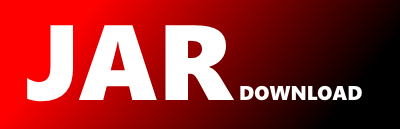
bpsim.impl.BpsimFactoryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbpm-bpmn2-emfextmodel Show documentation
Show all versions of jbpm-bpmn2-emfextmodel Show documentation
jBPM BPMN2 EMF Extension Model
/*
* Copyright 2015 Red Hat, Inc. and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
*/
package bpsim.impl;
import bpsim.*;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EDataType;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.impl.EFactoryImpl;
import org.eclipse.emf.ecore.plugin.EcorePlugin;
/**
*
* An implementation of the model Factory.
*
* @generated
*/
public class BpsimFactoryImpl extends EFactoryImpl implements BpsimFactory {
/**
* Creates the default factory implementation.
*
*
* @generated
*/
public static BpsimFactory init() {
try {
BpsimFactory theBpsimFactory = (BpsimFactory)EPackage.Registry.INSTANCE.getEFactory("http://www.bpsim.org/schemas/1.0");
if (theBpsimFactory != null) {
return theBpsimFactory;
}
}
catch (Exception exception) {
EcorePlugin.INSTANCE.log(exception);
}
return new BpsimFactoryImpl();
}
/**
* Creates an instance of the factory.
*
*
* @generated
*/
public BpsimFactoryImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
public EObject create(EClass eClass) {
switch (eClass.getClassifierID()) {
case BpsimPackage.BETA_DISTRIBUTION_TYPE: return createBetaDistributionType();
case BpsimPackage.BINOMIAL_DISTRIBUTION_TYPE: return createBinomialDistributionType();
case BpsimPackage.BOOLEAN_PARAMETER_TYPE: return createBooleanParameterType();
case BpsimPackage.BP_SIM_DATA_TYPE: return createBPSimDataType();
case BpsimPackage.CALENDAR: return createCalendar();
case BpsimPackage.CONSTANT_PARAMETER: return createConstantParameter();
case BpsimPackage.CONTROL_PARAMETERS: return createControlParameters();
case BpsimPackage.COST_PARAMETERS: return createCostParameters();
case BpsimPackage.DATE_TIME_PARAMETER_TYPE: return createDateTimeParameterType();
case BpsimPackage.DISTRIBUTION_PARAMETER: return createDistributionParameter();
case BpsimPackage.DOCUMENT_ROOT: return createDocumentRoot();
case BpsimPackage.DURATION_PARAMETER_TYPE: return createDurationParameterType();
case BpsimPackage.ELEMENT_PARAMETERS: return createElementParameters();
case BpsimPackage.ELEMENT_PARAMETERS_TYPE: return createElementParametersType();
case BpsimPackage.ENUM_PARAMETER_TYPE: return createEnumParameterType();
case BpsimPackage.ERLANG_DISTRIBUTION_TYPE: return createErlangDistributionType();
case BpsimPackage.EXPRESSION_PARAMETER_TYPE: return createExpressionParameterType();
case BpsimPackage.FLOATING_PARAMETER_TYPE: return createFloatingParameterType();
case BpsimPackage.GAMMA_DISTRIBUTION_TYPE: return createGammaDistributionType();
case BpsimPackage.LOG_NORMAL_DISTRIBUTION_TYPE: return createLogNormalDistributionType();
case BpsimPackage.NEGATIVE_EXPONENTIAL_DISTRIBUTION_TYPE: return createNegativeExponentialDistributionType();
case BpsimPackage.NORMAL_DISTRIBUTION_TYPE: return createNormalDistributionType();
case BpsimPackage.NUMERIC_PARAMETER_TYPE: return createNumericParameterType();
case BpsimPackage.PARAMETER: return createParameter();
case BpsimPackage.PARAMETER_VALUE: return createParameterValue();
case BpsimPackage.POISSON_DISTRIBUTION_TYPE: return createPoissonDistributionType();
case BpsimPackage.PRIORITY_PARAMETERS: return createPriorityParameters();
case BpsimPackage.PROPERTY_PARAMETERS: return createPropertyParameters();
case BpsimPackage.PROPERTY_TYPE: return createPropertyType();
case BpsimPackage.RESOURCE_PARAMETERS: return createResourceParameters();
case BpsimPackage.SCENARIO: return createScenario();
case BpsimPackage.SCENARIO_PARAMETERS: return createScenarioParameters();
case BpsimPackage.SCENARIO_PARAMETERS_TYPE: return createScenarioParametersType();
case BpsimPackage.STRING_PARAMETER_TYPE: return createStringParameterType();
case BpsimPackage.TIME_PARAMETERS: return createTimeParameters();
case BpsimPackage.TRIANGULAR_DISTRIBUTION_TYPE: return createTriangularDistributionType();
case BpsimPackage.TRUNCATED_NORMAL_DISTRIBUTION_TYPE: return createTruncatedNormalDistributionType();
case BpsimPackage.UNIFORM_DISTRIBUTION_TYPE: return createUniformDistributionType();
case BpsimPackage.USER_DISTRIBUTION_DATA_POINT_TYPE: return createUserDistributionDataPointType();
case BpsimPackage.USER_DISTRIBUTION_TYPE: return createUserDistributionType();
case BpsimPackage.VENDOR_EXTENSION: return createVendorExtension();
case BpsimPackage.WEIBULL_DISTRIBUTION_TYPE: return createWeibullDistributionType();
default:
throw new IllegalArgumentException("The class '" + eClass.getName() + "' is not a valid classifier");
}
}
/**
*
*
* @generated
*/
@Override
public Object createFromString(EDataType eDataType, String initialValue) {
switch (eDataType.getClassifierID()) {
case BpsimPackage.RESULT_TYPE:
return createResultTypeFromString(eDataType, initialValue);
case BpsimPackage.TIME_UNIT:
return createTimeUnitFromString(eDataType, initialValue);
case BpsimPackage.RESULT_TYPE_OBJECT:
return createResultTypeObjectFromString(eDataType, initialValue);
case BpsimPackage.TIME_UNIT_OBJECT:
return createTimeUnitObjectFromString(eDataType, initialValue);
default:
throw new IllegalArgumentException("The datatype '" + eDataType.getName() + "' is not a valid classifier");
}
}
/**
*
*
* @generated
*/
@Override
public String convertToString(EDataType eDataType, Object instanceValue) {
switch (eDataType.getClassifierID()) {
case BpsimPackage.RESULT_TYPE:
return convertResultTypeToString(eDataType, instanceValue);
case BpsimPackage.TIME_UNIT:
return convertTimeUnitToString(eDataType, instanceValue);
case BpsimPackage.RESULT_TYPE_OBJECT:
return convertResultTypeObjectToString(eDataType, instanceValue);
case BpsimPackage.TIME_UNIT_OBJECT:
return convertTimeUnitObjectToString(eDataType, instanceValue);
default:
throw new IllegalArgumentException("The datatype '" + eDataType.getName() + "' is not a valid classifier");
}
}
/**
*
*
* @generated
*/
public BetaDistributionType createBetaDistributionType() {
BetaDistributionTypeImpl betaDistributionType = new BetaDistributionTypeImpl();
return betaDistributionType;
}
/**
*
*
* @generated
*/
public BinomialDistributionType createBinomialDistributionType() {
BinomialDistributionTypeImpl binomialDistributionType = new BinomialDistributionTypeImpl();
return binomialDistributionType;
}
/**
*
*
* @generated
*/
public BooleanParameterType createBooleanParameterType() {
BooleanParameterTypeImpl booleanParameterType = new BooleanParameterTypeImpl();
return booleanParameterType;
}
/**
*
*
* @generated
*/
public BPSimDataType createBPSimDataType() {
BPSimDataTypeImpl bpSimDataType = new BPSimDataTypeImpl();
return bpSimDataType;
}
/**
*
*
* @generated
*/
public Calendar createCalendar() {
CalendarImpl calendar = new CalendarImpl();
return calendar;
}
/**
*
*
* @generated
*/
public ConstantParameter createConstantParameter() {
ConstantParameterImpl constantParameter = new ConstantParameterImpl();
return constantParameter;
}
/**
*
*
* @generated
*/
public ControlParameters createControlParameters() {
ControlParametersImpl controlParameters = new ControlParametersImpl();
return controlParameters;
}
/**
*
*
* @generated
*/
public CostParameters createCostParameters() {
CostParametersImpl costParameters = new CostParametersImpl();
return costParameters;
}
/**
*
*
* @generated
*/
public DateTimeParameterType createDateTimeParameterType() {
DateTimeParameterTypeImpl dateTimeParameterType = new DateTimeParameterTypeImpl();
return dateTimeParameterType;
}
/**
*
*
* @generated
*/
public DistributionParameter createDistributionParameter() {
DistributionParameterImpl distributionParameter = new DistributionParameterImpl();
return distributionParameter;
}
/**
*
*
* @generated
*/
public DocumentRoot createDocumentRoot() {
DocumentRootImpl documentRoot = new DocumentRootImpl();
return documentRoot;
}
/**
*
*
* @generated
*/
public DurationParameterType createDurationParameterType() {
DurationParameterTypeImpl durationParameterType = new DurationParameterTypeImpl();
return durationParameterType;
}
/**
*
*
* @generated
*/
public ElementParameters createElementParameters() {
ElementParametersImpl elementParameters = new ElementParametersImpl();
return elementParameters;
}
/**
*
*
* @generated
*/
public ElementParametersType createElementParametersType() {
ElementParametersTypeImpl elementParametersType = new ElementParametersTypeImpl();
return elementParametersType;
}
/**
*
*
* @generated
*/
public EnumParameterType createEnumParameterType() {
EnumParameterTypeImpl enumParameterType = new EnumParameterTypeImpl();
return enumParameterType;
}
/**
*
*
* @generated
*/
public ErlangDistributionType createErlangDistributionType() {
ErlangDistributionTypeImpl erlangDistributionType = new ErlangDistributionTypeImpl();
return erlangDistributionType;
}
/**
*
*
* @generated
*/
public ExpressionParameterType createExpressionParameterType() {
ExpressionParameterTypeImpl expressionParameterType = new ExpressionParameterTypeImpl();
return expressionParameterType;
}
/**
*
*
* @generated
*/
public FloatingParameterType createFloatingParameterType() {
FloatingParameterTypeImpl floatingParameterType = new FloatingParameterTypeImpl();
return floatingParameterType;
}
/**
*
*
* @generated
*/
public GammaDistributionType createGammaDistributionType() {
GammaDistributionTypeImpl gammaDistributionType = new GammaDistributionTypeImpl();
return gammaDistributionType;
}
/**
*
*
* @generated
*/
public LogNormalDistributionType createLogNormalDistributionType() {
LogNormalDistributionTypeImpl logNormalDistributionType = new LogNormalDistributionTypeImpl();
return logNormalDistributionType;
}
/**
*
*
* @generated
*/
public NegativeExponentialDistributionType createNegativeExponentialDistributionType() {
NegativeExponentialDistributionTypeImpl negativeExponentialDistributionType = new NegativeExponentialDistributionTypeImpl();
return negativeExponentialDistributionType;
}
/**
*
*
* @generated
*/
public NormalDistributionType createNormalDistributionType() {
NormalDistributionTypeImpl normalDistributionType = new NormalDistributionTypeImpl();
return normalDistributionType;
}
/**
*
*
* @generated
*/
public NumericParameterType createNumericParameterType() {
NumericParameterTypeImpl numericParameterType = new NumericParameterTypeImpl();
return numericParameterType;
}
/**
*
*
* @generated
*/
public Parameter createParameter() {
ParameterImpl parameter = new ParameterImpl();
return parameter;
}
/**
*
*
* @generated
*/
public ParameterValue createParameterValue() {
ParameterValueImpl parameterValue = new ParameterValueImpl();
return parameterValue;
}
/**
*
*
* @generated
*/
public PoissonDistributionType createPoissonDistributionType() {
PoissonDistributionTypeImpl poissonDistributionType = new PoissonDistributionTypeImpl();
return poissonDistributionType;
}
/**
*
*
* @generated
*/
public PriorityParameters createPriorityParameters() {
PriorityParametersImpl priorityParameters = new PriorityParametersImpl();
return priorityParameters;
}
/**
*
*
* @generated
*/
public PropertyParameters createPropertyParameters() {
PropertyParametersImpl propertyParameters = new PropertyParametersImpl();
return propertyParameters;
}
/**
*
*
* @generated
*/
public PropertyType createPropertyType() {
PropertyTypeImpl propertyType = new PropertyTypeImpl();
return propertyType;
}
/**
*
*
* @generated
*/
public ResourceParameters createResourceParameters() {
ResourceParametersImpl resourceParameters = new ResourceParametersImpl();
return resourceParameters;
}
/**
*
*
* @generated
*/
public Scenario createScenario() {
ScenarioImpl scenario = new ScenarioImpl();
return scenario;
}
/**
*
*
* @generated
*/
public ScenarioParameters createScenarioParameters() {
ScenarioParametersImpl scenarioParameters = new ScenarioParametersImpl();
return scenarioParameters;
}
/**
*
*
* @generated
*/
public ScenarioParametersType createScenarioParametersType() {
ScenarioParametersTypeImpl scenarioParametersType = new ScenarioParametersTypeImpl();
return scenarioParametersType;
}
/**
*
*
* @generated
*/
public StringParameterType createStringParameterType() {
StringParameterTypeImpl stringParameterType = new StringParameterTypeImpl();
return stringParameterType;
}
/**
*
*
* @generated
*/
public TimeParameters createTimeParameters() {
TimeParametersImpl timeParameters = new TimeParametersImpl();
return timeParameters;
}
/**
*
*
* @generated
*/
public TriangularDistributionType createTriangularDistributionType() {
TriangularDistributionTypeImpl triangularDistributionType = new TriangularDistributionTypeImpl();
return triangularDistributionType;
}
/**
*
*
* @generated
*/
public TruncatedNormalDistributionType createTruncatedNormalDistributionType() {
TruncatedNormalDistributionTypeImpl truncatedNormalDistributionType = new TruncatedNormalDistributionTypeImpl();
return truncatedNormalDistributionType;
}
/**
*
*
* @generated
*/
public UniformDistributionType createUniformDistributionType() {
UniformDistributionTypeImpl uniformDistributionType = new UniformDistributionTypeImpl();
return uniformDistributionType;
}
/**
*
*
* @generated
*/
public UserDistributionDataPointType createUserDistributionDataPointType() {
UserDistributionDataPointTypeImpl userDistributionDataPointType = new UserDistributionDataPointTypeImpl();
return userDistributionDataPointType;
}
/**
*
*
* @generated
*/
public UserDistributionType createUserDistributionType() {
UserDistributionTypeImpl userDistributionType = new UserDistributionTypeImpl();
return userDistributionType;
}
/**
*
*
* @generated
*/
public VendorExtension createVendorExtension() {
VendorExtensionImpl vendorExtension = new VendorExtensionImpl();
return vendorExtension;
}
/**
*
*
* @generated
*/
public WeibullDistributionType createWeibullDistributionType() {
WeibullDistributionTypeImpl weibullDistributionType = new WeibullDistributionTypeImpl();
return weibullDistributionType;
}
/**
*
*
* @generated
*/
public ResultType createResultTypeFromString(EDataType eDataType, String initialValue) {
ResultType result = ResultType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertResultTypeToString(EDataType eDataType, Object instanceValue) {
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public TimeUnit createTimeUnitFromString(EDataType eDataType, String initialValue) {
TimeUnit result = TimeUnit.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertTimeUnitToString(EDataType eDataType, Object instanceValue) {
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public ResultType createResultTypeObjectFromString(EDataType eDataType, String initialValue) {
return createResultTypeFromString(BpsimPackage.Literals.RESULT_TYPE, initialValue);
}
/**
*
*
* @generated
*/
public String convertResultTypeObjectToString(EDataType eDataType, Object instanceValue) {
return convertResultTypeToString(BpsimPackage.Literals.RESULT_TYPE, instanceValue);
}
/**
*
*
* @generated
*/
public TimeUnit createTimeUnitObjectFromString(EDataType eDataType, String initialValue) {
return createTimeUnitFromString(BpsimPackage.Literals.TIME_UNIT, initialValue);
}
/**
*
*
* @generated
*/
public String convertTimeUnitObjectToString(EDataType eDataType, Object instanceValue) {
return convertTimeUnitToString(BpsimPackage.Literals.TIME_UNIT, instanceValue);
}
/**
*
*
* @generated
*/
public BpsimPackage getBpsimPackage() {
return (BpsimPackage)getEPackage();
}
/**
*
*
* @deprecated
* @generated
*/
@Deprecated
public static BpsimPackage getPackage() {
return BpsimPackage.eINSTANCE;
}
} //BpsimFactoryImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy