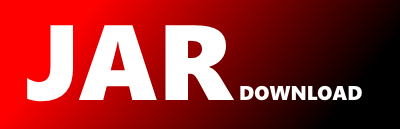
jcommon.extract.DefaultVariableProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of init Show documentation
Show all versions of init Show documentation
Java library for simple extracting and processing of embedded resources at runtime.
The newest version!
package jcommon.extract;
import jcommon.core.StringUtil;
import java.util.HashMap;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Holds variables, their values, and processes strings replacing variables with.
*
* @author David Hoyt
*/
public class DefaultVariableProcessor implements IVariableProcessor {
//
protected static final Pattern
REGEX_VAR = VariableProcessorFactory.REGEX_VAR
;
//
//
private Map vars = new HashMap(8, 0.5f);
//
//
public DefaultVariableProcessor() {
init();
}
protected void init() {
}
//
//
@Override
public boolean addVariables(final Map Variables) {
if (Variables == null || Variables.isEmpty())
return true;
for(Map.Entry e : Variables.entrySet())
vars.put(e.getKey(), e.getValue());
return true;
}
@Override
public boolean saveVariable(final String Name, String Value) {
if (Value == null)
Value = StringUtil.empty;
vars.put(Name, Matcher.quoteReplacement(Value));
return true;
}
@Override
public boolean removeVariable(final String Name) {
if (vars.isEmpty() || !vars.containsKey(Name))
return false;
vars.remove(Name);
return true;
}
@Override
public boolean clearVariables() {
vars.clear();
return true;
}
@Override
public boolean hasVariable(final String Name) {
return (vars != null && !vars.isEmpty() && vars.containsKey(Name));
}
@Override
public String findValue(final String Name) {
if (vars.isEmpty() || !vars.containsKey(Name))
return null;
return vars.get(Name);
}
@Override
public String process(final String value) {
final Matcher m = REGEX_VAR.matcher(value);
if (!m.find())
return value;
//We found a variable, so loop through all the matches
//and do our find/replace.
final StringBuffer sb = new StringBuffer(128);
do {
if (m.groupCount() < 1)
continue;
String varName = m.group(1);
String varValue = vars.get(varName);
if (varValue == null)
throw new MissingVariableException("Unable to locate variable: " + varName + ". Variable names are case-sensitive.");
m.appendReplacement(sb, varValue);
} while(m.find());
m.appendTail(sb);
return sb.toString();
}
//
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy