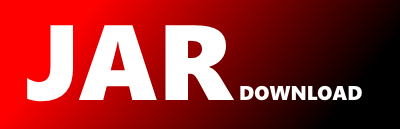
commonMain.org.jellyfin.sdk.api.operations.PlayStateApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jellyfin-api Show documentation
Show all versions of jellyfin-api Show documentation
Official Kotlin/Java SDK for Jellyfin. org.jellyfin.sdk:jellyfin-api
// !! WARNING
// !! DO NOT EDIT THIS FILE
//
// This file is generated by the openapi-generator module and is not meant for manual changes.
// Please read the README.md file in the openapi-generator module for additional information.
package org.jellyfin.sdk.api.operations
import kotlin.Any
import kotlin.Boolean
import kotlin.Int
import kotlin.Long
import kotlin.String
import kotlin.Unit
import kotlin.collections.emptyMap
import kotlin.collections.mutableMapOf
import org.jellyfin.sdk.api.client.ApiClient
import org.jellyfin.sdk.api.client.Response
import org.jellyfin.sdk.api.client.exception.MissingUserIdException
import org.jellyfin.sdk.api.client.extensions.delete
import org.jellyfin.sdk.api.client.extensions.post
import org.jellyfin.sdk.model.DateTime
import org.jellyfin.sdk.model.UUID
import org.jellyfin.sdk.model.api.PlayMethod
import org.jellyfin.sdk.model.api.PlaybackProgressInfo
import org.jellyfin.sdk.model.api.PlaybackStartInfo
import org.jellyfin.sdk.model.api.PlaybackStopInfo
import org.jellyfin.sdk.model.api.RepeatMode
import org.jellyfin.sdk.model.api.UserItemDataDto
public class PlayStateApi(
private val api: ApiClient,
) : Api {
/**
* Marks an item as played for user.
*
* @param userId User id.
* @param itemId Item id.
* @param datePlayed Optional. The date the item was played.
*/
public suspend fun markPlayedItem(
userId: UUID = api.userId ?: throw MissingUserIdException(),
itemId: UUID,
datePlayed: DateTime? = null,
): Response {
val pathParameters = mutableMapOf()
pathParameters["userId"] = userId
pathParameters["itemId"] = itemId
val queryParameters = mutableMapOf()
queryParameters["datePlayed"] = datePlayed
val data = null
val response = api.post("/Users/{userId}/PlayedItems/{itemId}", pathParameters,
queryParameters, data)
return response
}
/**
* Marks an item as unplayed for user.
*
* @param userId User id.
* @param itemId Item id.
*/
public suspend fun markUnplayedItem(userId: UUID = api.userId ?: throw MissingUserIdException(),
itemId: UUID): Response {
val pathParameters = mutableMapOf()
pathParameters["userId"] = userId
pathParameters["itemId"] = itemId
val queryParameters = emptyMap()
val data = null
val response = api.delete("/Users/{userId}/PlayedItems/{itemId}", pathParameters,
queryParameters, data)
return response
}
/**
* Reports a user's playback progress.
*
* @param userId User id.
* @param itemId Item id.
* @param mediaSourceId The id of the MediaSource.
* @param positionTicks Optional. The current position, in ticks. 1 tick = 10000 ms.
* @param audioStreamIndex The audio stream index.
* @param subtitleStreamIndex The subtitle stream index.
* @param volumeLevel Scale of 0-100.
* @param playMethod The play method.
* @param liveStreamId The live stream id.
* @param playSessionId The play session id.
* @param repeatMode The repeat mode.
* @param isPaused Indicates if the player is paused.
* @param isMuted Indicates if the player is muted.
*/
public suspend fun onPlaybackProgress(
userId: UUID = api.userId ?: throw MissingUserIdException(),
itemId: UUID,
mediaSourceId: String? = null,
positionTicks: Long? = null,
audioStreamIndex: Int? = null,
subtitleStreamIndex: Int? = null,
volumeLevel: Int? = null,
playMethod: PlayMethod? = null,
liveStreamId: String? = null,
playSessionId: String? = null,
repeatMode: RepeatMode? = null,
isPaused: Boolean? = false,
isMuted: Boolean? = false,
): Response {
val pathParameters = mutableMapOf()
pathParameters["userId"] = userId
pathParameters["itemId"] = itemId
val queryParameters = mutableMapOf()
queryParameters["mediaSourceId"] = mediaSourceId
queryParameters["positionTicks"] = positionTicks
queryParameters["audioStreamIndex"] = audioStreamIndex
queryParameters["subtitleStreamIndex"] = subtitleStreamIndex
queryParameters["volumeLevel"] = volumeLevel
queryParameters["playMethod"] = playMethod
queryParameters["liveStreamId"] = liveStreamId
queryParameters["playSessionId"] = playSessionId
queryParameters["repeatMode"] = repeatMode
queryParameters["isPaused"] = isPaused
queryParameters["isMuted"] = isMuted
val data = null
val response = api.post("/Users/{userId}/PlayingItems/{itemId}/Progress", pathParameters,
queryParameters, data)
return response
}
/**
* Reports that a user has begun playing an item.
*
* @param userId User id.
* @param itemId Item id.
* @param mediaSourceId The id of the MediaSource.
* @param audioStreamIndex The audio stream index.
* @param subtitleStreamIndex The subtitle stream index.
* @param playMethod The play method.
* @param liveStreamId The live stream id.
* @param playSessionId The play session id.
* @param canSeek Indicates if the client can seek.
*/
public suspend fun onPlaybackStart(
userId: UUID = api.userId ?: throw MissingUserIdException(),
itemId: UUID,
mediaSourceId: String? = null,
audioStreamIndex: Int? = null,
subtitleStreamIndex: Int? = null,
playMethod: PlayMethod? = null,
liveStreamId: String? = null,
playSessionId: String? = null,
canSeek: Boolean? = false,
): Response {
val pathParameters = mutableMapOf()
pathParameters["userId"] = userId
pathParameters["itemId"] = itemId
val queryParameters = mutableMapOf()
queryParameters["mediaSourceId"] = mediaSourceId
queryParameters["audioStreamIndex"] = audioStreamIndex
queryParameters["subtitleStreamIndex"] = subtitleStreamIndex
queryParameters["playMethod"] = playMethod
queryParameters["liveStreamId"] = liveStreamId
queryParameters["playSessionId"] = playSessionId
queryParameters["canSeek"] = canSeek
val data = null
val response = api.post("/Users/{userId}/PlayingItems/{itemId}", pathParameters,
queryParameters, data)
return response
}
/**
* Reports that a user has stopped playing an item.
*
* @param userId User id.
* @param itemId Item id.
* @param mediaSourceId The id of the MediaSource.
* @param nextMediaType The next media type that will play.
* @param positionTicks Optional. The position, in ticks, where playback stopped. 1 tick = 10000
* ms.
* @param liveStreamId The live stream id.
* @param playSessionId The play session id.
*/
public suspend fun onPlaybackStopped(
userId: UUID = api.userId ?: throw MissingUserIdException(),
itemId: UUID,
mediaSourceId: String? = null,
nextMediaType: String? = null,
positionTicks: Long? = null,
liveStreamId: String? = null,
playSessionId: String? = null,
): Response {
val pathParameters = mutableMapOf()
pathParameters["userId"] = userId
pathParameters["itemId"] = itemId
val queryParameters = mutableMapOf()
queryParameters["mediaSourceId"] = mediaSourceId
queryParameters["nextMediaType"] = nextMediaType
queryParameters["positionTicks"] = positionTicks
queryParameters["liveStreamId"] = liveStreamId
queryParameters["playSessionId"] = playSessionId
val data = null
val response = api.delete("/Users/{userId}/PlayingItems/{itemId}", pathParameters,
queryParameters, data)
return response
}
/**
* Pings a playback session.
*
* @param playSessionId Playback session id.
*/
public suspend fun pingPlaybackSession(playSessionId: String): Response {
val pathParameters = emptyMap()
val queryParameters = mutableMapOf()
queryParameters["playSessionId"] = playSessionId
val data = null
val response = api.post("/Sessions/Playing/Ping", pathParameters, queryParameters, data)
return response
}
/**
* Reports playback progress within a session.
*/
public suspend fun reportPlaybackProgress(`data`: PlaybackProgressInfo? = null): Response {
val pathParameters = emptyMap()
val queryParameters = emptyMap()
val response = api.post("/Sessions/Playing/Progress", pathParameters, queryParameters, data)
return response
}
/**
* Reports playback has started within a session.
*/
public suspend fun reportPlaybackStart(`data`: PlaybackStartInfo? = null): Response {
val pathParameters = emptyMap()
val queryParameters = emptyMap()
val response = api.post("/Sessions/Playing", pathParameters, queryParameters, data)
return response
}
/**
* Reports playback has stopped within a session.
*/
public suspend fun reportPlaybackStopped(`data`: PlaybackStopInfo? = null): Response {
val pathParameters = emptyMap()
val queryParameters = emptyMap()
val response = api.post("/Sessions/Playing/Stopped", pathParameters, queryParameters, data)
return response
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy