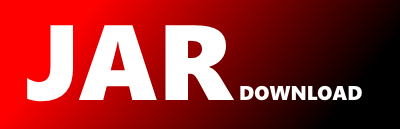
org.skife.jdbi.v2.SqlScriptLexer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdbi Show documentation
Show all versions of jdbi Show documentation
jDBI is designed to provide convenient tabular data access in
Java(tm). It uses the Java collections framework for query
results, provides a convenient means of externalizing sql
statements, and provides named parameter support for any database
being used.
// $ANTLR 3.4 org/skife/jdbi/v2/SqlScriptLexer.g 2016-08-03 15:57:59
package org.skife.jdbi.v2;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked"})
public class SqlScriptLexer extends Lexer {
public static final int EOF=-1;
public static final int COMMENT=4;
public static final int ESCAPE_SEQUENCE=5;
public static final int LITERAL=6;
public static final int MULTI_LINE_COMMENT=7;
public static final int NEWLINE=8;
public static final int NEWLINES=9;
public static final int OTHER=10;
public static final int QUOTED_TEXT=11;
public static final int SEMICOLON=12;
@Override
public void reportError(RecognitionException e) {
throw new IllegalArgumentException(e);
}
// delegates
// delegators
public Lexer[] getDelegates() {
return new Lexer[] {};
}
public SqlScriptLexer() {}
public SqlScriptLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public SqlScriptLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
public String getGrammarFileName() { return "org/skife/jdbi/v2/SqlScriptLexer.g"; }
// $ANTLR start "COMMENT"
public final void mCOMMENT() throws RecognitionException {
try {
int _type = COMMENT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/skife/jdbi/v2/SqlScriptLexer.g:15:5: ( '--' (~ ( NEWLINE ) )* | '//' (~ ( NEWLINE ) )* | '#' (~ ( NEWLINE ) )* )
int alt4=3;
switch ( input.LA(1) ) {
case '-':
{
alt4=1;
}
break;
case '/':
{
alt4=2;
}
break;
case '#':
{
alt4=3;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 4, 0, input);
throw nvae;
}
switch (alt4) {
case 1 :
// org/skife/jdbi/v2/SqlScriptLexer.g:15:7: '--' (~ ( NEWLINE ) )*
{
match("--");
// org/skife/jdbi/v2/SqlScriptLexer.g:15:12: (~ ( NEWLINE ) )*
loop1:
do {
int alt1=2;
int LA1_0 = input.LA(1);
if ( ((LA1_0 >= '\u0000' && LA1_0 <= '\t')||(LA1_0 >= '\u000B' && LA1_0 <= '\f')||(LA1_0 >= '\u000E' && LA1_0 <= '\uFFFF')) ) {
alt1=1;
}
switch (alt1) {
case 1 :
// org/skife/jdbi/v2/SqlScriptLexer.g:
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '\t')||(input.LA(1) >= '\u000B' && input.LA(1) <= '\f')||(input.LA(1) >= '\u000E' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop1;
}
} while (true);
}
break;
case 2 :
// org/skife/jdbi/v2/SqlScriptLexer.g:16:7: '//' (~ ( NEWLINE ) )*
{
match("//");
// org/skife/jdbi/v2/SqlScriptLexer.g:16:12: (~ ( NEWLINE ) )*
loop2:
do {
int alt2=2;
int LA2_0 = input.LA(1);
if ( ((LA2_0 >= '\u0000' && LA2_0 <= '\t')||(LA2_0 >= '\u000B' && LA2_0 <= '\f')||(LA2_0 >= '\u000E' && LA2_0 <= '\uFFFF')) ) {
alt2=1;
}
switch (alt2) {
case 1 :
// org/skife/jdbi/v2/SqlScriptLexer.g:
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '\t')||(input.LA(1) >= '\u000B' && input.LA(1) <= '\f')||(input.LA(1) >= '\u000E' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop2;
}
} while (true);
}
break;
case 3 :
// org/skife/jdbi/v2/SqlScriptLexer.g:17:7: '#' (~ ( NEWLINE ) )*
{
match('#');
// org/skife/jdbi/v2/SqlScriptLexer.g:17:12: (~ ( NEWLINE ) )*
loop3:
do {
int alt3=2;
int LA3_0 = input.LA(1);
if ( ((LA3_0 >= '\u0000' && LA3_0 <= '\t')||(LA3_0 >= '\u000B' && LA3_0 <= '\f')||(LA3_0 >= '\u000E' && LA3_0 <= '\uFFFF')) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// org/skife/jdbi/v2/SqlScriptLexer.g:
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '\t')||(input.LA(1) >= '\u000B' && input.LA(1) <= '\f')||(input.LA(1) >= '\u000E' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop3;
}
} while (true);
skip();
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COMMENT"
// $ANTLR start "MULTI_LINE_COMMENT"
public final void mMULTI_LINE_COMMENT() throws RecognitionException {
try {
int _type = MULTI_LINE_COMMENT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/skife/jdbi/v2/SqlScriptLexer.g:22:5: ( '/*' ( options {greedy=false; } : . )* '*/' )
// org/skife/jdbi/v2/SqlScriptLexer.g:22:7: '/*' ( options {greedy=false; } : . )* '*/'
{
match("/*");
// org/skife/jdbi/v2/SqlScriptLexer.g:22:12: ( options {greedy=false; } : . )*
loop5:
do {
int alt5=2;
int LA5_0 = input.LA(1);
if ( (LA5_0=='*') ) {
int LA5_1 = input.LA(2);
if ( (LA5_1=='/') ) {
alt5=2;
}
else if ( ((LA5_1 >= '\u0000' && LA5_1 <= '.')||(LA5_1 >= '0' && LA5_1 <= '\uFFFF')) ) {
alt5=1;
}
}
else if ( ((LA5_0 >= '\u0000' && LA5_0 <= ')')||(LA5_0 >= '+' && LA5_0 <= '\uFFFF')) ) {
alt5=1;
}
switch (alt5) {
case 1 :
// org/skife/jdbi/v2/SqlScriptLexer.g:22:38: .
{
matchAny();
}
break;
default :
break loop5;
}
} while (true);
match("*/");
skip();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MULTI_LINE_COMMENT"
// $ANTLR start "NEWLINES"
public final void mNEWLINES() throws RecognitionException {
try {
int _type = NEWLINES;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/skife/jdbi/v2/SqlScriptLexer.g:26:5: ( ( NEWLINE )+ )
// org/skife/jdbi/v2/SqlScriptLexer.g:26:7: ( NEWLINE )+
{
// org/skife/jdbi/v2/SqlScriptLexer.g:26:7: ( NEWLINE )+
int cnt6=0;
loop6:
do {
int alt6=2;
switch ( input.LA(1) ) {
case '\n':
case '\r':
{
alt6=1;
}
break;
}
switch (alt6) {
case 1 :
// org/skife/jdbi/v2/SqlScriptLexer.g:
{
if ( input.LA(1)=='\n'||input.LA(1)=='\r' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt6 >= 1 ) break loop6;
EarlyExitException eee =
new EarlyExitException(6, input);
throw eee;
}
cnt6++;
} while (true);
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NEWLINES"
// $ANTLR start "NEWLINE"
public final void mNEWLINE() throws RecognitionException {
try {
// org/skife/jdbi/v2/SqlScriptLexer.g:30:5: ( ( '\\n' | '\\r' ) )
// org/skife/jdbi/v2/SqlScriptLexer.g:
{
if ( input.LA(1)=='\n'||input.LA(1)=='\r' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NEWLINE"
// $ANTLR start "QUOTED_TEXT"
public final void mQUOTED_TEXT() throws RecognitionException {
try {
int _type = QUOTED_TEXT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/skife/jdbi/v2/SqlScriptLexer.g:34:5: ( ( '\\'' ( ESCAPE_SEQUENCE |~ '\\'' )* '\\'' ) )
// org/skife/jdbi/v2/SqlScriptLexer.g:34:9: ( '\\'' ( ESCAPE_SEQUENCE |~ '\\'' )* '\\'' )
{
// org/skife/jdbi/v2/SqlScriptLexer.g:34:9: ( '\\'' ( ESCAPE_SEQUENCE |~ '\\'' )* '\\'' )
// org/skife/jdbi/v2/SqlScriptLexer.g:34:10: '\\'' ( ESCAPE_SEQUENCE |~ '\\'' )* '\\''
{
match('\'');
// org/skife/jdbi/v2/SqlScriptLexer.g:34:15: ( ESCAPE_SEQUENCE |~ '\\'' )*
loop7:
do {
int alt7=3;
int LA7_0 = input.LA(1);
if ( (LA7_0=='\\') ) {
int LA7_2 = input.LA(2);
if ( (LA7_2=='\'') ) {
int LA7_4 = input.LA(3);
if ( ((LA7_4 >= '\u0000' && LA7_4 <= '\uFFFF')) ) {
alt7=1;
}
else {
alt7=2;
}
}
else if ( ((LA7_2 >= '\u0000' && LA7_2 <= '&')||(LA7_2 >= '(' && LA7_2 <= '\uFFFF')) ) {
alt7=2;
}
}
else if ( ((LA7_0 >= '\u0000' && LA7_0 <= '&')||(LA7_0 >= '(' && LA7_0 <= '[')||(LA7_0 >= ']' && LA7_0 <= '\uFFFF')) ) {
alt7=2;
}
switch (alt7) {
case 1 :
// org/skife/jdbi/v2/SqlScriptLexer.g:34:16: ESCAPE_SEQUENCE
{
mESCAPE_SEQUENCE();
}
break;
case 2 :
// org/skife/jdbi/v2/SqlScriptLexer.g:34:34: ~ '\\''
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '&')||(input.LA(1) >= '(' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop7;
}
} while (true);
match('\'');
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "QUOTED_TEXT"
// $ANTLR start "ESCAPE_SEQUENCE"
public final void mESCAPE_SEQUENCE() throws RecognitionException {
try {
// org/skife/jdbi/v2/SqlScriptLexer.g:38:5: ( '\\\\' '\\'' )
// org/skife/jdbi/v2/SqlScriptLexer.g:38:8: '\\\\' '\\''
{
match('\\');
match('\'');
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ESCAPE_SEQUENCE"
// $ANTLR start "SEMICOLON"
public final void mSEMICOLON() throws RecognitionException {
try {
int _type = SEMICOLON;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/skife/jdbi/v2/SqlScriptLexer.g:42:5: ( ';' )
// org/skife/jdbi/v2/SqlScriptLexer.g:42:8: ';'
{
match(';');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SEMICOLON"
// $ANTLR start "LITERAL"
public final void mLITERAL() throws RecognitionException {
try {
int _type = LITERAL;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/skife/jdbi/v2/SqlScriptLexer.g:46:5: ( ( 'a' .. 'z' | 'A' .. 'Z' | ' ' | '\\t' | '0' .. '9' | ',' | '*' | '.' | '@' | '_' | '!' | '=' | '(' | ')' | '[' | ']' )+ )
// org/skife/jdbi/v2/SqlScriptLexer.g:46:8: ( 'a' .. 'z' | 'A' .. 'Z' | ' ' | '\\t' | '0' .. '9' | ',' | '*' | '.' | '@' | '_' | '!' | '=' | '(' | ')' | '[' | ']' )+
{
// org/skife/jdbi/v2/SqlScriptLexer.g:46:8: ( 'a' .. 'z' | 'A' .. 'Z' | ' ' | '\\t' | '0' .. '9' | ',' | '*' | '.' | '@' | '_' | '!' | '=' | '(' | ')' | '[' | ']' )+
int cnt8=0;
loop8:
do {
int alt8=2;
switch ( input.LA(1) ) {
case '\t':
case ' ':
case '!':
case '(':
case ')':
case '*':
case ',':
case '.':
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
case '=':
case '@':
case 'A':
case 'B':
case 'C':
case 'D':
case 'E':
case 'F':
case 'G':
case 'H':
case 'I':
case 'J':
case 'K':
case 'L':
case 'M':
case 'N':
case 'O':
case 'P':
case 'Q':
case 'R':
case 'S':
case 'T':
case 'U':
case 'V':
case 'W':
case 'X':
case 'Y':
case 'Z':
case '[':
case ']':
case '_':
case 'a':
case 'b':
case 'c':
case 'd':
case 'e':
case 'f':
case 'g':
case 'h':
case 'i':
case 'j':
case 'k':
case 'l':
case 'm':
case 'n':
case 'o':
case 'p':
case 'q':
case 'r':
case 's':
case 't':
case 'u':
case 'v':
case 'w':
case 'x':
case 'y':
case 'z':
{
alt8=1;
}
break;
}
switch (alt8) {
case 1 :
// org/skife/jdbi/v2/SqlScriptLexer.g:
{
if ( input.LA(1)=='\t'||(input.LA(1) >= ' ' && input.LA(1) <= '!')||(input.LA(1) >= '(' && input.LA(1) <= '*')||input.LA(1)==','||input.LA(1)=='.'||(input.LA(1) >= '0' && input.LA(1) <= '9')||input.LA(1)=='='||(input.LA(1) >= '@' && input.LA(1) <= '[')||input.LA(1)==']'||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt8 >= 1 ) break loop8;
EarlyExitException eee =
new EarlyExitException(8, input);
throw eee;
}
cnt8++;
} while (true);
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LITERAL"
// $ANTLR start "OTHER"
public final void mOTHER() throws RecognitionException {
try {
int _type = OTHER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// org/skife/jdbi/v2/SqlScriptLexer.g:51:5: ( . )
// org/skife/jdbi/v2/SqlScriptLexer.g:51:8: .
{
matchAny();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "OTHER"
public void mTokens() throws RecognitionException {
// org/skife/jdbi/v2/SqlScriptLexer.g:1:8: ( COMMENT | MULTI_LINE_COMMENT | NEWLINES | QUOTED_TEXT | SEMICOLON | LITERAL | OTHER )
int alt9=7;
int LA9_0 = input.LA(1);
if ( (LA9_0=='-') ) {
switch ( input.LA(2) ) {
case '-':
{
alt9=1;
}
break;
default:
alt9=7;
}
}
else if ( (LA9_0=='/') ) {
switch ( input.LA(2) ) {
case '/':
{
alt9=1;
}
break;
case '*':
{
alt9=2;
}
break;
default:
alt9=7;
}
}
else if ( (LA9_0=='#') ) {
alt9=1;
}
else if ( (LA9_0=='\n'||LA9_0=='\r') ) {
alt9=3;
}
else if ( (LA9_0=='\'') ) {
int LA9_5 = input.LA(2);
if ( ((LA9_5 >= '\u0000' && LA9_5 <= '\uFFFF')) ) {
alt9=4;
}
else {
alt9=7;
}
}
else if ( (LA9_0==';') ) {
alt9=5;
}
else if ( (LA9_0=='\t'||(LA9_0 >= ' ' && LA9_0 <= '!')||(LA9_0 >= '(' && LA9_0 <= '*')||LA9_0==','||LA9_0=='.'||(LA9_0 >= '0' && LA9_0 <= '9')||LA9_0=='='||(LA9_0 >= '@' && LA9_0 <= '[')||LA9_0==']'||LA9_0=='_'||(LA9_0 >= 'a' && LA9_0 <= 'z')) ) {
alt9=6;
}
else if ( ((LA9_0 >= '\u0000' && LA9_0 <= '\b')||(LA9_0 >= '\u000B' && LA9_0 <= '\f')||(LA9_0 >= '\u000E' && LA9_0 <= '\u001F')||LA9_0=='\"'||(LA9_0 >= '$' && LA9_0 <= '&')||LA9_0=='+'||LA9_0==':'||LA9_0=='<'||(LA9_0 >= '>' && LA9_0 <= '?')||LA9_0=='\\'||LA9_0=='^'||LA9_0=='`'||(LA9_0 >= '{' && LA9_0 <= '\uFFFF')) ) {
alt9=7;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 9, 0, input);
throw nvae;
}
switch (alt9) {
case 1 :
// org/skife/jdbi/v2/SqlScriptLexer.g:1:10: COMMENT
{
mCOMMENT();
}
break;
case 2 :
// org/skife/jdbi/v2/SqlScriptLexer.g:1:18: MULTI_LINE_COMMENT
{
mMULTI_LINE_COMMENT();
}
break;
case 3 :
// org/skife/jdbi/v2/SqlScriptLexer.g:1:37: NEWLINES
{
mNEWLINES();
}
break;
case 4 :
// org/skife/jdbi/v2/SqlScriptLexer.g:1:46: QUOTED_TEXT
{
mQUOTED_TEXT();
}
break;
case 5 :
// org/skife/jdbi/v2/SqlScriptLexer.g:1:58: SEMICOLON
{
mSEMICOLON();
}
break;
case 6 :
// org/skife/jdbi/v2/SqlScriptLexer.g:1:68: LITERAL
{
mLITERAL();
}
break;
case 7 :
// org/skife/jdbi/v2/SqlScriptLexer.g:1:76: OTHER
{
mOTHER();
}
break;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy