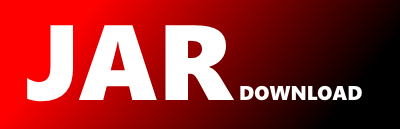
org.jdice.calc.internal.CacheExtension Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jcalc Show documentation
Show all versions of jcalc Show documentation
Fluent Java API for easier work with numbers, writing formula and calculations in Java.
The newest version!
/*
* Copyright 2014 Davor Sauer
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jdice.calc.internal;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map.Entry;
import org.jdice.calc.CalculatorException;
import org.jdice.calc.Function;
import org.jdice.calc.NumConverter;
import org.jdice.calc.Operator;
import org.jdice.calc.Properties;
import org.jdice.calc.SingletonExtension;
/**
* Global cache for operator, function, NumConverter classes and instances
*
* @author Davor Sauer
*
*/
public class CacheExtension {
private static volatile UseExtension cacheData = new UseExtension();
private static volatile HashMap converterCache = new HashMap();
private static volatile boolean numConverterPropLoaded = false;
public static void setOperator(Class extends Operator> operatorClass) {
cacheData.registerOperator(operatorClass);
}
public static HashMap> getOperatorSymbols() {
return cacheData.getOperatorSymbols();
}
public static Operator getOperator(Class extends Operator> operatorClass) {
return cacheData.getOperator(operatorClass);
}
public static Operator getOperator(String operator) {
return cacheData.getOperator(operator);
}
public static HashMap, Operator> getOperators() {
return cacheData.getOperators();
}
public static void setFunction(Class extends Function> functionClass) {
cacheData.registerFunction(functionClass);
}
public static HashMap> getFunctionSymbols() {
return cacheData.getFunctionSymbols();
}
public static Function getFunction(String function) {
return cacheData.getFunction(function);
}
public static Function getFunction(Class extends Function> functionClass) {
return cacheData.getFunction(functionClass);
}
public static HashMap, Function> getFunctions() {
return cacheData.getFunctions();
}
/**
* Register custom converter class on global scope.
*
* @param customClass define class type for conversion
* @param converterClass define class which knows how to convert customClass
*/
public static void setNumConverter(Class customClass, Class extends NumConverter> converterClass) {
NumConverter nc = converterCache.get(customClass);
if (nc == null) {
synchronized (converterClass) {
nc = converterCache.get(customClass);
if (nc == null) {
try {
nc = (NumConverter) converterClass.newInstance();
}
catch (Exception e) {
throw new CalculatorException(e);
}
if (nc != null) {
if (converterClass.isAnnotationPresent(SingletonExtension.class))
converterCache.put(customClass, nc);
}
}
}
}
}
/**
* Register custom converter class on global scope.
*
* @param customClass define class type for conversion
* @param converter define instance which knows how to convert customClass
*/
public static NumConverter setNumConverter(Class customClass, NumConverter converter) {
converterCache.put(customClass, converter);
return converter;
}
public static NumConverter getNumConverter(Class> customClass) {
NumConverter nc = converterCache.get(customClass);
return nc;
}
public static NumConverter getNumConverter(Class> customClass, Class extends NumConverter> convertClass) {
if (numConverterPropLoaded == false)
loadNumConvertersFromPropertiesFile(null);
NumConverter nc = converterCache.get(convertClass);
if (nc == null) {
try {
synchronized (convertClass) {
nc = convertClass.newInstance();
if (convertClass.isAnnotationPresent(SingletonExtension.class))
converterCache.put(customClass, nc);
}
} catch (Exception e) {
throw new CalculatorException(e);
}
}
return nc;
}
public static HashMap getAllNumConverter() {
return converterCache;
}
static void loadNumConvertersFromPropertiesFile(String absolutePath) {
if (absolutePath == null) {
numConverterPropLoaded = true;
absolutePath = Properties.getGlobalPropertiesFile();
}
File propFile = new File(absolutePath);
if (propFile.exists() && propFile.isFile() && propFile.canRead()) {
java.util.Properties prop = new java.util.Properties();
FileInputStream fis = null;
try {
fis = new FileInputStream(propFile);
prop.load(fis);
loadProperties(prop);
}
catch (Exception e) {
}
finally {
if (fis != null) {
try {
fis.close();
}
catch (IOException e) {
}
}
}
}
}
public static void loadProperties(java.util.Properties prop) {
for (Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy