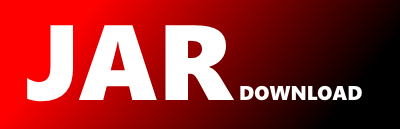
org.jbpm.examples.evaluation.EvaluationExample Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbpm-examples Show documentation
Show all versions of jbpm-examples Show documentation
Run JBPMExamplesApp to try the jBPM examples.
/*
* Copyright 2017 Red Hat, Inc. and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jbpm.examples.evaluation;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.kie.api.KieServices;
import org.kie.api.io.ResourceType;
import org.kie.api.runtime.KieSession;
import org.kie.api.runtime.manager.RuntimeEngine;
import org.kie.api.runtime.manager.RuntimeEnvironment;
import org.kie.api.runtime.manager.RuntimeEnvironmentBuilder;
import org.kie.api.runtime.manager.RuntimeManager;
import org.kie.api.runtime.manager.RuntimeManagerFactory;
import org.kie.api.task.TaskService;
import org.kie.api.task.model.TaskSummary;
import org.kie.test.util.db.PersistenceUtil;
public class EvaluationExample {
public static final void main(String[] args) {
try {
RuntimeManager manager = getRuntimeManager("evaluation/Evaluation.bpmn");
RuntimeEngine runtime = manager.getRuntimeEngine(null);
KieSession ksession = runtime.getKieSession();
// start a new process instance
Map params = new HashMap();
params.put("employee", "krisv");
params.put("reason", "Yearly performance evaluation");
ksession.startProcess("com.sample.evaluation", params);
// complete Self Evaluation
TaskService taskService = runtime.getTaskService();
List tasks = taskService.getTasksAssignedAsPotentialOwner("krisv", "en-UK");
TaskSummary task = tasks.get(0);
System.out.println("'krisv' completing task " + task.getName() + ": " + task.getDescription());
taskService.start(task.getId(), "krisv");
Map results = new HashMap();
results.put("performance", "exceeding");
taskService.complete(task.getId(), "krisv", results);
// john from HR
tasks = taskService.getTasksAssignedAsPotentialOwner("john", "en-UK");
task = tasks.get(0);
System.out.println("'john' completing task " + task.getName() + ": " + task.getDescription());
taskService.start(task.getId(), "john");
results = new HashMap();
results.put("performance", "acceptable");
taskService.complete(task.getId(), "john", results);
// mary from PM
tasks = taskService.getTasksAssignedAsPotentialOwner("mary", "en-UK");
task = tasks.get(0);
System.out.println("'mary' completing task " + task.getName() + ": " + task.getDescription());
taskService.start(task.getId(), "mary");
results = new HashMap();
results.put("performance", "outstanding");
taskService.complete(task.getId(), "mary", results);
System.out.println("Process instance completed");
manager.disposeRuntimeEngine(runtime);
} catch (Throwable t) {
t.printStackTrace();
}
System.exit(0);
}
private static RuntimeManager getRuntimeManager(String process) {
// load up the knowledge base
PersistenceUtil.setupPoolingDataSource();
RuntimeEnvironment environment = RuntimeEnvironmentBuilder.Factory.get().newDefaultBuilder()
.addAsset(KieServices.Factory.get().getResources().newClassPathResource(process), ResourceType.BPMN2)
.get();
return RuntimeManagerFactory.Factory.get().newSingletonRuntimeManager(environment);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy