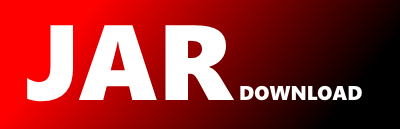
com.tinkerpop.frames.modules.javahandler.JavaHandlerContextImpl Maven / Gradle / Ivy
Go to download
Windup Frames is an extension of the upstream Frames project, with tools to ease debugging and integration within windup.
package com.tinkerpop.frames.modules.javahandler;
import java.lang.reflect.Method;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import com.tinkerpop.blueprints.Direction;
import com.tinkerpop.blueprints.Edge;
import com.tinkerpop.blueprints.Element;
import com.tinkerpop.blueprints.Vertex;
import com.tinkerpop.frames.ClassUtilities;
import com.tinkerpop.frames.FramedGraph;
import com.tinkerpop.gremlin.java.GremlinPipeline;
/**
* Implementation for java handler context.
*
* @author Bryn Cooke
*
*/
class JavaHandlerContextImpl implements JavaHandlerContext {
private final FramedGraph> graph;
private final Method method;
private final C context;
JavaHandlerContextImpl(FramedGraph> graph, Method method, C context) {
super();
this.graph = graph;
this.method = method;
this.context = context;
}
/**
* @return The framed graph
*/
public FramedGraph> g() {
return graph;
}
/**
* @return The element that was framed
*/
public C it() {
return context;
}
/**
* @return A gremlin pipeline at the context element
*/
public GremlinPipeline gremlin() {
return new GremlinPipeline(it());
}
/**
* Start a gremlin pipeline
*
* @param starts
* @return Start a gremlin pipeline at an element
*/
public GremlinPipeline gremlin(Object starts) {
return new GremlinPipeline(starts);
}
/**
* Frame a vertex using the return type of the method
*
* @param vertex The vertex to frame
* @return The framed vertex
*/
public T frame(Vertex vertex) {
return g().frame(vertex, (Class) method.getReturnType());
}
/**
* Frame a vertex using an explicit kind of frame
*
* @param vertex The vertex to frame
* @param kind The type of frame
* @return The framed vertex
*/
public T frame(Vertex vertex, Class kind) {
return g().frame(vertex, kind);
}
/**
* Frame an edge using the return type of the method
*
* @param edge The edge to frame
* @param direction The direction of the edge
* @return The framed edge
*/
public T frame(Edge edge, Direction direction) {
return g().frame(edge, direction, (Class) method.getReturnType());
}
/**
* Frame an edge using an explicit kind of frame
*
* @param edge The edge to frame
* @param direction The direction of the edge
* @param kind The type of frame
* @return The framed edge
*/
public T frame(Edge edge, Direction direction, Class kind) {
return (T) g().frame(edge, direction, kind);
}
/**
* Frame some vertices using the return type of the method
*
* @param vertices The vertices to frame
* @return The framed vertices
*/
public Iterable frameVertices(Iterable vertices) {
Type type = getIterableType();
return g().frameVertices(vertices, (Class) type);
}
/**
* Frame some vertices using an explicit kind of frame
*
* @param vertices The vertices to frame
* @param kind The kind of frame
* @return The framed vertices
*/
public Iterable frameVertices(Iterable vertices, Class kind) {
return (Iterable) g().frameVertices(vertices, kind);
}
/**
* Frame some edges using the return type of the method
*
* @param edges the edges to frame
* @param direction The direction of the edges
* @return The framed edges
*/
public Iterable frameEdges(Iterable edges, Direction direction) {
Type type = getIterableType();
return g().frameEdges(edges, direction, (Class) type);
}
/**
* Frame some edges using an explicit kind of frame
*
* @param edges the edges to frame
* @param direction The direction of the edges
* @param kind The kind of frame
* @return The framed edges
*/
public Iterable frameEdges(Iterable edges, Direction direction, Class kind) {
return (Iterable) g().frameEdges(edges, direction, kind);
}
private Type getIterableType() {
if(method.getReturnType() != Iterable.class) {
throw new JavaHandlerException("Method return type is not iterable: " + method);
}
Type genericReturnType = method.getGenericReturnType();
if(!(genericReturnType instanceof ParameterizedType)) {
throw new JavaHandlerException("Method must specify generic parameter for Iterable: " + method);
}
return ClassUtilities.getGenericClass(method);
}
@Override
public T frame(Edge edge) {
return g().frame(edge, (Class) method.getReturnType());
}
@Override
public T frame(Edge edge, Class kind) {
return g().frame(edge, kind);
}
@Override
public Iterable frameEdges(Iterable edges) {
Type type = getIterableType();
return g().frameEdges(edges, (Class) type);
}
@Override
public Iterable frameEdges(Iterable edges, Class kind) {
return g().frameEdges(edges, kind);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy