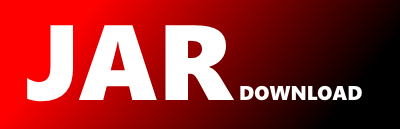
org.jbpt.pm.data.DataModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbpt-bpm Show documentation
Show all versions of jbpt-bpm Show documentation
The jBPT code library is a compendium of technologies that support research on design, execution, and evaluation of business processes.
package org.jbpt.pm.data;
import java.util.ArrayList;
import java.util.Collection;
import org.jbpt.graph.abs.AbstractDirectedGraph;
import org.jbpt.pm.DataNode;
/**
* basic data model implementation
*
* @author Andreas Meyer
*
*/
public class DataModel extends AbstractDirectedGraph, DataNode> implements IDataModel, DataNode> {
private String name;
/**
* Construct an empty data model
*/
public DataModel() {
this.name = "";
}
/**
* Construct an empty data model with name
*/
public DataModel(String name) {
this.name = name;
}
@Override
public String getName() {
return this.name;
}
@Override
public DataConnection addDataConnection(DataNode from, DataNode to, DataConnectionType type) {
if (from == null || to == null) {
return null;
}
from.setModel(this);
to.setModel(this);
Collection ss = new ArrayList();
ss.add(from);
Collection ts = new ArrayList();
ts.add(to);
if (!this.checkEdge(ss, ts)) return null;
return new DataConnection(this, from, to, type);
}
@Override
public DataConnection addGeneralization(DataNode from, DataNode to) {
if (from == null || to == null) {
return null;
}
from.setModel(this);
to.setModel(this);
Collection ss = new ArrayList();
ss.add(from);
Collection ts = new ArrayList();
ts.add(to);
if (!this.checkEdge(ss, ts)) return null;
return new DataConnection(this, from, to, DataConnectionType.G);
}
@Override
public DataConnection addAggregation(DataNode from, DataNode to) {
if (from == null || to == null) {
return null;
}
from.setModel(this);
to.setModel(this);
Collection ss = new ArrayList();
ss.add(from);
Collection ts = new ArrayList();
ts.add(to);
if (!this.checkEdge(ss, ts)) return null;
return new DataConnection(this, from, to, DataConnectionType.Agg);
}
@Override
public DataConnection addAssociation(DataNode from, DataNode to) {
if (from == null || to == null) {
return null;
}
from.setModel(this);
to.setModel(this);
Collection ss = new ArrayList();
ss.add(from);
Collection ts = new ArrayList();
ts.add(to);
if (!this.checkEdge(ss, ts)) return null;
return new DataConnection(this, from, to, DataConnectionType.Ass);
}
@Override
public DataNode addDataNode(DataNode dataNode) {
dataNode.setModel(this);
return (DataNode) super.addVertex((DataNode) dataNode);
}
@Override
public Collection> getDataConnections() {
return this.getEdges();
}
@Override
public Collection> getDataConnections(DataConnectionType type) {
Collection> result = new ArrayList>();
for (DataConnection e : this.getEdges()) {
if(e.getDataConnectionType().equals(type)) {
result.add(e);
}
}
return result;
}
@Override
public Collection getDataNodes() {
Collection dataNodes = new ArrayList();
for (DataNode node : this.vertices.keySet()){
if (node instanceof DataNode){
dataNodes.add((DataNode) node);
}
}
return dataNodes;
}
@Override
public String toDOT() {
String result = "";
if (this == null) {
return result;
}
result += "digraph G {\n";
result += "rankdir=TD \n"; //rankdir=LR for left to right graph; rankdir=TD for top to down graph
for (DataNode d : this.getDataNodes()) {
result += String.format(" n%s[shape=box,label=\"%s\"];\n", d.getId().replace("-", ""), d.getName());
}
result+="\n";
for (DataConnection dc: this.getDataConnections(DataConnectionType.Agg)) {
result += String.format(" n%s->n%s[dir=\"both\",arrowhead=\"none\",arrowtail=\"ediamond\"];\n", dc.getSource().getId().replace("-", ""), dc.getTarget().getId().replace("-", ""));
}
result+="\n";
for (DataConnection dc: this.getDataConnections(DataConnectionType.Ass)) {
result += String.format(" n%s->n%s[dir=\"both\",arrowhead=\"none\",arrowtail=\"none\"];\n", dc.getSource().getId().replace("-", ""), dc.getTarget().getId().replace("-", ""));
}
result+="\n";
for (DataConnection dc: this.getDataConnections(DataConnectionType.G)) {
result += String.format(" n%s->n%s[dir=\"both\",arrowhead=\"none\",arrowtail=\"empty\"];\n", dc.getSource().getId().replace("-", ""), dc.getTarget().getId().replace("-", ""));
}
result += "}";
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy