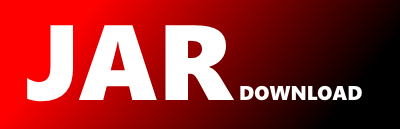
org.jboss.as.webservices.metadata.AbstractMetaDataBuilderPOJO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbossws-wildfly821-server-integration
Show all versions of jbossws-wildfly821-server-integration
JBossWS WildFly 8.2.1.Final Server Side Integration
The newest version!
/*
* JBoss, Home of Professional Open Source.
* Copyright 2014, Red Hat Middleware LLC, and individual contributors
* as indicated by the @author tags. See the copyright.txt file in the
* distribution for a full listing of individual contributors.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.jboss.as.webservices.metadata;
import static org.jboss.as.webservices.WSLogger.ROOT_LOGGER;
import static org.jboss.as.webservices.util.ASHelper.getContextRoot;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.jboss.as.server.deployment.DeploymentUnit;
import org.jboss.as.webservices.metadata.model.POJOEndpoint;
import org.jboss.as.webservices.util.WebMetaDataHelper;
import org.jboss.metadata.javaee.spec.ParamValueMetaData;
import org.jboss.metadata.web.jboss.JBossServletsMetaData;
import org.jboss.metadata.web.jboss.JBossWebMetaData;
import org.jboss.metadata.web.spec.SecurityConstraintMetaData;
import org.jboss.metadata.web.spec.ServletMappingMetaData;
import org.jboss.metadata.web.spec.WebResourceCollectionMetaData;
import org.jboss.ws.common.integration.WSConstants;
import org.jboss.ws.common.integration.WSHelper;
import org.jboss.wsf.spi.deployment.Deployment;
import org.jboss.wsf.spi.metadata.j2ee.JSEArchiveMetaData;
import org.jboss.wsf.spi.metadata.j2ee.JSESecurityMetaData;
import org.jboss.wsf.spi.metadata.j2ee.PublishLocationAdapter;
import org.jboss.wsf.spi.metadata.webservices.JBossWebservicesMetaData;
/**
* Builds container independent meta data from WEB container meta data.
*
* @author Richard Opalka
* @author Thomas Diesler
* @author Alessio Soldano
*/
abstract class AbstractMetaDataBuilderPOJO {
AbstractMetaDataBuilderPOJO() {
super();
}
/**
* Builds universal JSE meta data model that is AS agnostic.
*
* @param dep webservice deployment
* @return universal JSE meta data model
*/
JSEArchiveMetaData create(final Deployment dep) {
ROOT_LOGGER.creatingPojoDeployment(dep.getSimpleName());
final JBossWebMetaData jbossWebMD = WSHelper.getRequiredAttachment(dep, JBossWebMetaData.class);
final DeploymentUnit unit = WSHelper.getRequiredAttachment(dep, DeploymentUnit.class);
final List pojoEndpoints = getPojoEndpoints(unit);
final JSEArchiveMetaData.Builder jseArchiveMDBuilder = new JSEArchiveMetaData.Builder();
// set context root
final String contextRoot = getContextRoot(dep, jbossWebMD);
jseArchiveMDBuilder.setContextRoot(contextRoot);
ROOT_LOGGER.settingContextRoot(contextRoot);
// set servlet url patterns mappings
final Map servletMappings = getServletUrlPatternsMappings(jbossWebMD, pojoEndpoints);
jseArchiveMDBuilder.setServletMappings(servletMappings);
// set servlet class names mappings
final Map servletClassNamesMappings = getServletClassMappings(jbossWebMD, pojoEndpoints);
jseArchiveMDBuilder.setServletClassNames(servletClassNamesMappings);
// set security domain
final String securityDomain = jbossWebMD.getSecurityDomain();
jseArchiveMDBuilder.setSecurityDomain(securityDomain);
// set wsdl location resolver
final JBossWebservicesMetaData jbossWebservicesMD = WSHelper.getOptionalAttachment(dep, JBossWebservicesMetaData.class);
if (jbossWebservicesMD != null) {
final PublishLocationAdapter resolver = new PublishLocationAdapterImpl(jbossWebservicesMD.getWebserviceDescriptions());
jseArchiveMDBuilder.setPublishLocationAdapter(resolver);
}
// set security meta data
final List jseSecurityMDs = getSecurityMetaData(jbossWebMD.getSecurityConstraints());
jseArchiveMDBuilder.setSecurityMetaData(jseSecurityMDs);
// set config name and file
setConfigNameAndFile(jseArchiveMDBuilder, jbossWebMD, jbossWebservicesMD);
return jseArchiveMDBuilder.build();
}
protected abstract List getPojoEndpoints(final DeploymentUnit unit);
/**
* Sets config name and config file.
*
* @param jseArchiveMD universal JSE meta data model
* @param jbossWebMD jboss web meta data
*/
private void setConfigNameAndFile(final JSEArchiveMetaData.Builder jseArchiveMDBuilder, final JBossWebMetaData jbossWebMD, final JBossWebservicesMetaData jbossWebservicesMD) {
if (jbossWebservicesMD != null) {
if (jbossWebservicesMD.getConfigName() != null) {
final String configName = jbossWebservicesMD.getConfigName();
jseArchiveMDBuilder.setConfigName(configName);
ROOT_LOGGER.settingConfigName(configName);
final String configFile = jbossWebservicesMD.getConfigFile();
jseArchiveMDBuilder.setConfigFile(configFile);
ROOT_LOGGER.settingConfigFile(configFile);
// ensure higher priority against web.xml context parameters
return;
}
}
final List contextParams = jbossWebMD.getContextParams();
if (contextParams != null) {
for (final ParamValueMetaData contextParam : contextParams) {
if (WSConstants.JBOSSWS_CONFIG_NAME.equals(contextParam.getParamName())) {
final String configName = contextParam.getParamValue();
jseArchiveMDBuilder.setConfigName(configName);
ROOT_LOGGER.settingConfigName(configName);
}
if (WSConstants.JBOSSWS_CONFIG_FILE.equals(contextParam.getParamName())) {
final String configFile = contextParam.getParamValue();
jseArchiveMDBuilder.setConfigFile(configFile);
ROOT_LOGGER.settingConfigFile(configFile);
}
}
}
}
/**
* Builds security meta data.
*
* @param securityConstraintsMD security constraints meta data
* @return universal JSE security meta data model
*/
private List getSecurityMetaData(final List securityConstraintsMD) {
final List jseSecurityMDs = new LinkedList();
if (securityConstraintsMD != null) {
for (final SecurityConstraintMetaData securityConstraintMD : securityConstraintsMD) {
final JSESecurityMetaData.Builder jseSecurityMDBuilder = new JSESecurityMetaData.Builder();
// transport guarantee
jseSecurityMDBuilder.setTransportGuarantee(securityConstraintMD.getTransportGuarantee().name());
// web resources
for (final WebResourceCollectionMetaData webResourceMD : securityConstraintMD.getResourceCollections()) {
jseSecurityMDBuilder.addWebResource(webResourceMD.getName(), webResourceMD.getUrlPatterns());
}
jseSecurityMDs.add(jseSecurityMDBuilder.build());
}
}
return jseSecurityMDs;
}
/**
* Returns servlet name to url pattern mappings.
*
* @param jbossWebMD jboss web meta data
* @return servlet name to url pattern mappings
*/
private Map getServletUrlPatternsMappings(final JBossWebMetaData jbossWebMD, final List pojoEndpoints) {
final Map mappings = new HashMap();
final List servletMappings = WebMetaDataHelper.getServletMappings(jbossWebMD);
for (final POJOEndpoint pojoEndpoint : pojoEndpoints) {
mappings.put(pojoEndpoint.getName(), pojoEndpoint.getUrlPattern());
if (!pojoEndpoint.isDeclared()) {
final String endpointName = pojoEndpoint.getName();
final List urlPatterns = WebMetaDataHelper.getUrlPatterns(pojoEndpoint.getUrlPattern());
WebMetaDataHelper.newServletMapping(endpointName, urlPatterns, servletMappings);
}
}
return mappings;
}
/**
* Returns servlet name to servlet class mappings.
*
* @param jbossWebMD jboss web meta data
* @return servlet name to servlet mappings
*/
private Map getServletClassMappings(final JBossWebMetaData jbossWebMD, final List pojoEndpoints) {
final Map mappings = new HashMap();
final JBossServletsMetaData servlets = WebMetaDataHelper.getServlets(jbossWebMD);
for (final POJOEndpoint pojoEndpoint : pojoEndpoints) {
final String pojoName = pojoEndpoint.getName();
final String pojoClassName = pojoEndpoint.getClassName();
mappings.put(pojoName, pojoClassName);
if (!pojoEndpoint.isDeclared()) {
final String endpointName = pojoEndpoint.getName();
final String endpointClassName = pojoEndpoint.getClassName();
WebMetaDataHelper.newServlet(endpointName, endpointClassName, servlets);
}
}
return mappings;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy