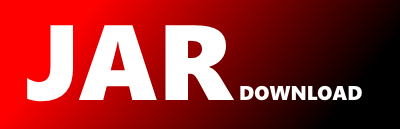
org.jboss.xnio.IoUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xnio-api Show documentation
Show all versions of xnio-api Show documentation
The API JAR of the XNIO project
/*
* JBoss, Home of Professional Open Source
* Copyright 2008, JBoss Inc., and individual contributors as indicated
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.jboss.xnio;
import java.io.Closeable;
import java.io.IOException;
import java.io.InterruptedIOException;
import java.net.DatagramSocket;
import java.net.ServerSocket;
import java.net.Socket;
import java.nio.channels.Selector;
import java.nio.channels.Channel;
import java.util.concurrent.CancellationException;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Executor;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import java.util.concurrent.RejectedExecutionException;
import java.util.concurrent.atomic.AtomicReferenceFieldUpdater;
import java.util.concurrent.atomic.AtomicReference;
import java.util.zip.ZipFile;
import org.jboss.xnio.log.Logger;
import java.util.logging.Handler;
/**
* General I/O utility methods.
*
* @apiviz.exclude
*/
public final class IoUtils {
private static final Executor NULL_EXECUTOR = new Executor() {
private final String string = String.format("null executor <%s>", Integer.toHexString(hashCode()));
public void execute(final Runnable command) {
// no operation
}
public String toString() {
return string;
}
};
private static final Executor DIRECT_EXECUTOR = new Executor() {
private final String string = String.format("direct executor <%s>", Integer.toHexString(hashCode()));
public void execute(final Runnable command) {
command.run();
}
public String toString() {
return string;
}
};
private static final Closeable NULL_CLOSEABLE = new Closeable() {
private final String string = String.format("null closeable <%s>", Integer.toHexString(hashCode()));
public void close() throws IOException {
// no operation
}
public String toString() {
return string;
}
};
private static final ChannelListener NULL_LISTENER = new ChannelListener() {
public void handleEvent(final Channel channel) {
}
};
private static final Cancellable NULL_CANCELLABLE = new Cancellable() {
public Cancellable cancel() {
return this;
}
};
private static final ChannelListener.Setter> NULL_SETTER = new ChannelListener.Setter() {
public void set(final ChannelListener super Channel> channelListener) {
}
};
private static final IoUtils.ResultNotifier RESULT_NOTIFIER = new IoUtils.ResultNotifier();
private IoUtils() {}
/**
* Get the direct executor. This is an executor that executes the provided task in the same thread.
*
* @return a direct executor
*/
public static Executor directExecutor() {
return DIRECT_EXECUTOR;
}
/**
* Get the null executor. This is an executor that never actually executes the provided task.
*
* @return a null executor
*/
public static Executor nullExecutor() {
return NULL_EXECUTOR;
}
/**
* Get a closeable executor wrapper for an {@code ExecutorService}. The given timeout is used to determine how long
* the {@code close()} method will wait for a clean shutdown before the executor is shut down forcefully.
*
* @param executorService the executor service
* @param timeout the timeout
* @param unit the unit for the timeout
* @return a new closeable executor
*/
public static CloseableExecutor closeableExecutor(final ExecutorService executorService, final long timeout, final TimeUnit unit) {
return new CloseableExecutor() {
public void close() throws IOException {
executorService.shutdown();
try {
if (executorService.awaitTermination(timeout, unit)) {
return;
}
executorService.shutdownNow();
throw new IOException("Executor did not shut down cleanly (killed)");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
executorService.shutdownNow();
throw new InterruptedIOException("Interrupted while awaiting executor shutdown");
}
}
public void execute(final Runnable command) {
executorService.execute(command);
}
};
}
/**
* Get the null closeable. This is a simple {@code Closeable} instance that does nothing when its {@code close()}
* method is invoked.
*
* @return the null closeable
*/
public static Closeable nullCloseable() {
return NULL_CLOSEABLE;
}
private static final Logger closeLog = Logger.getLogger("org.jboss.xnio.safe-close");
/**
* Close a resource, logging an error if an error occurs.
*
* @param resource the resource to close
*/
public static void safeClose(final Closeable resource) {
try {
if (resource != null) {
resource.close();
}
} catch (Throwable t) {
closeLog.trace(t, "Closing resource failed");
}
}
/**
* Close a resource, logging an error if an error occurs.
*
* @param resource the resource to close
*/
public static void safeClose(final Socket resource) {
try {
if (resource != null) {
resource.close();
}
} catch (Throwable t) {
closeLog.trace(t, "Closing resource failed");
}
}
/**
* Close a resource, logging an error if an error occurs.
*
* @param resource the resource to close
*/
public static void safeClose(final DatagramSocket resource) {
try {
if (resource != null) {
resource.close();
}
} catch (Throwable t) {
closeLog.trace(t, "Closing resource failed");
}
}
/**
* Close a resource, logging an error if an error occurs.
*
* @param resource the resource to close
*/
public static void safeClose(final Selector resource) {
try {
if (resource != null) {
resource.close();
}
} catch (Throwable t) {
closeLog.trace(t, "Closing resource failed");
}
}
/**
* Close a resource, logging an error if an error occurs.
*
* @param resource the resource to close
*/
public static void safeClose(final ServerSocket resource) {
try {
if (resource != null) {
resource.close();
}
} catch (Throwable t) {
closeLog.trace(t, "Closing resource failed");
}
}
/**
* Close a resource, logging an error if an error occurs.
*
* @param resource the resource to close
*/
public static void safeClose(final ZipFile resource) {
try {
if (resource != null) {
resource.close();
}
} catch (Throwable t) {
closeLog.trace(t, "Closing resource failed");
}
}
/**
* Close a resource, logging an error if an error occurs.
*
* @param resource the resource to close
*/
public static void safeClose(final Handler resource) {
try {
if (resource != null) {
resource.close();
}
} catch (Throwable t) {
closeLog.trace(t, "Closing resource failed");
}
}
/**
* Close a future resource, logging an error if an error occurs. Attempts to cancel the operation if it is
* still in progress.
*
* @param futureResource the resource to close
*/
public static void safeClose(final IoFuture extends Closeable> futureResource) {
futureResource.cancel().addNotifier(closingNotifier(), null);
}
private static final IoFuture.Notifier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy