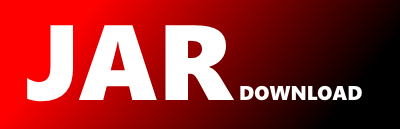
org.jclarion.clarion.util.SharedOutputStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clarion-runtime Show documentation
Show all versions of clarion-runtime Show documentation
JClarion runtime environment
The newest version!
/**
* Copyright 2010, by Andrew Barnham
*
* The contents of this file are subject to
* GNU Lesser General Public License (LGPL), v.3
* http://www.gnu.org/licenses/lgpl.txt
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied.
*/
package org.jclarion.clarion.util;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
public class SharedOutputStream extends OutputStream
{
private byte target[];
private int pos;
private int size;
public SharedOutputStream()
{
this(new byte[256],0,256);
}
public SharedOutputStream(int size)
{
this(new byte[size],0,size);
}
public SharedOutputStream(byte target[],int pos,int size)
{
this.target=target;
this.pos=pos;
this.size=size+pos;
}
public SharedInputStream getInputStream()
{
return new SharedInputStream(target,0,pos);
}
public SharedInputStream getInputStream(int size)
{
return new SharedInputStream(target,0,size);
}
public SharedInputStream getFullInputStream()
{
return new SharedInputStream(target,0,target.length,pos);
}
public SharedOutputStream like()
{
byte bits[] = toByteArray();
SharedOutputStream like = new SharedOutputStream(bits,bits.length,0);
return like;
}
@Override
public void write(int b)
{
resize(pos+1);
target[pos++]=(byte)b;
}
@Override
public void write(byte[] b, int off, int len)
{
resize(pos+len);
System.arraycopy(b,off,target,pos,len);
pos+=len;
}
@Override
public void write(byte[] b)
{
write(b,0,b.length);
}
public void pack()
{
target=toByteArray();
size=target.length;
}
public void reset()
{
pos=0;
}
public void skip(long ofs)
{
pos+=ofs;
}
public byte[] getBytes()
{
return target;
}
public int getLastByte()
{
if (pos==0) return -1;
return target[pos-1];
}
public byte getLastByteNoCheck()
{
return target[pos-1];
}
public void shrink()
{
if (pos>0) pos--;
}
public boolean endsWith(byte test[])
{
int t_scan = pos-test.length;
if (t_scan<0) return false;
for (byte t : test ) {
if (t!=target[t_scan++]) return false;
}
return true;
}
public void shrink(byte test[])
{
int scan=test.length;
while (pos>0 && scan>0) {
scan--;
if (target[pos-1]!=test[scan]) return;
pos--;
}
}
public boolean endsWith(byte test)
{
if (pos==0) return false;
return target[pos-1]==test;
}
public int getSize()
{
return pos;
}
public byte[] toByteArray()
{
byte b[]=new byte[pos];
System.arraycopy(target,0,b,0,pos);
return b;
}
private void resize(int reqSize) {
if (reqSize<=size) return;
if (size>4)&0x0f]);
sb.append(hex[(b)&0x0f]);
}
return sb.toString();
}
public void readAll(InputStream is) throws IOException
{
while ( true ) {
if (pos==target.length) {
if (target.length==0) {
resize(8192);
} else {
resize(target.length*2);
}
}
int r = is.read(target,pos,target.length-pos);
if (r<=0) return;
pos+=r;
}
}
public int readSome(InputStream is,int len) throws IOException
{
int total=0;
while ( true ) {
if (len==0) return total;
if (pos==target.length) {
if (target.length==0) {
resize(8192);
} else {
resize(target.length*2);
}
}
int thisRead = target.length-pos;
if (thisRead>len) thisRead=len;
int r = is.read(target,pos,thisRead);
if (r<=0) return total;
pos+=r;
total+=r;
len-=r;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy