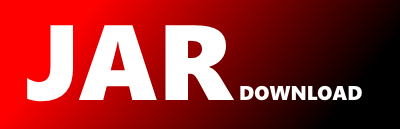
org.jdal.logic.ContextPersistentManager Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2009-2012 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jdal.logic;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import org.jdal.dao.Dao;
import org.jdal.dao.Page;
import org.springframework.beans.factory.annotation.Autowired;
/**
* Bulk all generics in one object to avoid excesive parametrization.
*
* @author Jose Luis Martin
*/
public class ContextPersistentManager implements Dao
© 2015 - 2025 Weber Informatics LLC | Privacy Policy