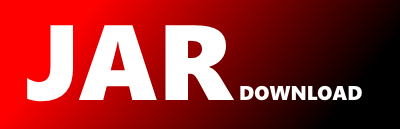
org.jclarion.clarion.runtime.CMemoryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clarion-runtime Show documentation
Show all versions of clarion-runtime Show documentation
JClarion runtime environment
/**
* Copyright 2010, by Andrew Barnham
*
* The contents of this file are subject to
* GNU Lesser General Public License (LGPL), v.3
* http://www.gnu.org/licenses/lgpl.txt
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied.
*/
package org.jclarion.clarion.runtime;
import java.lang.ref.WeakReference;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import org.jclarion.clarion.Threaded;
public class CMemoryImpl
{
private static CMemoryImpl instance ;
public static CMemoryImpl getInstance()
{
if (instance==null) {
synchronized(CMemoryImpl.class) {
if (instance==null) instance=new CMemoryImpl();
}
}
return instance;
}
private int lastMemRef=0;
private static class Entry {
private Thread _thread;
private WeakReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy