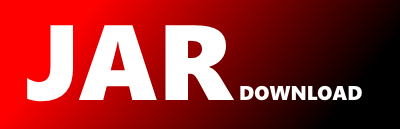
org.jdom2.test.cases.TestElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdom Show documentation
Show all versions of jdom Show documentation
A complete, Java-based solution for accessing, manipulating,
and outputting XML data
package org.jdom2.test.cases;
/*--
Copyright (C) 2000 Brett McLaughlin & Jason Hunter.
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions
are met:
1. Redistributions of source code must retain the above copyright
notice, this list of conditions, and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright
notice, this list of conditions, and the disclaimer that follows
these conditions in the documentation and/or other materials
provided with the distribution.
3. The name "JDOM" must not be used to endorse or promote products
derived from this software without prior written permission. For
written permission, please contact [email protected].
4. Products derived from this software may not be called "JDOM", nor
may "JDOM" appear in their name, without prior written permission
from the JDOM Project Management ([email protected]).
In addition, we request (but do not require) that you include in the
end-user documentation provided with the redistribution and/or in the
software itself an acknowledgement equivalent to the following:
"This product includes software developed by the
JDOM Project (http://www.jdom.org/)."
Alternatively, the acknowledgment may be graphical using the logos
available at http://www.jdom.org/images/logos.
THIS SOFTWARE IS PROVIDED ``AS IS'' AND ANY EXPRESSED OR IMPLIED
WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE JDOM AUTHORS OR THE PROJECT
CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF
USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT
OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF
SUCH DAMAGE.
This software consists of voluntary contributions made by many
individuals on behalf of the JDOM Project and was originally
created by Brett McLaughlin and
Jason Hunter . For more information on the
JDOM Project, please see .
*/
/**
* Please put a description of your test here.
*
* @author unascribed
* @version 0.1
*/
import static org.jdom2.test.util.UnitTestUtil.checkException;
import static org.jdom2.test.util.UnitTestUtil.failNoException;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertFalse;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertNull;
import static org.junit.Assert.assertTrue;
import static org.junit.Assert.fail;
import java.io.IOException;
import java.io.StringWriter;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.LinkedList;
import java.util.List;
import java.util.Set;
import org.junit.Test;
import org.junit.runner.JUnitCore;
import org.jdom2.Attribute;
import org.jdom2.AttributeType;
import org.jdom2.CDATA;
import org.jdom2.Comment;
import org.jdom2.Content;
import org.jdom2.DocType;
import org.jdom2.Document;
import org.jdom2.Element;
import org.jdom2.EntityRef;
import org.jdom2.IllegalAddException;
import org.jdom2.IllegalNameException;
import org.jdom2.Namespace;
import org.jdom2.ProcessingInstruction;
import org.jdom2.Text;
import org.jdom2.Content.CType;
import org.jdom2.filter.ContentFilter;
import org.jdom2.filter.ElementFilter;
import org.jdom2.filter.Filters;
import org.jdom2.output.Format;
import org.jdom2.output.XMLOutputter;
import org.jdom2.test.util.UnitTestUtil;
@SuppressWarnings("javadoc")
public final class TestElement {
/**
* The main method runs all the tests in the text ui
*/
public static void main(String args[]) {
JUnitCore.runClasses(TestElement.class);
}
/**
* Test the constructor for a subclass element
*/
@Test
public void test_TCC() {
Element emt = new Element() {
// change nothing
private static final long serialVersionUID = 200L;
};
assertNull(emt.getName());
}
/**
* Test the constructor for an empty element
*/
@Test
public void test_TCC___String() {
//create a new empty element
Element el = new Element("theElement");
assertTrue("wrong element name after constructor", el.getName().equals("theElement"));
assertTrue("expected NO_NAMESPACE", el.getNamespace().equals(Namespace.NO_NAMESPACE));
assertTrue("expected no child elements", el.getChildren().equals(Collections.EMPTY_LIST));
assertTrue("expected no attributes", el.getAttributes().equals(Collections.EMPTY_LIST));
//must have a name
try {
el = new Element("");
fail("allowed creation of an element with no name");
}
catch (IllegalNameException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
//name can't be null
try {
el = new Element(null);
fail("allowed creation of an element with null name");
}
catch (IllegalNameException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
//we can assume the Verifier has been called by now so we are done
}
/**
* Test the Element constructor with an name and namespace
*/
@Test
public void test_TCC___String_OrgJdomNamespace() {
//create a new empty element with a namespace
Namespace ns = Namespace.getNamespace("urn:foo");
Element el = new Element("theElement", ns);
assertTrue("wrong element name after constructor", el.getName().equals("theElement"));
assertTrue("expected urn:foo namespace", el.getNamespace().equals(Namespace.getNamespace("urn:foo")));
assertTrue("expected no child elements", el.getChildren().equals(Collections.EMPTY_LIST));
assertTrue("expected no attributes", el.getAttributes().equals(Collections.EMPTY_LIST));
//must have a name
try {
el = new Element("", ns);
fail("allowed creation of an element with no name");
}
catch (IllegalNameException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
//name can't be null
try {
el = new Element(null, ns);
fail("allowed creation of an element with null name");
}
catch (IllegalNameException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
//we can assume the Verifier has been called by now so we are done
}
/**
* Test the Element constructor with a string default namespace
*/
@Test
public void test_TCC___String_String() {
//create a new empty element with a namespace
Element el = new Element("theElement", "urn:foo");
assertTrue("wrong element name after constructor", el.getName().equals("theElement"));
assertTrue("expected urn:foo namespace", el.getNamespace().equals(Namespace.getNamespace("urn:foo")));
assertTrue("expected no child elements", el.getChildren().equals(Collections.EMPTY_LIST));
assertTrue("expected no attributes", el.getAttributes().equals(Collections.EMPTY_LIST));
//must have a name
try {
el = new Element("", "urn:foo");
fail("allowed creation of an element with no name");
}
catch (IllegalNameException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
//name can't be null
try {
el = new Element(null, "urn:foo");
fail("allowed creation of an element with null name");
}
catch (IllegalNameException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
//we can assume the Verifier has been called by now so we are done
}
/**
* Test the Element constructor with a namespace uri and prefix
*/
@Test
public void test_TCC___String_String_String() {
//create a new empty element with a namespace
Element el = new Element("theElement", "x", "urn:foo");
assertTrue("wrong element name after constructor", el.getName().equals("theElement"));
assertTrue("expected urn:foo namespace", el.getNamespace().equals(Namespace.getNamespace("x", "urn:foo")));
assertTrue("expected no child elements", el.getChildren().equals(Collections.EMPTY_LIST));
assertTrue("expected no attributes", el.getAttributes().equals(Collections.EMPTY_LIST));
//must have a name
try {
el = new Element("", "x", "urn:foo");
fail("allowed creation of an element with no name");
}
catch (IllegalNameException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
//name can't be null
try {
el = new Element(null, "x", "urn:foo");
fail("allowed creation of an element with null name");
}
catch (IllegalNameException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
//we can assume the Verifier has been called by now so we are done
}
/**
* Test the equals compares only object instances
*/
@Test
public void test_TCM__boolean_equals_Object() {
Element el = new Element("theElement", "x", "urn:foo");
Element el2 = new Element("theElement", "x", "urn:foo");
assertTrue("incorrect equals evaluation", ((Object) el).equals(el));
assertTrue("incorrect equals evaluation", !((Object) el2).equals(el));
}
/**
* Test that hasChildren only reports true for actual child elements.
*/
/*
public void test_TCM__boolean_hasChildren() {
//set up an element to test with
Element element = new Element("element", Namespace.getNamespace("http://foo"));
assertTrue("reported children when there are none", !element.hasChildren());
Attribute att1 = new Attribute("anAttribute", "boo");
element.setAttribute(att1);
assertTrue("reported children when there are none", !element.hasChildren());
//add some text
element.addContent("the text");
assertTrue("reported children when there are none", !element.hasChildren());
//add some CDATA
element.addContent(new CDATA("the text"));
assertTrue("reported children when there are none", !element.hasChildren());
//add a PI
element.addContent(new ProcessingInstruction("pi", "the text"));
assertTrue("reported children when there are none", !element.hasChildren());
//add Comment
element.addContent(new Comment("the text"));
assertTrue("reported children when there are none", !element.hasChildren());
//finally a child element
Element child1 = new Element("child1");
element.addContent(child1);
assertTrue("reported no children when there is a child element", element.hasChildren());
}
*/
/**
* Test that hasMixedContent works for varying types of possible
* child and other content
*/
@Test
public void test_TCM__boolean_hasMixedContent() {
/** No op because the method is deprecated
//set up an element to test with
Element element= new Element("element", Namespace.getNamespace("http://foo"));
assertTrue("reported mixed content when there is none", ! element.hasMixedContent());
Attribute att1 = new Attribute("anAttribute", "boo");
element.setAttribute(att1);
assertTrue("reported mixed content when there is none", ! element.hasMixedContent());
//add some text
element.addContent("the text");
assertTrue("reported mixed content when there is none", ! element.hasMixedContent());
//add some CDATA
element.addContent(new CDATA("the text"));
assertTrue("reported no mixed content when there is", element.hasMixedContent());
element= new Element("element");
//add a PI
element.addContent(new ProcessingInstruction("pi", "the text"));
assertTrue("reported mixed content when there is none", ! element.hasMixedContent());
//add Comment
element.addContent(new Comment("the text"));
assertTrue("reported no mixed content when there is", element.hasMixedContent());
element= new Element("element");
//finally a child element
Element child1= new Element("child1");
element.addContent(child1);
assertTrue("reported mixed content when there is none", ! element.hasMixedContent());
element.addContent("some text");
assertTrue("reported no mixed content when there is", element.hasMixedContent());
*/
}
/**
* Test that an Element can determine if it is a root element
*/
@Test
public void test_TCM__boolean_isRootElement() {
Element element = new Element("element");
assertTrue("incorrectly identified element as root", !element.isRootElement());
new Document(element);
assertTrue("incorrectly identified element as non root", element.isRootElement());
}
/**
* Test than an Element can remove an Attribute by name
*/
@Test
public void test_TCM__boolean_removeAttribute_String() {
Element element = new Element("test");
assertFalse(element.removeAttribute("att"));
Attribute att = new Attribute("anAttribute", "test");
element.setAttribute(att);
//make sure it's there
assertNotNull("attribute not found after add", element.getAttribute("anAttribute"));
//and remove it
assertTrue("attribute not removed", element.removeAttribute("anAttribute"));
//make sure it's not there
assertNull("attribute found after remove", element.getAttribute("anAttribute"));
assertFalse("non-existing attribute remove returned true", element.removeAttribute("anAttribute"));
}
/**
* Test than an Element can remove an Attribute by name
*/
@Test
public void test_TCM__boolean_removeAttribute_Attribute() {
Element element = new Element("test");
Attribute att = new Attribute("anAttribute", "test");
assertFalse(element.removeAttribute(att));
element.setAttribute(att);
//make sure it's there
assertNotNull("attribute not found after add", element.getAttribute("anAttribute"));
//and remove it
assertTrue("attribute not removed", element.removeAttribute(att));
//make sure it's not there
assertNull("attribute found after remove", element.getAttribute("anAttribute"));
assertFalse("non-existing attribute remove returned true", element.removeAttribute(att));
}
/**
* Test removeAttribute with a namespace
*/
@Test
public void test_TCM__boolean_removeAttribute_String_OrgJdomNamespace() {
Element element = new Element("test");
Namespace ns = Namespace.getNamespace("x", "urn:test");
assertFalse(element.removeAttribute("anAttribute", ns));
Attribute att = new Attribute("anAttribute", "test", ns);
element.setAttribute(att);
//make sure it's there
assertNotNull("attribute not found after add", element.getAttribute("anAttribute", ns));
//make sure we check namespaces - a different namespace
assertFalse("wrong attribute removed", element.removeAttribute("anAttribute", Namespace.NO_NAMESPACE));
//and remove it
assertTrue("attribute not removed", element.removeAttribute("anAttribute", ns));
//make sure it's not there
assertNull("attribute found after remove", element.getAttribute("anAttribute", ns));
}
/**
* Test removeAtttribute with a namespace uri.
*/
@Test
public void test_TCM__boolean_removeAttribute_String_String() {
/** No op because the method is deprecated
Element element = new Element("test");
Namespace ns = Namespace.getNamespace("x", "urn:test");
Attribute att = new Attribute("anAttribute", "test", ns);
element.setAttribute(att);
//make sure it's there
assertNotNull("attribute not found after add", element.getAttribute("anAttribute", ns));
//and remove it
assertTrue("attribute not removed", element.removeAttribute("anAttribute", "urn:test"));
//make sure it's not there
assertNull("attribute found after remove", element.getAttribute("anAttribute", ns));
*/
}
/**
* Test removeChild by name
*/
@Test
public void test_TCM__boolean_removeChild_String() {
Element element = new Element("element");
Element child = new Element("child");
element.addContent(child);
assertTrue("couldn't remove child content", element.removeChild("child"));
assertNull("child not removed", element.getChild("child"));
}
/**
* Test removeChild by name and namespace
*/
@Test
public void test_TCM__boolean_removeChild_String_OrgJdomNamespace() {
Namespace ns = Namespace.getNamespace("x", "urn:fudge");
Element element = new Element("element");
Element child = new Element("child", ns);
Element child2 = new Element("child", ns);
element.addContent(child);
assertTrue("couldn't remove child content", element.removeChild("child", ns));
assertNull("child not removed", element.getChild("child", ns));
//now test that only the first child is removed
element.addContent(child);
element.addContent(child2);
assertTrue("couldn't remove child content", element.removeChild("child", ns));
assertNotNull("child not removed", element.getChild("child", ns));
}
/**
* Test removeChildren which removes all child elements
*/
/*
public void test_TCM__boolean_removeChildren() {
Namespace ns = Namespace.getNamespace("x", "urn:fudge");
Element element = new Element("element");
assertTrue("incorrectly returned true when deleting no content", element.removeChildren() == false);
Element child = new Element("child", ns);
Element child2 = new Element("child", ns);
element.addContent(child);
element.addContent(child2);
assertTrue("couldn't remove child content", element.removeChildren());
assertNull("child not removed", element.getContent("child", ns));
}
*/
/**
* Test removeChildren by name.
*/
/*
public void test_TCM__boolean_removeChildren_String() {
Element element = new Element("element");
assertTrue("incorrectly returned true when deleting no content", element.removeChildren() == false);
Element child = new Element("child");
Element child2 = new Element("child");
element.addContent(child);
element.addContent(child2);
assertTrue("incorrect return on bogus child", !element.removeChildren("test"));
assertNotNull("child incorrectly removed", element.getContent("child"));
assertTrue("couldn't remove child content", element.removeChildren("child"));
assertNull("children not removed", element.getContent("child"));
}
*/
/**
* Test removeChildren with a name and namespace
*/
/*
public void test_TCM__boolean_removeChildren_String_OrgJdomNamespace() {
Namespace ns = Namespace.getNamespace("x", "urn:fudge");
Element element = new Element("element");
assertTrue("incorrectly returned true when deleting no content", element.removeChildren() == false);
Element child = new Element("child", ns);
Element child2 = new Element("child", ns);
element.addContent(child);
element.addContent(child2);
assertTrue("incorrect return on bogus child", !element.removeChildren("child"));
assertNotNull("child incorrectly removed", element.getContent("child", ns));
assertTrue("couldn't remove child content", element.removeChildren("child", ns));
assertNull("children not removed", element.getContent("child", ns));
}
*/
/**
* Test removeContent for a Comment
*/
@Test
public void test_TCM__boolean_removeContent_OrgJdomComment() {
Element element = new Element("element");
Comment comm = new Comment("a comment");
element.addContent(comm);
assertTrue("couldn't remove comment content", element.removeContent(comm));
assertTrue("didn't remove comment content", element.getContent().equals(Collections.EMPTY_LIST));
}
/**
* Test removeContent for an Element.
*/
@Test
public void test_TCM__boolean_removeContent_OrgJdomElement() {
Element element = new Element("element");
Element child = new Element("child");
element.addContent(child);
assertTrue("couldn't remove element content", element.removeContent(child));
assertTrue("didn't remove element content", element.getContent().equals(Collections.EMPTY_LIST));
}
/**
* Test removeContent for entities.
*/
@Test
public void test_TCM__boolean_removeContent_OrgJdomEntity() {
Element element = new Element("element");
EntityRef ent = new EntityRef("anEntity");
element.addContent(ent);
assertTrue("couldn't remove entity content", element.removeContent(ent));
assertTrue("didn't remove entity content", element.getContent().equals(Collections.EMPTY_LIST));
}
/**
* Test removeContent for processing instructions.
*/
@Test
public void test_TCM__boolean_removeContent_OrgJdomProcessingInstruction() {
Element element = new Element("element");
ProcessingInstruction pi = new ProcessingInstruction("aPi", "something");
element.addContent(pi);
assertTrue("couldn't remove entity content", element.removeContent(pi));
assertTrue("didn't remove entity content", element.getContent().equals(Collections.EMPTY_LIST));
}
/**
* Test hashcode functions.
*/
@Test
public void test_TCM__int_hashCode() {
Element element = new Element("test");
//only an exception would be a problem
int i = -1;
try {
i = element.hashCode();
}
catch(Exception e) {
fail("bad hashCode");
}
Element element2 = new Element("test");
//different Elements, same text
int x = element2.hashCode();
assertTrue("Different Elements with same value have same hashcode", x != i);
Element element3 = new Element("test2");
//only an exception would be a problem
int y = element3.hashCode();
assertTrue("Different Elements have same hashcode", y != x);
}
/**
* Test that additionalNamespaces are returned.
*/
@Test
public void test_TCM__List_getAdditionalNamespaces() {
Element element = new Element("element");
element.addNamespaceDeclaration(Namespace.getNamespace("x", "urn:foo"));
element.addNamespaceDeclaration(Namespace.getNamespace("y", "urn:bar"));
element.addNamespaceDeclaration(Namespace.getNamespace("z", "urn:baz"));
List list = element.getAdditionalNamespaces();
Namespace ns = list.get(0);
assertTrue("didn't return added namespace", ns.getURI().equals("urn:foo"));
ns = list.get(1);
assertTrue("didn't return added namespace", ns.getURI().equals("urn:bar"));
ns = list.get(2);
assertTrue("didn't return added namespace", ns.getURI().equals("urn:baz"));
}
/**
* Test that getAttribute returns all attributes of this element.
*/
@Test
public void test_TCM__List_getAttributes() {
Element element = new Element("test");
Attribute att = new Attribute("anAttribute", "test");
Attribute att2 = new Attribute("anotherAttribute", "test");
Attribute att3 = new Attribute("anotherAttribute", "test", Namespace.getNamespace("x", "urn:JDOM"));
element.setAttribute(att);
element.setAttribute(att2);
element.setAttribute(att3);
List list = element.getAttributes();
assertEquals("incorrect size returned", list.size(), 3);
assertEquals("incorrect attribute returned", list.get(0), att);
assertEquals("incorrect attribute returned", list.get(1), att2);
}
/**
* Test getChildren to return all children from all namespaces.
*/
@Test
public void test_TCM__List_getChildren() {
Element element = new Element("el");
assertEquals("did not return Collections.EMPTY_LIST on empty element", Collections.EMPTY_LIST, element.getChildren("child"));
Element child1 = new Element("child");
child1.setAttribute(new Attribute("name", "first"));
Element child2 = new Element("child");
child2.setAttribute(new Attribute("name", "second"));
Element child3 = new Element("child", Namespace.getNamespace("ftp://wombat.stew"));
element.addContent(child1);
element.addContent(child2);
element.addContent(child3);
//should only get the child elements in NO_NAMESPACE
List list = element.getChildren();
assertEquals("incorrect number of children returned", list.size(), 3);
assertEquals("incorrect child returned", list.get(0), child1);
assertEquals("incorrect child returned", list.get(1), child2);
assertEquals("incorrect child returned", list.get(2), child3);
}
/**
* Test that Element returns a List of children by name
*/
@Test
public void test_TCM__List_getChildren_String() {
Element element = new Element("el");
assertEquals("did not return Collections.EMPTY_LIST on empty element", Collections.EMPTY_LIST, element.getChildren("child"));
Element child1 = new Element("child");
child1.setAttribute(new Attribute("name", "first"));
Element child2 = new Element("child");
child2.setAttribute(new Attribute("name", "second"));
Element child3 = new Element("child", Namespace.getNamespace("ftp://wombat.stew"));
element.addContent(child1);
element.addContent(child2);
element.addContent(child3);
//should only get the child elements in NO_NAMESPACE
List list = element.getChildren("child");
assertEquals("incorrect number of children returned", list.size(), 2);
assertEquals("incorrect child returned", list.get(0).getAttribute("name").getValue(), "first");
assertEquals("incorrect child returned", list.get(1).getAttribute("name").getValue(), "second");
}
/**
* Test that Element returns a List of children by name and namespace
*/
@Test
public void test_TCM__List_getChildren_String_OrgJdomNamespace() {
Element element = new Element("el");
assertEquals("did not return Collections.EMPTY_LIST on empty element", Collections.EMPTY_LIST, element.getChildren("child"));
Namespace ns = Namespace.getNamespace("urn:hogwarts");
Element child1 = new Element("child", ns);
child1.setAttribute(new Attribute("name", "first"));
Element child2 = new Element("child", ns);
child2.setAttribute(new Attribute("name", "second"));
Element child3 = new Element("child", Namespace.getNamespace("ftp://wombat.stew"));
element.addContent(child1);
element.addContent(child2);
element.addContent(child3);
//should only get the child elements in the hogwarts namespace
List list = element.getChildren("child", ns);
assertEquals("incorrect number of children returned", list.size(), 2);
assertEquals("incorrect child returned", list.get(0).getAttribute("name").getValue(), "first");
assertEquals("incorrect child returned", list.get(1).getAttribute("name").getValue(), "second");
}
/**
* Test that getContent returns all the content for the element
*/
@Test
public void test_TCM__List_getContent() {
Element element = new Element("el");
assertEquals("did not return Collections.EMPTY_LIST on empty element", Collections.EMPTY_LIST, element.getContent());
Namespace ns = Namespace.getNamespace("urn:hogwarts");
Element child1 = new Element("child", ns);
child1.setAttribute(new Attribute("name", "first"));
Element child2 = new Element("child", ns);
child2.setAttribute(new Attribute("name", "second"));
Element child3 = new Element("child", Namespace.getNamespace("ftp://wombat.stew"));
element.addContent(child1);
element.addContent(child2);
element.addContent(child3);
Comment comment = new Comment("hi");
element.addContent(comment);
CDATA cdata = new CDATA("gotcha");
element.addContent(cdata);
ProcessingInstruction pi = new ProcessingInstruction("tester", "do=something");
element.addContent(pi);
EntityRef entity = new EntityRef("wizards");
element.addContent(entity);
Text text = new Text("finally a new wand!");
element.addContent(text);
List list = element.getContent();
assertEquals("incorrect number of content items", 8, list.size());
assertEquals("wrong child element", child1, list.get(0));
assertEquals("wrong child element", child2, list.get(1));
assertEquals("wrong child element", child3, list.get(2));
assertEquals("wrong comment", comment, list.get(3));
assertEquals("wrong CDATA", cdata, list.get(4));
assertEquals("wrong ProcessingInstruction", pi, list.get(5));
assertEquals("wrong EntityRef", entity, list.get(6));
assertEquals("wrong text", text, list.get(7));
}
/**
* Test that clone returns a disconnected copy of the original element.
* Since clone is a deep copy, that must be tested also
*/
@Test
public void test_TCM__Object_clone() {
//first the simple case
Element element = new Element("simple");
Element clone = element.clone();
assertTrue("clone should not be the same object", element != clone);
assertEquals("clone should not have a parent", null, clone.getParent());
assertEquals("names do not match", element.getName(), clone.getName());
//now do the content tests to 2 levels deep to verify recursion
element = new Element("el");
Namespace ns = Namespace.getNamespace("urn:hogwarts");
element.setAttribute(new Attribute("name", "anElement"));
Element child1 = new Element("child", ns);
child1.setAttribute(new Attribute("name", "first"));
Element child2 = new Element("firstChild", ns);
child2.setAttribute(new Attribute("name", "second"));
Element child3 = new Element("child", Namespace.getNamespace("ftp://wombat.stew"));
child1.addContent(child2);
element.addContent(child1);
element.addContent(child3);
element.addNamespaceDeclaration(Namespace.getNamespace("foo", "http://test1"));
element.addNamespaceDeclaration(Namespace.getNamespace("bar", "http://test2"));
//add mixed content to the nested child2 element
Comment comment = new Comment("hi");
child2.addContent(comment);
CDATA cdata = new CDATA("gotcha");
child2.addContent(cdata);
ProcessingInstruction pi = new ProcessingInstruction("tester", "do=something");
child2.addContent(pi);
EntityRef entity = new EntityRef("wizards");
child2.addContent(entity);
child2.addContent("finally a new wand!");
//a little more for the element
element.addContent("top level element text");
clone = element.clone();
element = null;
child3 = null;
child2 = null;
child1 = null;
// additional namespaces
List additional = clone.getAdditionalNamespaces();
assertEquals("incorrect deep clone additional namespace",
additional.get(0),
Namespace.getNamespace("foo", "http://test1"));
assertEquals("incorrect deep clone additional namespace",
additional.get(1),
Namespace.getNamespace("bar", "http://test2"));
List list = clone.getContent();
//finally the test
assertEquals("wrong child element", ((Element) list.get(0)).getName(), "child");
assertEquals("wrong child element", ((Element) list.get(1)).getName(), "child");
Element deepClone = ((Element) list.get(0)).getChild("firstChild", Namespace.getNamespace("urn:hogwarts"));
assertEquals("wrong nested element", "firstChild", deepClone.getName());
//comment
assertTrue("deep clone comment not a clone", deepClone.getContent().get(0) != comment);
comment = null;
assertEquals("incorrect deep clone comment", "hi", ((Comment) deepClone.getContent().get(0)).getText());
//CDATA
assertTrue("deep clone CDATA not a clone", ((CDATA) deepClone.getContent().get(1)).getText().equals(cdata.getText()));
cdata = null;
assertEquals("incorrect deep clone CDATA", "gotcha", ((CDATA) deepClone.getContent().get(1)).getText());
//PI
assertTrue("deep clone PI not a clone", deepClone.getContent().get(2) != pi);
pi = null;
assertEquals("incorrect deep clone PI", "do=something", ((ProcessingInstruction) deepClone.getContent().get(2)).getData());
//entity
assertTrue("deep clone Entity not a clone", deepClone.getContent().get(3) != entity);
entity = null;
assertEquals("incorrect deep clone EntityRef", "wizards", ((EntityRef) deepClone.getContent().get(3)).getName());
//text
assertEquals("incorrect deep clone test", "finally a new wand!", ((Text) deepClone.getContent().get(4)).getText());
}
/**
* Test getAttribute by name
*/
@Test
public void test_TCM__OrgJdomAttribute_getAttribute_String() {
Element element = new Element("el");
Attribute att = new Attribute("name", "first");
element.setAttribute(att);
assertEquals("incorrect Attribute returned", element.getAttribute("name"), att);
}
/**
* Test getAttribute by name and namespace
*/
@Test
public void test_TCM__OrgJdomAttribute_getAttribute_String_OrgJdomNamespace() {
Element element = new Element("el");
Namespace ns = Namespace.getNamespace("x", "urn:foo");
Attribute att = new Attribute("name", "first", ns);
element.setAttribute(att);
element.setAttribute(new Attribute("name", "first"));
assertEquals("incorrect Attribute returned", element.getAttribute("name", ns), att);
}
/**
* Test that an element returns the reference to it's enclosing document
*/
@Test
public void test_TCM__OrgJdomDocument_getDocument() {
Element element = new Element("element");
assertNull("incorrectly returned document before there is one", element.getDocument());
Element child = new Element("child");
Element child2 = new Element("child");
element.addContent(child);
element.addContent(child2);
Document doc = new Document(element);
assertEquals("didn't return correct Document", element.getDocument(), doc);
assertEquals("didn't return correct Document", child.getDocument(), doc);
}
/**
* Test addContent for CDATA.
*/
@Test
public void test_TCM__OrgJdomElement_addContent_OrgJdomCDATA() {
//positive test is covered in test__List_getContent()
//test with null
Element element = new Element("element");
CDATA cdata = null;
try {
element.addContent(cdata);
fail("didn't catch null CDATA element");
}
catch (NullPointerException e) {
// this is what List interface expects
}
catch (Exception e) {
fail("Expect NPE, not Exception " + e.getClass().getName()
+ ": " + e.getMessage());
}
}
/**
* Test adding comment content.
*/
@Test
public void test_TCM__OrgJdomElement_addContent_OrgJdomComment() {
Element element = new Element("element");
Comment comm = new Comment("a comment");
element.addContent(comm);
assertEquals("didn't add comment content", element.getContent().get(0), comm);
try {
comm = null;
element.addContent(comm);
fail("didn't catch null Comment");
}
catch (NullPointerException e) {
// OK
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
}
/**
* Test addContent for Element.
*/
@Test
public void test_TCM__OrgJdomElement_addContent_OrgJdomElement() {
//positive test is covered in test__List_getContent()
//test with null
Element element = new Element("element");
try {
Element el = null;
element.addContent(el);
fail("didn't catch null Element");
}
catch (NullPointerException e) {
// OK
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
}
/**
* Test addContent for Entity
*/
@Test
public void test_TCM__OrgJdomElement_addContent_OrgJdomEntityRef() {
//positive test is covered in test__List_getContent()
//try with null
Element element = new Element("element");
try {
EntityRef entity = null;
element.addContent(entity);
fail("didn't catch null EntityRef");
}
catch (NullPointerException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
}
/**
* Test addContent for ProcessingInstruction.
*/
@Test
public void test_TCM__OrgJdomElement_addContent_OrgJdomProcessingInstruction() {
//positive test is covered in test__List_getContent()
//try with null data
Element element = new Element("element");
try {
ProcessingInstruction pi = null;
element.addContent(pi);
fail("didn't catch null ProcessingInstruction");
}
catch (NullPointerException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
}
/**
* Test that string based content is added correctly to an Element.
*/
@Test
public void test_TCM__OrgJdomElement_addContent_String() {
Element element = new Element("element");
element.addContent("the first part");
assertEquals("incorrect string value", "the first part", element.getText());
element.addContent("the second part");
assertEquals("incorrect string value", "the first partthe second part", element.getText());
//make sure it still works with mixed content
element.addContent(new Element("child"));
element.addContent("the third part");
assertEquals("incorrect string value", "the first partthe second partthe third part", element.getText());
// RolfL: I'v tried not to mess with the 'original' JUnit tests, but the
// following comment does not match the code, or the tests.
// vvvvvvvvvv
//test that add content null clears the content
// ^^^^^^^^^^
// Adding a null String appears to have always had the effect of adding
// a new 'empty' Text content...: new Text("")
// I am updating this test to match the current reality.
try {
// we expect addContent(null) to add a new Empty Text element.
// thus 'last' will be accurate after the add.
int last = element.getContentSize();
String data = null;
element.addContent(data);
// ensure there is a new content at 'last', and that it is an empty string.
assertEquals("", element.getContent().get(last).getValue());
}
catch (IllegalAddException e) {
fail("didn't handle null String content");
}
catch (NullPointerException e) {
fail("didn't handle null String content");
}
}
/**
* Test getContent by child name.
*/
@Test
public void test_TCM__OrgJdomElement_getChild_String() {
Element element = new Element("element");
Element child = new Element("child");
Element childNS = new Element("child", Namespace.getNamespace("urn:foo"));
element.addContent(child);
element.addContent(childNS);
assertEquals("incorrect child returned", element.getChild("child"), child);
}
/**
* Test getContent by child name and namespace.
*/
@Test
public void test_TCM__OrgJdomElement_getChild_String_OrgJdomNamespace() {
Element element = new Element("element");
Element child = new Element("child");
Namespace ns = Namespace.getNamespace("urn:foo");
Element childNS = new Element("child", ns);
element.addContent(child);
element.addContent(childNS);
assertEquals("incorrect child returned", element.getChild("child", ns), childNS);
}
/**
* Test getCopy with only the name argument. Since the copy is just a clone,
* the clone test covers most of the testing.
*/
@Test
public void test_TCM__OrgJdomElement_getCopy_String() {
/** No op because the method is deprecated
Element element = new Element("element", Namespace.getNamespace("urn:foo"));
element.addContent("text");
Element newElement = element.getCopy("new");
assertEquals("incorrect name", "new", newElement.getName());
assertEquals("incorrect namespace", Namespace.NO_NAMESPACE, newElement.getNamespace());
assertEquals("incorrect content", "text", newElement.getText());
*/
}
/**
* Test getCopy with the name and namespace arguments.
* Since the copy is just a clone, the clone test
* covers most of the testing.
*/
@Test
public void test_TCM__OrgJdomElement_getCopy_String_OrgJdomNamespace() {
/** No op because the method is deprecated
Element element = new Element("element", Namespace.getNamespace("urn:foo"));
element.addContent("text");
Namespace ns = Namespace.getNamespace("urn:test:new");
Element newElement = element.getCopy("new", ns);
assertEquals("incorrect name", "new", newElement.getName());
assertEquals("incorrect namespace", ns, newElement.getNamespace());
assertEquals("incorrect content", "text", newElement.getText());
*/
}
/**
* Test test that a child element can return it's parent.
*/
@Test
public void test_TCM__OrgJdomElement_getParent() {
Element element = new Element("el");
Element child = new Element("child");
element.addContent(child);
assertEquals("parent not found", element, child.getParent());
}
/**
* Test addAttribute with an Attribute
*/
@Test
public void test_TCM__OrgJdomElement_setAttribute_OrgJdomAttribute() {
Element element = new Element("el");
Attribute att = new Attribute("name", "first");
element.setAttribute(att);
assertEquals("incorrect Attribute returned", element.getAttribute("name"), att);
//update the value
Attribute att2 = new Attribute("name", "replacefirst");
element.setAttribute(att2);
assertEquals("incorrect Attribute value returned", "replacefirst", element.getAttribute("name").getValue());
//test with bad data
try {
element.setAttribute(null);
fail("didn't catch null attribute");
}
catch (NullPointerException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
try {
Element emt = new Element("dummy");
Attribute ma = new Attribute("hi", "there");
emt.setAttribute(ma);
element.setAttribute(ma);
fail("Should not be able to add already added Attribute.");
} catch (IllegalAddException iae) {
// good
} catch (Exception e) {
fail("Expect IllegalAddException, not " + e.getClass().getName());
}
}
/**
* Test addAttribute with a supplied name and value
*/
@Test
public void test_TCM__OrgJdomElement_setAttribute_String_String__invalidCharacters() {
final Element element = new Element("el");
//try with null name
try {
element.setAttribute(null, "value");
fail("didn't catch null attribute name");
}
catch (NullPointerException ignore) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
//try with null value
try {
element.setAttribute("name2", null);
fail("didn't catch null attribute value");
}
catch (NullPointerException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
//try with bad characters
try {
element.setAttribute("\n", "value");
fail("didn't catch bad characters in attribute name");
}
catch (IllegalArgumentException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
try {
element.setAttribute("name2", "" + (char) 0x01);
fail("didn't catch bad characters in attribute value");
}
catch (IllegalArgumentException e) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
}
/**
* Test addAttribute with a supplied name and value
*/
@Test
public void test_setAttribute_String_String__attributeTypes() {
helper_setAttribute_String_String__attributeType(Attribute.UNDECLARED_TYPE);
helper_setAttribute_String_String__attributeType(Attribute.CDATA_TYPE);
helper_setAttribute_String_String__attributeType(Attribute.ID_TYPE);
helper_setAttribute_String_String__attributeType(Attribute.IDREF_TYPE);
helper_setAttribute_String_String__attributeType(Attribute.IDREFS_TYPE);
helper_setAttribute_String_String__attributeType(Attribute.ENTITY_TYPE);
helper_setAttribute_String_String__attributeType(Attribute.ENTITIES_TYPE);
helper_setAttribute_String_String__attributeType(Attribute.NMTOKEN_TYPE);
helper_setAttribute_String_String__attributeType(Attribute.NMTOKENS_TYPE);
helper_setAttribute_String_String__attributeType(Attribute.NOTATION_TYPE);
helper_setAttribute_String_String__attributeType(Attribute.ENUMERATED_TYPE);
}
/**
* Test setAttribute with a supplied name and value
*/
private void helper_setAttribute_String_String__attributeType(final AttributeType attributeType) {
final Element element = new Element("el");
final String attributeName = "name";
final String attributeValue = "value";
final String attributeNewValue = "newValue";
final Attribute attribute = new Attribute(attributeName, attributeValue, attributeType);
// add attribute to element
element.setAttribute(attribute);
final Attribute foundAttribute = element.getAttribute(attributeName);
assertNotNull("no Attribute found", foundAttribute);
assertEquals("incorrect Attribute name returned", attributeName, foundAttribute.getName());
assertEquals("incorrect Attribute value returned", attributeValue, foundAttribute.getValue());
assertEquals("incorrect Attribute type returned", attributeType, foundAttribute.getAttributeType());
//update the value
element.setAttribute(attributeName, attributeNewValue);
final Attribute changedAttribute = element.getAttribute(attributeName);
assertNotNull("no Attribute found", changedAttribute);
assertEquals("incorrect Attribute name returned", attributeName, changedAttribute.getName());
assertEquals("incorrect Attribute value returned", attributeNewValue, changedAttribute.getValue());
assertEquals("incorrect Attribute type returned", attributeType, changedAttribute.getAttributeType());
}
/**
* Test addAttribute with a supplied name and value
*/
@Test
public void test_setAttribute_String_String_String__attributeTypes() {
helper_setAttribute_String_String_String__attributeType(Attribute.UNDECLARED_TYPE);
helper_setAttribute_String_String_String__attributeType(Attribute.CDATA_TYPE);
helper_setAttribute_String_String_String__attributeType(Attribute.ID_TYPE);
helper_setAttribute_String_String_String__attributeType(Attribute.IDREF_TYPE);
helper_setAttribute_String_String_String__attributeType(Attribute.IDREFS_TYPE);
helper_setAttribute_String_String_String__attributeType(Attribute.ENTITY_TYPE);
helper_setAttribute_String_String_String__attributeType(Attribute.ENTITIES_TYPE);
helper_setAttribute_String_String_String__attributeType(Attribute.NMTOKEN_TYPE);
helper_setAttribute_String_String_String__attributeType(Attribute.NMTOKENS_TYPE);
helper_setAttribute_String_String_String__attributeType(Attribute.NOTATION_TYPE);
helper_setAttribute_String_String_String__attributeType(Attribute.ENUMERATED_TYPE);
}
/**
* Test setAttribute with a supplied name and value
*
* @author Victor Toni
*/
private void helper_setAttribute_String_String_String__attributeType(final AttributeType attributeType) {
try {
final Namespace defaultNamespace = Namespace.getNamespace(null, "http://test.org/default");
helper_setAttribute_String_String_String__attributeType(defaultNamespace, attributeType);
fail("didn't catch empty prefix for attribute ");
}
catch( final IllegalNameException ignore) {
// Do nothing
} catch (Exception e) {
fail("Unexpected exception " + e.getClass());
}
final Namespace testNamespace = Namespace.getNamespace("test", "http://test.org/test");
helper_setAttribute_String_String_String__attributeType(testNamespace, attributeType);
}
/**
* Test setAttribute with a supplied name, namespace and value
*
* @author Victor Toni
*/
private void helper_setAttribute_String_String_String__attributeType(final Namespace namespace, final AttributeType attributeType) {
final Element element = new Element("el");
final String attributeName = "name";
final String attributeValue = "value";
final String attributeNewValue = "newValue";
final Attribute attribute = new Attribute(attributeName, attributeValue, attributeType, namespace);
// add attribute to element
element.setAttribute(attribute);
final Attribute foundAttribute = element.getAttribute(attributeName, namespace);
assertNotNull("no Attribute found", foundAttribute);
assertEquals("incorrect Attribute name returned", attributeName, foundAttribute.getName());
assertEquals("incorrect Attribute value returned", attributeValue, foundAttribute.getValue());
assertEquals("incorrect Attribute type returned", attributeType, foundAttribute.getAttributeType());
//update the value
element.setAttribute(attributeName, attributeNewValue, namespace);
final Attribute changedAttribute = element.getAttribute(attributeName, namespace);
assertNotNull("no Attribute found", changedAttribute);
assertEquals("incorrect Attribute name returned", attributeName, changedAttribute.getName());
assertEquals("incorrect Attribute value returned", attributeNewValue, changedAttribute.getValue());
assertEquals("incorrect Attribute type returned", attributeType, changedAttribute.getAttributeType());
}
/**
* Test that attributes are added correctly as a list
*/
@Test
public void test_TCM__OrgJdomElement_setAttributes_List() {
Element element = new Element("element");
Attribute one = new Attribute("one", "value");
Attribute two = new Attribute("two", "value");
Attribute three = new Attribute("three", "value");
ArrayList list = new ArrayList();
list.add(one);
list.add(two);
list.add(three);
//put in an attribute that will get blown away later
element.setAttribute(new Attribute("type", "test"));
element.setAttributes(list);
assertEquals("attribute not found", one, element.getAttribute("one"));
assertEquals("attribute not found", two, element.getAttribute("two"));
assertEquals("attribute not found", three, element.getAttribute("three"));
assertNull("attribute should not have been found", element.getAttribute("type"));
//now try to add something that isn't an attribute which should still add those
//attributes added before the mistake.
// Element bogus = new Element("bogus");
// Attribute four = new Attribute("four", "value");
//
// ArrayList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy