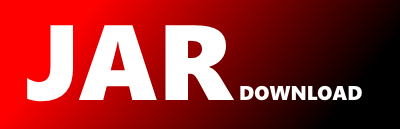
org.jboss.ws.common.Messages_$bundle Maven / Gradle / Ivy
The newest version!
package org.jboss.ws.common;
import java.util.Locale;
import java.lang.IllegalStateException;
import java.io.Serializable;
import java.net.MalformedURLException;
import java.lang.String;
import org.jboss.wsf.spi.metadata.j2ee.serviceref.UnifiedServiceRefMetaData;
import org.jboss.ws.common.injection.InjectionException;
import org.jboss.wsf.spi.deployment.EndpointState;
import java.lang.reflect.Field;
import java.lang.RuntimeException;
import java.lang.ClassLoader;
import org.jboss.wsf.spi.WSFException;
import org.jboss.wsf.spi.deployment.WSFDeploymentException;
import java.lang.IllegalArgumentException;
import javax.annotation.processing.Generated;
import jakarta.xml.ws.WebServiceException;
import java.lang.Error;
import java.io.IOException;
import java.lang.reflect.Method;
import javax.management.ObjectName;
import java.lang.Throwable;
import java.util.Collection;
import java.lang.Class;
import java.lang.Object;
import java.util.Arrays;
/**
* Warning this class consists of generated code.
*/
@Generated(value = "org.jboss.logging.processor.generator.model.MessageBundleImplementor", date = "2024-12-11T10:35:49+0800")
public class Messages_$bundle implements Messages, Serializable {
private static final long serialVersionUID = 1L;
protected Messages_$bundle() {}
public static final Messages_$bundle INSTANCE = new Messages_$bundle();
protected Object readResolve() {
return INSTANCE;
}
private static final Locale LOCALE = Locale.ROOT;
protected Locale getLoggingLocale() {
return LOCALE;
}
protected String cannotGetURLFor$str() {
return "JBWS022000: Cannot get URL for %s";
}
@Override
public final IOException cannotGetURLFor(final String path) {
final IOException result = new IOException(String.format(getLoggingLocale(), cannotGetURLFor$str(), path));
_copyStackTraceMinusOne(result);
return result;
}
private static void _copyStackTraceMinusOne(final Throwable e) {
final StackTraceElement[] st = e.getStackTrace();
e.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
}
protected String unifiedVirtualFileNotInitialized$str() {
return "JBWS022003: UnifiedVirtualFile not initialized; could not load resources from classloader: %s";
}
@Override
public final IllegalStateException unifiedVirtualFileNotInitialized(final ClassLoader cl) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), unifiedVirtualFileNotInitialized$str(), cl));
_copyStackTraceMinusOne(result);
return result;
}
protected String invalidObjectName$str() {
return "JBWS022004: Invalid ObjectName: %s";
}
@Override
public final Error invalidObjectName(final Throwable cause, final String message) {
final Error result = new Error(String.format(getLoggingLocale(), invalidObjectName$str(), message), cause);
_copyStackTraceMinusOne(result);
return result;
}
protected String invalidBinaryComponentForArray$str() {
return "JBWS022005: Could not get component type from array; invalid binary component for array: %s";
}
@Override
public final IllegalArgumentException invalidBinaryComponentForArray(final String arrayType) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), invalidBinaryComponentForArray$str(), arrayType));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotCheckClassIsAssignableFrom$str() {
return "JBWS022006: Could not check whether class '%s' is assignable from class '%s'; both classes must not be null.";
}
@Override
public final IllegalArgumentException cannotCheckClassIsAssignableFrom(final Class> dest, final Class> src) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), cannotCheckClassIsAssignableFrom$str(), dest, src));
_copyStackTraceMinusOne(result);
return result;
}
protected String unableToConvertDataHandler$str() {
return "JBWS022007: Unable to convert DataHandler to byte[]: %s";
}
@Override
public final WebServiceException unableToConvertDataHandler(final Throwable cause, final String dhName) {
final WebServiceException result = new WebServiceException(String.format(getLoggingLocale(), unableToConvertDataHandler$str(), dhName), cause);
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotPrettyPrintAndIgnoreWhiteSpaces$str() {
return "JBWS022008: Cannot pretty print document and ignore white spaces at the same time";
}
@Override
public final IllegalStateException cannotPrettyPrintAndIgnoreWhiteSpaces() {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), cannotPrettyPrintAndIgnoreWhiteSpaces$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String unableToCreateInstanceOf$str() {
return "JBWS022009: Unable to create instance of %s";
}
@Override
public final RuntimeException unableToCreateInstanceOf(final Throwable cause, final String className) {
final RuntimeException result = new RuntimeException(String.format(getLoggingLocale(), unableToCreateInstanceOf$str(), className), cause);
_copyStackTraceMinusOne(result);
return result;
}
protected String sourceTypeNotImplemented$str() {
return "JBWS022014: Source type not implemented: %s";
}
@Override
public final RuntimeException sourceTypeNotImplemented(final Class> clazz) {
final RuntimeException result = new RuntimeException(String.format(getLoggingLocale(), sourceTypeNotImplemented$str(), clazz));
_copyStackTraceMinusOne(result);
return result;
}
protected String entityResolutionInvalidCharacterReference$str() {
return "JBWS022015: Entity resolution: invalid character reference in %s";
}
@Override
public final IllegalArgumentException entityResolutionInvalidCharacterReference(final String string) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), entityResolutionInvalidCharacterReference$str(), string));
_copyStackTraceMinusOne(result);
return result;
}
protected String entityResolutionInvalidEntityReference$str() {
return "JBWS022016: Entity resolution: invalid entity reference in %s";
}
@Override
public final IllegalArgumentException entityResolutionInvalidEntityReference(final String string) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), entityResolutionInvalidEntityReference$str(), string));
_copyStackTraceMinusOne(result);
return result;
}
protected String entityResolutionInvalidEntity$str() {
return "JBWS022017: Entity resolution: invalid entity %s";
}
@Override
public final IllegalArgumentException entityResolutionInvalidEntity(final String entity) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), entityResolutionInvalidEntity$str(), entity));
_copyStackTraceMinusOne(result);
return result;
}
protected String uuidMustBeOf16Bytes$str() {
return "JBWS022018: A UUID must be 16 bytes!";
}
@Override
public final IllegalArgumentException uuidMustBeOf16Bytes() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), uuidMustBeOf16Bytes$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String entityResolutionNoEntityMapppingDefined$str() {
return "JBWS022019: Entity resolution: no entities mapping defined in resource file: %s";
}
@Override
public final IllegalArgumentException entityResolutionNoEntityMapppingDefined(final String file) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), entityResolutionNoEntityMapppingDefined$str(), file));
_copyStackTraceMinusOne(result);
return result;
}
protected String entityResolutionResourceNotFound$str() {
return "JBWS022020: Entity resolution: resource not found: %s";
}
@Override
public final IllegalArgumentException entityResolutionResourceNotFound(final String res) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), entityResolutionResourceNotFound$str(), res));
_copyStackTraceMinusOne(result);
return result;
}
protected String jarUrlConnectionBuildError$str() {
return "JBWS022023: Error building an URLConnection capable of handling multiply-nested jars; '!' missing or in unexpected position in provided url: %s";
}
@Override
public final MalformedURLException jarUrlConnectionBuildError(final String url) {
final MalformedURLException result = new MalformedURLException(String.format(getLoggingLocale(), jarUrlConnectionBuildError$str(), url));
_copyStackTraceMinusOne(result);
return result;
}
protected String jarUrlConnectionUnableToLocateSegment$str() {
return "JBWS022024: JAR URLConnection unable to locate segment %s for url %s";
}
@Override
public final IOException jarUrlConnectionUnableToLocateSegment(final String segment, final String url) {
final IOException result = new IOException(String.format(getLoggingLocale(), jarUrlConnectionUnableToLocateSegment$str(), segment, url));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotFindSchemaImportInDeployment$str() {
return "JBWS022028: Cannot find schema import '%s' in deployment '%s'";
}
@Override
public final IllegalArgumentException cannotFindSchemaImportInDeployment(final String xsdImport, final String deploymentName) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), cannotFindSchemaImportInDeployment$str(), xsdImport, deploymentName));
_copyStackTraceMinusOne(result);
return result;
}
protected String failedToProvideSPI$str() {
return "JBWS022029: Failed to provide spi: %s";
}
@Override
public final WSFException failedToProvideSPI(final Class> spiType) {
final WSFException result = new WSFException(String.format(getLoggingLocale(), failedToProvideSPI$str(), spiType));
_copyStackTraceMinusOne(result);
return result;
}
protected String noDeploymentAspectFoundWithAttributeLast$str() {
return "JBWS022030: No deployment aspect found with attribute last='true'";
}
@Override
public final IllegalStateException noDeploymentAspectFoundWithAttributeLast() {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), noDeploymentAspectFoundWithAttributeLast$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String cycleDetectedInSubGraph$str() {
return "JBWS022031: Cycle detected in sub-graph: %s";
}
@Override
public final IllegalStateException cycleDetectedInSubGraph(final Collection> c) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), cycleDetectedInSubGraph$str(), c));
_copyStackTraceMinusOne(result);
return result;
}
protected String missingVFSRootInServiceRef$str() {
return "JBWS022034: Missing VFS root for service-ref: %s";
}
@Override
public final IllegalStateException missingVFSRootInServiceRef(final String serviceRefName) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), missingVFSRootInServiceRef$str(), serviceRefName));
_copyStackTraceMinusOne(result);
return result;
}
protected String missingServiceRefTypeInServiceRef$str() {
return "JBWS022035: Missing service reference type for service-ref: %s";
}
@Override
public final IllegalStateException missingServiceRefTypeInServiceRef(final String serviceRefName) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), missingServiceRefTypeInServiceRef$str(), serviceRefName));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotCreateServiceWithoutWsdlLocation$str() {
return "JBWS022036: Cannot create generic jakarta.xml.ws.Service without wsdlLocation; service-ref metadata = '%s'";
}
@Override
public final IllegalArgumentException cannotCreateServiceWithoutWsdlLocation(final UnifiedServiceRefMetaData serviceRefMD) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), cannotCreateServiceWithoutWsdlLocation$str(), serviceRefMD));
_copyStackTraceMinusOne(result);
return result;
}
protected String annotationClassCannotBeNull$str() {
return "JBWS022037: Annotation class cannot be null";
}
@Override
public final IllegalArgumentException annotationClassCannotBeNull() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), annotationClassCannotBeNull$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotFindAttachmentInDeployment$str() {
return "JBWS022062: Cannot find attachment %s in webservice deployment %s";
}
@Override
public final IllegalStateException cannotFindAttachmentInDeployment(final Class> attachmentClass, final String dep) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), cannotFindAttachmentInDeployment$str(), attachmentClass, dep));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotResolve$str() {
return "JBWS022063: Reference resolution: cannot resolve %s";
}
@Override
public final IllegalArgumentException cannotResolve(final Object obj) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), cannotResolve$str(), obj));
_copyStackTraceMinusOne(result);
return result;
}
protected String accessibleObjectClassCannotBeNull$str() {
return "JBWS022064: Reference resolution: accessible object class cannot be null";
}
@Override
public final IllegalArgumentException accessibleObjectClassCannotBeNull() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), accessibleObjectClassCannotBeNull$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodCannotDeclarePrimitiveParameters2$str() {
return "JBWS022065: Method %s annotated with @%s can't declare primitive parameters";
}
@Override
public final InjectionException methodCannotDeclarePrimitiveParameters2(final Method m, final Class extends java.lang.annotation.Annotation> ann) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodCannotDeclarePrimitiveParameters2$str(), m, ann));
_copyStackTraceMinusOne(result);
return result;
}
protected String fieldCannotBeOfPrimitiveOrVoidType$str() {
return "JBWS022066: Field %s can't be of primitive or void type";
}
@Override
public final InjectionException fieldCannotBeOfPrimitiveOrVoidType(final Field f) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), fieldCannotBeOfPrimitiveOrVoidType$str(), f));
_copyStackTraceMinusOne(result);
return result;
}
protected String fieldCannotBeOfPrimitiveOrVoidType2$str() {
return "JBWS022067: Method %s annotated with @%s can't be of primitive or void type";
}
@Override
public final InjectionException fieldCannotBeOfPrimitiveOrVoidType2(final Field f, final Class extends java.lang.annotation.Annotation> ann) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), fieldCannotBeOfPrimitiveOrVoidType2$str(), f, ann));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodHasToHaveNoParameters$str() {
return "JBWS022068: Method %s has to have no parameters";
}
@Override
public final InjectionException methodHasToHaveNoParameters(final Method m) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodHasToHaveNoParameters$str(), m));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodHasToHaveNoParameters2$str() {
return "JBWS022069: Method %s annotated with @%s has to have no parameters";
}
@Override
public final InjectionException methodHasToHaveNoParameters2(final Method m, final Class extends java.lang.annotation.Annotation> ann) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodHasToHaveNoParameters2$str(), m, ann));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodHasToReturnVoid$str() {
return "JBWS022070: Method %s has to return void";
}
@Override
public final InjectionException methodHasToReturnVoid(final Method m) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodHasToReturnVoid$str(), m));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodHasToReturnVoid2$str() {
return "JBWS022071: Method %s annotated with @%s has to return void";
}
@Override
public final InjectionException methodHasToReturnVoid2(final Method m, final Class extends java.lang.annotation.Annotation> ann) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodHasToReturnVoid2$str(), m, ann));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodCannotThrowCheckedException$str() {
return "JBWS022072: Method %s cannot throw checked exceptions";
}
@Override
public final InjectionException methodCannotThrowCheckedException(final Method m) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodCannotThrowCheckedException$str(), m));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodCannotThrowCheckedException2$str() {
return "JBWS022073: Method %s annotated with @%s cannot throw checked exception";
}
@Override
public final InjectionException methodCannotThrowCheckedException2(final Method m, final Class extends java.lang.annotation.Annotation> ann) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodCannotThrowCheckedException2$str(), m, ann));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodCannotBeStatic$str() {
return "JBWS022074: Method %s cannot be static";
}
@Override
public final InjectionException methodCannotBeStatic(final Method m) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodCannotBeStatic$str(), m));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodCannotBeStatic2$str() {
return "JBWS022075: Method %s annotated with @%s cannot be static";
}
@Override
public final InjectionException methodCannotBeStatic2(final Method m, final Class extends java.lang.annotation.Annotation> ann) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodCannotBeStatic2$str(), m, ann));
_copyStackTraceMinusOne(result);
return result;
}
protected String fieldCannotBeStaticOrFinal$str() {
return "JBWS022076: Field %s cannot be static or final";
}
@Override
public final InjectionException fieldCannotBeStaticOrFinal(final Field f) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), fieldCannotBeStaticOrFinal$str(), f));
_copyStackTraceMinusOne(result);
return result;
}
protected String fieldCannotBeStaticOrFinal2$str() {
return "JBWS022077: Field %s annotated with @%s cannot be static";
}
@Override
public final InjectionException fieldCannotBeStaticOrFinal2(final Field f, final Class extends java.lang.annotation.Annotation> ann) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), fieldCannotBeStaticOrFinal2$str(), f, ann));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodHasToDeclareExactlyOneParameter$str() {
return "JBWS022078: Method %s has to declare exactly one parameter";
}
@Override
public final InjectionException methodHasToDeclareExactlyOneParameter(final Method m) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodHasToDeclareExactlyOneParameter$str(), m));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodHasToDeclareExactlyOneParameter2$str() {
return "JBWS022079: Method %s annotated with @%s has to declare exactly one parameter";
}
@Override
public final InjectionException methodHasToDeclareExactlyOneParameter2(final Method m, final Class extends java.lang.annotation.Annotation> ann) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodHasToDeclareExactlyOneParameter2$str(), m, ann));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodDoesNotRespectJavaBeanSetterMethodName$str() {
return "JBWS022080: Method %s doesn't respect Java Beans setter method name";
}
@Override
public final InjectionException methodDoesNotRespectJavaBeanSetterMethodName(final Method m) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodDoesNotRespectJavaBeanSetterMethodName$str(), m));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodDoesNotRespectJavaBeanSetterMethodName2$str() {
return "JBWS022081: Method %s annotated with @%s doesn't respect Java Beans setter method name";
}
@Override
public final InjectionException methodDoesNotRespectJavaBeanSetterMethodName2(final Method m, final Class extends java.lang.annotation.Annotation> ann) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodDoesNotRespectJavaBeanSetterMethodName2$str(), m, ann));
_copyStackTraceMinusOne(result);
return result;
}
protected String onlyOneMethodCanExist$str() {
return "JBWS022082: Only one method can exist";
}
@Override
public final InjectionException onlyOneMethodCanExist() {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), onlyOneMethodCanExist$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String onlyOneMethodCanExist2$str() {
return "JBWS022083: Only one method annotated with @%s can exist";
}
@Override
public final InjectionException onlyOneMethodCanExist2(final Class extends java.lang.annotation.Annotation> ann) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), onlyOneMethodCanExist2$str(), ann));
_copyStackTraceMinusOne(result);
return result;
}
protected String methodCannotDeclarePrimitiveParameters$str() {
return "JBWS022084: Method %s can't declare primitive parameters";
}
@Override
public final InjectionException methodCannotDeclarePrimitiveParameters(final Method m) {
final InjectionException result = new InjectionException(String.format(getLoggingLocale(), methodCannotDeclarePrimitiveParameters$str(), m));
_copyStackTraceMinusOne(result);
return result;
}
protected String virtualHostMustBeTheSameForAllEndpoints$str() {
return "JBWS022085: Virtual host must be the same for all endpoints of the deployment %s";
}
@Override
public final IllegalStateException virtualHostMustBeTheSameForAllEndpoints(final String dep) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), virtualHostMustBeTheSameForAllEndpoints$str(), dep));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotObtainServletMapping$str() {
return "JBWS022086: Cannot obtain servlet mapping for %s";
}
@Override
public final IllegalStateException cannotObtainServletMapping(final String name) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), cannotObtainServletMapping$str(), name));
_copyStackTraceMinusOne(result);
return result;
}
protected String failedToRead$str() {
return "JBWS022087: Failed to read %s: %s";
}
@Override
public final WebServiceException failedToRead(final String descriptor, final String mex, final Throwable cause) {
final WebServiceException result = new WebServiceException(String.format(getLoggingLocale(), failedToRead$str(), descriptor, mex), cause);
_copyStackTraceMinusOne(result);
return result;
}
protected String unexpectedElement$str() {
return "JBWS022088: Unexpected element parsing %s: %s";
}
@Override
public final IllegalStateException unexpectedElement(final String descriptor, final String elem) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), unexpectedElement$str(), descriptor, elem));
_copyStackTraceMinusOne(result);
return result;
}
protected String reachedEndOfXMLDocUnexpectedly$str() {
return "JBWS022089: Unexpectedly reached end of XML document: %s";
}
@Override
public final IllegalStateException reachedEndOfXMLDocUnexpectedly(final String descriptor) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), reachedEndOfXMLDocUnexpectedly$str(), descriptor));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotResolveResource$str() {
return "JBWS022092: Could not resolve %s in deployment %s";
}
@Override
public final IOException cannotResolveResource(final String resourcePath, final String deploymentName) {
final IOException result = new IOException(String.format(getLoggingLocale(), cannotResolveResource$str(), resourcePath, deploymentName));
_copyStackTraceMinusOne(result);
return result;
}
protected String contextRootExpectedToStartWithLeadingSlash$str() {
return "JBWS022093: Context root expected to start with leading slash: %s";
}
@Override
public final IllegalStateException contextRootExpectedToStartWithLeadingSlash(final String contextRoot) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), contextRootExpectedToStartWithLeadingSlash$str(), contextRoot));
_copyStackTraceMinusOne(result);
return result;
}
protected String lifecycleHandlerNotInitialized$str() {
return "JBWS022094: Lifecycle handler not initialized for endpoint %s";
}
@Override
public final IllegalStateException lifecycleHandlerNotInitialized(final ObjectName epName) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), lifecycleHandlerNotInitialized$str(), epName));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotObtainContextRoot$str() {
return "JBWS022095: Cannot obtain context root for deployment %s";
}
@Override
public final IllegalStateException cannotObtainContextRoot(final String dep) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), cannotObtainContextRoot$str(), dep));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotObtainUrlPattern$str() {
return "JBWS022096: Cannot obtain url pattern for endpoint %s";
}
@Override
public final IllegalStateException cannotObtainUrlPattern(final ObjectName epName) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), cannotObtainUrlPattern$str(), epName));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotFindUrlPatternForServletName$str() {
return "JBWS022097: Cannot find for servlet-name %s";
}
@Override
public final RuntimeException cannotFindUrlPatternForServletName(final String s) {
final RuntimeException result = new RuntimeException(String.format(getLoggingLocale(), cannotFindUrlPatternForServletName$str(), s));
_copyStackTraceMinusOne(result);
return result;
}
protected String invocationHandlerNotAvailable$str() {
return "JBWS022101: Invocation handler not available for endpoint %s";
}
@Override
public final IllegalStateException invocationHandlerNotAvailable(final ObjectName epName) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), invocationHandlerNotAvailable$str(), epName));
_copyStackTraceMinusOne(result);
return result;
}
protected String allEndpointsMustShareSameContextRoot$str() {
return "JBWS022104: All endpoints must share the same context root; deployment = %s";
}
@Override
public final IllegalStateException allEndpointsMustShareSameContextRoot(final String dep) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), allEndpointsMustShareSameContextRoot$str(), dep));
_copyStackTraceMinusOne(result);
return result;
}
protected String cannotModifyEndpointInState$str() {
return "JBWS022105: Cannot modify endpoint in state %s: %s";
}
@Override
public final IllegalStateException cannotModifyEndpointInState(final EndpointState state, final ObjectName epName) {
final IllegalStateException result = new IllegalStateException(String.format(getLoggingLocale(), cannotModifyEndpointInState$str(), state, epName));
_copyStackTraceMinusOne(result);
return result;
}
protected String operationNotSupportedBy$str() {
return "JBWS022106: Operation %s not supported by %s";
}
@Override
public final RuntimeException operationNotSupportedBy(final String op, final Class> clazz) {
final RuntimeException result = new RuntimeException(String.format(getLoggingLocale(), operationNotSupportedBy$str(), op, clazz));
_copyStackTraceMinusOne(result);
return result;
}
protected String couldNotReadConfiguration$str() {
return "JBWS022107: Could not read configuration from %s";
}
@Override
public final RuntimeException couldNotReadConfiguration(final String path, final Throwable cause) {
final RuntimeException result = new RuntimeException(String.format(getLoggingLocale(), couldNotReadConfiguration$str(), path), cause);
_copyStackTraceMinusOne(result);
return result;
}
protected String configurationNotFound$str() {
return "JBWS022108: Configuration %s not found";
}
@Override
public final RuntimeException configurationNotFound(final String conf) {
final RuntimeException result = new RuntimeException(String.format(getLoggingLocale(), configurationNotFound$str(), conf));
_copyStackTraceMinusOne(result);
return result;
}
protected String notJAXWSHandler$str() {
return "JBWS022109: %s is not a JAX-WS Handler";
}
@Override
public final RuntimeException notJAXWSHandler(final String className) {
final RuntimeException result = new RuntimeException(String.format(getLoggingLocale(), notJAXWSHandler$str(), className));
_copyStackTraceMinusOne(result);
return result;
}
protected String invalidAddressProvided$str() {
return "JBWS022117: Invalid address provided: %s";
}
@Override
public final IllegalArgumentException invalidAddressProvided(final String address) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), invalidAddressProvided$str(), address));
_copyStackTraceMinusOne(result);
return result;
}
protected String couldNotReadConfigFile$str() {
return "JBWS022120: Could not read from config file: %s";
}
@Override
public final RuntimeException couldNotReadConfigFile(final String file) {
final RuntimeException result = new RuntimeException(String.format(getLoggingLocale(), couldNotReadConfigFile$str(), file));
_copyStackTraceMinusOne(result);
return result;
}
protected String couldNotFindEndpointConfigName$str() {
return "JBWS022121: Could not find endpoint config name: %s";
}
@Override
public final WSFDeploymentException couldNotFindEndpointConfigName(final String name) {
final WSFDeploymentException result = new WSFDeploymentException(String.format(getLoggingLocale(), couldNotFindEndpointConfigName$str(), name));
_copyStackTraceMinusOne(result);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy