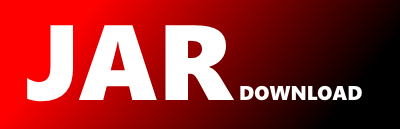
bpsim.TimeParameters Maven / Gradle / Ivy
Show all versions of jbpm-bpmn2-emfextmodel Show documentation
/*
* Copyright 2015 Red Hat, Inc. and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
*/
package bpsim;
import org.eclipse.emf.ecore.EObject;
/**
*
* A representation of the model object 'Time Parameters'.
*
*
*
* The following features are supported:
*
* - {@link bpsim.TimeParameters#getTransferTime Transfer Time}
* - {@link bpsim.TimeParameters#getQueueTime Queue Time}
* - {@link bpsim.TimeParameters#getWaitTime Wait Time}
* - {@link bpsim.TimeParameters#getSetUpTime Set Up Time}
* - {@link bpsim.TimeParameters#getProcessingTime Processing Time}
* - {@link bpsim.TimeParameters#getValidationTime Validation Time}
* - {@link bpsim.TimeParameters#getReworkTime Rework Time}
*
*
*
* @see bpsim.BpsimPackage#getTimeParameters()
* @model extendedMetaData="name='TimeParameters' kind='elementOnly'"
* @generated
*/
public interface TimeParameters extends EObject {
/**
* Returns the value of the 'Transfer Time' containment reference.
*
*
* If the meaning of the 'Transfer Time' containment reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Transfer Time' containment reference.
* @see #setTransferTime(Parameter)
* @see bpsim.BpsimPackage#getTimeParameters_TransferTime()
* @model containment="true"
* extendedMetaData="kind='element' name='TransferTime' namespace='##targetNamespace'"
* @generated
*/
Parameter getTransferTime();
/**
* Sets the value of the '{@link bpsim.TimeParameters#getTransferTime Transfer Time}' containment reference.
*
*
* @param value the new value of the 'Transfer Time' containment reference.
* @see #getTransferTime()
* @generated
*/
void setTransferTime(Parameter value);
/**
* Returns the value of the 'Queue Time' containment reference.
*
*
* If the meaning of the 'Queue Time' containment reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Queue Time' containment reference.
* @see #setQueueTime(Parameter)
* @see bpsim.BpsimPackage#getTimeParameters_QueueTime()
* @model containment="true"
* extendedMetaData="kind='element' name='QueueTime' namespace='##targetNamespace'"
* @generated
*/
Parameter getQueueTime();
/**
* Sets the value of the '{@link bpsim.TimeParameters#getQueueTime Queue Time}' containment reference.
*
*
* @param value the new value of the 'Queue Time' containment reference.
* @see #getQueueTime()
* @generated
*/
void setQueueTime(Parameter value);
/**
* Returns the value of the 'Wait Time' containment reference.
*
*
* If the meaning of the 'Wait Time' containment reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Wait Time' containment reference.
* @see #setWaitTime(Parameter)
* @see bpsim.BpsimPackage#getTimeParameters_WaitTime()
* @model containment="true"
* extendedMetaData="kind='element' name='WaitTime' namespace='##targetNamespace'"
* @generated
*/
Parameter getWaitTime();
/**
* Sets the value of the '{@link bpsim.TimeParameters#getWaitTime Wait Time}' containment reference.
*
*
* @param value the new value of the 'Wait Time' containment reference.
* @see #getWaitTime()
* @generated
*/
void setWaitTime(Parameter value);
/**
* Returns the value of the 'Set Up Time' containment reference.
*
*
* If the meaning of the 'Set Up Time' containment reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Set Up Time' containment reference.
* @see #setSetUpTime(Parameter)
* @see bpsim.BpsimPackage#getTimeParameters_SetUpTime()
* @model containment="true"
* extendedMetaData="kind='element' name='SetUpTime' namespace='##targetNamespace'"
* @generated
*/
Parameter getSetUpTime();
/**
* Sets the value of the '{@link bpsim.TimeParameters#getSetUpTime Set Up Time}' containment reference.
*
*
* @param value the new value of the 'Set Up Time' containment reference.
* @see #getSetUpTime()
* @generated
*/
void setSetUpTime(Parameter value);
/**
* Returns the value of the 'Processing Time' containment reference.
*
*
* If the meaning of the 'Processing Time' containment reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Processing Time' containment reference.
* @see #setProcessingTime(Parameter)
* @see bpsim.BpsimPackage#getTimeParameters_ProcessingTime()
* @model containment="true"
* extendedMetaData="kind='element' name='ProcessingTime' namespace='##targetNamespace'"
* @generated
*/
Parameter getProcessingTime();
/**
* Sets the value of the '{@link bpsim.TimeParameters#getProcessingTime Processing Time}' containment reference.
*
*
* @param value the new value of the 'Processing Time' containment reference.
* @see #getProcessingTime()
* @generated
*/
void setProcessingTime(Parameter value);
/**
* Returns the value of the 'Validation Time' containment reference.
*
*
* If the meaning of the 'Validation Time' containment reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Validation Time' containment reference.
* @see #setValidationTime(Parameter)
* @see bpsim.BpsimPackage#getTimeParameters_ValidationTime()
* @model containment="true"
* extendedMetaData="kind='element' name='ValidationTime' namespace='##targetNamespace'"
* @generated
*/
Parameter getValidationTime();
/**
* Sets the value of the '{@link bpsim.TimeParameters#getValidationTime Validation Time}' containment reference.
*
*
* @param value the new value of the 'Validation Time' containment reference.
* @see #getValidationTime()
* @generated
*/
void setValidationTime(Parameter value);
/**
* Returns the value of the 'Rework Time' containment reference.
*
*
* If the meaning of the 'Rework Time' containment reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Rework Time' containment reference.
* @see #setReworkTime(Parameter)
* @see bpsim.BpsimPackage#getTimeParameters_ReworkTime()
* @model containment="true"
* extendedMetaData="kind='element' name='ReworkTime' namespace='##targetNamespace'"
* @generated
*/
Parameter getReworkTime();
/**
* Sets the value of the '{@link bpsim.TimeParameters#getReworkTime Rework Time}' containment reference.
*
*
* @param value the new value of the 'Rework Time' containment reference.
* @see #getReworkTime()
* @generated
*/
void setReworkTime(Parameter value);
} // TimeParameters