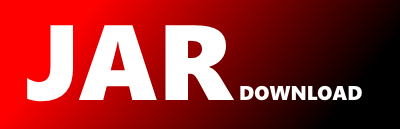
commonMain.org.jellyfin.sdk.api.operations.PluginsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jellyfin-api Show documentation
Show all versions of jellyfin-api Show documentation
Official Kotlin/Java SDK for Jellyfin. org.jellyfin.sdk:jellyfin-api
// !! WARNING
// !! DO NOT EDIT THIS FILE
//
// This file is generated by the openapi-generator module and is not meant for manual changes.
// Please read the README.md file in the openapi-generator module for additional information.
package org.jellyfin.sdk.api.operations
import io.ktor.utils.io.ByteReadChannel
import kotlin.Any
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.emptyMap
import kotlin.collections.mutableMapOf
import org.jellyfin.sdk.api.client.ApiClient
import org.jellyfin.sdk.api.client.Response
import org.jellyfin.sdk.api.client.extensions.`get`
import org.jellyfin.sdk.api.client.extensions.delete
import org.jellyfin.sdk.api.client.extensions.post
import org.jellyfin.sdk.model.UUID
import org.jellyfin.sdk.model.api.BasePluginConfiguration
import org.jellyfin.sdk.model.api.PluginInfo
public class PluginsApi(
private val api: ApiClient,
) : Api {
/**
* Disable a plugin.
*
* @param pluginId Plugin id.
* @param version Plugin version.
*/
public suspend fun disablePlugin(pluginId: UUID, version: String): Response {
val pathParameters = mutableMapOf()
pathParameters["pluginId"] = pluginId
pathParameters["version"] = version
val queryParameters = emptyMap()
val data = null
val response = api.post("/Plugins/{pluginId}/{version}/Disable", pathParameters,
queryParameters, data)
return response
}
/**
* Enables a disabled plugin.
*
* @param pluginId Plugin id.
* @param version Plugin version.
*/
public suspend fun enablePlugin(pluginId: UUID, version: String): Response {
val pathParameters = mutableMapOf()
pathParameters["pluginId"] = pluginId
pathParameters["version"] = version
val queryParameters = emptyMap()
val data = null
val response = api.post("/Plugins/{pluginId}/{version}/Enable", pathParameters,
queryParameters, data)
return response
}
/**
* Gets plugin configuration.
*
* @param pluginId Plugin id.
*/
public suspend fun getPluginConfiguration(pluginId: UUID): Response {
val pathParameters = mutableMapOf()
pathParameters["pluginId"] = pluginId
val queryParameters = emptyMap()
val data = null
val response = api.`get`("/Plugins/{pluginId}/Configuration",
pathParameters, queryParameters, data)
return response
}
/**
* Gets a plugin's image.
*
* @param pluginId Plugin id.
* @param version Plugin version.
*/
public suspend fun getPluginImage(pluginId: UUID, version: String): Response {
val pathParameters = mutableMapOf()
pathParameters["pluginId"] = pluginId
pathParameters["version"] = version
val queryParameters = emptyMap()
val data = null
val response = api.`get`("/Plugins/{pluginId}/{version}/Image", pathParameters,
queryParameters, data)
return response
}
/**
* Gets a plugin's image.
*
* @param pluginId Plugin id.
* @param version Plugin version.
* @param includeCredentials Add the access token to the url to make an authenticated request.
*/
public fun getPluginImageUrl(
pluginId: UUID,
version: String,
includeCredentials: Boolean = true,
): String {
val pathParameters = mutableMapOf()
pathParameters["pluginId"] = pluginId
pathParameters["version"] = version
val queryParameters = emptyMap()
return api.createUrl("/Plugins/{pluginId}/{version}/Image", pathParameters, queryParameters,
includeCredentials)
}
/**
* Gets a plugin's manifest.
*
* @param pluginId Plugin id.
*/
public suspend fun getPluginManifest(pluginId: UUID): Response {
val pathParameters = mutableMapOf()
pathParameters["pluginId"] = pluginId
val queryParameters = emptyMap()
val data = null
val response = api.post("/Plugins/{pluginId}/Manifest", pathParameters, queryParameters,
data)
return response
}
/**
* Gets a list of currently installed plugins.
*/
public suspend fun getPlugins(): Response> {
val pathParameters = emptyMap()
val queryParameters = emptyMap()
val data = null
val response = api.`get`>("/Plugins", pathParameters, queryParameters, data)
return response
}
/**
* Uninstalls a plugin.
*
* @param pluginId Plugin id.
*/
@Deprecated("This member is deprecated and may be removed in the future")
public suspend fun uninstallPlugin(pluginId: UUID): Response {
val pathParameters = mutableMapOf()
pathParameters["pluginId"] = pluginId
val queryParameters = emptyMap()
val data = null
val response = api.delete("/Plugins/{pluginId}", pathParameters, queryParameters, data)
return response
}
/**
* Uninstalls a plugin by version.
*
* @param pluginId Plugin id.
* @param version Plugin version.
*/
public suspend fun uninstallPluginByVersion(pluginId: UUID, version: String): Response {
val pathParameters = mutableMapOf()
pathParameters["pluginId"] = pluginId
pathParameters["version"] = version
val queryParameters = emptyMap()
val data = null
val response = api.delete("/Plugins/{pluginId}/{version}", pathParameters, queryParameters,
data)
return response
}
/**
* Accepts plugin configuration as JSON body.
*
* @param pluginId Plugin id.
*/
public suspend fun updatePluginConfiguration(pluginId: UUID): Response {
val pathParameters = mutableMapOf()
pathParameters["pluginId"] = pluginId
val queryParameters = emptyMap()
val data = null
val response = api.post("/Plugins/{pluginId}/Configuration", pathParameters,
queryParameters, data)
return response
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy