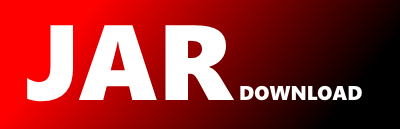
quarkus.jdbc.jdbc-to-quarkus.windup.groovy Maven / Gradle / Ivy
The newest version!
package quarkus.jdbc
import org.jboss.windup.config.GraphRewrite
import org.jboss.windup.config.metadata.TechnologyReference
import org.jboss.windup.config.operation.iteration.AbstractIterationOperation
import org.jboss.windup.config.query.Query
import org.jboss.windup.config.query.QueryPropertyComparisonType
import org.jboss.windup.graph.model.FileLocationModel
import org.jboss.windup.graph.model.WindupConfigurationModel
import org.jboss.windup.graph.model.resource.FileModel
import org.jboss.windup.graph.service.GraphService
import org.jboss.windup.graph.service.WindupConfigurationService
import org.jboss.windup.reporting.category.IssueCategory
import org.jboss.windup.reporting.category.IssueCategoryRegistry
import org.jboss.windup.reporting.config.Hint
import org.jboss.windup.reporting.config.Link
import org.jboss.windup.rules.apps.java.condition.SourceMode
import org.ocpsoft.rewrite.context.EvaluationContext
final IssueCategory mandatoryIssueCategory = new IssueCategoryRegistry().getByID(IssueCategoryRegistry.MANDATORY)
ruleSet("jdbc-to-quarkus-groovy")
.addSourceTechnology(new TechnologyReference("jdbc", null))
.addTargetTechnology(new TechnologyReference("quarkus", null))
.addRule()
.when(SourceMode.isDisabled(),
Query.fromType(FileModel)
.withProperty(FileModel.IS_DIRECTORY, Boolean.TRUE)
.withProperty(FileModel.FILE_PATH, QueryPropertyComparisonType.REGEX, ".*/org/mariadb/jdbc\$"))
.perform(new AbstractIterationOperation() {
void perform(GraphRewrite event, EvaluationContext context, FileModel payload) {
final String sourceBasePath = payload.getFilePath().replaceAll("/org/mariadb/jdbc\$", "")
final String dependencyJarName = sourceBasePath.substring(sourceBasePath.lastIndexOf("/") + 1)
WindupConfigurationModel windupConfigurationModel = WindupConfigurationService.getConfigurationModel(event.getGraphContext())
boolean packageComesFromAnalyzedApplication = false
windupConfigurationModel.getInputPaths().each {
if (!packageComesFromAnalyzedApplication && it.filePath.endsWith(dependencyJarName)) packageComesFromAnalyzedApplication = true
}
if (!packageComesFromAnalyzedApplication) return
final String targetFolderPath = sourceBasePath +"/io/quarkus/jdbc/mariadb/runtime"
final boolean foundQuarkusExtensionFolder = Query.fromType(FileModel)
.withProperty(FileModel.IS_DIRECTORY, Boolean.TRUE)
.withProperty(FileModel.FILE_PATH, targetFolderPath).as("target_folder").evaluate(event, context)
if (foundQuarkusExtensionFolder) return
final GraphService fileLocationService = new GraphService<>(event.getGraphContext(), FileLocationModel.class)
final FileLocationModel folderLocationModel = fileLocationService.create()
folderLocationModel.setFile(payload)
folderLocationModel.setColumnNumber(1)
folderLocationModel.setLineNumber(1)
folderLocationModel.setLength(1)
folderLocationModel.setSourceSnippit("Folder Match")
((Hint) Hint.titled("Replace the 'mariadb-java-client' dependency with Quarkus 'quarkus-jdbc-mariadb' extension")
.withText("""A folder path related to a package from the `org.mariadb.jdbc:mariadb-java-client` dependency has been found.
Replace the `org.mariadb.jdbc:mariadb-java-client` dependency with the Quarkus dependency `io.quarkus:quarkus-jdbc-mariadb` in the application's dependencies management system (Maven, Gradle).
Further information in the link below.""")
.withIssueCategory(mandatoryIssueCategory)
.with(Link.to("Quarkus - Guide", "https://quarkus.io/guides/hibernate-orm"))
.withEffort(1)
).performParameterized(event, context, folderLocationModel)
}
})
.withId("quarkus-jdbc-mariadb-groovy-00000")
.addRule()
.when(SourceMode.isDisabled(),
Query.fromType(FileModel)
.withProperty(FileModel.IS_DIRECTORY, Boolean.TRUE)
.withProperty(FileModel.FILE_PATH, QueryPropertyComparisonType.REGEX, ".*/oracle/jdbc\$"))
.perform(new AbstractIterationOperation() {
void perform(GraphRewrite event, EvaluationContext context, FileModel payload) {
final String sourceBasePath = payload.getFilePath().replaceAll("/oracle/jdbc\$", "")
final String dependencyJarName = sourceBasePath.substring(sourceBasePath.lastIndexOf("/") + 1)
WindupConfigurationModel windupConfigurationModel = WindupConfigurationService.getConfigurationModel(event.getGraphContext())
boolean packageComesFromAnalyzedApplication = false
windupConfigurationModel.getInputPaths().each {
if (!packageComesFromAnalyzedApplication && it.filePath.endsWith(dependencyJarName)) packageComesFromAnalyzedApplication = true
}
if (!packageComesFromAnalyzedApplication) return
final String targetFolderPath = sourceBasePath +"/io/quarkus/jdbc/oracle/runtime"
final boolean foundQuarkusExtensionFolder = Query.fromType(FileModel)
.withProperty(FileModel.IS_DIRECTORY, Boolean.TRUE)
.withProperty(FileModel.FILE_PATH, targetFolderPath).as("target_folder").evaluate(event, context)
if (foundQuarkusExtensionFolder) return
final GraphService fileLocationService = new GraphService<>(event.getGraphContext(), FileLocationModel.class)
final FileLocationModel folderLocationModel = fileLocationService.create()
folderLocationModel.setFile(payload)
folderLocationModel.setColumnNumber(1)
folderLocationModel.setLineNumber(1)
folderLocationModel.setLength(1)
folderLocationModel.setSourceSnippit("Folder Match")
((Hint) Hint.titled("Replace the 'ojdbc11' dependency with Quarkus 'quarkus-jdbc-oracle' extension")
.withText("""A folder path related to a package from the `com.oracle.database.jdbc:ojdbc11` dependency has been found.
Replace the `com.oracle.database.jdbc:ojdbc11` dependency with the Quarkus dependency `io.quarkus:quarkus-jdbc-oracle` in the application's dependencies management system (Maven, Gradle).
Further information in the link below.""")
.withIssueCategory(mandatoryIssueCategory)
.with(Link.to("Quarkus - Guide", "https://quarkus.io/guides/hibernate-orm"))
.withEffort(1)
).performParameterized(event, context, folderLocationModel)
}
})
.withId("quarkus-jdbc-oracle-groovy-00000")
.addRule()
.when(SourceMode.isDisabled(),
Query.fromType(FileModel)
.withProperty(FileModel.IS_DIRECTORY, Boolean.TRUE)
.withProperty(FileModel.FILE_PATH, QueryPropertyComparisonType.REGEX, ".*/org/postgresql\$"))
.perform(new AbstractIterationOperation() {
void perform(GraphRewrite event, EvaluationContext context, FileModel payload) {
final String sourceBasePath = payload.getFilePath().replaceAll("/org/postgresql\$", "")
final String dependencyJarName = sourceBasePath.substring(sourceBasePath.lastIndexOf("/") + 1)
WindupConfigurationModel windupConfigurationModel = WindupConfigurationService.getConfigurationModel(event.getGraphContext())
boolean packageComesFromAnalyzedApplication = false
windupConfigurationModel.getInputPaths().each {
if (!packageComesFromAnalyzedApplication && it.filePath.endsWith(dependencyJarName)) packageComesFromAnalyzedApplication = true
}
if (!packageComesFromAnalyzedApplication) return
final String targetFolderPath = sourceBasePath +"/io/quarkus/jdbc/postgresql/runtime"
final boolean foundQuarkusExtensionFolder = Query.fromType(FileModel)
.withProperty(FileModel.IS_DIRECTORY, Boolean.TRUE)
.withProperty(FileModel.FILE_PATH, targetFolderPath).as("target_folder").evaluate(event, context)
if (foundQuarkusExtensionFolder) return
final GraphService fileLocationService = new GraphService<>(event.getGraphContext(), FileLocationModel.class)
final FileLocationModel folderLocationModel = fileLocationService.create()
folderLocationModel.setFile(payload)
folderLocationModel.setColumnNumber(1)
folderLocationModel.setLineNumber(1)
folderLocationModel.setLength(1)
folderLocationModel.setSourceSnippit("Folder Match")
((Hint) Hint.titled("Replace the 'postgresql' dependency with Quarkus 'quarkus-jdbc-postgresql' extension")
.withText("""A folder path related to a package from the `org.postgresql:postgresql` dependency has been found.
Replace the `org.postgresql:postgresql` dependency with the Quarkus dependency `io.quarkus:quarkus-jdbc-postgresql` in the application's dependencies management system (Maven, Gradle).
Further information in the link below.""")
.withIssueCategory(mandatoryIssueCategory)
.with(Link.to("Quarkus - Guide", "https://quarkus.io/guides/hibernate-orm"))
.withEffort(1)
).performParameterized(event, context, folderLocationModel)
}
})
.withId("quarkus-jdbc-postgresql-groovy-00000")
© 2015 - 2025 Weber Informatics LLC | Privacy Policy