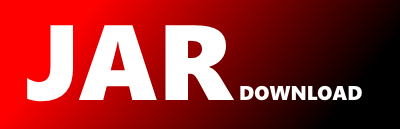
org.jclouds.collect.PagedIterables Maven / Gradle / Ivy
/**
* Licensed to jclouds, Inc. (jclouds) under one or more
* contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. jclouds licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.jclouds.collect;
import static com.google.common.base.Preconditions.checkNotNull;
import java.util.Iterator;
import com.google.common.annotations.Beta;
import com.google.common.base.Function;
import com.google.common.base.Objects;
import com.google.common.collect.AbstractIterator;
import com.google.common.collect.ImmutableSet;
/**
* Utilities for using {@link PagedIterable}s.
*
* @author Adrian Cole
*/
@Beta
public class PagedIterables {
/**
* @param only
* the only page of data
*
* @return iterable with only the one page
*/
public static PagedIterable onlyPage(final IterableWithMarker only) {
return new PagedIterable() {
public Iterator> iterator() {
return ImmutableSet.of(only).iterator();
}
};
}
/**
* @see #onlyPage(IterableWithMarker)
*/
@Deprecated
public static PagedIterable of(IterableWithMarker only) {
return onlyPage(only);
}
/**
*
*
* @param initial
* the initial set current data
* @param markerToNext
* produces the next set based on the marker
*
* @return iterable current data which continues if the user iterates beyond
* the first page
*/
public static PagedIterable advance(final IterableWithMarker initial,
final Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy