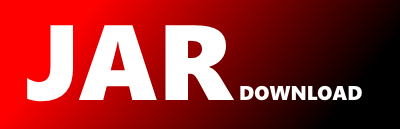
org.jdal.logic.CollectionPersistenceService Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2009-2012 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jdal.logic;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang.StringUtils;
import org.jdal.dao.Dao;
import org.jdal.dao.Page;
import org.jdal.util.BeanUtils;
import org.jdal.util.comparator.PropertyComparator;
/**
* Persistence Service using a Collection as entity store.
*
* @author Jose Luis Martin - ([email protected])
*/
public class CollectionPersistenceService implements Dao {
private Collection collection;
private String propertyKey = "id";
public CollectionPersistenceService() {
collection = new ArrayList();
}
/**
* @param collection
*/
public CollectionPersistenceService(Collection collection) {
this.collection = collection;
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
public Page getPage(Page page) {
if (collection.isEmpty()) {
page.setCount(0);
page.setData(new ArrayList());
return page;
}
List list = (List) new ArrayList(collection);
int startIndex = page.getStartIndex() < list.size() ? page.getStartIndex() : list.size() - 1;
int toIndex = (page.getStartIndex() + page.getPageSize());
toIndex = toIndex < list.size() ? toIndex : list.size();
if (!StringUtils.isEmpty(page.getSortName())) {
Collections.sort(list, new PropertyComparator(page.getSortName()));
if (page.getOrder() == Page.Order.DESC)
Collections.reverse(list);
}
page.setData(list.subList(startIndex, toIndex));
page.setCount(list.size());
return page;
}
/**
* {@inheritDoc}
*/
public List getKeys(Page page) {
List keys = new ArrayList();
Page keyPage = page.clone();
getPage(keyPage);
for (T model : keyPage.getData()) {
Object value = BeanUtils.getProperty(model, propertyKey);
if (value != null)
keys.add((Serializable) value);
}
return keys;
}
/**
* {@inheritDoc}
*/
public T initialize(T entity, int depth) {
return entity;
}
/**
* {@inheritDoc}
*/
public T initialize(T entity) {
return entity;
}
/**
* {@inheritDoc}
*/
public T save(T entity) {
if ( collection.contains(entity)) {
collection.remove(entity);
}
collection.add(entity);
return entity;
}
/**
* {@inheritDoc}
*/
public void delete(T entity) {
collection.remove(entity);
}
/**
* {@inheritDoc}
*/
public void deleteById(PK id) {
collection.remove(get(id));
}
/**
* {@inheritDoc}
*/
public List getAll() {
return new ArrayList(collection);
}
/**
* {@inheritDoc}
*/
public Collection save(Collection collection) {
for (T model : collection) {
save(model);
}
return collection;
}
/**
* {@inheritDoc}
*/
public void delete(Collection collection) {
for(T model : collection)
delete(model);
}
/**
* {@inheritDoc}
*/
public void deleteById(Collection ids) {
for (PK id : ids)
deleteById(id);
}
/**
* {@inheritDoc}
*/
public T get(PK id) {
for (T model : collection) {
Object value = BeanUtils.getProperty(model, propertyKey);
if (value != null && value.equals(id))
return model;
}
return null;
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
public E get(PK id, Class clazz) {
return (E) get(id);
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
public List getAll(Class clazz) {
return (List) getAll();
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
public Class getEntityClass() {
return (Class) (collection.isEmpty() ? null : collection.iterator().next().getClass());
}
/**
* @return the collection
*/
public Collection getCollection() {
return collection;
}
/**
* @param collection the collection to set
*/
public void setCollection(Collection collection) {
this.collection = collection;
}
/**
* @return the propertyKey
*/
public String getPropertyKey() {
return propertyKey;
}
/**
* @param propertyKey the propertyKey to set
*/
public void setPropertyKey(String propertyKey) {
this.propertyKey = propertyKey;
}
/**
* {@inheritDoc}
*/
@Override
public boolean exists(PK id) {
return get(id) != null;
}
/**
* {@inheritDoc}
*/
@Override
public List findByNamedQuery(String queryName,
Map queryParams) {
throw new UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy