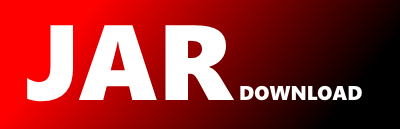
com.tinkerpop.frames.structures.FramedVertexMap Maven / Gradle / Ivy
Go to download
Windup Frames is an extension of the upstream Frames project, with tools to ease debugging and integration within windup.
package com.tinkerpop.frames.structures;
import com.tinkerpop.blueprints.Graph;
import com.tinkerpop.blueprints.Vertex;
import com.tinkerpop.frames.FramedGraph;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
/**
* @author Marko A. Rodriguez (http://markorodriguez.com)
*/
public class FramedVertexMap implements Map {
private final Map map;
protected final Class kind;
protected final FramedGraph extends Graph> framedGraph;
public FramedVertexMap(final FramedGraph extends Graph> framedGraph, final Map map, final Class kind) {
this.framedGraph = framedGraph;
this.map = map;
this.kind = kind;
}
public Object get(final Object key) {
return this.map.get(key);
}
public Object remove(final Object key) {
return this.map.remove(key);
}
public int size() {
return this.map.size();
}
public boolean isEmpty() {
return this.map.isEmpty();
}
public Object put(final T key, final Object value) {
throw new UnsupportedOperationException();
}
public void putAll(final Map otherMap) {
throw new UnsupportedOperationException();
}
public boolean containsValue(final Object value) {
return this.map.containsValue(value);
}
public boolean containsKey(final Object key) {
return this.map.containsKey(key);
}
public Set keySet() {
return new FramedVertexSet(framedGraph, this.map.keySet(), kind);
}
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy