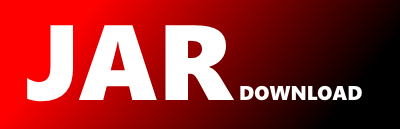
org.jcodec.containers.mp4.boxes.ChannelBox Maven / Gradle / Ivy
package org.jcodec.containers.mp4.boxes;
import java.io.DataOutput;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.jcodec.containers.mp4.io.Input;
import org.jcodec.containers.mp4.io.Parser;
/**
* This class is part of JCodec ( www.jcodec.org ) This software is distributed
* under FreeBSD License
*
* @author stan
*
*/
public class ChannelBox extends FullBox {
private int channelLayout;
private int channelBitmap;
private List descriptions = new ArrayList();
public static class ChannelDescription {
private int channelLabel;
private int channelFlags;
private float[] coordinates = new float[3];
private ChannelDescription(int channelLabel, int channelFlags, float[] coordinates) {
this.channelLabel = channelLabel;
this.channelFlags = channelFlags;
this.coordinates = coordinates;
}
public int getChannelLabel() {
return channelLabel;
}
public int getChannelFlags() {
return channelFlags;
}
public float[] getCoordinates() {
return coordinates;
}
}
public ChannelBox(Header atom) {
super(atom);
}
public ChannelBox() {
super(new Header(fourcc()));
}
public static String fourcc() {
return "chan";
}
public void parse(Input input) throws IOException {
super.parse(input);
channelLayout = (int) Parser.readInt32(input);
channelBitmap = (int) Parser.readInt32(input);
long numDescriptions = Parser.readInt32(input);
for (int i = 0; i < numDescriptions; i++) {
descriptions.add(new ChannelDescription((int) Parser.readInt32(input), (int) Parser.readInt32(input),
new float[] { Float.intBitsToFloat((int) Parser.readInt32(input)),
Float.intBitsToFloat((int) Parser.readInt32(input)),
Float.intBitsToFloat((int) Parser.readInt32(input)) }));
}
}
protected void doWrite(DataOutput out) throws IOException {
super.doWrite(out);
out.writeInt(channelLayout);
out.writeInt(channelBitmap);
out.writeInt(descriptions.size());
List descriptions2 = descriptions;
for (ChannelDescription channelDescription : descriptions2) {
out.writeInt(channelDescription.getChannelLabel());
out.writeInt(channelDescription.getChannelFlags());
out.writeFloat(channelDescription.getCoordinates()[0]);
out.writeFloat(channelDescription.getCoordinates()[1]);
out.writeFloat(channelDescription.getCoordinates()[2]);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy