The type of frame
* @return The framed edge
*/
public T frame(Edge edge, Direction direction, Class kind);
/**
* Frame some vertices using the return type of the method
*
* @param vertices The vertices to frame
* @return The framed vertices
*/
public Iterable frameVertices(Iterable vertices);
/**
* Frame some vertices using an explicit kind of frame
*
* @param vertices The vertices to frame
* @param kind The kind of frame
* @return The framed vertices
*/
public Iterable frameVertices(Iterable vertices, Class kind);
/**
* Frame some edges using the return type of the method
*
* @param edges the edges to frame
* @param direction The direction of the edges
* @return The framed edges
*/
public Iterable frameEdges(Iterable edges);
/**
* Frame some edges using an explicit kind of frame
*
* @param edges the edges to frame
* @param direction The direction of the edges
* @param kind The kind of frame
* @return The framed edges
*/
public Iterable frameEdges(Iterable edges, Class kind);
/**
* Frame some edges using the return type of the method
*
* @param edges the edges to frame
* @param direction The direction of the edges
* @return The framed edges
*/
public Iterable frameEdges(Iterable edges, Direction direction);
/**
* Frame some edges using an explicit kind of frame
*
* @param edges the edges to frame
* @param direction The direction of the edges
* @param kind The kind of frame
* @return The framed edges
*/
public Iterable frameEdges(Iterable edges, Direction direction, Class kind);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy