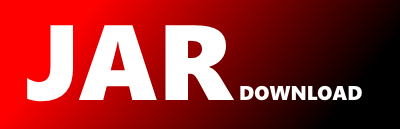
org.jclarion.clarion.swing.QueueTableModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clarion-runtime Show documentation
Show all versions of clarion-runtime Show documentation
JClarion runtime environment
The newest version!
/**
* Copyright 2010, by Andrew Barnham
*
* The contents of this file are subject to
* GNU Lesser General Public License (LGPL), v.3
* http://www.gnu.org/licenses/lgpl.txt
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied.
*/
package org.jclarion.clarion.swing;
import javax.swing.JTable;
import javax.swing.event.TableModelEvent;
import javax.swing.event.TableModelListener;
import javax.swing.table.TableColumn;
import javax.swing.table.TableColumnModel;
import javax.swing.table.TableModel;
import java.util.ArrayList;
import java.util.List;
import org.jclarion.clarion.ClarionObject;
import org.jclarion.clarion.ClarionQueueEvent;
import org.jclarion.clarion.ClarionQueueListener;
import org.jclarion.clarion.ClarionQueueReader;
import org.jclarion.clarion.TreeClarionQueue;
import org.jclarion.clarion.control.ListColumn;
import org.jclarion.clarion.control.ListControl;
import org.jclarion.clarion.constants.*;
public class QueueTableModel implements TableModel,ClarionQueueListener
{
private ClarionQueueReader queue;
private ListControl control;
private List listeners=new ArrayList();
private ListColumn columns[];
private JTable table;
private boolean hasMark;
private int last_size=-1;
private boolean isTree=false;
public QueueTableModel(ListControl control)
{
this.control=control;
this.queue=control.getFrom();
hasMark = control.getRawProperty(Prop.MARK) != null;
columns=ListColumn.construct(control.getProperty(Prop.FORMAT).toString());
if (columns.length==0) columns=ListColumn.constructDefault();
for (int scan=0;scan getColumnClass(int columnIndex) {
return String.class;
}
@Override
public int getColumnCount() {
return columns.length;
}
@Override
public String getColumnName(int columnIndex) {
return columns[columnIndex].getHeader();
}
private int assumedRows;
@Override
public int getRowCount() {
assumedRows=getCapturedRowCount();
return assumedRows;
}
private int lastPos=-1;
private ClarionObject lastGet[];
@Override
public Object getValueAt(int rowIndex, int columnIndex) {
if (columnIndex>=columns.length) return null;
if (lastPos!=rowIndex) {
lastGet=queue.getRecord(rowIndex+1);
lastPos=rowIndex;
}
if (lastGet==null) {
lastPos=-1;
return null;
}
return new ClarionQueueTableCell(queue,rowIndex+1,columns[columnIndex],lastGet);
}
@Override
public boolean isCellEditable(int rowIndex, int columnIndex) {
return false;
}
@Override
public void removeTableModelListener(TableModelListener l) {
listeners.remove(l);
}
@Override
public void setValueAt(Object value, int rowIndex, int columnIndex) {
}
private boolean postNS = false;
private TableModelEvent tme = null;
private boolean tmeAll = false;
private boolean ignore;
public void setIgnoreModificationEvents(boolean ignore)
{
this.ignore=ignore;
}
public boolean isIgnoreModificationEvent()
{
return ignore;
}
@Override
public void queueModified(ClarionQueueEvent event)
{
if (ignore) return;
lastPos=-1;
if (tme!=null) {
tmeAll=true;
postNS=true;
tme=null;
}
if (!tmeAll) {
if (event.event==ClarionQueueEvent.EventType.ADD) {
tme=new TableModelEvent(this,event.record-1,event.record-1,
TableModelEvent.ALL_COLUMNS,TableModelEvent.INSERT);
}
if (event.event==ClarionQueueEvent.EventType.PUT) {
tme=new TableModelEvent(this,event.record-1,event.record-1,
TableModelEvent.ALL_COLUMNS,TableModelEvent.UPDATE);
}
if (event.event==ClarionQueueEvent.EventType.DELETE) {
tme=new TableModelEvent(this,event.record-1,event.record-1,
TableModelEvent.ALL_COLUMNS,TableModelEvent.DELETE);
}
if (event.event==ClarionQueueEvent.EventType.FREE) {
postNS=true;
tmeAll=true;
}
if (event.event==ClarionQueueEvent.EventType.SORT) {
postNS=true;
tmeAll=true;
}
}
}
private Integer currentRows;
private int getCapturedRowCount()
{
if (isTree) return queue.records();
if (currentRows==null) currentRows=queue.records();
return currentRows;
}
public void setAssumedRows(int count) {
currentRows=count;
}
public void processModelChange(int size)
{
if (postNS) {
control.post(Event.NEWSELECTION);
postNS=false;
}
setAssumedRows(size);
if (tmeAll) {
if (assumedRows==getCapturedRowCount()) {
tme=new TableModelEvent(this,0,queue.records(),TableModelEvent.ALL_COLUMNS,TableModelEvent.UPDATE);
} else {
tme=null;
}
}
if (tme==null) tme=new TableModelEvent(this);
int sel = table.getSelectedRow();
for ( TableModelListener l : listeners) {
l.tableChanged(tme);
}
if (!hasMark) {
if (sel>=queue.records()) sel=queue.records()-1;
if (sel>=0) {
table.getSelectionModel().setSelectionInterval(sel,sel);
}
}
tme=null;
tmeAll=false;
if (queue.records()!=last_size) {
table.invalidate();
table.repaint();
last_size=queue.records();
}
}
public JTable getTable()
{
return table;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy