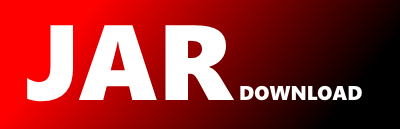
JCusolverJNI.src.JCusolverRf.hpp Maven / Gradle / Ivy
/*
* JCusolver - Java bindings for CUSOLVER, the NVIDIA CUDA solver
* library, to be used with JCuda
*
* Copyright (c) 2010-2016 Marco Hutter - http://www.jcuda.org
*
* Permission is hereby granted, free of charge, to any person
* obtaining a copy of this software and associated documentation
* files (the "Software"), to deal in the Software without
* restriction, including without limitation the rights to use,
* copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the
* Software is furnished to do so, subject to the following
* conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
* OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
* HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
* WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
* FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
* OTHER DEALINGS IN THE SOFTWARE.
*/
/* DO NOT EDIT THIS FILE - it is machine generated */
#include
/* Header for class jcuda_jcusolver_JCusolverRf */
#ifndef _Included_jcuda_jcusolver_JCusolverRf
#define _Included_jcuda_jcusolver_JCusolverRf
#ifdef __cplusplus
extern "C" {
#endif
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfCreateNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfCreateNative
(JNIEnv *, jclass, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfDestroyNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfDestroyNative
(JNIEnv *, jclass, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfGetMatrixFormatNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;[I[I)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfGetMatrixFormatNative
(JNIEnv *, jclass, jobject, jintArray, jintArray);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfSetMatrixFormatNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;II)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfSetMatrixFormatNative
(JNIEnv *, jclass, jobject, jint, jint);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfSetNumericPropertiesNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;DD)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfSetNumericPropertiesNative
(JNIEnv *, jclass, jobject, jdouble, jdouble);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfGetNumericPropertiesNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;[D[D)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfGetNumericPropertiesNative
(JNIEnv *, jclass, jobject, jdoubleArray, jdoubleArray);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfGetNumericBoostReportNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;[I)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfGetNumericBoostReportNative
(JNIEnv *, jclass, jobject, jintArray);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfSetAlgsNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;II)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfSetAlgsNative
(JNIEnv *, jclass, jobject, jint, jint);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfGetAlgsNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;[I[I)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfGetAlgsNative
(JNIEnv *, jclass, jobject, jintArray, jintArray);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfGetResetValuesFastModeNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;[I)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfGetResetValuesFastModeNative
(JNIEnv *, jclass, jobject, jintArray);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfSetResetValuesFastModeNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;I)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfSetResetValuesFastModeNative
(JNIEnv *, jclass, jobject, jint);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfSetupHostNative
* Signature: (IILjcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;ILjcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;ILjcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/jcusolver/cusolverRfHandle;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfSetupHostNative
(JNIEnv *, jclass, jint, jint, jobject, jobject, jobject, jint, jobject, jobject, jobject, jint, jobject, jobject, jobject, jobject, jobject, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfSetupDeviceNative
* Signature: (IILjcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;ILjcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;ILjcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/jcusolver/cusolverRfHandle;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfSetupDeviceNative
(JNIEnv *, jclass, jint, jint, jobject, jobject, jobject, jint, jobject, jobject, jobject, jint, jobject, jobject, jobject, jobject, jobject, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfResetValuesNative
* Signature: (IILjcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/jcusolver/cusolverRfHandle;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfResetValuesNative
(JNIEnv *, jclass, jint, jint, jobject, jobject, jobject, jobject, jobject, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfAnalyzeNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfAnalyzeNative
(JNIEnv *, jclass, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfRefactorNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfRefactorNative
(JNIEnv *, jclass, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfAccessBundledFactorsDeviceNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfAccessBundledFactorsDeviceNative
(JNIEnv *, jclass, jobject, jobject, jobject, jobject, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfExtractBundledFactorsHostNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfExtractBundledFactorsHostNative
(JNIEnv *, jclass, jobject, jobject, jobject, jobject, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfExtractSplitFactorsHostNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfExtractSplitFactorsHostNative
(JNIEnv *, jclass, jobject, jobject, jobject, jobject, jobject, jobject, jobject, jobject, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfSolveNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;Ljcuda/Pointer;Ljcuda/Pointer;ILjcuda/Pointer;ILjcuda/Pointer;I)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfSolveNative
(JNIEnv *, jclass, jobject, jobject, jobject, jint, jobject, jint, jobject, jint);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfBatchSetupHostNative
* Signature: (IIILjcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;ILjcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;ILjcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/jcusolver/cusolverRfHandle;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfBatchSetupHostNative
(JNIEnv *, jclass, jint, jint, jint, jobject, jobject, jobject, jint, jobject, jobject, jobject, jint, jobject, jobject, jobject, jobject, jobject, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfBatchResetValuesNative
* Signature: (IIILjcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/Pointer;Ljcuda/jcusolver/cusolverRfHandle;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfBatchResetValuesNative
(JNIEnv *, jclass, jint, jint, jint, jobject, jobject, jobject, jobject, jobject, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfBatchAnalyzeNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfBatchAnalyzeNative
(JNIEnv *, jclass, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfBatchRefactorNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfBatchRefactorNative
(JNIEnv *, jclass, jobject);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfBatchSolveNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;Ljcuda/Pointer;Ljcuda/Pointer;ILjcuda/Pointer;ILjcuda/Pointer;I)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfBatchSolveNative
(JNIEnv *, jclass, jobject, jobject, jobject, jint, jobject, jint, jobject, jint);
/*
* Class: jcuda_jcusolver_JCusolverRf
* Method: cusolverRfBatchZeroPivotNative
* Signature: (Ljcuda/jcusolver/cusolverRfHandle;Ljcuda/Pointer;)I
*/
JNIEXPORT jint JNICALL Java_jcuda_jcusolver_JCusolverRf_cusolverRfBatchZeroPivotNative
(JNIEnv *, jclass, jobject, jobject);
#ifdef __cplusplus
}
#endif
#endif
© 2015 - 2024 Weber Informatics LLC | Privacy Policy