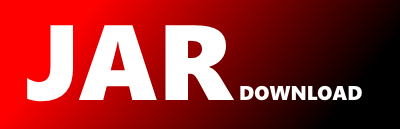
org.jboss.ws.tools.cmd.WSConsume Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source.
* Copyright 2006, Red Hat Middleware LLC, and individual contributors
* as indicated by the @author tags. See the copyright.txt file in the
* distribution for a full listing of individual contributors.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.jboss.ws.tools.cmd;
import gnu.getopt.Getopt;
import gnu.getopt.LongOpt;
import org.apache.log4j.Logger;
import org.apache.log4j.Level;
import org.jboss.ws.api.tools.WSContractConsumer;
import java.io.File;
import java.io.PrintStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
/**
* WSConsumeTask is a cmd line tool that generates portable JAX-WS artifacts
* from a WSDL file.
*
*
* usage: WSConsumeTask [options] <wsdl-url>
* options:
*
* -h, --help Show this help message
* -b, --binding=<file> One or more JAX-WS or JAXB binding files
* -k, --keep Keep/Generate Java source
* -c, --catalog=<file> Oasis XML Catalog file for entity resolution
* -p, --package=<name> The target package for generated source
* -j, --clientjar=<name> Create a jar file of the generated artifacts for calling the webservice
* -w, --wsdlLocation=<loc> Value to use for @@WebService.wsdlLocation
* -o, --output=<directory> The directory to put generated artifacts
* -s, --source=<directory> The directory to put Java source
* -t, --target=<2.1|2.2> The target specification target
* -n, --nocompile Do not compile generated sources
* -q, --quiet Be somewhat more quiet
* -v, --verbose Show full exception stack traces
* -l, --load-consumer Load the consumer and exit (debug utility)
* -e, --extension Enable SOAP 1.2 binding extension
* -a, --additionalHeaders Enable SOAP 1.2 binding extension
*
*
*
* @author Jason T. Greene
*/
public class WSConsume
{
private List© 2015 - 2025 Weber Informatics LLC | Privacy Policy