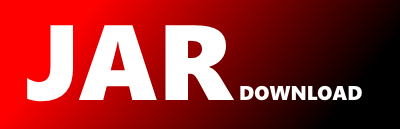
org.jetbrains.bsp.bazel.info.BspTargetInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bazel-bsp Show documentation
Show all versions of bazel-bsp Show documentation
An implementation of the Build Server Protocol for Bazel. Allows clients such as IntelliJ to obtain build
specific
information in order to improve the experience of users in the IDE.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: server/server/src/main/kotlin/org/jetbrains/bsp/bazel/server/sync/proto/bsp_target_info.proto
// Protobuf Java Version: 4.28.3
package org.jetbrains.bsp.bazel.info;
public final class BspTargetInfo {
private BspTargetInfo() {}
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
BspTargetInfo.class.getName());
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code bazelbsp.RustCrateLocation}
*/
public enum RustCrateLocation
implements com.google.protobuf.ProtocolMessageEnum {
/**
* WORKSPACE_DIR = 0;
*/
WORKSPACE_DIR(0),
/**
* EXEC_ROOT = 1;
*/
EXEC_ROOT(1),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
RustCrateLocation.class.getName());
}
/**
* WORKSPACE_DIR = 0;
*/
public static final int WORKSPACE_DIR_VALUE = 0;
/**
* EXEC_ROOT = 1;
*/
public static final int EXEC_ROOT_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static RustCrateLocation valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static RustCrateLocation forNumber(int value) {
switch (value) {
case 0: return WORKSPACE_DIR;
case 1: return EXEC_ROOT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
RustCrateLocation> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public RustCrateLocation findValueByNumber(int number) {
return RustCrateLocation.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.getDescriptor().getEnumTypes().get(0);
}
private static final RustCrateLocation[] VALUES = values();
public static RustCrateLocation valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private RustCrateLocation(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bazelbsp.RustCrateLocation)
}
public interface FileLocationOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.FileLocation)
com.google.protobuf.MessageOrBuilder {
/**
* string relative_path = 1;
* @return The relativePath.
*/
java.lang.String getRelativePath();
/**
* string relative_path = 1;
* @return The bytes for relativePath.
*/
com.google.protobuf.ByteString
getRelativePathBytes();
/**
* bool is_source = 2;
* @return The isSource.
*/
boolean getIsSource();
/**
* bool is_external = 3;
* @return The isExternal.
*/
boolean getIsExternal();
/**
* string root_execution_path_fragment = 4;
* @return The rootExecutionPathFragment.
*/
java.lang.String getRootExecutionPathFragment();
/**
* string root_execution_path_fragment = 4;
* @return The bytes for rootExecutionPathFragment.
*/
com.google.protobuf.ByteString
getRootExecutionPathFragmentBytes();
}
/**
* Protobuf type {@code bazelbsp.FileLocation}
*/
public static final class FileLocation extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.FileLocation)
FileLocationOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
FileLocation.class.getName());
}
// Use FileLocation.newBuilder() to construct.
private FileLocation(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private FileLocation() {
relativePath_ = "";
rootExecutionPathFragment_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_FileLocation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_FileLocation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder.class);
}
public static final int RELATIVE_PATH_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object relativePath_ = "";
/**
* string relative_path = 1;
* @return The relativePath.
*/
@java.lang.Override
public java.lang.String getRelativePath() {
java.lang.Object ref = relativePath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
relativePath_ = s;
return s;
}
}
/**
* string relative_path = 1;
* @return The bytes for relativePath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRelativePathBytes() {
java.lang.Object ref = relativePath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
relativePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IS_SOURCE_FIELD_NUMBER = 2;
private boolean isSource_ = false;
/**
* bool is_source = 2;
* @return The isSource.
*/
@java.lang.Override
public boolean getIsSource() {
return isSource_;
}
public static final int IS_EXTERNAL_FIELD_NUMBER = 3;
private boolean isExternal_ = false;
/**
* bool is_external = 3;
* @return The isExternal.
*/
@java.lang.Override
public boolean getIsExternal() {
return isExternal_;
}
public static final int ROOT_EXECUTION_PATH_FRAGMENT_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object rootExecutionPathFragment_ = "";
/**
* string root_execution_path_fragment = 4;
* @return The rootExecutionPathFragment.
*/
@java.lang.Override
public java.lang.String getRootExecutionPathFragment() {
java.lang.Object ref = rootExecutionPathFragment_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
rootExecutionPathFragment_ = s;
return s;
}
}
/**
* string root_execution_path_fragment = 4;
* @return The bytes for rootExecutionPathFragment.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRootExecutionPathFragmentBytes() {
java.lang.Object ref = rootExecutionPathFragment_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
rootExecutionPathFragment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(relativePath_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, relativePath_);
}
if (isSource_ != false) {
output.writeBool(2, isSource_);
}
if (isExternal_ != false) {
output.writeBool(3, isExternal_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(rootExecutionPathFragment_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 4, rootExecutionPathFragment_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(relativePath_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, relativePath_);
}
if (isSource_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, isSource_);
}
if (isExternal_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, isExternal_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(rootExecutionPathFragment_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(4, rootExecutionPathFragment_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation) obj;
if (!getRelativePath()
.equals(other.getRelativePath())) return false;
if (getIsSource()
!= other.getIsSource()) return false;
if (getIsExternal()
!= other.getIsExternal()) return false;
if (!getRootExecutionPathFragment()
.equals(other.getRootExecutionPathFragment())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RELATIVE_PATH_FIELD_NUMBER;
hash = (53 * hash) + getRelativePath().hashCode();
hash = (37 * hash) + IS_SOURCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsSource());
hash = (37 * hash) + IS_EXTERNAL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsExternal());
hash = (37 * hash) + ROOT_EXECUTION_PATH_FRAGMENT_FIELD_NUMBER;
hash = (53 * hash) + getRootExecutionPathFragment().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.FileLocation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.FileLocation)
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_FileLocation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_FileLocation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
relativePath_ = "";
isSource_ = false;
isExternal_ = false;
rootExecutionPathFragment_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_FileLocation_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.relativePath_ = relativePath_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.isSource_ = isSource_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.isExternal_ = isExternal_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.rootExecutionPathFragment_ = rootExecutionPathFragment_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) return this;
if (!other.getRelativePath().isEmpty()) {
relativePath_ = other.relativePath_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.getIsSource() != false) {
setIsSource(other.getIsSource());
}
if (other.getIsExternal() != false) {
setIsExternal(other.getIsExternal());
}
if (!other.getRootExecutionPathFragment().isEmpty()) {
rootExecutionPathFragment_ = other.rootExecutionPathFragment_;
bitField0_ |= 0x00000008;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
relativePath_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
isSource_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
isExternal_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34: {
rootExecutionPathFragment_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object relativePath_ = "";
/**
* string relative_path = 1;
* @return The relativePath.
*/
public java.lang.String getRelativePath() {
java.lang.Object ref = relativePath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
relativePath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string relative_path = 1;
* @return The bytes for relativePath.
*/
public com.google.protobuf.ByteString
getRelativePathBytes() {
java.lang.Object ref = relativePath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
relativePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string relative_path = 1;
* @param value The relativePath to set.
* @return This builder for chaining.
*/
public Builder setRelativePath(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
relativePath_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string relative_path = 1;
* @return This builder for chaining.
*/
public Builder clearRelativePath() {
relativePath_ = getDefaultInstance().getRelativePath();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string relative_path = 1;
* @param value The bytes for relativePath to set.
* @return This builder for chaining.
*/
public Builder setRelativePathBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
relativePath_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private boolean isSource_ ;
/**
* bool is_source = 2;
* @return The isSource.
*/
@java.lang.Override
public boolean getIsSource() {
return isSource_;
}
/**
* bool is_source = 2;
* @param value The isSource to set.
* @return This builder for chaining.
*/
public Builder setIsSource(boolean value) {
isSource_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* bool is_source = 2;
* @return This builder for chaining.
*/
public Builder clearIsSource() {
bitField0_ = (bitField0_ & ~0x00000002);
isSource_ = false;
onChanged();
return this;
}
private boolean isExternal_ ;
/**
* bool is_external = 3;
* @return The isExternal.
*/
@java.lang.Override
public boolean getIsExternal() {
return isExternal_;
}
/**
* bool is_external = 3;
* @param value The isExternal to set.
* @return This builder for chaining.
*/
public Builder setIsExternal(boolean value) {
isExternal_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* bool is_external = 3;
* @return This builder for chaining.
*/
public Builder clearIsExternal() {
bitField0_ = (bitField0_ & ~0x00000004);
isExternal_ = false;
onChanged();
return this;
}
private java.lang.Object rootExecutionPathFragment_ = "";
/**
* string root_execution_path_fragment = 4;
* @return The rootExecutionPathFragment.
*/
public java.lang.String getRootExecutionPathFragment() {
java.lang.Object ref = rootExecutionPathFragment_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
rootExecutionPathFragment_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string root_execution_path_fragment = 4;
* @return The bytes for rootExecutionPathFragment.
*/
public com.google.protobuf.ByteString
getRootExecutionPathFragmentBytes() {
java.lang.Object ref = rootExecutionPathFragment_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
rootExecutionPathFragment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string root_execution_path_fragment = 4;
* @param value The rootExecutionPathFragment to set.
* @return This builder for chaining.
*/
public Builder setRootExecutionPathFragment(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
rootExecutionPathFragment_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* string root_execution_path_fragment = 4;
* @return This builder for chaining.
*/
public Builder clearRootExecutionPathFragment() {
rootExecutionPathFragment_ = getDefaultInstance().getRootExecutionPathFragment();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* string root_execution_path_fragment = 4;
* @param value The bytes for rootExecutionPathFragment to set.
* @return This builder for chaining.
*/
public Builder setRootExecutionPathFragmentBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
rootExecutionPathFragment_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.FileLocation)
}
// @@protoc_insertion_point(class_scope:bazelbsp.FileLocation)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FileLocation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DependencyOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.Dependency)
com.google.protobuf.MessageOrBuilder {
/**
* string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
* string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* .bazelbsp.Dependency.DependencyType dependency_type = 2;
* @return The enum numeric value on the wire for dependencyType.
*/
int getDependencyTypeValue();
/**
* .bazelbsp.Dependency.DependencyType dependency_type = 2;
* @return The dependencyType.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType getDependencyType();
}
/**
* Protobuf type {@code bazelbsp.Dependency}
*/
public static final class Dependency extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.Dependency)
DependencyOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
Dependency.class.getName());
}
// Use Dependency.newBuilder() to construct.
private Dependency(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Dependency() {
id_ = "";
dependencyType_ = 0;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_Dependency_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_Dependency_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.Builder.class);
}
/**
* Protobuf enum {@code bazelbsp.Dependency.DependencyType}
*/
public enum DependencyType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* COMPILE = 0;
*/
COMPILE(0),
/**
* RUNTIME = 1;
*/
RUNTIME(1),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
DependencyType.class.getName());
}
/**
* COMPILE = 0;
*/
public static final int COMPILE_VALUE = 0;
/**
* RUNTIME = 1;
*/
public static final int RUNTIME_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DependencyType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static DependencyType forNumber(int value) {
switch (value) {
case 0: return COMPILE;
case 1: return RUNTIME;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
DependencyType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DependencyType findValueByNumber(int number) {
return DependencyType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.getDescriptor().getEnumTypes().get(0);
}
private static final DependencyType[] VALUES = values();
public static DependencyType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private DependencyType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:bazelbsp.Dependency.DependencyType)
}
public static final int ID_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object id_ = "";
/**
* string id = 1;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEPENDENCY_TYPE_FIELD_NUMBER = 2;
private int dependencyType_ = 0;
/**
* .bazelbsp.Dependency.DependencyType dependency_type = 2;
* @return The enum numeric value on the wire for dependencyType.
*/
@java.lang.Override public int getDependencyTypeValue() {
return dependencyType_;
}
/**
* .bazelbsp.Dependency.DependencyType dependency_type = 2;
* @return The dependencyType.
*/
@java.lang.Override public org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType getDependencyType() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType result = org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType.forNumber(dependencyType_);
return result == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(id_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, id_);
}
if (dependencyType_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType.COMPILE.getNumber()) {
output.writeEnum(2, dependencyType_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(id_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, id_);
}
if (dependencyType_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType.COMPILE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, dependencyType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency) obj;
if (!getId()
.equals(other.getId())) return false;
if (dependencyType_ != other.dependencyType_) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + DEPENDENCY_TYPE_FIELD_NUMBER;
hash = (53 * hash) + dependencyType_;
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.Dependency}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.Dependency)
org.jetbrains.bsp.bazel.info.BspTargetInfo.DependencyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_Dependency_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_Dependency_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
id_ = "";
dependencyType_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_Dependency_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.dependencyType_ = dependencyType_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.dependencyType_ != 0) {
setDependencyTypeValue(other.getDependencyTypeValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
id_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
dependencyType_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
* string id = 1;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int dependencyType_ = 0;
/**
* .bazelbsp.Dependency.DependencyType dependency_type = 2;
* @return The enum numeric value on the wire for dependencyType.
*/
@java.lang.Override public int getDependencyTypeValue() {
return dependencyType_;
}
/**
* .bazelbsp.Dependency.DependencyType dependency_type = 2;
* @param value The enum numeric value on the wire for dependencyType to set.
* @return This builder for chaining.
*/
public Builder setDependencyTypeValue(int value) {
dependencyType_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .bazelbsp.Dependency.DependencyType dependency_type = 2;
* @return The dependencyType.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType getDependencyType() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType result = org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType.forNumber(dependencyType_);
return result == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType.UNRECOGNIZED : result;
}
/**
* .bazelbsp.Dependency.DependencyType dependency_type = 2;
* @param value The dependencyType to set.
* @return This builder for chaining.
*/
public Builder setDependencyType(org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency.DependencyType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
dependencyType_ = value.getNumber();
onChanged();
return this;
}
/**
* .bazelbsp.Dependency.DependencyType dependency_type = 2;
* @return This builder for chaining.
*/
public Builder clearDependencyType() {
bitField0_ = (bitField0_ & ~0x00000002);
dependencyType_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.Dependency)
}
// @@protoc_insertion_point(class_scope:bazelbsp.Dependency)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Dependency parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JvmOutputsOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.JvmOutputs)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
java.util.List
getBinaryJarsList();
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getBinaryJars(int index);
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
int getBinaryJarsCount();
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getBinaryJarsOrBuilderList();
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getBinaryJarsOrBuilder(
int index);
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
java.util.List
getInterfaceJarsList();
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getInterfaceJars(int index);
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
int getInterfaceJarsCount();
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getInterfaceJarsOrBuilderList();
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getInterfaceJarsOrBuilder(
int index);
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
java.util.List
getSourceJarsList();
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getSourceJars(int index);
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
int getSourceJarsCount();
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getSourceJarsOrBuilderList();
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getSourceJarsOrBuilder(
int index);
}
/**
* Protobuf type {@code bazelbsp.JvmOutputs}
*/
public static final class JvmOutputs extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.JvmOutputs)
JvmOutputsOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
JvmOutputs.class.getName());
}
// Use JvmOutputs.newBuilder() to construct.
private JvmOutputs(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private JvmOutputs() {
binaryJars_ = java.util.Collections.emptyList();
interfaceJars_ = java.util.Collections.emptyList();
sourceJars_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JvmOutputs_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JvmOutputs_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder.class);
}
public static final int BINARY_JARS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List binaryJars_;
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
@java.lang.Override
public java.util.List getBinaryJarsList() {
return binaryJars_;
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getBinaryJarsOrBuilderList() {
return binaryJars_;
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
@java.lang.Override
public int getBinaryJarsCount() {
return binaryJars_.size();
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getBinaryJars(int index) {
return binaryJars_.get(index);
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getBinaryJarsOrBuilder(
int index) {
return binaryJars_.get(index);
}
public static final int INTERFACE_JARS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List interfaceJars_;
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
@java.lang.Override
public java.util.List getInterfaceJarsList() {
return interfaceJars_;
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getInterfaceJarsOrBuilderList() {
return interfaceJars_;
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
@java.lang.Override
public int getInterfaceJarsCount() {
return interfaceJars_.size();
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getInterfaceJars(int index) {
return interfaceJars_.get(index);
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getInterfaceJarsOrBuilder(
int index) {
return interfaceJars_.get(index);
}
public static final int SOURCE_JARS_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List sourceJars_;
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
@java.lang.Override
public java.util.List getSourceJarsList() {
return sourceJars_;
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getSourceJarsOrBuilderList() {
return sourceJars_;
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
@java.lang.Override
public int getSourceJarsCount() {
return sourceJars_.size();
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getSourceJars(int index) {
return sourceJars_.get(index);
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getSourceJarsOrBuilder(
int index) {
return sourceJars_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < binaryJars_.size(); i++) {
output.writeMessage(1, binaryJars_.get(i));
}
for (int i = 0; i < interfaceJars_.size(); i++) {
output.writeMessage(2, interfaceJars_.get(i));
}
for (int i = 0; i < sourceJars_.size(); i++) {
output.writeMessage(3, sourceJars_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < binaryJars_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, binaryJars_.get(i));
}
for (int i = 0; i < interfaceJars_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, interfaceJars_.get(i));
}
for (int i = 0; i < sourceJars_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, sourceJars_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs) obj;
if (!getBinaryJarsList()
.equals(other.getBinaryJarsList())) return false;
if (!getInterfaceJarsList()
.equals(other.getInterfaceJarsList())) return false;
if (!getSourceJarsList()
.equals(other.getSourceJarsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getBinaryJarsCount() > 0) {
hash = (37 * hash) + BINARY_JARS_FIELD_NUMBER;
hash = (53 * hash) + getBinaryJarsList().hashCode();
}
if (getInterfaceJarsCount() > 0) {
hash = (37 * hash) + INTERFACE_JARS_FIELD_NUMBER;
hash = (53 * hash) + getInterfaceJarsList().hashCode();
}
if (getSourceJarsCount() > 0) {
hash = (37 * hash) + SOURCE_JARS_FIELD_NUMBER;
hash = (53 * hash) + getSourceJarsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.JvmOutputs}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.JvmOutputs)
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JvmOutputs_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JvmOutputs_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (binaryJarsBuilder_ == null) {
binaryJars_ = java.util.Collections.emptyList();
} else {
binaryJars_ = null;
binaryJarsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (interfaceJarsBuilder_ == null) {
interfaceJars_ = java.util.Collections.emptyList();
} else {
interfaceJars_ = null;
interfaceJarsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (sourceJarsBuilder_ == null) {
sourceJars_ = java.util.Collections.emptyList();
} else {
sourceJars_ = null;
sourceJarsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JvmOutputs_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs result) {
if (binaryJarsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
binaryJars_ = java.util.Collections.unmodifiableList(binaryJars_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.binaryJars_ = binaryJars_;
} else {
result.binaryJars_ = binaryJarsBuilder_.build();
}
if (interfaceJarsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
interfaceJars_ = java.util.Collections.unmodifiableList(interfaceJars_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.interfaceJars_ = interfaceJars_;
} else {
result.interfaceJars_ = interfaceJarsBuilder_.build();
}
if (sourceJarsBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
sourceJars_ = java.util.Collections.unmodifiableList(sourceJars_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.sourceJars_ = sourceJars_;
} else {
result.sourceJars_ = sourceJarsBuilder_.build();
}
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.getDefaultInstance()) return this;
if (binaryJarsBuilder_ == null) {
if (!other.binaryJars_.isEmpty()) {
if (binaryJars_.isEmpty()) {
binaryJars_ = other.binaryJars_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureBinaryJarsIsMutable();
binaryJars_.addAll(other.binaryJars_);
}
onChanged();
}
} else {
if (!other.binaryJars_.isEmpty()) {
if (binaryJarsBuilder_.isEmpty()) {
binaryJarsBuilder_.dispose();
binaryJarsBuilder_ = null;
binaryJars_ = other.binaryJars_;
bitField0_ = (bitField0_ & ~0x00000001);
binaryJarsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getBinaryJarsFieldBuilder() : null;
} else {
binaryJarsBuilder_.addAllMessages(other.binaryJars_);
}
}
}
if (interfaceJarsBuilder_ == null) {
if (!other.interfaceJars_.isEmpty()) {
if (interfaceJars_.isEmpty()) {
interfaceJars_ = other.interfaceJars_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureInterfaceJarsIsMutable();
interfaceJars_.addAll(other.interfaceJars_);
}
onChanged();
}
} else {
if (!other.interfaceJars_.isEmpty()) {
if (interfaceJarsBuilder_.isEmpty()) {
interfaceJarsBuilder_.dispose();
interfaceJarsBuilder_ = null;
interfaceJars_ = other.interfaceJars_;
bitField0_ = (bitField0_ & ~0x00000002);
interfaceJarsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getInterfaceJarsFieldBuilder() : null;
} else {
interfaceJarsBuilder_.addAllMessages(other.interfaceJars_);
}
}
}
if (sourceJarsBuilder_ == null) {
if (!other.sourceJars_.isEmpty()) {
if (sourceJars_.isEmpty()) {
sourceJars_ = other.sourceJars_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureSourceJarsIsMutable();
sourceJars_.addAll(other.sourceJars_);
}
onChanged();
}
} else {
if (!other.sourceJars_.isEmpty()) {
if (sourceJarsBuilder_.isEmpty()) {
sourceJarsBuilder_.dispose();
sourceJarsBuilder_ = null;
sourceJars_ = other.sourceJars_;
bitField0_ = (bitField0_ & ~0x00000004);
sourceJarsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getSourceJarsFieldBuilder() : null;
} else {
sourceJarsBuilder_.addAllMessages(other.sourceJars_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (binaryJarsBuilder_ == null) {
ensureBinaryJarsIsMutable();
binaryJars_.add(m);
} else {
binaryJarsBuilder_.addMessage(m);
}
break;
} // case 10
case 18: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (interfaceJarsBuilder_ == null) {
ensureInterfaceJarsIsMutable();
interfaceJars_.add(m);
} else {
interfaceJarsBuilder_.addMessage(m);
}
break;
} // case 18
case 26: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (sourceJarsBuilder_ == null) {
ensureSourceJarsIsMutable();
sourceJars_.add(m);
} else {
sourceJarsBuilder_.addMessage(m);
}
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List binaryJars_ =
java.util.Collections.emptyList();
private void ensureBinaryJarsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
binaryJars_ = new java.util.ArrayList(binaryJars_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> binaryJarsBuilder_;
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public java.util.List getBinaryJarsList() {
if (binaryJarsBuilder_ == null) {
return java.util.Collections.unmodifiableList(binaryJars_);
} else {
return binaryJarsBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public int getBinaryJarsCount() {
if (binaryJarsBuilder_ == null) {
return binaryJars_.size();
} else {
return binaryJarsBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getBinaryJars(int index) {
if (binaryJarsBuilder_ == null) {
return binaryJars_.get(index);
} else {
return binaryJarsBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public Builder setBinaryJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (binaryJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBinaryJarsIsMutable();
binaryJars_.set(index, value);
onChanged();
} else {
binaryJarsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public Builder setBinaryJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (binaryJarsBuilder_ == null) {
ensureBinaryJarsIsMutable();
binaryJars_.set(index, builderForValue.build());
onChanged();
} else {
binaryJarsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public Builder addBinaryJars(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (binaryJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBinaryJarsIsMutable();
binaryJars_.add(value);
onChanged();
} else {
binaryJarsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public Builder addBinaryJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (binaryJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBinaryJarsIsMutable();
binaryJars_.add(index, value);
onChanged();
} else {
binaryJarsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public Builder addBinaryJars(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (binaryJarsBuilder_ == null) {
ensureBinaryJarsIsMutable();
binaryJars_.add(builderForValue.build());
onChanged();
} else {
binaryJarsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public Builder addBinaryJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (binaryJarsBuilder_ == null) {
ensureBinaryJarsIsMutable();
binaryJars_.add(index, builderForValue.build());
onChanged();
} else {
binaryJarsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public Builder addAllBinaryJars(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (binaryJarsBuilder_ == null) {
ensureBinaryJarsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, binaryJars_);
onChanged();
} else {
binaryJarsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public Builder clearBinaryJars() {
if (binaryJarsBuilder_ == null) {
binaryJars_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
binaryJarsBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public Builder removeBinaryJars(int index) {
if (binaryJarsBuilder_ == null) {
ensureBinaryJarsIsMutable();
binaryJars_.remove(index);
onChanged();
} else {
binaryJarsBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getBinaryJarsBuilder(
int index) {
return getBinaryJarsFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getBinaryJarsOrBuilder(
int index) {
if (binaryJarsBuilder_ == null) {
return binaryJars_.get(index); } else {
return binaryJarsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getBinaryJarsOrBuilderList() {
if (binaryJarsBuilder_ != null) {
return binaryJarsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(binaryJars_);
}
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addBinaryJarsBuilder() {
return getBinaryJarsFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addBinaryJarsBuilder(
int index) {
return getBinaryJarsFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation binary_jars = 1;
*/
public java.util.List
getBinaryJarsBuilderList() {
return getBinaryJarsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getBinaryJarsFieldBuilder() {
if (binaryJarsBuilder_ == null) {
binaryJarsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
binaryJars_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
binaryJars_ = null;
}
return binaryJarsBuilder_;
}
private java.util.List interfaceJars_ =
java.util.Collections.emptyList();
private void ensureInterfaceJarsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
interfaceJars_ = new java.util.ArrayList(interfaceJars_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> interfaceJarsBuilder_;
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public java.util.List getInterfaceJarsList() {
if (interfaceJarsBuilder_ == null) {
return java.util.Collections.unmodifiableList(interfaceJars_);
} else {
return interfaceJarsBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public int getInterfaceJarsCount() {
if (interfaceJarsBuilder_ == null) {
return interfaceJars_.size();
} else {
return interfaceJarsBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getInterfaceJars(int index) {
if (interfaceJarsBuilder_ == null) {
return interfaceJars_.get(index);
} else {
return interfaceJarsBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public Builder setInterfaceJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (interfaceJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInterfaceJarsIsMutable();
interfaceJars_.set(index, value);
onChanged();
} else {
interfaceJarsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public Builder setInterfaceJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (interfaceJarsBuilder_ == null) {
ensureInterfaceJarsIsMutable();
interfaceJars_.set(index, builderForValue.build());
onChanged();
} else {
interfaceJarsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public Builder addInterfaceJars(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (interfaceJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInterfaceJarsIsMutable();
interfaceJars_.add(value);
onChanged();
} else {
interfaceJarsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public Builder addInterfaceJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (interfaceJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInterfaceJarsIsMutable();
interfaceJars_.add(index, value);
onChanged();
} else {
interfaceJarsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public Builder addInterfaceJars(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (interfaceJarsBuilder_ == null) {
ensureInterfaceJarsIsMutable();
interfaceJars_.add(builderForValue.build());
onChanged();
} else {
interfaceJarsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public Builder addInterfaceJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (interfaceJarsBuilder_ == null) {
ensureInterfaceJarsIsMutable();
interfaceJars_.add(index, builderForValue.build());
onChanged();
} else {
interfaceJarsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public Builder addAllInterfaceJars(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (interfaceJarsBuilder_ == null) {
ensureInterfaceJarsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, interfaceJars_);
onChanged();
} else {
interfaceJarsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public Builder clearInterfaceJars() {
if (interfaceJarsBuilder_ == null) {
interfaceJars_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
interfaceJarsBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public Builder removeInterfaceJars(int index) {
if (interfaceJarsBuilder_ == null) {
ensureInterfaceJarsIsMutable();
interfaceJars_.remove(index);
onChanged();
} else {
interfaceJarsBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getInterfaceJarsBuilder(
int index) {
return getInterfaceJarsFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getInterfaceJarsOrBuilder(
int index) {
if (interfaceJarsBuilder_ == null) {
return interfaceJars_.get(index); } else {
return interfaceJarsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getInterfaceJarsOrBuilderList() {
if (interfaceJarsBuilder_ != null) {
return interfaceJarsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(interfaceJars_);
}
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addInterfaceJarsBuilder() {
return getInterfaceJarsFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addInterfaceJarsBuilder(
int index) {
return getInterfaceJarsFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation interface_jars = 2;
*/
public java.util.List
getInterfaceJarsBuilderList() {
return getInterfaceJarsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getInterfaceJarsFieldBuilder() {
if (interfaceJarsBuilder_ == null) {
interfaceJarsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
interfaceJars_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
interfaceJars_ = null;
}
return interfaceJarsBuilder_;
}
private java.util.List sourceJars_ =
java.util.Collections.emptyList();
private void ensureSourceJarsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
sourceJars_ = new java.util.ArrayList(sourceJars_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> sourceJarsBuilder_;
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public java.util.List getSourceJarsList() {
if (sourceJarsBuilder_ == null) {
return java.util.Collections.unmodifiableList(sourceJars_);
} else {
return sourceJarsBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public int getSourceJarsCount() {
if (sourceJarsBuilder_ == null) {
return sourceJars_.size();
} else {
return sourceJarsBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getSourceJars(int index) {
if (sourceJarsBuilder_ == null) {
return sourceJars_.get(index);
} else {
return sourceJarsBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public Builder setSourceJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (sourceJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSourceJarsIsMutable();
sourceJars_.set(index, value);
onChanged();
} else {
sourceJarsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public Builder setSourceJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (sourceJarsBuilder_ == null) {
ensureSourceJarsIsMutable();
sourceJars_.set(index, builderForValue.build());
onChanged();
} else {
sourceJarsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public Builder addSourceJars(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (sourceJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSourceJarsIsMutable();
sourceJars_.add(value);
onChanged();
} else {
sourceJarsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public Builder addSourceJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (sourceJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSourceJarsIsMutable();
sourceJars_.add(index, value);
onChanged();
} else {
sourceJarsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public Builder addSourceJars(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (sourceJarsBuilder_ == null) {
ensureSourceJarsIsMutable();
sourceJars_.add(builderForValue.build());
onChanged();
} else {
sourceJarsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public Builder addSourceJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (sourceJarsBuilder_ == null) {
ensureSourceJarsIsMutable();
sourceJars_.add(index, builderForValue.build());
onChanged();
} else {
sourceJarsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public Builder addAllSourceJars(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (sourceJarsBuilder_ == null) {
ensureSourceJarsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sourceJars_);
onChanged();
} else {
sourceJarsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public Builder clearSourceJars() {
if (sourceJarsBuilder_ == null) {
sourceJars_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
sourceJarsBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public Builder removeSourceJars(int index) {
if (sourceJarsBuilder_ == null) {
ensureSourceJarsIsMutable();
sourceJars_.remove(index);
onChanged();
} else {
sourceJarsBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getSourceJarsBuilder(
int index) {
return getSourceJarsFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getSourceJarsOrBuilder(
int index) {
if (sourceJarsBuilder_ == null) {
return sourceJars_.get(index); } else {
return sourceJarsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getSourceJarsOrBuilderList() {
if (sourceJarsBuilder_ != null) {
return sourceJarsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(sourceJars_);
}
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addSourceJarsBuilder() {
return getSourceJarsFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addSourceJarsBuilder(
int index) {
return getSourceJarsFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation source_jars = 3;
*/
public java.util.List
getSourceJarsBuilderList() {
return getSourceJarsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getSourceJarsFieldBuilder() {
if (sourceJarsBuilder_ == null) {
sourceJarsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
sourceJars_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
sourceJars_ = null;
}
return sourceJarsBuilder_;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.JvmOutputs)
}
// @@protoc_insertion_point(class_scope:bazelbsp.JvmOutputs)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JvmOutputs parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JvmTargetInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.JvmTargetInfo)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
java.util.List
getJarsList();
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs getJars(int index);
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
int getJarsCount();
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder>
getJarsOrBuilderList();
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder getJarsOrBuilder(
int index);
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
java.util.List
getGeneratedJarsList();
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs getGeneratedJars(int index);
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
int getGeneratedJarsCount();
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder>
getGeneratedJarsOrBuilderList();
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder getGeneratedJarsOrBuilder(
int index);
/**
* repeated string javac_opts = 6;
* @return A list containing the javacOpts.
*/
java.util.List
getJavacOptsList();
/**
* repeated string javac_opts = 6;
* @return The count of javacOpts.
*/
int getJavacOptsCount();
/**
* repeated string javac_opts = 6;
* @param index The index of the element to return.
* @return The javacOpts at the given index.
*/
java.lang.String getJavacOpts(int index);
/**
* repeated string javac_opts = 6;
* @param index The index of the value to return.
* @return The bytes of the javacOpts at the given index.
*/
com.google.protobuf.ByteString
getJavacOptsBytes(int index);
/**
* repeated string jvm_flags = 7;
* @return A list containing the jvmFlags.
*/
java.util.List
getJvmFlagsList();
/**
* repeated string jvm_flags = 7;
* @return The count of jvmFlags.
*/
int getJvmFlagsCount();
/**
* repeated string jvm_flags = 7;
* @param index The index of the element to return.
* @return The jvmFlags at the given index.
*/
java.lang.String getJvmFlags(int index);
/**
* repeated string jvm_flags = 7;
* @param index The index of the value to return.
* @return The bytes of the jvmFlags at the given index.
*/
com.google.protobuf.ByteString
getJvmFlagsBytes(int index);
/**
* string main_class = 8;
* @return The mainClass.
*/
java.lang.String getMainClass();
/**
* string main_class = 8;
* @return The bytes for mainClass.
*/
com.google.protobuf.ByteString
getMainClassBytes();
/**
* repeated string args = 9;
* @return A list containing the args.
*/
java.util.List
getArgsList();
/**
* repeated string args = 9;
* @return The count of args.
*/
int getArgsCount();
/**
* repeated string args = 9;
* @param index The index of the element to return.
* @return The args at the given index.
*/
java.lang.String getArgs(int index);
/**
* repeated string args = 9;
* @param index The index of the value to return.
* @return The bytes of the args at the given index.
*/
com.google.protobuf.ByteString
getArgsBytes(int index);
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
java.util.List
getJdepsList();
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getJdeps(int index);
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
int getJdepsCount();
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getJdepsOrBuilderList();
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getJdepsOrBuilder(
int index);
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
java.util.List
getTransitiveCompileTimeJarsList();
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getTransitiveCompileTimeJars(int index);
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
int getTransitiveCompileTimeJarsCount();
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getTransitiveCompileTimeJarsOrBuilderList();
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getTransitiveCompileTimeJarsOrBuilder(
int index);
}
/**
* Protobuf type {@code bazelbsp.JvmTargetInfo}
*/
public static final class JvmTargetInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.JvmTargetInfo)
JvmTargetInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
JvmTargetInfo.class.getName());
}
// Use JvmTargetInfo.newBuilder() to construct.
private JvmTargetInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private JvmTargetInfo() {
jars_ = java.util.Collections.emptyList();
generatedJars_ = java.util.Collections.emptyList();
javacOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
jvmFlags_ =
com.google.protobuf.LazyStringArrayList.emptyList();
mainClass_ = "";
args_ =
com.google.protobuf.LazyStringArrayList.emptyList();
jdeps_ = java.util.Collections.emptyList();
transitiveCompileTimeJars_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JvmTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JvmTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo.Builder.class);
}
public static final int JARS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List jars_;
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
@java.lang.Override
public java.util.List getJarsList() {
return jars_;
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder>
getJarsOrBuilderList() {
return jars_;
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
@java.lang.Override
public int getJarsCount() {
return jars_.size();
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs getJars(int index) {
return jars_.get(index);
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder getJarsOrBuilder(
int index) {
return jars_.get(index);
}
public static final int GENERATED_JARS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List generatedJars_;
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
@java.lang.Override
public java.util.List getGeneratedJarsList() {
return generatedJars_;
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder>
getGeneratedJarsOrBuilderList() {
return generatedJars_;
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
@java.lang.Override
public int getGeneratedJarsCount() {
return generatedJars_.size();
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs getGeneratedJars(int index) {
return generatedJars_.get(index);
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder getGeneratedJarsOrBuilder(
int index) {
return generatedJars_.get(index);
}
public static final int JAVAC_OPTS_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList javacOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string javac_opts = 6;
* @return A list containing the javacOpts.
*/
public com.google.protobuf.ProtocolStringList
getJavacOptsList() {
return javacOpts_;
}
/**
* repeated string javac_opts = 6;
* @return The count of javacOpts.
*/
public int getJavacOptsCount() {
return javacOpts_.size();
}
/**
* repeated string javac_opts = 6;
* @param index The index of the element to return.
* @return The javacOpts at the given index.
*/
public java.lang.String getJavacOpts(int index) {
return javacOpts_.get(index);
}
/**
* repeated string javac_opts = 6;
* @param index The index of the value to return.
* @return The bytes of the javacOpts at the given index.
*/
public com.google.protobuf.ByteString
getJavacOptsBytes(int index) {
return javacOpts_.getByteString(index);
}
public static final int JVM_FLAGS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList jvmFlags_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string jvm_flags = 7;
* @return A list containing the jvmFlags.
*/
public com.google.protobuf.ProtocolStringList
getJvmFlagsList() {
return jvmFlags_;
}
/**
* repeated string jvm_flags = 7;
* @return The count of jvmFlags.
*/
public int getJvmFlagsCount() {
return jvmFlags_.size();
}
/**
* repeated string jvm_flags = 7;
* @param index The index of the element to return.
* @return The jvmFlags at the given index.
*/
public java.lang.String getJvmFlags(int index) {
return jvmFlags_.get(index);
}
/**
* repeated string jvm_flags = 7;
* @param index The index of the value to return.
* @return The bytes of the jvmFlags at the given index.
*/
public com.google.protobuf.ByteString
getJvmFlagsBytes(int index) {
return jvmFlags_.getByteString(index);
}
public static final int MAIN_CLASS_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private volatile java.lang.Object mainClass_ = "";
/**
* string main_class = 8;
* @return The mainClass.
*/
@java.lang.Override
public java.lang.String getMainClass() {
java.lang.Object ref = mainClass_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mainClass_ = s;
return s;
}
}
/**
* string main_class = 8;
* @return The bytes for mainClass.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMainClassBytes() {
java.lang.Object ref = mainClass_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mainClass_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ARGS_FIELD_NUMBER = 9;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList args_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string args = 9;
* @return A list containing the args.
*/
public com.google.protobuf.ProtocolStringList
getArgsList() {
return args_;
}
/**
* repeated string args = 9;
* @return The count of args.
*/
public int getArgsCount() {
return args_.size();
}
/**
* repeated string args = 9;
* @param index The index of the element to return.
* @return The args at the given index.
*/
public java.lang.String getArgs(int index) {
return args_.get(index);
}
/**
* repeated string args = 9;
* @param index The index of the value to return.
* @return The bytes of the args at the given index.
*/
public com.google.protobuf.ByteString
getArgsBytes(int index) {
return args_.getByteString(index);
}
public static final int JDEPS_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private java.util.List jdeps_;
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
@java.lang.Override
public java.util.List getJdepsList() {
return jdeps_;
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getJdepsOrBuilderList() {
return jdeps_;
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
@java.lang.Override
public int getJdepsCount() {
return jdeps_.size();
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getJdeps(int index) {
return jdeps_.get(index);
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getJdepsOrBuilder(
int index) {
return jdeps_.get(index);
}
public static final int TRANSITIVE_COMPILE_TIME_JARS_FIELD_NUMBER = 11;
@SuppressWarnings("serial")
private java.util.List transitiveCompileTimeJars_;
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
@java.lang.Override
public java.util.List getTransitiveCompileTimeJarsList() {
return transitiveCompileTimeJars_;
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getTransitiveCompileTimeJarsOrBuilderList() {
return transitiveCompileTimeJars_;
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
@java.lang.Override
public int getTransitiveCompileTimeJarsCount() {
return transitiveCompileTimeJars_.size();
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getTransitiveCompileTimeJars(int index) {
return transitiveCompileTimeJars_.get(index);
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getTransitiveCompileTimeJarsOrBuilder(
int index) {
return transitiveCompileTimeJars_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < jars_.size(); i++) {
output.writeMessage(1, jars_.get(i));
}
for (int i = 0; i < generatedJars_.size(); i++) {
output.writeMessage(2, generatedJars_.get(i));
}
for (int i = 0; i < javacOpts_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 6, javacOpts_.getRaw(i));
}
for (int i = 0; i < jvmFlags_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 7, jvmFlags_.getRaw(i));
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(mainClass_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 8, mainClass_);
}
for (int i = 0; i < args_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 9, args_.getRaw(i));
}
for (int i = 0; i < jdeps_.size(); i++) {
output.writeMessage(10, jdeps_.get(i));
}
for (int i = 0; i < transitiveCompileTimeJars_.size(); i++) {
output.writeMessage(11, transitiveCompileTimeJars_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < jars_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, jars_.get(i));
}
for (int i = 0; i < generatedJars_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, generatedJars_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < javacOpts_.size(); i++) {
dataSize += computeStringSizeNoTag(javacOpts_.getRaw(i));
}
size += dataSize;
size += 1 * getJavacOptsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < jvmFlags_.size(); i++) {
dataSize += computeStringSizeNoTag(jvmFlags_.getRaw(i));
}
size += dataSize;
size += 1 * getJvmFlagsList().size();
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(mainClass_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(8, mainClass_);
}
{
int dataSize = 0;
for (int i = 0; i < args_.size(); i++) {
dataSize += computeStringSizeNoTag(args_.getRaw(i));
}
size += dataSize;
size += 1 * getArgsList().size();
}
for (int i = 0; i < jdeps_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, jdeps_.get(i));
}
for (int i = 0; i < transitiveCompileTimeJars_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, transitiveCompileTimeJars_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo) obj;
if (!getJarsList()
.equals(other.getJarsList())) return false;
if (!getGeneratedJarsList()
.equals(other.getGeneratedJarsList())) return false;
if (!getJavacOptsList()
.equals(other.getJavacOptsList())) return false;
if (!getJvmFlagsList()
.equals(other.getJvmFlagsList())) return false;
if (!getMainClass()
.equals(other.getMainClass())) return false;
if (!getArgsList()
.equals(other.getArgsList())) return false;
if (!getJdepsList()
.equals(other.getJdepsList())) return false;
if (!getTransitiveCompileTimeJarsList()
.equals(other.getTransitiveCompileTimeJarsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getJarsCount() > 0) {
hash = (37 * hash) + JARS_FIELD_NUMBER;
hash = (53 * hash) + getJarsList().hashCode();
}
if (getGeneratedJarsCount() > 0) {
hash = (37 * hash) + GENERATED_JARS_FIELD_NUMBER;
hash = (53 * hash) + getGeneratedJarsList().hashCode();
}
if (getJavacOptsCount() > 0) {
hash = (37 * hash) + JAVAC_OPTS_FIELD_NUMBER;
hash = (53 * hash) + getJavacOptsList().hashCode();
}
if (getJvmFlagsCount() > 0) {
hash = (37 * hash) + JVM_FLAGS_FIELD_NUMBER;
hash = (53 * hash) + getJvmFlagsList().hashCode();
}
hash = (37 * hash) + MAIN_CLASS_FIELD_NUMBER;
hash = (53 * hash) + getMainClass().hashCode();
if (getArgsCount() > 0) {
hash = (37 * hash) + ARGS_FIELD_NUMBER;
hash = (53 * hash) + getArgsList().hashCode();
}
if (getJdepsCount() > 0) {
hash = (37 * hash) + JDEPS_FIELD_NUMBER;
hash = (53 * hash) + getJdepsList().hashCode();
}
if (getTransitiveCompileTimeJarsCount() > 0) {
hash = (37 * hash) + TRANSITIVE_COMPILE_TIME_JARS_FIELD_NUMBER;
hash = (53 * hash) + getTransitiveCompileTimeJarsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.JvmTargetInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.JvmTargetInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JvmTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JvmTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (jarsBuilder_ == null) {
jars_ = java.util.Collections.emptyList();
} else {
jars_ = null;
jarsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (generatedJarsBuilder_ == null) {
generatedJars_ = java.util.Collections.emptyList();
} else {
generatedJars_ = null;
generatedJarsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
javacOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
jvmFlags_ =
com.google.protobuf.LazyStringArrayList.emptyList();
mainClass_ = "";
args_ =
com.google.protobuf.LazyStringArrayList.emptyList();
if (jdepsBuilder_ == null) {
jdeps_ = java.util.Collections.emptyList();
} else {
jdeps_ = null;
jdepsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
if (transitiveCompileTimeJarsBuilder_ == null) {
transitiveCompileTimeJars_ = java.util.Collections.emptyList();
} else {
transitiveCompileTimeJars_ = null;
transitiveCompileTimeJarsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JvmTargetInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo result) {
if (jarsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
jars_ = java.util.Collections.unmodifiableList(jars_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.jars_ = jars_;
} else {
result.jars_ = jarsBuilder_.build();
}
if (generatedJarsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
generatedJars_ = java.util.Collections.unmodifiableList(generatedJars_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.generatedJars_ = generatedJars_;
} else {
result.generatedJars_ = generatedJarsBuilder_.build();
}
if (jdepsBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
jdeps_ = java.util.Collections.unmodifiableList(jdeps_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.jdeps_ = jdeps_;
} else {
result.jdeps_ = jdepsBuilder_.build();
}
if (transitiveCompileTimeJarsBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
transitiveCompileTimeJars_ = java.util.Collections.unmodifiableList(transitiveCompileTimeJars_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.transitiveCompileTimeJars_ = transitiveCompileTimeJars_;
} else {
result.transitiveCompileTimeJars_ = transitiveCompileTimeJarsBuilder_.build();
}
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000004) != 0)) {
javacOpts_.makeImmutable();
result.javacOpts_ = javacOpts_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
jvmFlags_.makeImmutable();
result.jvmFlags_ = jvmFlags_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.mainClass_ = mainClass_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
args_.makeImmutable();
result.args_ = args_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo.getDefaultInstance()) return this;
if (jarsBuilder_ == null) {
if (!other.jars_.isEmpty()) {
if (jars_.isEmpty()) {
jars_ = other.jars_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureJarsIsMutable();
jars_.addAll(other.jars_);
}
onChanged();
}
} else {
if (!other.jars_.isEmpty()) {
if (jarsBuilder_.isEmpty()) {
jarsBuilder_.dispose();
jarsBuilder_ = null;
jars_ = other.jars_;
bitField0_ = (bitField0_ & ~0x00000001);
jarsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getJarsFieldBuilder() : null;
} else {
jarsBuilder_.addAllMessages(other.jars_);
}
}
}
if (generatedJarsBuilder_ == null) {
if (!other.generatedJars_.isEmpty()) {
if (generatedJars_.isEmpty()) {
generatedJars_ = other.generatedJars_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureGeneratedJarsIsMutable();
generatedJars_.addAll(other.generatedJars_);
}
onChanged();
}
} else {
if (!other.generatedJars_.isEmpty()) {
if (generatedJarsBuilder_.isEmpty()) {
generatedJarsBuilder_.dispose();
generatedJarsBuilder_ = null;
generatedJars_ = other.generatedJars_;
bitField0_ = (bitField0_ & ~0x00000002);
generatedJarsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getGeneratedJarsFieldBuilder() : null;
} else {
generatedJarsBuilder_.addAllMessages(other.generatedJars_);
}
}
}
if (!other.javacOpts_.isEmpty()) {
if (javacOpts_.isEmpty()) {
javacOpts_ = other.javacOpts_;
bitField0_ |= 0x00000004;
} else {
ensureJavacOptsIsMutable();
javacOpts_.addAll(other.javacOpts_);
}
onChanged();
}
if (!other.jvmFlags_.isEmpty()) {
if (jvmFlags_.isEmpty()) {
jvmFlags_ = other.jvmFlags_;
bitField0_ |= 0x00000008;
} else {
ensureJvmFlagsIsMutable();
jvmFlags_.addAll(other.jvmFlags_);
}
onChanged();
}
if (!other.getMainClass().isEmpty()) {
mainClass_ = other.mainClass_;
bitField0_ |= 0x00000010;
onChanged();
}
if (!other.args_.isEmpty()) {
if (args_.isEmpty()) {
args_ = other.args_;
bitField0_ |= 0x00000020;
} else {
ensureArgsIsMutable();
args_.addAll(other.args_);
}
onChanged();
}
if (jdepsBuilder_ == null) {
if (!other.jdeps_.isEmpty()) {
if (jdeps_.isEmpty()) {
jdeps_ = other.jdeps_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureJdepsIsMutable();
jdeps_.addAll(other.jdeps_);
}
onChanged();
}
} else {
if (!other.jdeps_.isEmpty()) {
if (jdepsBuilder_.isEmpty()) {
jdepsBuilder_.dispose();
jdepsBuilder_ = null;
jdeps_ = other.jdeps_;
bitField0_ = (bitField0_ & ~0x00000040);
jdepsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getJdepsFieldBuilder() : null;
} else {
jdepsBuilder_.addAllMessages(other.jdeps_);
}
}
}
if (transitiveCompileTimeJarsBuilder_ == null) {
if (!other.transitiveCompileTimeJars_.isEmpty()) {
if (transitiveCompileTimeJars_.isEmpty()) {
transitiveCompileTimeJars_ = other.transitiveCompileTimeJars_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureTransitiveCompileTimeJarsIsMutable();
transitiveCompileTimeJars_.addAll(other.transitiveCompileTimeJars_);
}
onChanged();
}
} else {
if (!other.transitiveCompileTimeJars_.isEmpty()) {
if (transitiveCompileTimeJarsBuilder_.isEmpty()) {
transitiveCompileTimeJarsBuilder_.dispose();
transitiveCompileTimeJarsBuilder_ = null;
transitiveCompileTimeJars_ = other.transitiveCompileTimeJars_;
bitField0_ = (bitField0_ & ~0x00000080);
transitiveCompileTimeJarsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getTransitiveCompileTimeJarsFieldBuilder() : null;
} else {
transitiveCompileTimeJarsBuilder_.addAllMessages(other.transitiveCompileTimeJars_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.parser(),
extensionRegistry);
if (jarsBuilder_ == null) {
ensureJarsIsMutable();
jars_.add(m);
} else {
jarsBuilder_.addMessage(m);
}
break;
} // case 10
case 18: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.parser(),
extensionRegistry);
if (generatedJarsBuilder_ == null) {
ensureGeneratedJarsIsMutable();
generatedJars_.add(m);
} else {
generatedJarsBuilder_.addMessage(m);
}
break;
} // case 18
case 50: {
java.lang.String s = input.readStringRequireUtf8();
ensureJavacOptsIsMutable();
javacOpts_.add(s);
break;
} // case 50
case 58: {
java.lang.String s = input.readStringRequireUtf8();
ensureJvmFlagsIsMutable();
jvmFlags_.add(s);
break;
} // case 58
case 66: {
mainClass_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 66
case 74: {
java.lang.String s = input.readStringRequireUtf8();
ensureArgsIsMutable();
args_.add(s);
break;
} // case 74
case 82: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (jdepsBuilder_ == null) {
ensureJdepsIsMutable();
jdeps_.add(m);
} else {
jdepsBuilder_.addMessage(m);
}
break;
} // case 82
case 90: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (transitiveCompileTimeJarsBuilder_ == null) {
ensureTransitiveCompileTimeJarsIsMutable();
transitiveCompileTimeJars_.add(m);
} else {
transitiveCompileTimeJarsBuilder_.addMessage(m);
}
break;
} // case 90
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List jars_ =
java.util.Collections.emptyList();
private void ensureJarsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
jars_ = new java.util.ArrayList(jars_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder> jarsBuilder_;
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public java.util.List getJarsList() {
if (jarsBuilder_ == null) {
return java.util.Collections.unmodifiableList(jars_);
} else {
return jarsBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public int getJarsCount() {
if (jarsBuilder_ == null) {
return jars_.size();
} else {
return jarsBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs getJars(int index) {
if (jarsBuilder_ == null) {
return jars_.get(index);
} else {
return jarsBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public Builder setJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs value) {
if (jarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJarsIsMutable();
jars_.set(index, value);
onChanged();
} else {
jarsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public Builder setJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder builderForValue) {
if (jarsBuilder_ == null) {
ensureJarsIsMutable();
jars_.set(index, builderForValue.build());
onChanged();
} else {
jarsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public Builder addJars(org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs value) {
if (jarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJarsIsMutable();
jars_.add(value);
onChanged();
} else {
jarsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public Builder addJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs value) {
if (jarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJarsIsMutable();
jars_.add(index, value);
onChanged();
} else {
jarsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public Builder addJars(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder builderForValue) {
if (jarsBuilder_ == null) {
ensureJarsIsMutable();
jars_.add(builderForValue.build());
onChanged();
} else {
jarsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public Builder addJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder builderForValue) {
if (jarsBuilder_ == null) {
ensureJarsIsMutable();
jars_.add(index, builderForValue.build());
onChanged();
} else {
jarsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public Builder addAllJars(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs> values) {
if (jarsBuilder_ == null) {
ensureJarsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, jars_);
onChanged();
} else {
jarsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public Builder clearJars() {
if (jarsBuilder_ == null) {
jars_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
jarsBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public Builder removeJars(int index) {
if (jarsBuilder_ == null) {
ensureJarsIsMutable();
jars_.remove(index);
onChanged();
} else {
jarsBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder getJarsBuilder(
int index) {
return getJarsFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder getJarsOrBuilder(
int index) {
if (jarsBuilder_ == null) {
return jars_.get(index); } else {
return jarsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder>
getJarsOrBuilderList() {
if (jarsBuilder_ != null) {
return jarsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(jars_);
}
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder addJarsBuilder() {
return getJarsFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.getDefaultInstance());
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder addJarsBuilder(
int index) {
return getJarsFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.getDefaultInstance());
}
/**
* repeated .bazelbsp.JvmOutputs jars = 1;
*/
public java.util.List
getJarsBuilderList() {
return getJarsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder>
getJarsFieldBuilder() {
if (jarsBuilder_ == null) {
jarsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder>(
jars_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
jars_ = null;
}
return jarsBuilder_;
}
private java.util.List generatedJars_ =
java.util.Collections.emptyList();
private void ensureGeneratedJarsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
generatedJars_ = new java.util.ArrayList(generatedJars_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder> generatedJarsBuilder_;
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public java.util.List getGeneratedJarsList() {
if (generatedJarsBuilder_ == null) {
return java.util.Collections.unmodifiableList(generatedJars_);
} else {
return generatedJarsBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public int getGeneratedJarsCount() {
if (generatedJarsBuilder_ == null) {
return generatedJars_.size();
} else {
return generatedJarsBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs getGeneratedJars(int index) {
if (generatedJarsBuilder_ == null) {
return generatedJars_.get(index);
} else {
return generatedJarsBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public Builder setGeneratedJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs value) {
if (generatedJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneratedJarsIsMutable();
generatedJars_.set(index, value);
onChanged();
} else {
generatedJarsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public Builder setGeneratedJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder builderForValue) {
if (generatedJarsBuilder_ == null) {
ensureGeneratedJarsIsMutable();
generatedJars_.set(index, builderForValue.build());
onChanged();
} else {
generatedJarsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public Builder addGeneratedJars(org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs value) {
if (generatedJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneratedJarsIsMutable();
generatedJars_.add(value);
onChanged();
} else {
generatedJarsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public Builder addGeneratedJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs value) {
if (generatedJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneratedJarsIsMutable();
generatedJars_.add(index, value);
onChanged();
} else {
generatedJarsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public Builder addGeneratedJars(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder builderForValue) {
if (generatedJarsBuilder_ == null) {
ensureGeneratedJarsIsMutable();
generatedJars_.add(builderForValue.build());
onChanged();
} else {
generatedJarsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public Builder addGeneratedJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder builderForValue) {
if (generatedJarsBuilder_ == null) {
ensureGeneratedJarsIsMutable();
generatedJars_.add(index, builderForValue.build());
onChanged();
} else {
generatedJarsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public Builder addAllGeneratedJars(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs> values) {
if (generatedJarsBuilder_ == null) {
ensureGeneratedJarsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, generatedJars_);
onChanged();
} else {
generatedJarsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public Builder clearGeneratedJars() {
if (generatedJarsBuilder_ == null) {
generatedJars_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
generatedJarsBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public Builder removeGeneratedJars(int index) {
if (generatedJarsBuilder_ == null) {
ensureGeneratedJarsIsMutable();
generatedJars_.remove(index);
onChanged();
} else {
generatedJarsBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder getGeneratedJarsBuilder(
int index) {
return getGeneratedJarsFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder getGeneratedJarsOrBuilder(
int index) {
if (generatedJarsBuilder_ == null) {
return generatedJars_.get(index); } else {
return generatedJarsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder>
getGeneratedJarsOrBuilderList() {
if (generatedJarsBuilder_ != null) {
return generatedJarsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(generatedJars_);
}
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder addGeneratedJarsBuilder() {
return getGeneratedJarsFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.getDefaultInstance());
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder addGeneratedJarsBuilder(
int index) {
return getGeneratedJarsFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.getDefaultInstance());
}
/**
* repeated .bazelbsp.JvmOutputs generated_jars = 2;
*/
public java.util.List
getGeneratedJarsBuilderList() {
return getGeneratedJarsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder>
getGeneratedJarsFieldBuilder() {
if (generatedJarsBuilder_ == null) {
generatedJarsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputs.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmOutputsOrBuilder>(
generatedJars_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
generatedJars_ = null;
}
return generatedJarsBuilder_;
}
private com.google.protobuf.LazyStringArrayList javacOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureJavacOptsIsMutable() {
if (!javacOpts_.isModifiable()) {
javacOpts_ = new com.google.protobuf.LazyStringArrayList(javacOpts_);
}
bitField0_ |= 0x00000004;
}
/**
* repeated string javac_opts = 6;
* @return A list containing the javacOpts.
*/
public com.google.protobuf.ProtocolStringList
getJavacOptsList() {
javacOpts_.makeImmutable();
return javacOpts_;
}
/**
* repeated string javac_opts = 6;
* @return The count of javacOpts.
*/
public int getJavacOptsCount() {
return javacOpts_.size();
}
/**
* repeated string javac_opts = 6;
* @param index The index of the element to return.
* @return The javacOpts at the given index.
*/
public java.lang.String getJavacOpts(int index) {
return javacOpts_.get(index);
}
/**
* repeated string javac_opts = 6;
* @param index The index of the value to return.
* @return The bytes of the javacOpts at the given index.
*/
public com.google.protobuf.ByteString
getJavacOptsBytes(int index) {
return javacOpts_.getByteString(index);
}
/**
* repeated string javac_opts = 6;
* @param index The index to set the value at.
* @param value The javacOpts to set.
* @return This builder for chaining.
*/
public Builder setJavacOpts(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureJavacOptsIsMutable();
javacOpts_.set(index, value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* repeated string javac_opts = 6;
* @param value The javacOpts to add.
* @return This builder for chaining.
*/
public Builder addJavacOpts(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureJavacOptsIsMutable();
javacOpts_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* repeated string javac_opts = 6;
* @param values The javacOpts to add.
* @return This builder for chaining.
*/
public Builder addAllJavacOpts(
java.lang.Iterable values) {
ensureJavacOptsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, javacOpts_);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* repeated string javac_opts = 6;
* @return This builder for chaining.
*/
public Builder clearJavacOpts() {
javacOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);;
onChanged();
return this;
}
/**
* repeated string javac_opts = 6;
* @param value The bytes of the javacOpts to add.
* @return This builder for chaining.
*/
public Builder addJavacOptsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureJavacOptsIsMutable();
javacOpts_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList jvmFlags_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureJvmFlagsIsMutable() {
if (!jvmFlags_.isModifiable()) {
jvmFlags_ = new com.google.protobuf.LazyStringArrayList(jvmFlags_);
}
bitField0_ |= 0x00000008;
}
/**
* repeated string jvm_flags = 7;
* @return A list containing the jvmFlags.
*/
public com.google.protobuf.ProtocolStringList
getJvmFlagsList() {
jvmFlags_.makeImmutable();
return jvmFlags_;
}
/**
* repeated string jvm_flags = 7;
* @return The count of jvmFlags.
*/
public int getJvmFlagsCount() {
return jvmFlags_.size();
}
/**
* repeated string jvm_flags = 7;
* @param index The index of the element to return.
* @return The jvmFlags at the given index.
*/
public java.lang.String getJvmFlags(int index) {
return jvmFlags_.get(index);
}
/**
* repeated string jvm_flags = 7;
* @param index The index of the value to return.
* @return The bytes of the jvmFlags at the given index.
*/
public com.google.protobuf.ByteString
getJvmFlagsBytes(int index) {
return jvmFlags_.getByteString(index);
}
/**
* repeated string jvm_flags = 7;
* @param index The index to set the value at.
* @param value The jvmFlags to set.
* @return This builder for chaining.
*/
public Builder setJvmFlags(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureJvmFlagsIsMutable();
jvmFlags_.set(index, value);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* repeated string jvm_flags = 7;
* @param value The jvmFlags to add.
* @return This builder for chaining.
*/
public Builder addJvmFlags(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureJvmFlagsIsMutable();
jvmFlags_.add(value);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* repeated string jvm_flags = 7;
* @param values The jvmFlags to add.
* @return This builder for chaining.
*/
public Builder addAllJvmFlags(
java.lang.Iterable values) {
ensureJvmFlagsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, jvmFlags_);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* repeated string jvm_flags = 7;
* @return This builder for chaining.
*/
public Builder clearJvmFlags() {
jvmFlags_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);;
onChanged();
return this;
}
/**
* repeated string jvm_flags = 7;
* @param value The bytes of the jvmFlags to add.
* @return This builder for chaining.
*/
public Builder addJvmFlagsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureJvmFlagsIsMutable();
jvmFlags_.add(value);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object mainClass_ = "";
/**
* string main_class = 8;
* @return The mainClass.
*/
public java.lang.String getMainClass() {
java.lang.Object ref = mainClass_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mainClass_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string main_class = 8;
* @return The bytes for mainClass.
*/
public com.google.protobuf.ByteString
getMainClassBytes() {
java.lang.Object ref = mainClass_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mainClass_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string main_class = 8;
* @param value The mainClass to set.
* @return This builder for chaining.
*/
public Builder setMainClass(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
mainClass_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* string main_class = 8;
* @return This builder for chaining.
*/
public Builder clearMainClass() {
mainClass_ = getDefaultInstance().getMainClass();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* string main_class = 8;
* @param value The bytes for mainClass to set.
* @return This builder for chaining.
*/
public Builder setMainClassBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
mainClass_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList args_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureArgsIsMutable() {
if (!args_.isModifiable()) {
args_ = new com.google.protobuf.LazyStringArrayList(args_);
}
bitField0_ |= 0x00000020;
}
/**
* repeated string args = 9;
* @return A list containing the args.
*/
public com.google.protobuf.ProtocolStringList
getArgsList() {
args_.makeImmutable();
return args_;
}
/**
* repeated string args = 9;
* @return The count of args.
*/
public int getArgsCount() {
return args_.size();
}
/**
* repeated string args = 9;
* @param index The index of the element to return.
* @return The args at the given index.
*/
public java.lang.String getArgs(int index) {
return args_.get(index);
}
/**
* repeated string args = 9;
* @param index The index of the value to return.
* @return The bytes of the args at the given index.
*/
public com.google.protobuf.ByteString
getArgsBytes(int index) {
return args_.getByteString(index);
}
/**
* repeated string args = 9;
* @param index The index to set the value at.
* @param value The args to set.
* @return This builder for chaining.
*/
public Builder setArgs(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureArgsIsMutable();
args_.set(index, value);
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* repeated string args = 9;
* @param value The args to add.
* @return This builder for chaining.
*/
public Builder addArgs(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureArgsIsMutable();
args_.add(value);
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* repeated string args = 9;
* @param values The args to add.
* @return This builder for chaining.
*/
public Builder addAllArgs(
java.lang.Iterable values) {
ensureArgsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, args_);
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* repeated string args = 9;
* @return This builder for chaining.
*/
public Builder clearArgs() {
args_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);;
onChanged();
return this;
}
/**
* repeated string args = 9;
* @param value The bytes of the args to add.
* @return This builder for chaining.
*/
public Builder addArgsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureArgsIsMutable();
args_.add(value);
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.util.List jdeps_ =
java.util.Collections.emptyList();
private void ensureJdepsIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
jdeps_ = new java.util.ArrayList(jdeps_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> jdepsBuilder_;
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public java.util.List getJdepsList() {
if (jdepsBuilder_ == null) {
return java.util.Collections.unmodifiableList(jdeps_);
} else {
return jdepsBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public int getJdepsCount() {
if (jdepsBuilder_ == null) {
return jdeps_.size();
} else {
return jdepsBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getJdeps(int index) {
if (jdepsBuilder_ == null) {
return jdeps_.get(index);
} else {
return jdepsBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public Builder setJdeps(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (jdepsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJdepsIsMutable();
jdeps_.set(index, value);
onChanged();
} else {
jdepsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public Builder setJdeps(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (jdepsBuilder_ == null) {
ensureJdepsIsMutable();
jdeps_.set(index, builderForValue.build());
onChanged();
} else {
jdepsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public Builder addJdeps(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (jdepsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJdepsIsMutable();
jdeps_.add(value);
onChanged();
} else {
jdepsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public Builder addJdeps(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (jdepsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJdepsIsMutable();
jdeps_.add(index, value);
onChanged();
} else {
jdepsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public Builder addJdeps(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (jdepsBuilder_ == null) {
ensureJdepsIsMutable();
jdeps_.add(builderForValue.build());
onChanged();
} else {
jdepsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public Builder addJdeps(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (jdepsBuilder_ == null) {
ensureJdepsIsMutable();
jdeps_.add(index, builderForValue.build());
onChanged();
} else {
jdepsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public Builder addAllJdeps(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (jdepsBuilder_ == null) {
ensureJdepsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, jdeps_);
onChanged();
} else {
jdepsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public Builder clearJdeps() {
if (jdepsBuilder_ == null) {
jdeps_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
jdepsBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public Builder removeJdeps(int index) {
if (jdepsBuilder_ == null) {
ensureJdepsIsMutable();
jdeps_.remove(index);
onChanged();
} else {
jdepsBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getJdepsBuilder(
int index) {
return getJdepsFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getJdepsOrBuilder(
int index) {
if (jdepsBuilder_ == null) {
return jdeps_.get(index); } else {
return jdepsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getJdepsOrBuilderList() {
if (jdepsBuilder_ != null) {
return jdepsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(jdeps_);
}
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addJdepsBuilder() {
return getJdepsFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addJdepsBuilder(
int index) {
return getJdepsFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation jdeps = 10;
*/
public java.util.List
getJdepsBuilderList() {
return getJdepsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getJdepsFieldBuilder() {
if (jdepsBuilder_ == null) {
jdepsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
jdeps_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
jdeps_ = null;
}
return jdepsBuilder_;
}
private java.util.List transitiveCompileTimeJars_ =
java.util.Collections.emptyList();
private void ensureTransitiveCompileTimeJarsIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
transitiveCompileTimeJars_ = new java.util.ArrayList(transitiveCompileTimeJars_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> transitiveCompileTimeJarsBuilder_;
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public java.util.List getTransitiveCompileTimeJarsList() {
if (transitiveCompileTimeJarsBuilder_ == null) {
return java.util.Collections.unmodifiableList(transitiveCompileTimeJars_);
} else {
return transitiveCompileTimeJarsBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public int getTransitiveCompileTimeJarsCount() {
if (transitiveCompileTimeJarsBuilder_ == null) {
return transitiveCompileTimeJars_.size();
} else {
return transitiveCompileTimeJarsBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getTransitiveCompileTimeJars(int index) {
if (transitiveCompileTimeJarsBuilder_ == null) {
return transitiveCompileTimeJars_.get(index);
} else {
return transitiveCompileTimeJarsBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public Builder setTransitiveCompileTimeJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (transitiveCompileTimeJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTransitiveCompileTimeJarsIsMutable();
transitiveCompileTimeJars_.set(index, value);
onChanged();
} else {
transitiveCompileTimeJarsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public Builder setTransitiveCompileTimeJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (transitiveCompileTimeJarsBuilder_ == null) {
ensureTransitiveCompileTimeJarsIsMutable();
transitiveCompileTimeJars_.set(index, builderForValue.build());
onChanged();
} else {
transitiveCompileTimeJarsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public Builder addTransitiveCompileTimeJars(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (transitiveCompileTimeJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTransitiveCompileTimeJarsIsMutable();
transitiveCompileTimeJars_.add(value);
onChanged();
} else {
transitiveCompileTimeJarsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public Builder addTransitiveCompileTimeJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (transitiveCompileTimeJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTransitiveCompileTimeJarsIsMutable();
transitiveCompileTimeJars_.add(index, value);
onChanged();
} else {
transitiveCompileTimeJarsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public Builder addTransitiveCompileTimeJars(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (transitiveCompileTimeJarsBuilder_ == null) {
ensureTransitiveCompileTimeJarsIsMutable();
transitiveCompileTimeJars_.add(builderForValue.build());
onChanged();
} else {
transitiveCompileTimeJarsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public Builder addTransitiveCompileTimeJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (transitiveCompileTimeJarsBuilder_ == null) {
ensureTransitiveCompileTimeJarsIsMutable();
transitiveCompileTimeJars_.add(index, builderForValue.build());
onChanged();
} else {
transitiveCompileTimeJarsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public Builder addAllTransitiveCompileTimeJars(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (transitiveCompileTimeJarsBuilder_ == null) {
ensureTransitiveCompileTimeJarsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, transitiveCompileTimeJars_);
onChanged();
} else {
transitiveCompileTimeJarsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public Builder clearTransitiveCompileTimeJars() {
if (transitiveCompileTimeJarsBuilder_ == null) {
transitiveCompileTimeJars_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
transitiveCompileTimeJarsBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public Builder removeTransitiveCompileTimeJars(int index) {
if (transitiveCompileTimeJarsBuilder_ == null) {
ensureTransitiveCompileTimeJarsIsMutable();
transitiveCompileTimeJars_.remove(index);
onChanged();
} else {
transitiveCompileTimeJarsBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getTransitiveCompileTimeJarsBuilder(
int index) {
return getTransitiveCompileTimeJarsFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getTransitiveCompileTimeJarsOrBuilder(
int index) {
if (transitiveCompileTimeJarsBuilder_ == null) {
return transitiveCompileTimeJars_.get(index); } else {
return transitiveCompileTimeJarsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getTransitiveCompileTimeJarsOrBuilderList() {
if (transitiveCompileTimeJarsBuilder_ != null) {
return transitiveCompileTimeJarsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(transitiveCompileTimeJars_);
}
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addTransitiveCompileTimeJarsBuilder() {
return getTransitiveCompileTimeJarsFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addTransitiveCompileTimeJarsBuilder(
int index) {
return getTransitiveCompileTimeJarsFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation transitive_compile_time_jars = 11;
*/
public java.util.List
getTransitiveCompileTimeJarsBuilderList() {
return getTransitiveCompileTimeJarsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getTransitiveCompileTimeJarsFieldBuilder() {
if (transitiveCompileTimeJarsBuilder_ == null) {
transitiveCompileTimeJarsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
transitiveCompileTimeJars_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
transitiveCompileTimeJars_ = null;
}
return transitiveCompileTimeJarsBuilder_;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.JvmTargetInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.JvmTargetInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JvmTargetInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JvmTargetInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JavaToolchainInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.JavaToolchainInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string source_version = 1;
* @return The sourceVersion.
*/
java.lang.String getSourceVersion();
/**
* string source_version = 1;
* @return The bytes for sourceVersion.
*/
com.google.protobuf.ByteString
getSourceVersionBytes();
/**
* string target_version = 2;
* @return The targetVersion.
*/
java.lang.String getTargetVersion();
/**
* string target_version = 2;
* @return The bytes for targetVersion.
*/
com.google.protobuf.ByteString
getTargetVersionBytes();
/**
* .bazelbsp.FileLocation java_home = 3;
* @return Whether the javaHome field is set.
*/
boolean hasJavaHome();
/**
* .bazelbsp.FileLocation java_home = 3;
* @return The javaHome.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getJavaHome();
/**
* .bazelbsp.FileLocation java_home = 3;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getJavaHomeOrBuilder();
}
/**
* Protobuf type {@code bazelbsp.JavaToolchainInfo}
*/
public static final class JavaToolchainInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.JavaToolchainInfo)
JavaToolchainInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
JavaToolchainInfo.class.getName());
}
// Use JavaToolchainInfo.newBuilder() to construct.
private JavaToolchainInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private JavaToolchainInfo() {
sourceVersion_ = "";
targetVersion_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JavaToolchainInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JavaToolchainInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo.Builder.class);
}
private int bitField0_;
public static final int SOURCE_VERSION_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceVersion_ = "";
/**
* string source_version = 1;
* @return The sourceVersion.
*/
@java.lang.Override
public java.lang.String getSourceVersion() {
java.lang.Object ref = sourceVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceVersion_ = s;
return s;
}
}
/**
* string source_version = 1;
* @return The bytes for sourceVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSourceVersionBytes() {
java.lang.Object ref = sourceVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TARGET_VERSION_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object targetVersion_ = "";
/**
* string target_version = 2;
* @return The targetVersion.
*/
@java.lang.Override
public java.lang.String getTargetVersion() {
java.lang.Object ref = targetVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
targetVersion_ = s;
return s;
}
}
/**
* string target_version = 2;
* @return The bytes for targetVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTargetVersionBytes() {
java.lang.Object ref = targetVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
targetVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JAVA_HOME_FIELD_NUMBER = 3;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation javaHome_;
/**
* .bazelbsp.FileLocation java_home = 3;
* @return Whether the javaHome field is set.
*/
@java.lang.Override
public boolean hasJavaHome() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .bazelbsp.FileLocation java_home = 3;
* @return The javaHome.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getJavaHome() {
return javaHome_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : javaHome_;
}
/**
* .bazelbsp.FileLocation java_home = 3;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getJavaHomeOrBuilder() {
return javaHome_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : javaHome_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(sourceVersion_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, sourceVersion_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(targetVersion_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, targetVersion_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(3, getJavaHome());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(sourceVersion_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, sourceVersion_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(targetVersion_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, targetVersion_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getJavaHome());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo) obj;
if (!getSourceVersion()
.equals(other.getSourceVersion())) return false;
if (!getTargetVersion()
.equals(other.getTargetVersion())) return false;
if (hasJavaHome() != other.hasJavaHome()) return false;
if (hasJavaHome()) {
if (!getJavaHome()
.equals(other.getJavaHome())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SOURCE_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getSourceVersion().hashCode();
hash = (37 * hash) + TARGET_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getTargetVersion().hashCode();
if (hasJavaHome()) {
hash = (37 * hash) + JAVA_HOME_FIELD_NUMBER;
hash = (53 * hash) + getJavaHome().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.JavaToolchainInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.JavaToolchainInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JavaToolchainInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JavaToolchainInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage
.alwaysUseFieldBuilders) {
getJavaHomeFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
sourceVersion_ = "";
targetVersion_ = "";
javaHome_ = null;
if (javaHomeBuilder_ != null) {
javaHomeBuilder_.dispose();
javaHomeBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JavaToolchainInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.sourceVersion_ = sourceVersion_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.targetVersion_ = targetVersion_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.javaHome_ = javaHomeBuilder_ == null
? javaHome_
: javaHomeBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo.getDefaultInstance()) return this;
if (!other.getSourceVersion().isEmpty()) {
sourceVersion_ = other.sourceVersion_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getTargetVersion().isEmpty()) {
targetVersion_ = other.targetVersion_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasJavaHome()) {
mergeJavaHome(other.getJavaHome());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
sourceVersion_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
targetVersion_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
input.readMessage(
getJavaHomeFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object sourceVersion_ = "";
/**
* string source_version = 1;
* @return The sourceVersion.
*/
public java.lang.String getSourceVersion() {
java.lang.Object ref = sourceVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string source_version = 1;
* @return The bytes for sourceVersion.
*/
public com.google.protobuf.ByteString
getSourceVersionBytes() {
java.lang.Object ref = sourceVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string source_version = 1;
* @param value The sourceVersion to set.
* @return This builder for chaining.
*/
public Builder setSourceVersion(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
sourceVersion_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string source_version = 1;
* @return This builder for chaining.
*/
public Builder clearSourceVersion() {
sourceVersion_ = getDefaultInstance().getSourceVersion();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string source_version = 1;
* @param value The bytes for sourceVersion to set.
* @return This builder for chaining.
*/
public Builder setSourceVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
sourceVersion_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object targetVersion_ = "";
/**
* string target_version = 2;
* @return The targetVersion.
*/
public java.lang.String getTargetVersion() {
java.lang.Object ref = targetVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
targetVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string target_version = 2;
* @return The bytes for targetVersion.
*/
public com.google.protobuf.ByteString
getTargetVersionBytes() {
java.lang.Object ref = targetVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
targetVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string target_version = 2;
* @param value The targetVersion to set.
* @return This builder for chaining.
*/
public Builder setTargetVersion(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
targetVersion_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string target_version = 2;
* @return This builder for chaining.
*/
public Builder clearTargetVersion() {
targetVersion_ = getDefaultInstance().getTargetVersion();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string target_version = 2;
* @param value The bytes for targetVersion to set.
* @return This builder for chaining.
*/
public Builder setTargetVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
targetVersion_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation javaHome_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> javaHomeBuilder_;
/**
* .bazelbsp.FileLocation java_home = 3;
* @return Whether the javaHome field is set.
*/
public boolean hasJavaHome() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* .bazelbsp.FileLocation java_home = 3;
* @return The javaHome.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getJavaHome() {
if (javaHomeBuilder_ == null) {
return javaHome_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : javaHome_;
} else {
return javaHomeBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation java_home = 3;
*/
public Builder setJavaHome(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (javaHomeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
javaHome_ = value;
} else {
javaHomeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation java_home = 3;
*/
public Builder setJavaHome(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (javaHomeBuilder_ == null) {
javaHome_ = builderForValue.build();
} else {
javaHomeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation java_home = 3;
*/
public Builder mergeJavaHome(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (javaHomeBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
javaHome_ != null &&
javaHome_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getJavaHomeBuilder().mergeFrom(value);
} else {
javaHome_ = value;
}
} else {
javaHomeBuilder_.mergeFrom(value);
}
if (javaHome_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation java_home = 3;
*/
public Builder clearJavaHome() {
bitField0_ = (bitField0_ & ~0x00000004);
javaHome_ = null;
if (javaHomeBuilder_ != null) {
javaHomeBuilder_.dispose();
javaHomeBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation java_home = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getJavaHomeBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getJavaHomeFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation java_home = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getJavaHomeOrBuilder() {
if (javaHomeBuilder_ != null) {
return javaHomeBuilder_.getMessageOrBuilder();
} else {
return javaHome_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : javaHome_;
}
}
/**
* .bazelbsp.FileLocation java_home = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getJavaHomeFieldBuilder() {
if (javaHomeBuilder_ == null) {
javaHomeBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getJavaHome(),
getParentForChildren(),
isClean());
javaHome_ = null;
}
return javaHomeBuilder_;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.JavaToolchainInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.JavaToolchainInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JavaToolchainInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaToolchainInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JavaRuntimeInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.JavaRuntimeInfo)
com.google.protobuf.MessageOrBuilder {
/**
* .bazelbsp.FileLocation java_home = 1;
* @return Whether the javaHome field is set.
*/
boolean hasJavaHome();
/**
* .bazelbsp.FileLocation java_home = 1;
* @return The javaHome.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getJavaHome();
/**
* .bazelbsp.FileLocation java_home = 1;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getJavaHomeOrBuilder();
}
/**
* Protobuf type {@code bazelbsp.JavaRuntimeInfo}
*/
public static final class JavaRuntimeInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.JavaRuntimeInfo)
JavaRuntimeInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
JavaRuntimeInfo.class.getName());
}
// Use JavaRuntimeInfo.newBuilder() to construct.
private JavaRuntimeInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private JavaRuntimeInfo() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JavaRuntimeInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JavaRuntimeInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo.Builder.class);
}
private int bitField0_;
public static final int JAVA_HOME_FIELD_NUMBER = 1;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation javaHome_;
/**
* .bazelbsp.FileLocation java_home = 1;
* @return Whether the javaHome field is set.
*/
@java.lang.Override
public boolean hasJavaHome() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .bazelbsp.FileLocation java_home = 1;
* @return The javaHome.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getJavaHome() {
return javaHome_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : javaHome_;
}
/**
* .bazelbsp.FileLocation java_home = 1;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getJavaHomeOrBuilder() {
return javaHome_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : javaHome_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getJavaHome());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getJavaHome());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo) obj;
if (hasJavaHome() != other.hasJavaHome()) return false;
if (hasJavaHome()) {
if (!getJavaHome()
.equals(other.getJavaHome())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasJavaHome()) {
hash = (37 * hash) + JAVA_HOME_FIELD_NUMBER;
hash = (53 * hash) + getJavaHome().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.JavaRuntimeInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.JavaRuntimeInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JavaRuntimeInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JavaRuntimeInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage
.alwaysUseFieldBuilders) {
getJavaHomeFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
javaHome_ = null;
if (javaHomeBuilder_ != null) {
javaHomeBuilder_.dispose();
javaHomeBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_JavaRuntimeInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.javaHome_ = javaHomeBuilder_ == null
? javaHome_
: javaHomeBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo.getDefaultInstance()) return this;
if (other.hasJavaHome()) {
mergeJavaHome(other.getJavaHome());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getJavaHomeFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation javaHome_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> javaHomeBuilder_;
/**
* .bazelbsp.FileLocation java_home = 1;
* @return Whether the javaHome field is set.
*/
public boolean hasJavaHome() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .bazelbsp.FileLocation java_home = 1;
* @return The javaHome.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getJavaHome() {
if (javaHomeBuilder_ == null) {
return javaHome_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : javaHome_;
} else {
return javaHomeBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation java_home = 1;
*/
public Builder setJavaHome(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (javaHomeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
javaHome_ = value;
} else {
javaHomeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation java_home = 1;
*/
public Builder setJavaHome(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (javaHomeBuilder_ == null) {
javaHome_ = builderForValue.build();
} else {
javaHomeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation java_home = 1;
*/
public Builder mergeJavaHome(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (javaHomeBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
javaHome_ != null &&
javaHome_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getJavaHomeBuilder().mergeFrom(value);
} else {
javaHome_ = value;
}
} else {
javaHomeBuilder_.mergeFrom(value);
}
if (javaHome_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation java_home = 1;
*/
public Builder clearJavaHome() {
bitField0_ = (bitField0_ & ~0x00000001);
javaHome_ = null;
if (javaHomeBuilder_ != null) {
javaHomeBuilder_.dispose();
javaHomeBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation java_home = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getJavaHomeBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getJavaHomeFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation java_home = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getJavaHomeOrBuilder() {
if (javaHomeBuilder_ != null) {
return javaHomeBuilder_.getMessageOrBuilder();
} else {
return javaHome_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : javaHome_;
}
}
/**
* .bazelbsp.FileLocation java_home = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getJavaHomeFieldBuilder() {
if (javaHomeBuilder_ == null) {
javaHomeBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getJavaHome(),
getParentForChildren(),
isClean());
javaHome_ = null;
}
return javaHomeBuilder_;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.JavaRuntimeInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.JavaRuntimeInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JavaRuntimeInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.JavaRuntimeInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ScalaTargetInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.ScalaTargetInfo)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string scalac_opts = 1;
* @return A list containing the scalacOpts.
*/
java.util.List
getScalacOptsList();
/**
* repeated string scalac_opts = 1;
* @return The count of scalacOpts.
*/
int getScalacOptsCount();
/**
* repeated string scalac_opts = 1;
* @param index The index of the element to return.
* @return The scalacOpts at the given index.
*/
java.lang.String getScalacOpts(int index);
/**
* repeated string scalac_opts = 1;
* @param index The index of the value to return.
* @return The bytes of the scalacOpts at the given index.
*/
com.google.protobuf.ByteString
getScalacOptsBytes(int index);
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
java.util.List
getCompilerClasspathList();
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getCompilerClasspath(int index);
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
int getCompilerClasspathCount();
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getCompilerClasspathOrBuilderList();
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getCompilerClasspathOrBuilder(
int index);
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
java.util.List
getScalatestClasspathList();
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getScalatestClasspath(int index);
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
int getScalatestClasspathCount();
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getScalatestClasspathOrBuilderList();
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getScalatestClasspathOrBuilder(
int index);
}
/**
* Protobuf type {@code bazelbsp.ScalaTargetInfo}
*/
public static final class ScalaTargetInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.ScalaTargetInfo)
ScalaTargetInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
ScalaTargetInfo.class.getName());
}
// Use ScalaTargetInfo.newBuilder() to construct.
private ScalaTargetInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ScalaTargetInfo() {
scalacOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
compilerClasspath_ = java.util.Collections.emptyList();
scalatestClasspath_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_ScalaTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_ScalaTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo.Builder.class);
}
public static final int SCALAC_OPTS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList scalacOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string scalac_opts = 1;
* @return A list containing the scalacOpts.
*/
public com.google.protobuf.ProtocolStringList
getScalacOptsList() {
return scalacOpts_;
}
/**
* repeated string scalac_opts = 1;
* @return The count of scalacOpts.
*/
public int getScalacOptsCount() {
return scalacOpts_.size();
}
/**
* repeated string scalac_opts = 1;
* @param index The index of the element to return.
* @return The scalacOpts at the given index.
*/
public java.lang.String getScalacOpts(int index) {
return scalacOpts_.get(index);
}
/**
* repeated string scalac_opts = 1;
* @param index The index of the value to return.
* @return The bytes of the scalacOpts at the given index.
*/
public com.google.protobuf.ByteString
getScalacOptsBytes(int index) {
return scalacOpts_.getByteString(index);
}
public static final int COMPILER_CLASSPATH_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List compilerClasspath_;
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
@java.lang.Override
public java.util.List getCompilerClasspathList() {
return compilerClasspath_;
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getCompilerClasspathOrBuilderList() {
return compilerClasspath_;
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
@java.lang.Override
public int getCompilerClasspathCount() {
return compilerClasspath_.size();
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getCompilerClasspath(int index) {
return compilerClasspath_.get(index);
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getCompilerClasspathOrBuilder(
int index) {
return compilerClasspath_.get(index);
}
public static final int SCALATEST_CLASSPATH_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List scalatestClasspath_;
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
@java.lang.Override
public java.util.List getScalatestClasspathList() {
return scalatestClasspath_;
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getScalatestClasspathOrBuilderList() {
return scalatestClasspath_;
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
@java.lang.Override
public int getScalatestClasspathCount() {
return scalatestClasspath_.size();
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getScalatestClasspath(int index) {
return scalatestClasspath_.get(index);
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getScalatestClasspathOrBuilder(
int index) {
return scalatestClasspath_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < scalacOpts_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, scalacOpts_.getRaw(i));
}
for (int i = 0; i < compilerClasspath_.size(); i++) {
output.writeMessage(2, compilerClasspath_.get(i));
}
for (int i = 0; i < scalatestClasspath_.size(); i++) {
output.writeMessage(3, scalatestClasspath_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < scalacOpts_.size(); i++) {
dataSize += computeStringSizeNoTag(scalacOpts_.getRaw(i));
}
size += dataSize;
size += 1 * getScalacOptsList().size();
}
for (int i = 0; i < compilerClasspath_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, compilerClasspath_.get(i));
}
for (int i = 0; i < scalatestClasspath_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, scalatestClasspath_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo) obj;
if (!getScalacOptsList()
.equals(other.getScalacOptsList())) return false;
if (!getCompilerClasspathList()
.equals(other.getCompilerClasspathList())) return false;
if (!getScalatestClasspathList()
.equals(other.getScalatestClasspathList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getScalacOptsCount() > 0) {
hash = (37 * hash) + SCALAC_OPTS_FIELD_NUMBER;
hash = (53 * hash) + getScalacOptsList().hashCode();
}
if (getCompilerClasspathCount() > 0) {
hash = (37 * hash) + COMPILER_CLASSPATH_FIELD_NUMBER;
hash = (53 * hash) + getCompilerClasspathList().hashCode();
}
if (getScalatestClasspathCount() > 0) {
hash = (37 * hash) + SCALATEST_CLASSPATH_FIELD_NUMBER;
hash = (53 * hash) + getScalatestClasspathList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.ScalaTargetInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.ScalaTargetInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_ScalaTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_ScalaTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
scalacOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
if (compilerClasspathBuilder_ == null) {
compilerClasspath_ = java.util.Collections.emptyList();
} else {
compilerClasspath_ = null;
compilerClasspathBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (scalatestClasspathBuilder_ == null) {
scalatestClasspath_ = java.util.Collections.emptyList();
} else {
scalatestClasspath_ = null;
scalatestClasspathBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_ScalaTargetInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo result) {
if (compilerClasspathBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
compilerClasspath_ = java.util.Collections.unmodifiableList(compilerClasspath_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.compilerClasspath_ = compilerClasspath_;
} else {
result.compilerClasspath_ = compilerClasspathBuilder_.build();
}
if (scalatestClasspathBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
scalatestClasspath_ = java.util.Collections.unmodifiableList(scalatestClasspath_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.scalatestClasspath_ = scalatestClasspath_;
} else {
result.scalatestClasspath_ = scalatestClasspathBuilder_.build();
}
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
scalacOpts_.makeImmutable();
result.scalacOpts_ = scalacOpts_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo.getDefaultInstance()) return this;
if (!other.scalacOpts_.isEmpty()) {
if (scalacOpts_.isEmpty()) {
scalacOpts_ = other.scalacOpts_;
bitField0_ |= 0x00000001;
} else {
ensureScalacOptsIsMutable();
scalacOpts_.addAll(other.scalacOpts_);
}
onChanged();
}
if (compilerClasspathBuilder_ == null) {
if (!other.compilerClasspath_.isEmpty()) {
if (compilerClasspath_.isEmpty()) {
compilerClasspath_ = other.compilerClasspath_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureCompilerClasspathIsMutable();
compilerClasspath_.addAll(other.compilerClasspath_);
}
onChanged();
}
} else {
if (!other.compilerClasspath_.isEmpty()) {
if (compilerClasspathBuilder_.isEmpty()) {
compilerClasspathBuilder_.dispose();
compilerClasspathBuilder_ = null;
compilerClasspath_ = other.compilerClasspath_;
bitField0_ = (bitField0_ & ~0x00000002);
compilerClasspathBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getCompilerClasspathFieldBuilder() : null;
} else {
compilerClasspathBuilder_.addAllMessages(other.compilerClasspath_);
}
}
}
if (scalatestClasspathBuilder_ == null) {
if (!other.scalatestClasspath_.isEmpty()) {
if (scalatestClasspath_.isEmpty()) {
scalatestClasspath_ = other.scalatestClasspath_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureScalatestClasspathIsMutable();
scalatestClasspath_.addAll(other.scalatestClasspath_);
}
onChanged();
}
} else {
if (!other.scalatestClasspath_.isEmpty()) {
if (scalatestClasspathBuilder_.isEmpty()) {
scalatestClasspathBuilder_.dispose();
scalatestClasspathBuilder_ = null;
scalatestClasspath_ = other.scalatestClasspath_;
bitField0_ = (bitField0_ & ~0x00000004);
scalatestClasspathBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getScalatestClasspathFieldBuilder() : null;
} else {
scalatestClasspathBuilder_.addAllMessages(other.scalatestClasspath_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
ensureScalacOptsIsMutable();
scalacOpts_.add(s);
break;
} // case 10
case 18: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (compilerClasspathBuilder_ == null) {
ensureCompilerClasspathIsMutable();
compilerClasspath_.add(m);
} else {
compilerClasspathBuilder_.addMessage(m);
}
break;
} // case 18
case 26: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (scalatestClasspathBuilder_ == null) {
ensureScalatestClasspathIsMutable();
scalatestClasspath_.add(m);
} else {
scalatestClasspathBuilder_.addMessage(m);
}
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList scalacOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureScalacOptsIsMutable() {
if (!scalacOpts_.isModifiable()) {
scalacOpts_ = new com.google.protobuf.LazyStringArrayList(scalacOpts_);
}
bitField0_ |= 0x00000001;
}
/**
* repeated string scalac_opts = 1;
* @return A list containing the scalacOpts.
*/
public com.google.protobuf.ProtocolStringList
getScalacOptsList() {
scalacOpts_.makeImmutable();
return scalacOpts_;
}
/**
* repeated string scalac_opts = 1;
* @return The count of scalacOpts.
*/
public int getScalacOptsCount() {
return scalacOpts_.size();
}
/**
* repeated string scalac_opts = 1;
* @param index The index of the element to return.
* @return The scalacOpts at the given index.
*/
public java.lang.String getScalacOpts(int index) {
return scalacOpts_.get(index);
}
/**
* repeated string scalac_opts = 1;
* @param index The index of the value to return.
* @return The bytes of the scalacOpts at the given index.
*/
public com.google.protobuf.ByteString
getScalacOptsBytes(int index) {
return scalacOpts_.getByteString(index);
}
/**
* repeated string scalac_opts = 1;
* @param index The index to set the value at.
* @param value The scalacOpts to set.
* @return This builder for chaining.
*/
public Builder setScalacOpts(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureScalacOptsIsMutable();
scalacOpts_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* repeated string scalac_opts = 1;
* @param value The scalacOpts to add.
* @return This builder for chaining.
*/
public Builder addScalacOpts(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureScalacOptsIsMutable();
scalacOpts_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* repeated string scalac_opts = 1;
* @param values The scalacOpts to add.
* @return This builder for chaining.
*/
public Builder addAllScalacOpts(
java.lang.Iterable values) {
ensureScalacOptsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, scalacOpts_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* repeated string scalac_opts = 1;
* @return This builder for chaining.
*/
public Builder clearScalacOpts() {
scalacOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);;
onChanged();
return this;
}
/**
* repeated string scalac_opts = 1;
* @param value The bytes of the scalacOpts to add.
* @return This builder for chaining.
*/
public Builder addScalacOptsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureScalacOptsIsMutable();
scalacOpts_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.util.List compilerClasspath_ =
java.util.Collections.emptyList();
private void ensureCompilerClasspathIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
compilerClasspath_ = new java.util.ArrayList(compilerClasspath_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> compilerClasspathBuilder_;
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public java.util.List getCompilerClasspathList() {
if (compilerClasspathBuilder_ == null) {
return java.util.Collections.unmodifiableList(compilerClasspath_);
} else {
return compilerClasspathBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public int getCompilerClasspathCount() {
if (compilerClasspathBuilder_ == null) {
return compilerClasspath_.size();
} else {
return compilerClasspathBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getCompilerClasspath(int index) {
if (compilerClasspathBuilder_ == null) {
return compilerClasspath_.get(index);
} else {
return compilerClasspathBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public Builder setCompilerClasspath(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (compilerClasspathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCompilerClasspathIsMutable();
compilerClasspath_.set(index, value);
onChanged();
} else {
compilerClasspathBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public Builder setCompilerClasspath(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (compilerClasspathBuilder_ == null) {
ensureCompilerClasspathIsMutable();
compilerClasspath_.set(index, builderForValue.build());
onChanged();
} else {
compilerClasspathBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public Builder addCompilerClasspath(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (compilerClasspathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCompilerClasspathIsMutable();
compilerClasspath_.add(value);
onChanged();
} else {
compilerClasspathBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public Builder addCompilerClasspath(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (compilerClasspathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCompilerClasspathIsMutable();
compilerClasspath_.add(index, value);
onChanged();
} else {
compilerClasspathBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public Builder addCompilerClasspath(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (compilerClasspathBuilder_ == null) {
ensureCompilerClasspathIsMutable();
compilerClasspath_.add(builderForValue.build());
onChanged();
} else {
compilerClasspathBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public Builder addCompilerClasspath(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (compilerClasspathBuilder_ == null) {
ensureCompilerClasspathIsMutable();
compilerClasspath_.add(index, builderForValue.build());
onChanged();
} else {
compilerClasspathBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public Builder addAllCompilerClasspath(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (compilerClasspathBuilder_ == null) {
ensureCompilerClasspathIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, compilerClasspath_);
onChanged();
} else {
compilerClasspathBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public Builder clearCompilerClasspath() {
if (compilerClasspathBuilder_ == null) {
compilerClasspath_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
compilerClasspathBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public Builder removeCompilerClasspath(int index) {
if (compilerClasspathBuilder_ == null) {
ensureCompilerClasspathIsMutable();
compilerClasspath_.remove(index);
onChanged();
} else {
compilerClasspathBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getCompilerClasspathBuilder(
int index) {
return getCompilerClasspathFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getCompilerClasspathOrBuilder(
int index) {
if (compilerClasspathBuilder_ == null) {
return compilerClasspath_.get(index); } else {
return compilerClasspathBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getCompilerClasspathOrBuilderList() {
if (compilerClasspathBuilder_ != null) {
return compilerClasspathBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(compilerClasspath_);
}
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addCompilerClasspathBuilder() {
return getCompilerClasspathFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addCompilerClasspathBuilder(
int index) {
return getCompilerClasspathFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation compiler_classpath = 2;
*/
public java.util.List
getCompilerClasspathBuilderList() {
return getCompilerClasspathFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getCompilerClasspathFieldBuilder() {
if (compilerClasspathBuilder_ == null) {
compilerClasspathBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
compilerClasspath_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
compilerClasspath_ = null;
}
return compilerClasspathBuilder_;
}
private java.util.List scalatestClasspath_ =
java.util.Collections.emptyList();
private void ensureScalatestClasspathIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
scalatestClasspath_ = new java.util.ArrayList(scalatestClasspath_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> scalatestClasspathBuilder_;
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public java.util.List getScalatestClasspathList() {
if (scalatestClasspathBuilder_ == null) {
return java.util.Collections.unmodifiableList(scalatestClasspath_);
} else {
return scalatestClasspathBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public int getScalatestClasspathCount() {
if (scalatestClasspathBuilder_ == null) {
return scalatestClasspath_.size();
} else {
return scalatestClasspathBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getScalatestClasspath(int index) {
if (scalatestClasspathBuilder_ == null) {
return scalatestClasspath_.get(index);
} else {
return scalatestClasspathBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public Builder setScalatestClasspath(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (scalatestClasspathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureScalatestClasspathIsMutable();
scalatestClasspath_.set(index, value);
onChanged();
} else {
scalatestClasspathBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public Builder setScalatestClasspath(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (scalatestClasspathBuilder_ == null) {
ensureScalatestClasspathIsMutable();
scalatestClasspath_.set(index, builderForValue.build());
onChanged();
} else {
scalatestClasspathBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public Builder addScalatestClasspath(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (scalatestClasspathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureScalatestClasspathIsMutable();
scalatestClasspath_.add(value);
onChanged();
} else {
scalatestClasspathBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public Builder addScalatestClasspath(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (scalatestClasspathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureScalatestClasspathIsMutable();
scalatestClasspath_.add(index, value);
onChanged();
} else {
scalatestClasspathBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public Builder addScalatestClasspath(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (scalatestClasspathBuilder_ == null) {
ensureScalatestClasspathIsMutable();
scalatestClasspath_.add(builderForValue.build());
onChanged();
} else {
scalatestClasspathBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public Builder addScalatestClasspath(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (scalatestClasspathBuilder_ == null) {
ensureScalatestClasspathIsMutable();
scalatestClasspath_.add(index, builderForValue.build());
onChanged();
} else {
scalatestClasspathBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public Builder addAllScalatestClasspath(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (scalatestClasspathBuilder_ == null) {
ensureScalatestClasspathIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, scalatestClasspath_);
onChanged();
} else {
scalatestClasspathBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public Builder clearScalatestClasspath() {
if (scalatestClasspathBuilder_ == null) {
scalatestClasspath_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
scalatestClasspathBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public Builder removeScalatestClasspath(int index) {
if (scalatestClasspathBuilder_ == null) {
ensureScalatestClasspathIsMutable();
scalatestClasspath_.remove(index);
onChanged();
} else {
scalatestClasspathBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getScalatestClasspathBuilder(
int index) {
return getScalatestClasspathFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getScalatestClasspathOrBuilder(
int index) {
if (scalatestClasspathBuilder_ == null) {
return scalatestClasspath_.get(index); } else {
return scalatestClasspathBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getScalatestClasspathOrBuilderList() {
if (scalatestClasspathBuilder_ != null) {
return scalatestClasspathBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(scalatestClasspath_);
}
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addScalatestClasspathBuilder() {
return getScalatestClasspathFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addScalatestClasspathBuilder(
int index) {
return getScalatestClasspathFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation scalatest_classpath = 3;
*/
public java.util.List
getScalatestClasspathBuilderList() {
return getScalatestClasspathFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getScalatestClasspathFieldBuilder() {
if (scalatestClasspathBuilder_ == null) {
scalatestClasspathBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
scalatestClasspath_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
scalatestClasspath_ = null;
}
return scalatestClasspathBuilder_;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.ScalaTargetInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.ScalaTargetInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ScalaTargetInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.ScalaTargetInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CppTargetInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.CppTargetInfo)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string copts = 1;
* @return A list containing the copts.
*/
java.util.List
getCoptsList();
/**
* repeated string copts = 1;
* @return The count of copts.
*/
int getCoptsCount();
/**
* repeated string copts = 1;
* @param index The index of the element to return.
* @return The copts at the given index.
*/
java.lang.String getCopts(int index);
/**
* repeated string copts = 1;
* @param index The index of the value to return.
* @return The bytes of the copts at the given index.
*/
com.google.protobuf.ByteString
getCoptsBytes(int index);
/**
* repeated string defines = 2;
* @return A list containing the defines.
*/
java.util.List
getDefinesList();
/**
* repeated string defines = 2;
* @return The count of defines.
*/
int getDefinesCount();
/**
* repeated string defines = 2;
* @param index The index of the element to return.
* @return The defines at the given index.
*/
java.lang.String getDefines(int index);
/**
* repeated string defines = 2;
* @param index The index of the value to return.
* @return The bytes of the defines at the given index.
*/
com.google.protobuf.ByteString
getDefinesBytes(int index);
/**
* repeated string link_opts = 3;
* @return A list containing the linkOpts.
*/
java.util.List
getLinkOptsList();
/**
* repeated string link_opts = 3;
* @return The count of linkOpts.
*/
int getLinkOptsCount();
/**
* repeated string link_opts = 3;
* @param index The index of the element to return.
* @return The linkOpts at the given index.
*/
java.lang.String getLinkOpts(int index);
/**
* repeated string link_opts = 3;
* @param index The index of the value to return.
* @return The bytes of the linkOpts at the given index.
*/
com.google.protobuf.ByteString
getLinkOptsBytes(int index);
/**
* bool link_shared = 4;
* @return The linkShared.
*/
boolean getLinkShared();
}
/**
* Protobuf type {@code bazelbsp.CppTargetInfo}
*/
public static final class CppTargetInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.CppTargetInfo)
CppTargetInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
CppTargetInfo.class.getName());
}
// Use CppTargetInfo.newBuilder() to construct.
private CppTargetInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private CppTargetInfo() {
copts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
defines_ =
com.google.protobuf.LazyStringArrayList.emptyList();
linkOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_CppTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_CppTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo.Builder.class);
}
public static final int COPTS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList copts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string copts = 1;
* @return A list containing the copts.
*/
public com.google.protobuf.ProtocolStringList
getCoptsList() {
return copts_;
}
/**
* repeated string copts = 1;
* @return The count of copts.
*/
public int getCoptsCount() {
return copts_.size();
}
/**
* repeated string copts = 1;
* @param index The index of the element to return.
* @return The copts at the given index.
*/
public java.lang.String getCopts(int index) {
return copts_.get(index);
}
/**
* repeated string copts = 1;
* @param index The index of the value to return.
* @return The bytes of the copts at the given index.
*/
public com.google.protobuf.ByteString
getCoptsBytes(int index) {
return copts_.getByteString(index);
}
public static final int DEFINES_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList defines_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string defines = 2;
* @return A list containing the defines.
*/
public com.google.protobuf.ProtocolStringList
getDefinesList() {
return defines_;
}
/**
* repeated string defines = 2;
* @return The count of defines.
*/
public int getDefinesCount() {
return defines_.size();
}
/**
* repeated string defines = 2;
* @param index The index of the element to return.
* @return The defines at the given index.
*/
public java.lang.String getDefines(int index) {
return defines_.get(index);
}
/**
* repeated string defines = 2;
* @param index The index of the value to return.
* @return The bytes of the defines at the given index.
*/
public com.google.protobuf.ByteString
getDefinesBytes(int index) {
return defines_.getByteString(index);
}
public static final int LINK_OPTS_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList linkOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string link_opts = 3;
* @return A list containing the linkOpts.
*/
public com.google.protobuf.ProtocolStringList
getLinkOptsList() {
return linkOpts_;
}
/**
* repeated string link_opts = 3;
* @return The count of linkOpts.
*/
public int getLinkOptsCount() {
return linkOpts_.size();
}
/**
* repeated string link_opts = 3;
* @param index The index of the element to return.
* @return The linkOpts at the given index.
*/
public java.lang.String getLinkOpts(int index) {
return linkOpts_.get(index);
}
/**
* repeated string link_opts = 3;
* @param index The index of the value to return.
* @return The bytes of the linkOpts at the given index.
*/
public com.google.protobuf.ByteString
getLinkOptsBytes(int index) {
return linkOpts_.getByteString(index);
}
public static final int LINK_SHARED_FIELD_NUMBER = 4;
private boolean linkShared_ = false;
/**
* bool link_shared = 4;
* @return The linkShared.
*/
@java.lang.Override
public boolean getLinkShared() {
return linkShared_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < copts_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, copts_.getRaw(i));
}
for (int i = 0; i < defines_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, defines_.getRaw(i));
}
for (int i = 0; i < linkOpts_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, linkOpts_.getRaw(i));
}
if (linkShared_ != false) {
output.writeBool(4, linkShared_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < copts_.size(); i++) {
dataSize += computeStringSizeNoTag(copts_.getRaw(i));
}
size += dataSize;
size += 1 * getCoptsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < defines_.size(); i++) {
dataSize += computeStringSizeNoTag(defines_.getRaw(i));
}
size += dataSize;
size += 1 * getDefinesList().size();
}
{
int dataSize = 0;
for (int i = 0; i < linkOpts_.size(); i++) {
dataSize += computeStringSizeNoTag(linkOpts_.getRaw(i));
}
size += dataSize;
size += 1 * getLinkOptsList().size();
}
if (linkShared_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, linkShared_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo) obj;
if (!getCoptsList()
.equals(other.getCoptsList())) return false;
if (!getDefinesList()
.equals(other.getDefinesList())) return false;
if (!getLinkOptsList()
.equals(other.getLinkOptsList())) return false;
if (getLinkShared()
!= other.getLinkShared()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getCoptsCount() > 0) {
hash = (37 * hash) + COPTS_FIELD_NUMBER;
hash = (53 * hash) + getCoptsList().hashCode();
}
if (getDefinesCount() > 0) {
hash = (37 * hash) + DEFINES_FIELD_NUMBER;
hash = (53 * hash) + getDefinesList().hashCode();
}
if (getLinkOptsCount() > 0) {
hash = (37 * hash) + LINK_OPTS_FIELD_NUMBER;
hash = (53 * hash) + getLinkOptsList().hashCode();
}
hash = (37 * hash) + LINK_SHARED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getLinkShared());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.CppTargetInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.CppTargetInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_CppTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_CppTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
copts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
defines_ =
com.google.protobuf.LazyStringArrayList.emptyList();
linkOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
linkShared_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_CppTargetInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
copts_.makeImmutable();
result.copts_ = copts_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
defines_.makeImmutable();
result.defines_ = defines_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
linkOpts_.makeImmutable();
result.linkOpts_ = linkOpts_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.linkShared_ = linkShared_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo.getDefaultInstance()) return this;
if (!other.copts_.isEmpty()) {
if (copts_.isEmpty()) {
copts_ = other.copts_;
bitField0_ |= 0x00000001;
} else {
ensureCoptsIsMutable();
copts_.addAll(other.copts_);
}
onChanged();
}
if (!other.defines_.isEmpty()) {
if (defines_.isEmpty()) {
defines_ = other.defines_;
bitField0_ |= 0x00000002;
} else {
ensureDefinesIsMutable();
defines_.addAll(other.defines_);
}
onChanged();
}
if (!other.linkOpts_.isEmpty()) {
if (linkOpts_.isEmpty()) {
linkOpts_ = other.linkOpts_;
bitField0_ |= 0x00000004;
} else {
ensureLinkOptsIsMutable();
linkOpts_.addAll(other.linkOpts_);
}
onChanged();
}
if (other.getLinkShared() != false) {
setLinkShared(other.getLinkShared());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
ensureCoptsIsMutable();
copts_.add(s);
break;
} // case 10
case 18: {
java.lang.String s = input.readStringRequireUtf8();
ensureDefinesIsMutable();
defines_.add(s);
break;
} // case 18
case 26: {
java.lang.String s = input.readStringRequireUtf8();
ensureLinkOptsIsMutable();
linkOpts_.add(s);
break;
} // case 26
case 32: {
linkShared_ = input.readBool();
bitField0_ |= 0x00000008;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList copts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureCoptsIsMutable() {
if (!copts_.isModifiable()) {
copts_ = new com.google.protobuf.LazyStringArrayList(copts_);
}
bitField0_ |= 0x00000001;
}
/**
* repeated string copts = 1;
* @return A list containing the copts.
*/
public com.google.protobuf.ProtocolStringList
getCoptsList() {
copts_.makeImmutable();
return copts_;
}
/**
* repeated string copts = 1;
* @return The count of copts.
*/
public int getCoptsCount() {
return copts_.size();
}
/**
* repeated string copts = 1;
* @param index The index of the element to return.
* @return The copts at the given index.
*/
public java.lang.String getCopts(int index) {
return copts_.get(index);
}
/**
* repeated string copts = 1;
* @param index The index of the value to return.
* @return The bytes of the copts at the given index.
*/
public com.google.protobuf.ByteString
getCoptsBytes(int index) {
return copts_.getByteString(index);
}
/**
* repeated string copts = 1;
* @param index The index to set the value at.
* @param value The copts to set.
* @return This builder for chaining.
*/
public Builder setCopts(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureCoptsIsMutable();
copts_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* repeated string copts = 1;
* @param value The copts to add.
* @return This builder for chaining.
*/
public Builder addCopts(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureCoptsIsMutable();
copts_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* repeated string copts = 1;
* @param values The copts to add.
* @return This builder for chaining.
*/
public Builder addAllCopts(
java.lang.Iterable values) {
ensureCoptsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, copts_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* repeated string copts = 1;
* @return This builder for chaining.
*/
public Builder clearCopts() {
copts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);;
onChanged();
return this;
}
/**
* repeated string copts = 1;
* @param value The bytes of the copts to add.
* @return This builder for chaining.
*/
public Builder addCoptsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureCoptsIsMutable();
copts_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList defines_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureDefinesIsMutable() {
if (!defines_.isModifiable()) {
defines_ = new com.google.protobuf.LazyStringArrayList(defines_);
}
bitField0_ |= 0x00000002;
}
/**
* repeated string defines = 2;
* @return A list containing the defines.
*/
public com.google.protobuf.ProtocolStringList
getDefinesList() {
defines_.makeImmutable();
return defines_;
}
/**
* repeated string defines = 2;
* @return The count of defines.
*/
public int getDefinesCount() {
return defines_.size();
}
/**
* repeated string defines = 2;
* @param index The index of the element to return.
* @return The defines at the given index.
*/
public java.lang.String getDefines(int index) {
return defines_.get(index);
}
/**
* repeated string defines = 2;
* @param index The index of the value to return.
* @return The bytes of the defines at the given index.
*/
public com.google.protobuf.ByteString
getDefinesBytes(int index) {
return defines_.getByteString(index);
}
/**
* repeated string defines = 2;
* @param index The index to set the value at.
* @param value The defines to set.
* @return This builder for chaining.
*/
public Builder setDefines(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureDefinesIsMutable();
defines_.set(index, value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* repeated string defines = 2;
* @param value The defines to add.
* @return This builder for chaining.
*/
public Builder addDefines(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureDefinesIsMutable();
defines_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* repeated string defines = 2;
* @param values The defines to add.
* @return This builder for chaining.
*/
public Builder addAllDefines(
java.lang.Iterable values) {
ensureDefinesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, defines_);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* repeated string defines = 2;
* @return This builder for chaining.
*/
public Builder clearDefines() {
defines_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);;
onChanged();
return this;
}
/**
* repeated string defines = 2;
* @param value The bytes of the defines to add.
* @return This builder for chaining.
*/
public Builder addDefinesBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureDefinesIsMutable();
defines_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList linkOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureLinkOptsIsMutable() {
if (!linkOpts_.isModifiable()) {
linkOpts_ = new com.google.protobuf.LazyStringArrayList(linkOpts_);
}
bitField0_ |= 0x00000004;
}
/**
* repeated string link_opts = 3;
* @return A list containing the linkOpts.
*/
public com.google.protobuf.ProtocolStringList
getLinkOptsList() {
linkOpts_.makeImmutable();
return linkOpts_;
}
/**
* repeated string link_opts = 3;
* @return The count of linkOpts.
*/
public int getLinkOptsCount() {
return linkOpts_.size();
}
/**
* repeated string link_opts = 3;
* @param index The index of the element to return.
* @return The linkOpts at the given index.
*/
public java.lang.String getLinkOpts(int index) {
return linkOpts_.get(index);
}
/**
* repeated string link_opts = 3;
* @param index The index of the value to return.
* @return The bytes of the linkOpts at the given index.
*/
public com.google.protobuf.ByteString
getLinkOptsBytes(int index) {
return linkOpts_.getByteString(index);
}
/**
* repeated string link_opts = 3;
* @param index The index to set the value at.
* @param value The linkOpts to set.
* @return This builder for chaining.
*/
public Builder setLinkOpts(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureLinkOptsIsMutable();
linkOpts_.set(index, value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* repeated string link_opts = 3;
* @param value The linkOpts to add.
* @return This builder for chaining.
*/
public Builder addLinkOpts(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureLinkOptsIsMutable();
linkOpts_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* repeated string link_opts = 3;
* @param values The linkOpts to add.
* @return This builder for chaining.
*/
public Builder addAllLinkOpts(
java.lang.Iterable values) {
ensureLinkOptsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, linkOpts_);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* repeated string link_opts = 3;
* @return This builder for chaining.
*/
public Builder clearLinkOpts() {
linkOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);;
onChanged();
return this;
}
/**
* repeated string link_opts = 3;
* @param value The bytes of the linkOpts to add.
* @return This builder for chaining.
*/
public Builder addLinkOptsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureLinkOptsIsMutable();
linkOpts_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private boolean linkShared_ ;
/**
* bool link_shared = 4;
* @return The linkShared.
*/
@java.lang.Override
public boolean getLinkShared() {
return linkShared_;
}
/**
* bool link_shared = 4;
* @param value The linkShared to set.
* @return This builder for chaining.
*/
public Builder setLinkShared(boolean value) {
linkShared_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* bool link_shared = 4;
* @return This builder for chaining.
*/
public Builder clearLinkShared() {
bitField0_ = (bitField0_ & ~0x00000008);
linkShared_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.CppTargetInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.CppTargetInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CppTargetInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.CppTargetInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface KotlincPluginOptionOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.KotlincPluginOption)
com.google.protobuf.MessageOrBuilder {
/**
* string plugin_id = 100;
* @return The pluginId.
*/
java.lang.String getPluginId();
/**
* string plugin_id = 100;
* @return The bytes for pluginId.
*/
com.google.protobuf.ByteString
getPluginIdBytes();
/**
* string option_value = 200;
* @return The optionValue.
*/
java.lang.String getOptionValue();
/**
* string option_value = 200;
* @return The bytes for optionValue.
*/
com.google.protobuf.ByteString
getOptionValueBytes();
}
/**
* Protobuf type {@code bazelbsp.KotlincPluginOption}
*/
public static final class KotlincPluginOption extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.KotlincPluginOption)
KotlincPluginOptionOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
KotlincPluginOption.class.getName());
}
// Use KotlincPluginOption.newBuilder() to construct.
private KotlincPluginOption(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private KotlincPluginOption() {
pluginId_ = "";
optionValue_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlincPluginOption_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlincPluginOption_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.Builder.class);
}
public static final int PLUGIN_ID_FIELD_NUMBER = 100;
@SuppressWarnings("serial")
private volatile java.lang.Object pluginId_ = "";
/**
* string plugin_id = 100;
* @return The pluginId.
*/
@java.lang.Override
public java.lang.String getPluginId() {
java.lang.Object ref = pluginId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
pluginId_ = s;
return s;
}
}
/**
* string plugin_id = 100;
* @return The bytes for pluginId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPluginIdBytes() {
java.lang.Object ref = pluginId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pluginId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPTION_VALUE_FIELD_NUMBER = 200;
@SuppressWarnings("serial")
private volatile java.lang.Object optionValue_ = "";
/**
* string option_value = 200;
* @return The optionValue.
*/
@java.lang.Override
public java.lang.String getOptionValue() {
java.lang.Object ref = optionValue_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionValue_ = s;
return s;
}
}
/**
* string option_value = 200;
* @return The bytes for optionValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOptionValueBytes() {
java.lang.Object ref = optionValue_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(pluginId_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 100, pluginId_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(optionValue_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 200, optionValue_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(pluginId_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(100, pluginId_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(optionValue_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(200, optionValue_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption) obj;
if (!getPluginId()
.equals(other.getPluginId())) return false;
if (!getOptionValue()
.equals(other.getOptionValue())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PLUGIN_ID_FIELD_NUMBER;
hash = (53 * hash) + getPluginId().hashCode();
hash = (37 * hash) + OPTION_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getOptionValue().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.KotlincPluginOption}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.KotlincPluginOption)
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOptionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlincPluginOption_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlincPluginOption_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
pluginId_ = "";
optionValue_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlincPluginOption_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.pluginId_ = pluginId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.optionValue_ = optionValue_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.getDefaultInstance()) return this;
if (!other.getPluginId().isEmpty()) {
pluginId_ = other.pluginId_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getOptionValue().isEmpty()) {
optionValue_ = other.optionValue_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 802: {
pluginId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 802
case 1602: {
optionValue_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 1602
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object pluginId_ = "";
/**
* string plugin_id = 100;
* @return The pluginId.
*/
public java.lang.String getPluginId() {
java.lang.Object ref = pluginId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
pluginId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string plugin_id = 100;
* @return The bytes for pluginId.
*/
public com.google.protobuf.ByteString
getPluginIdBytes() {
java.lang.Object ref = pluginId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pluginId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string plugin_id = 100;
* @param value The pluginId to set.
* @return This builder for chaining.
*/
public Builder setPluginId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
pluginId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string plugin_id = 100;
* @return This builder for chaining.
*/
public Builder clearPluginId() {
pluginId_ = getDefaultInstance().getPluginId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string plugin_id = 100;
* @param value The bytes for pluginId to set.
* @return This builder for chaining.
*/
public Builder setPluginIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
pluginId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object optionValue_ = "";
/**
* string option_value = 200;
* @return The optionValue.
*/
public java.lang.String getOptionValue() {
java.lang.Object ref = optionValue_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionValue_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string option_value = 200;
* @return The bytes for optionValue.
*/
public com.google.protobuf.ByteString
getOptionValueBytes() {
java.lang.Object ref = optionValue_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string option_value = 200;
* @param value The optionValue to set.
* @return This builder for chaining.
*/
public Builder setOptionValue(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
optionValue_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string option_value = 200;
* @return This builder for chaining.
*/
public Builder clearOptionValue() {
optionValue_ = getDefaultInstance().getOptionValue();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string option_value = 200;
* @param value The bytes for optionValue to set.
* @return This builder for chaining.
*/
public Builder setOptionValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
optionValue_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.KotlincPluginOption)
}
// @@protoc_insertion_point(class_scope:bazelbsp.KotlincPluginOption)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public KotlincPluginOption parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface KotlincPluginInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.KotlincPluginInfo)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
java.util.List
getPluginJarsList();
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getPluginJars(int index);
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
int getPluginJarsCount();
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getPluginJarsOrBuilderList();
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getPluginJarsOrBuilder(
int index);
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
java.util.List
getKotlincPluginOptionsList();
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption getKotlincPluginOptions(int index);
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
int getKotlincPluginOptionsCount();
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOptionOrBuilder>
getKotlincPluginOptionsOrBuilderList();
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOptionOrBuilder getKotlincPluginOptionsOrBuilder(
int index);
}
/**
* Protobuf type {@code bazelbsp.KotlincPluginInfo}
*/
public static final class KotlincPluginInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.KotlincPluginInfo)
KotlincPluginInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
KotlincPluginInfo.class.getName());
}
// Use KotlincPluginInfo.newBuilder() to construct.
private KotlincPluginInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private KotlincPluginInfo() {
pluginJars_ = java.util.Collections.emptyList();
kotlincPluginOptions_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlincPluginInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlincPluginInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.Builder.class);
}
public static final int PLUGIN_JARS_FIELD_NUMBER = 100;
@SuppressWarnings("serial")
private java.util.List pluginJars_;
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
@java.lang.Override
public java.util.List getPluginJarsList() {
return pluginJars_;
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getPluginJarsOrBuilderList() {
return pluginJars_;
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
@java.lang.Override
public int getPluginJarsCount() {
return pluginJars_.size();
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getPluginJars(int index) {
return pluginJars_.get(index);
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getPluginJarsOrBuilder(
int index) {
return pluginJars_.get(index);
}
public static final int KOTLINC_PLUGIN_OPTIONS_FIELD_NUMBER = 200;
@SuppressWarnings("serial")
private java.util.List kotlincPluginOptions_;
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
@java.lang.Override
public java.util.List getKotlincPluginOptionsList() {
return kotlincPluginOptions_;
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOptionOrBuilder>
getKotlincPluginOptionsOrBuilderList() {
return kotlincPluginOptions_;
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
@java.lang.Override
public int getKotlincPluginOptionsCount() {
return kotlincPluginOptions_.size();
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption getKotlincPluginOptions(int index) {
return kotlincPluginOptions_.get(index);
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOptionOrBuilder getKotlincPluginOptionsOrBuilder(
int index) {
return kotlincPluginOptions_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < pluginJars_.size(); i++) {
output.writeMessage(100, pluginJars_.get(i));
}
for (int i = 0; i < kotlincPluginOptions_.size(); i++) {
output.writeMessage(200, kotlincPluginOptions_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < pluginJars_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(100, pluginJars_.get(i));
}
for (int i = 0; i < kotlincPluginOptions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(200, kotlincPluginOptions_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo) obj;
if (!getPluginJarsList()
.equals(other.getPluginJarsList())) return false;
if (!getKotlincPluginOptionsList()
.equals(other.getKotlincPluginOptionsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getPluginJarsCount() > 0) {
hash = (37 * hash) + PLUGIN_JARS_FIELD_NUMBER;
hash = (53 * hash) + getPluginJarsList().hashCode();
}
if (getKotlincPluginOptionsCount() > 0) {
hash = (37 * hash) + KOTLINC_PLUGIN_OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + getKotlincPluginOptionsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.KotlincPluginInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.KotlincPluginInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlincPluginInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlincPluginInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (pluginJarsBuilder_ == null) {
pluginJars_ = java.util.Collections.emptyList();
} else {
pluginJars_ = null;
pluginJarsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (kotlincPluginOptionsBuilder_ == null) {
kotlincPluginOptions_ = java.util.Collections.emptyList();
} else {
kotlincPluginOptions_ = null;
kotlincPluginOptionsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlincPluginInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo result) {
if (pluginJarsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
pluginJars_ = java.util.Collections.unmodifiableList(pluginJars_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.pluginJars_ = pluginJars_;
} else {
result.pluginJars_ = pluginJarsBuilder_.build();
}
if (kotlincPluginOptionsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
kotlincPluginOptions_ = java.util.Collections.unmodifiableList(kotlincPluginOptions_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.kotlincPluginOptions_ = kotlincPluginOptions_;
} else {
result.kotlincPluginOptions_ = kotlincPluginOptionsBuilder_.build();
}
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.getDefaultInstance()) return this;
if (pluginJarsBuilder_ == null) {
if (!other.pluginJars_.isEmpty()) {
if (pluginJars_.isEmpty()) {
pluginJars_ = other.pluginJars_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensurePluginJarsIsMutable();
pluginJars_.addAll(other.pluginJars_);
}
onChanged();
}
} else {
if (!other.pluginJars_.isEmpty()) {
if (pluginJarsBuilder_.isEmpty()) {
pluginJarsBuilder_.dispose();
pluginJarsBuilder_ = null;
pluginJars_ = other.pluginJars_;
bitField0_ = (bitField0_ & ~0x00000001);
pluginJarsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getPluginJarsFieldBuilder() : null;
} else {
pluginJarsBuilder_.addAllMessages(other.pluginJars_);
}
}
}
if (kotlincPluginOptionsBuilder_ == null) {
if (!other.kotlincPluginOptions_.isEmpty()) {
if (kotlincPluginOptions_.isEmpty()) {
kotlincPluginOptions_ = other.kotlincPluginOptions_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureKotlincPluginOptionsIsMutable();
kotlincPluginOptions_.addAll(other.kotlincPluginOptions_);
}
onChanged();
}
} else {
if (!other.kotlincPluginOptions_.isEmpty()) {
if (kotlincPluginOptionsBuilder_.isEmpty()) {
kotlincPluginOptionsBuilder_.dispose();
kotlincPluginOptionsBuilder_ = null;
kotlincPluginOptions_ = other.kotlincPluginOptions_;
bitField0_ = (bitField0_ & ~0x00000002);
kotlincPluginOptionsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getKotlincPluginOptionsFieldBuilder() : null;
} else {
kotlincPluginOptionsBuilder_.addAllMessages(other.kotlincPluginOptions_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 802: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (pluginJarsBuilder_ == null) {
ensurePluginJarsIsMutable();
pluginJars_.add(m);
} else {
pluginJarsBuilder_.addMessage(m);
}
break;
} // case 802
case 1602: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.parser(),
extensionRegistry);
if (kotlincPluginOptionsBuilder_ == null) {
ensureKotlincPluginOptionsIsMutable();
kotlincPluginOptions_.add(m);
} else {
kotlincPluginOptionsBuilder_.addMessage(m);
}
break;
} // case 1602
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List pluginJars_ =
java.util.Collections.emptyList();
private void ensurePluginJarsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
pluginJars_ = new java.util.ArrayList(pluginJars_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> pluginJarsBuilder_;
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public java.util.List getPluginJarsList() {
if (pluginJarsBuilder_ == null) {
return java.util.Collections.unmodifiableList(pluginJars_);
} else {
return pluginJarsBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public int getPluginJarsCount() {
if (pluginJarsBuilder_ == null) {
return pluginJars_.size();
} else {
return pluginJarsBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getPluginJars(int index) {
if (pluginJarsBuilder_ == null) {
return pluginJars_.get(index);
} else {
return pluginJarsBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public Builder setPluginJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (pluginJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePluginJarsIsMutable();
pluginJars_.set(index, value);
onChanged();
} else {
pluginJarsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public Builder setPluginJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (pluginJarsBuilder_ == null) {
ensurePluginJarsIsMutable();
pluginJars_.set(index, builderForValue.build());
onChanged();
} else {
pluginJarsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public Builder addPluginJars(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (pluginJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePluginJarsIsMutable();
pluginJars_.add(value);
onChanged();
} else {
pluginJarsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public Builder addPluginJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (pluginJarsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePluginJarsIsMutable();
pluginJars_.add(index, value);
onChanged();
} else {
pluginJarsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public Builder addPluginJars(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (pluginJarsBuilder_ == null) {
ensurePluginJarsIsMutable();
pluginJars_.add(builderForValue.build());
onChanged();
} else {
pluginJarsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public Builder addPluginJars(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (pluginJarsBuilder_ == null) {
ensurePluginJarsIsMutable();
pluginJars_.add(index, builderForValue.build());
onChanged();
} else {
pluginJarsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public Builder addAllPluginJars(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (pluginJarsBuilder_ == null) {
ensurePluginJarsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, pluginJars_);
onChanged();
} else {
pluginJarsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public Builder clearPluginJars() {
if (pluginJarsBuilder_ == null) {
pluginJars_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
pluginJarsBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public Builder removePluginJars(int index) {
if (pluginJarsBuilder_ == null) {
ensurePluginJarsIsMutable();
pluginJars_.remove(index);
onChanged();
} else {
pluginJarsBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getPluginJarsBuilder(
int index) {
return getPluginJarsFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getPluginJarsOrBuilder(
int index) {
if (pluginJarsBuilder_ == null) {
return pluginJars_.get(index); } else {
return pluginJarsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getPluginJarsOrBuilderList() {
if (pluginJarsBuilder_ != null) {
return pluginJarsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(pluginJars_);
}
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addPluginJarsBuilder() {
return getPluginJarsFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addPluginJarsBuilder(
int index) {
return getPluginJarsFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation plugin_jars = 100;
*/
public java.util.List
getPluginJarsBuilderList() {
return getPluginJarsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getPluginJarsFieldBuilder() {
if (pluginJarsBuilder_ == null) {
pluginJarsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
pluginJars_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
pluginJars_ = null;
}
return pluginJarsBuilder_;
}
private java.util.List kotlincPluginOptions_ =
java.util.Collections.emptyList();
private void ensureKotlincPluginOptionsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
kotlincPluginOptions_ = new java.util.ArrayList(kotlincPluginOptions_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOptionOrBuilder> kotlincPluginOptionsBuilder_;
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public java.util.List getKotlincPluginOptionsList() {
if (kotlincPluginOptionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(kotlincPluginOptions_);
} else {
return kotlincPluginOptionsBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public int getKotlincPluginOptionsCount() {
if (kotlincPluginOptionsBuilder_ == null) {
return kotlincPluginOptions_.size();
} else {
return kotlincPluginOptionsBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption getKotlincPluginOptions(int index) {
if (kotlincPluginOptionsBuilder_ == null) {
return kotlincPluginOptions_.get(index);
} else {
return kotlincPluginOptionsBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public Builder setKotlincPluginOptions(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption value) {
if (kotlincPluginOptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKotlincPluginOptionsIsMutable();
kotlincPluginOptions_.set(index, value);
onChanged();
} else {
kotlincPluginOptionsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public Builder setKotlincPluginOptions(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.Builder builderForValue) {
if (kotlincPluginOptionsBuilder_ == null) {
ensureKotlincPluginOptionsIsMutable();
kotlincPluginOptions_.set(index, builderForValue.build());
onChanged();
} else {
kotlincPluginOptionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public Builder addKotlincPluginOptions(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption value) {
if (kotlincPluginOptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKotlincPluginOptionsIsMutable();
kotlincPluginOptions_.add(value);
onChanged();
} else {
kotlincPluginOptionsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public Builder addKotlincPluginOptions(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption value) {
if (kotlincPluginOptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKotlincPluginOptionsIsMutable();
kotlincPluginOptions_.add(index, value);
onChanged();
} else {
kotlincPluginOptionsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public Builder addKotlincPluginOptions(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.Builder builderForValue) {
if (kotlincPluginOptionsBuilder_ == null) {
ensureKotlincPluginOptionsIsMutable();
kotlincPluginOptions_.add(builderForValue.build());
onChanged();
} else {
kotlincPluginOptionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public Builder addKotlincPluginOptions(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.Builder builderForValue) {
if (kotlincPluginOptionsBuilder_ == null) {
ensureKotlincPluginOptionsIsMutable();
kotlincPluginOptions_.add(index, builderForValue.build());
onChanged();
} else {
kotlincPluginOptionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public Builder addAllKotlincPluginOptions(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption> values) {
if (kotlincPluginOptionsBuilder_ == null) {
ensureKotlincPluginOptionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, kotlincPluginOptions_);
onChanged();
} else {
kotlincPluginOptionsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public Builder clearKotlincPluginOptions() {
if (kotlincPluginOptionsBuilder_ == null) {
kotlincPluginOptions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
kotlincPluginOptionsBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public Builder removeKotlincPluginOptions(int index) {
if (kotlincPluginOptionsBuilder_ == null) {
ensureKotlincPluginOptionsIsMutable();
kotlincPluginOptions_.remove(index);
onChanged();
} else {
kotlincPluginOptionsBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.Builder getKotlincPluginOptionsBuilder(
int index) {
return getKotlincPluginOptionsFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOptionOrBuilder getKotlincPluginOptionsOrBuilder(
int index) {
if (kotlincPluginOptionsBuilder_ == null) {
return kotlincPluginOptions_.get(index); } else {
return kotlincPluginOptionsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOptionOrBuilder>
getKotlincPluginOptionsOrBuilderList() {
if (kotlincPluginOptionsBuilder_ != null) {
return kotlincPluginOptionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(kotlincPluginOptions_);
}
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.Builder addKotlincPluginOptionsBuilder() {
return getKotlincPluginOptionsFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.getDefaultInstance());
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.Builder addKotlincPluginOptionsBuilder(
int index) {
return getKotlincPluginOptionsFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.getDefaultInstance());
}
/**
* repeated .bazelbsp.KotlincPluginOption kotlinc_plugin_options = 200;
*/
public java.util.List
getKotlincPluginOptionsBuilderList() {
return getKotlincPluginOptionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOptionOrBuilder>
getKotlincPluginOptionsFieldBuilder() {
if (kotlincPluginOptionsBuilder_ == null) {
kotlincPluginOptionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOption.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginOptionOrBuilder>(
kotlincPluginOptions_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
kotlincPluginOptions_ = null;
}
return kotlincPluginOptionsBuilder_;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.KotlincPluginInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.KotlincPluginInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public KotlincPluginInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface KotlinTargetInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.KotlinTargetInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string language_version = 100;
* @return The languageVersion.
*/
java.lang.String getLanguageVersion();
/**
* string language_version = 100;
* @return The bytes for languageVersion.
*/
com.google.protobuf.ByteString
getLanguageVersionBytes();
/**
* string api_version = 200;
* @return The apiVersion.
*/
java.lang.String getApiVersion();
/**
* string api_version = 200;
* @return The bytes for apiVersion.
*/
com.google.protobuf.ByteString
getApiVersionBytes();
/**
* repeated string associates = 300;
* @return A list containing the associates.
*/
java.util.List
getAssociatesList();
/**
* repeated string associates = 300;
* @return The count of associates.
*/
int getAssociatesCount();
/**
* repeated string associates = 300;
* @param index The index of the element to return.
* @return The associates at the given index.
*/
java.lang.String getAssociates(int index);
/**
* repeated string associates = 300;
* @param index The index of the value to return.
* @return The bytes of the associates at the given index.
*/
com.google.protobuf.ByteString
getAssociatesBytes(int index);
/**
* repeated string kotlinc_opts = 400;
* @return A list containing the kotlincOpts.
*/
java.util.List
getKotlincOptsList();
/**
* repeated string kotlinc_opts = 400;
* @return The count of kotlincOpts.
*/
int getKotlincOptsCount();
/**
* repeated string kotlinc_opts = 400;
* @param index The index of the element to return.
* @return The kotlincOpts at the given index.
*/
java.lang.String getKotlincOpts(int index);
/**
* repeated string kotlinc_opts = 400;
* @param index The index of the value to return.
* @return The bytes of the kotlincOpts at the given index.
*/
com.google.protobuf.ByteString
getKotlincOptsBytes(int index);
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
java.util.List
getStdlibsList();
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getStdlibs(int index);
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
int getStdlibsCount();
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getStdlibsOrBuilderList();
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getStdlibsOrBuilder(
int index);
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
java.util.List
getKotlincPluginInfosList();
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo getKotlincPluginInfos(int index);
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
int getKotlincPluginInfosCount();
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfoOrBuilder>
getKotlincPluginInfosOrBuilderList();
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfoOrBuilder getKotlincPluginInfosOrBuilder(
int index);
}
/**
* Protobuf type {@code bazelbsp.KotlinTargetInfo}
*/
public static final class KotlinTargetInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.KotlinTargetInfo)
KotlinTargetInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
KotlinTargetInfo.class.getName());
}
// Use KotlinTargetInfo.newBuilder() to construct.
private KotlinTargetInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private KotlinTargetInfo() {
languageVersion_ = "";
apiVersion_ = "";
associates_ =
com.google.protobuf.LazyStringArrayList.emptyList();
kotlincOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
stdlibs_ = java.util.Collections.emptyList();
kotlincPluginInfos_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlinTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlinTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo.Builder.class);
}
public static final int LANGUAGE_VERSION_FIELD_NUMBER = 100;
@SuppressWarnings("serial")
private volatile java.lang.Object languageVersion_ = "";
/**
* string language_version = 100;
* @return The languageVersion.
*/
@java.lang.Override
public java.lang.String getLanguageVersion() {
java.lang.Object ref = languageVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
languageVersion_ = s;
return s;
}
}
/**
* string language_version = 100;
* @return The bytes for languageVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLanguageVersionBytes() {
java.lang.Object ref = languageVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
languageVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int API_VERSION_FIELD_NUMBER = 200;
@SuppressWarnings("serial")
private volatile java.lang.Object apiVersion_ = "";
/**
* string api_version = 200;
* @return The apiVersion.
*/
@java.lang.Override
public java.lang.String getApiVersion() {
java.lang.Object ref = apiVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
apiVersion_ = s;
return s;
}
}
/**
* string api_version = 200;
* @return The bytes for apiVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getApiVersionBytes() {
java.lang.Object ref = apiVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
apiVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ASSOCIATES_FIELD_NUMBER = 300;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList associates_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string associates = 300;
* @return A list containing the associates.
*/
public com.google.protobuf.ProtocolStringList
getAssociatesList() {
return associates_;
}
/**
* repeated string associates = 300;
* @return The count of associates.
*/
public int getAssociatesCount() {
return associates_.size();
}
/**
* repeated string associates = 300;
* @param index The index of the element to return.
* @return The associates at the given index.
*/
public java.lang.String getAssociates(int index) {
return associates_.get(index);
}
/**
* repeated string associates = 300;
* @param index The index of the value to return.
* @return The bytes of the associates at the given index.
*/
public com.google.protobuf.ByteString
getAssociatesBytes(int index) {
return associates_.getByteString(index);
}
public static final int KOTLINC_OPTS_FIELD_NUMBER = 400;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList kotlincOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string kotlinc_opts = 400;
* @return A list containing the kotlincOpts.
*/
public com.google.protobuf.ProtocolStringList
getKotlincOptsList() {
return kotlincOpts_;
}
/**
* repeated string kotlinc_opts = 400;
* @return The count of kotlincOpts.
*/
public int getKotlincOptsCount() {
return kotlincOpts_.size();
}
/**
* repeated string kotlinc_opts = 400;
* @param index The index of the element to return.
* @return The kotlincOpts at the given index.
*/
public java.lang.String getKotlincOpts(int index) {
return kotlincOpts_.get(index);
}
/**
* repeated string kotlinc_opts = 400;
* @param index The index of the value to return.
* @return The bytes of the kotlincOpts at the given index.
*/
public com.google.protobuf.ByteString
getKotlincOptsBytes(int index) {
return kotlincOpts_.getByteString(index);
}
public static final int STDLIBS_FIELD_NUMBER = 500;
@SuppressWarnings("serial")
private java.util.List stdlibs_;
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
@java.lang.Override
public java.util.List getStdlibsList() {
return stdlibs_;
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getStdlibsOrBuilderList() {
return stdlibs_;
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
@java.lang.Override
public int getStdlibsCount() {
return stdlibs_.size();
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getStdlibs(int index) {
return stdlibs_.get(index);
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getStdlibsOrBuilder(
int index) {
return stdlibs_.get(index);
}
public static final int KOTLINC_PLUGIN_INFOS_FIELD_NUMBER = 600;
@SuppressWarnings("serial")
private java.util.List kotlincPluginInfos_;
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
@java.lang.Override
public java.util.List getKotlincPluginInfosList() {
return kotlincPluginInfos_;
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfoOrBuilder>
getKotlincPluginInfosOrBuilderList() {
return kotlincPluginInfos_;
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
@java.lang.Override
public int getKotlincPluginInfosCount() {
return kotlincPluginInfos_.size();
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo getKotlincPluginInfos(int index) {
return kotlincPluginInfos_.get(index);
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfoOrBuilder getKotlincPluginInfosOrBuilder(
int index) {
return kotlincPluginInfos_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(languageVersion_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 100, languageVersion_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(apiVersion_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 200, apiVersion_);
}
for (int i = 0; i < associates_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 300, associates_.getRaw(i));
}
for (int i = 0; i < kotlincOpts_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 400, kotlincOpts_.getRaw(i));
}
for (int i = 0; i < stdlibs_.size(); i++) {
output.writeMessage(500, stdlibs_.get(i));
}
for (int i = 0; i < kotlincPluginInfos_.size(); i++) {
output.writeMessage(600, kotlincPluginInfos_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(languageVersion_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(100, languageVersion_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(apiVersion_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(200, apiVersion_);
}
{
int dataSize = 0;
for (int i = 0; i < associates_.size(); i++) {
dataSize += computeStringSizeNoTag(associates_.getRaw(i));
}
size += dataSize;
size += 2 * getAssociatesList().size();
}
{
int dataSize = 0;
for (int i = 0; i < kotlincOpts_.size(); i++) {
dataSize += computeStringSizeNoTag(kotlincOpts_.getRaw(i));
}
size += dataSize;
size += 2 * getKotlincOptsList().size();
}
for (int i = 0; i < stdlibs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(500, stdlibs_.get(i));
}
for (int i = 0; i < kotlincPluginInfos_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(600, kotlincPluginInfos_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo) obj;
if (!getLanguageVersion()
.equals(other.getLanguageVersion())) return false;
if (!getApiVersion()
.equals(other.getApiVersion())) return false;
if (!getAssociatesList()
.equals(other.getAssociatesList())) return false;
if (!getKotlincOptsList()
.equals(other.getKotlincOptsList())) return false;
if (!getStdlibsList()
.equals(other.getStdlibsList())) return false;
if (!getKotlincPluginInfosList()
.equals(other.getKotlincPluginInfosList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + LANGUAGE_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getLanguageVersion().hashCode();
hash = (37 * hash) + API_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getApiVersion().hashCode();
if (getAssociatesCount() > 0) {
hash = (37 * hash) + ASSOCIATES_FIELD_NUMBER;
hash = (53 * hash) + getAssociatesList().hashCode();
}
if (getKotlincOptsCount() > 0) {
hash = (37 * hash) + KOTLINC_OPTS_FIELD_NUMBER;
hash = (53 * hash) + getKotlincOptsList().hashCode();
}
if (getStdlibsCount() > 0) {
hash = (37 * hash) + STDLIBS_FIELD_NUMBER;
hash = (53 * hash) + getStdlibsList().hashCode();
}
if (getKotlincPluginInfosCount() > 0) {
hash = (37 * hash) + KOTLINC_PLUGIN_INFOS_FIELD_NUMBER;
hash = (53 * hash) + getKotlincPluginInfosList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.KotlinTargetInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.KotlinTargetInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlinTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlinTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
languageVersion_ = "";
apiVersion_ = "";
associates_ =
com.google.protobuf.LazyStringArrayList.emptyList();
kotlincOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
if (stdlibsBuilder_ == null) {
stdlibs_ = java.util.Collections.emptyList();
} else {
stdlibs_ = null;
stdlibsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (kotlincPluginInfosBuilder_ == null) {
kotlincPluginInfos_ = java.util.Collections.emptyList();
} else {
kotlincPluginInfos_ = null;
kotlincPluginInfosBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_KotlinTargetInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo result) {
if (stdlibsBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
stdlibs_ = java.util.Collections.unmodifiableList(stdlibs_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.stdlibs_ = stdlibs_;
} else {
result.stdlibs_ = stdlibsBuilder_.build();
}
if (kotlincPluginInfosBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
kotlincPluginInfos_ = java.util.Collections.unmodifiableList(kotlincPluginInfos_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.kotlincPluginInfos_ = kotlincPluginInfos_;
} else {
result.kotlincPluginInfos_ = kotlincPluginInfosBuilder_.build();
}
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.languageVersion_ = languageVersion_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.apiVersion_ = apiVersion_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
associates_.makeImmutable();
result.associates_ = associates_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
kotlincOpts_.makeImmutable();
result.kotlincOpts_ = kotlincOpts_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo.getDefaultInstance()) return this;
if (!other.getLanguageVersion().isEmpty()) {
languageVersion_ = other.languageVersion_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getApiVersion().isEmpty()) {
apiVersion_ = other.apiVersion_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.associates_.isEmpty()) {
if (associates_.isEmpty()) {
associates_ = other.associates_;
bitField0_ |= 0x00000004;
} else {
ensureAssociatesIsMutable();
associates_.addAll(other.associates_);
}
onChanged();
}
if (!other.kotlincOpts_.isEmpty()) {
if (kotlincOpts_.isEmpty()) {
kotlincOpts_ = other.kotlincOpts_;
bitField0_ |= 0x00000008;
} else {
ensureKotlincOptsIsMutable();
kotlincOpts_.addAll(other.kotlincOpts_);
}
onChanged();
}
if (stdlibsBuilder_ == null) {
if (!other.stdlibs_.isEmpty()) {
if (stdlibs_.isEmpty()) {
stdlibs_ = other.stdlibs_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureStdlibsIsMutable();
stdlibs_.addAll(other.stdlibs_);
}
onChanged();
}
} else {
if (!other.stdlibs_.isEmpty()) {
if (stdlibsBuilder_.isEmpty()) {
stdlibsBuilder_.dispose();
stdlibsBuilder_ = null;
stdlibs_ = other.stdlibs_;
bitField0_ = (bitField0_ & ~0x00000010);
stdlibsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getStdlibsFieldBuilder() : null;
} else {
stdlibsBuilder_.addAllMessages(other.stdlibs_);
}
}
}
if (kotlincPluginInfosBuilder_ == null) {
if (!other.kotlincPluginInfos_.isEmpty()) {
if (kotlincPluginInfos_.isEmpty()) {
kotlincPluginInfos_ = other.kotlincPluginInfos_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureKotlincPluginInfosIsMutable();
kotlincPluginInfos_.addAll(other.kotlincPluginInfos_);
}
onChanged();
}
} else {
if (!other.kotlincPluginInfos_.isEmpty()) {
if (kotlincPluginInfosBuilder_.isEmpty()) {
kotlincPluginInfosBuilder_.dispose();
kotlincPluginInfosBuilder_ = null;
kotlincPluginInfos_ = other.kotlincPluginInfos_;
bitField0_ = (bitField0_ & ~0x00000020);
kotlincPluginInfosBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getKotlincPluginInfosFieldBuilder() : null;
} else {
kotlincPluginInfosBuilder_.addAllMessages(other.kotlincPluginInfos_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 802: {
languageVersion_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 802
case 1602: {
apiVersion_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 1602
case 2402: {
java.lang.String s = input.readStringRequireUtf8();
ensureAssociatesIsMutable();
associates_.add(s);
break;
} // case 2402
case 3202: {
java.lang.String s = input.readStringRequireUtf8();
ensureKotlincOptsIsMutable();
kotlincOpts_.add(s);
break;
} // case 3202
case 4002: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (stdlibsBuilder_ == null) {
ensureStdlibsIsMutable();
stdlibs_.add(m);
} else {
stdlibsBuilder_.addMessage(m);
}
break;
} // case 4002
case 4802: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.parser(),
extensionRegistry);
if (kotlincPluginInfosBuilder_ == null) {
ensureKotlincPluginInfosIsMutable();
kotlincPluginInfos_.add(m);
} else {
kotlincPluginInfosBuilder_.addMessage(m);
}
break;
} // case 4802
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object languageVersion_ = "";
/**
* string language_version = 100;
* @return The languageVersion.
*/
public java.lang.String getLanguageVersion() {
java.lang.Object ref = languageVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
languageVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string language_version = 100;
* @return The bytes for languageVersion.
*/
public com.google.protobuf.ByteString
getLanguageVersionBytes() {
java.lang.Object ref = languageVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
languageVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string language_version = 100;
* @param value The languageVersion to set.
* @return This builder for chaining.
*/
public Builder setLanguageVersion(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
languageVersion_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string language_version = 100;
* @return This builder for chaining.
*/
public Builder clearLanguageVersion() {
languageVersion_ = getDefaultInstance().getLanguageVersion();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string language_version = 100;
* @param value The bytes for languageVersion to set.
* @return This builder for chaining.
*/
public Builder setLanguageVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
languageVersion_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object apiVersion_ = "";
/**
* string api_version = 200;
* @return The apiVersion.
*/
public java.lang.String getApiVersion() {
java.lang.Object ref = apiVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
apiVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string api_version = 200;
* @return The bytes for apiVersion.
*/
public com.google.protobuf.ByteString
getApiVersionBytes() {
java.lang.Object ref = apiVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
apiVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string api_version = 200;
* @param value The apiVersion to set.
* @return This builder for chaining.
*/
public Builder setApiVersion(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
apiVersion_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string api_version = 200;
* @return This builder for chaining.
*/
public Builder clearApiVersion() {
apiVersion_ = getDefaultInstance().getApiVersion();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string api_version = 200;
* @param value The bytes for apiVersion to set.
* @return This builder for chaining.
*/
public Builder setApiVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
apiVersion_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList associates_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureAssociatesIsMutable() {
if (!associates_.isModifiable()) {
associates_ = new com.google.protobuf.LazyStringArrayList(associates_);
}
bitField0_ |= 0x00000004;
}
/**
* repeated string associates = 300;
* @return A list containing the associates.
*/
public com.google.protobuf.ProtocolStringList
getAssociatesList() {
associates_.makeImmutable();
return associates_;
}
/**
* repeated string associates = 300;
* @return The count of associates.
*/
public int getAssociatesCount() {
return associates_.size();
}
/**
* repeated string associates = 300;
* @param index The index of the element to return.
* @return The associates at the given index.
*/
public java.lang.String getAssociates(int index) {
return associates_.get(index);
}
/**
* repeated string associates = 300;
* @param index The index of the value to return.
* @return The bytes of the associates at the given index.
*/
public com.google.protobuf.ByteString
getAssociatesBytes(int index) {
return associates_.getByteString(index);
}
/**
* repeated string associates = 300;
* @param index The index to set the value at.
* @param value The associates to set.
* @return This builder for chaining.
*/
public Builder setAssociates(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureAssociatesIsMutable();
associates_.set(index, value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* repeated string associates = 300;
* @param value The associates to add.
* @return This builder for chaining.
*/
public Builder addAssociates(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureAssociatesIsMutable();
associates_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* repeated string associates = 300;
* @param values The associates to add.
* @return This builder for chaining.
*/
public Builder addAllAssociates(
java.lang.Iterable values) {
ensureAssociatesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, associates_);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* repeated string associates = 300;
* @return This builder for chaining.
*/
public Builder clearAssociates() {
associates_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);;
onChanged();
return this;
}
/**
* repeated string associates = 300;
* @param value The bytes of the associates to add.
* @return This builder for chaining.
*/
public Builder addAssociatesBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureAssociatesIsMutable();
associates_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList kotlincOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureKotlincOptsIsMutable() {
if (!kotlincOpts_.isModifiable()) {
kotlincOpts_ = new com.google.protobuf.LazyStringArrayList(kotlincOpts_);
}
bitField0_ |= 0x00000008;
}
/**
* repeated string kotlinc_opts = 400;
* @return A list containing the kotlincOpts.
*/
public com.google.protobuf.ProtocolStringList
getKotlincOptsList() {
kotlincOpts_.makeImmutable();
return kotlincOpts_;
}
/**
* repeated string kotlinc_opts = 400;
* @return The count of kotlincOpts.
*/
public int getKotlincOptsCount() {
return kotlincOpts_.size();
}
/**
* repeated string kotlinc_opts = 400;
* @param index The index of the element to return.
* @return The kotlincOpts at the given index.
*/
public java.lang.String getKotlincOpts(int index) {
return kotlincOpts_.get(index);
}
/**
* repeated string kotlinc_opts = 400;
* @param index The index of the value to return.
* @return The bytes of the kotlincOpts at the given index.
*/
public com.google.protobuf.ByteString
getKotlincOptsBytes(int index) {
return kotlincOpts_.getByteString(index);
}
/**
* repeated string kotlinc_opts = 400;
* @param index The index to set the value at.
* @param value The kotlincOpts to set.
* @return This builder for chaining.
*/
public Builder setKotlincOpts(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureKotlincOptsIsMutable();
kotlincOpts_.set(index, value);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* repeated string kotlinc_opts = 400;
* @param value The kotlincOpts to add.
* @return This builder for chaining.
*/
public Builder addKotlincOpts(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureKotlincOptsIsMutable();
kotlincOpts_.add(value);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* repeated string kotlinc_opts = 400;
* @param values The kotlincOpts to add.
* @return This builder for chaining.
*/
public Builder addAllKotlincOpts(
java.lang.Iterable values) {
ensureKotlincOptsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, kotlincOpts_);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* repeated string kotlinc_opts = 400;
* @return This builder for chaining.
*/
public Builder clearKotlincOpts() {
kotlincOpts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);;
onChanged();
return this;
}
/**
* repeated string kotlinc_opts = 400;
* @param value The bytes of the kotlincOpts to add.
* @return This builder for chaining.
*/
public Builder addKotlincOptsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureKotlincOptsIsMutable();
kotlincOpts_.add(value);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.util.List stdlibs_ =
java.util.Collections.emptyList();
private void ensureStdlibsIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
stdlibs_ = new java.util.ArrayList(stdlibs_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> stdlibsBuilder_;
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public java.util.List getStdlibsList() {
if (stdlibsBuilder_ == null) {
return java.util.Collections.unmodifiableList(stdlibs_);
} else {
return stdlibsBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public int getStdlibsCount() {
if (stdlibsBuilder_ == null) {
return stdlibs_.size();
} else {
return stdlibsBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getStdlibs(int index) {
if (stdlibsBuilder_ == null) {
return stdlibs_.get(index);
} else {
return stdlibsBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public Builder setStdlibs(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (stdlibsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStdlibsIsMutable();
stdlibs_.set(index, value);
onChanged();
} else {
stdlibsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public Builder setStdlibs(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (stdlibsBuilder_ == null) {
ensureStdlibsIsMutable();
stdlibs_.set(index, builderForValue.build());
onChanged();
} else {
stdlibsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public Builder addStdlibs(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (stdlibsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStdlibsIsMutable();
stdlibs_.add(value);
onChanged();
} else {
stdlibsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public Builder addStdlibs(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (stdlibsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStdlibsIsMutable();
stdlibs_.add(index, value);
onChanged();
} else {
stdlibsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public Builder addStdlibs(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (stdlibsBuilder_ == null) {
ensureStdlibsIsMutable();
stdlibs_.add(builderForValue.build());
onChanged();
} else {
stdlibsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public Builder addStdlibs(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (stdlibsBuilder_ == null) {
ensureStdlibsIsMutable();
stdlibs_.add(index, builderForValue.build());
onChanged();
} else {
stdlibsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public Builder addAllStdlibs(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (stdlibsBuilder_ == null) {
ensureStdlibsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, stdlibs_);
onChanged();
} else {
stdlibsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public Builder clearStdlibs() {
if (stdlibsBuilder_ == null) {
stdlibs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
stdlibsBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public Builder removeStdlibs(int index) {
if (stdlibsBuilder_ == null) {
ensureStdlibsIsMutable();
stdlibs_.remove(index);
onChanged();
} else {
stdlibsBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getStdlibsBuilder(
int index) {
return getStdlibsFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getStdlibsOrBuilder(
int index) {
if (stdlibsBuilder_ == null) {
return stdlibs_.get(index); } else {
return stdlibsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getStdlibsOrBuilderList() {
if (stdlibsBuilder_ != null) {
return stdlibsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(stdlibs_);
}
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addStdlibsBuilder() {
return getStdlibsFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addStdlibsBuilder(
int index) {
return getStdlibsFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation stdlibs = 500;
*/
public java.util.List
getStdlibsBuilderList() {
return getStdlibsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getStdlibsFieldBuilder() {
if (stdlibsBuilder_ == null) {
stdlibsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
stdlibs_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
stdlibs_ = null;
}
return stdlibsBuilder_;
}
private java.util.List kotlincPluginInfos_ =
java.util.Collections.emptyList();
private void ensureKotlincPluginInfosIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
kotlincPluginInfos_ = new java.util.ArrayList(kotlincPluginInfos_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfoOrBuilder> kotlincPluginInfosBuilder_;
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public java.util.List getKotlincPluginInfosList() {
if (kotlincPluginInfosBuilder_ == null) {
return java.util.Collections.unmodifiableList(kotlincPluginInfos_);
} else {
return kotlincPluginInfosBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public int getKotlincPluginInfosCount() {
if (kotlincPluginInfosBuilder_ == null) {
return kotlincPluginInfos_.size();
} else {
return kotlincPluginInfosBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo getKotlincPluginInfos(int index) {
if (kotlincPluginInfosBuilder_ == null) {
return kotlincPluginInfos_.get(index);
} else {
return kotlincPluginInfosBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public Builder setKotlincPluginInfos(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo value) {
if (kotlincPluginInfosBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKotlincPluginInfosIsMutable();
kotlincPluginInfos_.set(index, value);
onChanged();
} else {
kotlincPluginInfosBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public Builder setKotlincPluginInfos(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.Builder builderForValue) {
if (kotlincPluginInfosBuilder_ == null) {
ensureKotlincPluginInfosIsMutable();
kotlincPluginInfos_.set(index, builderForValue.build());
onChanged();
} else {
kotlincPluginInfosBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public Builder addKotlincPluginInfos(org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo value) {
if (kotlincPluginInfosBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKotlincPluginInfosIsMutable();
kotlincPluginInfos_.add(value);
onChanged();
} else {
kotlincPluginInfosBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public Builder addKotlincPluginInfos(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo value) {
if (kotlincPluginInfosBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKotlincPluginInfosIsMutable();
kotlincPluginInfos_.add(index, value);
onChanged();
} else {
kotlincPluginInfosBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public Builder addKotlincPluginInfos(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.Builder builderForValue) {
if (kotlincPluginInfosBuilder_ == null) {
ensureKotlincPluginInfosIsMutable();
kotlincPluginInfos_.add(builderForValue.build());
onChanged();
} else {
kotlincPluginInfosBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public Builder addKotlincPluginInfos(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.Builder builderForValue) {
if (kotlincPluginInfosBuilder_ == null) {
ensureKotlincPluginInfosIsMutable();
kotlincPluginInfos_.add(index, builderForValue.build());
onChanged();
} else {
kotlincPluginInfosBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public Builder addAllKotlincPluginInfos(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo> values) {
if (kotlincPluginInfosBuilder_ == null) {
ensureKotlincPluginInfosIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, kotlincPluginInfos_);
onChanged();
} else {
kotlincPluginInfosBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public Builder clearKotlincPluginInfos() {
if (kotlincPluginInfosBuilder_ == null) {
kotlincPluginInfos_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
kotlincPluginInfosBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public Builder removeKotlincPluginInfos(int index) {
if (kotlincPluginInfosBuilder_ == null) {
ensureKotlincPluginInfosIsMutable();
kotlincPluginInfos_.remove(index);
onChanged();
} else {
kotlincPluginInfosBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.Builder getKotlincPluginInfosBuilder(
int index) {
return getKotlincPluginInfosFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfoOrBuilder getKotlincPluginInfosOrBuilder(
int index) {
if (kotlincPluginInfosBuilder_ == null) {
return kotlincPluginInfos_.get(index); } else {
return kotlincPluginInfosBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfoOrBuilder>
getKotlincPluginInfosOrBuilderList() {
if (kotlincPluginInfosBuilder_ != null) {
return kotlincPluginInfosBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(kotlincPluginInfos_);
}
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.Builder addKotlincPluginInfosBuilder() {
return getKotlincPluginInfosFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.getDefaultInstance());
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.Builder addKotlincPluginInfosBuilder(
int index) {
return getKotlincPluginInfosFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.getDefaultInstance());
}
/**
* repeated .bazelbsp.KotlincPluginInfo kotlinc_plugin_infos = 600;
*/
public java.util.List
getKotlincPluginInfosBuilderList() {
return getKotlincPluginInfosFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfoOrBuilder>
getKotlincPluginInfosFieldBuilder() {
if (kotlincPluginInfosBuilder_ == null) {
kotlincPluginInfosBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfo.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlincPluginInfoOrBuilder>(
kotlincPluginInfos_,
((bitField0_ & 0x00000020) != 0),
getParentForChildren(),
isClean());
kotlincPluginInfos_ = null;
}
return kotlincPluginInfosBuilder_;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.KotlinTargetInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.KotlinTargetInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public KotlinTargetInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.KotlinTargetInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PythonTargetInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.PythonTargetInfo)
com.google.protobuf.MessageOrBuilder {
/**
* .bazelbsp.FileLocation interpreter = 1;
* @return Whether the interpreter field is set.
*/
boolean hasInterpreter();
/**
* .bazelbsp.FileLocation interpreter = 1;
* @return The interpreter.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getInterpreter();
/**
* .bazelbsp.FileLocation interpreter = 1;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getInterpreterOrBuilder();
/**
* string version = 2;
* @return The version.
*/
java.lang.String getVersion();
/**
* string version = 2;
* @return The bytes for version.
*/
com.google.protobuf.ByteString
getVersionBytes();
}
/**
* Protobuf type {@code bazelbsp.PythonTargetInfo}
*/
public static final class PythonTargetInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.PythonTargetInfo)
PythonTargetInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
PythonTargetInfo.class.getName());
}
// Use PythonTargetInfo.newBuilder() to construct.
private PythonTargetInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private PythonTargetInfo() {
version_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_PythonTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_PythonTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo.Builder.class);
}
private int bitField0_;
public static final int INTERPRETER_FIELD_NUMBER = 1;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation interpreter_;
/**
* .bazelbsp.FileLocation interpreter = 1;
* @return Whether the interpreter field is set.
*/
@java.lang.Override
public boolean hasInterpreter() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .bazelbsp.FileLocation interpreter = 1;
* @return The interpreter.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getInterpreter() {
return interpreter_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : interpreter_;
}
/**
* .bazelbsp.FileLocation interpreter = 1;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getInterpreterOrBuilder() {
return interpreter_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : interpreter_;
}
public static final int VERSION_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object version_ = "";
/**
* string version = 2;
* @return The version.
*/
@java.lang.Override
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
}
}
/**
* string version = 2;
* @return The bytes for version.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getInterpreter());
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(version_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, version_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getInterpreter());
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(version_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, version_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo) obj;
if (hasInterpreter() != other.hasInterpreter()) return false;
if (hasInterpreter()) {
if (!getInterpreter()
.equals(other.getInterpreter())) return false;
}
if (!getVersion()
.equals(other.getVersion())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInterpreter()) {
hash = (37 * hash) + INTERPRETER_FIELD_NUMBER;
hash = (53 * hash) + getInterpreter().hashCode();
}
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.PythonTargetInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.PythonTargetInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_PythonTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_PythonTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage
.alwaysUseFieldBuilders) {
getInterpreterFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
interpreter_ = null;
if (interpreterBuilder_ != null) {
interpreterBuilder_.dispose();
interpreterBuilder_ = null;
}
version_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_PythonTargetInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.interpreter_ = interpreterBuilder_ == null
? interpreter_
: interpreterBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.version_ = version_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo.getDefaultInstance()) return this;
if (other.hasInterpreter()) {
mergeInterpreter(other.getInterpreter());
}
if (!other.getVersion().isEmpty()) {
version_ = other.version_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getInterpreterFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
version_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation interpreter_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> interpreterBuilder_;
/**
* .bazelbsp.FileLocation interpreter = 1;
* @return Whether the interpreter field is set.
*/
public boolean hasInterpreter() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .bazelbsp.FileLocation interpreter = 1;
* @return The interpreter.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getInterpreter() {
if (interpreterBuilder_ == null) {
return interpreter_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : interpreter_;
} else {
return interpreterBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation interpreter = 1;
*/
public Builder setInterpreter(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (interpreterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
interpreter_ = value;
} else {
interpreterBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation interpreter = 1;
*/
public Builder setInterpreter(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (interpreterBuilder_ == null) {
interpreter_ = builderForValue.build();
} else {
interpreterBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation interpreter = 1;
*/
public Builder mergeInterpreter(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (interpreterBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
interpreter_ != null &&
interpreter_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getInterpreterBuilder().mergeFrom(value);
} else {
interpreter_ = value;
}
} else {
interpreterBuilder_.mergeFrom(value);
}
if (interpreter_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation interpreter = 1;
*/
public Builder clearInterpreter() {
bitField0_ = (bitField0_ & ~0x00000001);
interpreter_ = null;
if (interpreterBuilder_ != null) {
interpreterBuilder_.dispose();
interpreterBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation interpreter = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getInterpreterBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getInterpreterFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation interpreter = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getInterpreterOrBuilder() {
if (interpreterBuilder_ != null) {
return interpreterBuilder_.getMessageOrBuilder();
} else {
return interpreter_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : interpreter_;
}
}
/**
* .bazelbsp.FileLocation interpreter = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getInterpreterFieldBuilder() {
if (interpreterBuilder_ == null) {
interpreterBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getInterpreter(),
getParentForChildren(),
isClean());
interpreter_ = null;
}
return interpreterBuilder_;
}
private java.lang.Object version_ = "";
/**
* string version = 2;
* @return The version.
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string version = 2;
* @return The bytes for version.
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string version = 2;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
version_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string version = 2;
* @return This builder for chaining.
*/
public Builder clearVersion() {
version_ = getDefaultInstance().getVersion();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string version = 2;
* @param value The bytes for version to set.
* @return This builder for chaining.
*/
public Builder setVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
version_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.PythonTargetInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.PythonTargetInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PythonTargetInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.PythonTargetInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RustCrateInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.RustCrateInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string crate_id = 1;
* @return The crateId.
*/
java.lang.String getCrateId();
/**
* string crate_id = 1;
* @return The bytes for crateId.
*/
com.google.protobuf.ByteString
getCrateIdBytes();
/**
* .bazelbsp.RustCrateLocation location = 2;
* @return The enum numeric value on the wire for location.
*/
int getLocationValue();
/**
* .bazelbsp.RustCrateLocation location = 2;
* @return The location.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation getLocation();
/**
* bool from_workspace = 3;
* @return The fromWorkspace.
*/
boolean getFromWorkspace();
/**
* string name = 4;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 4;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* string kind = 5;
* @return The kind.
*/
java.lang.String getKind();
/**
* string kind = 5;
* @return The bytes for kind.
*/
com.google.protobuf.ByteString
getKindBytes();
/**
* string edition = 6;
* @return The edition.
*/
java.lang.String getEdition();
/**
* string edition = 6;
* @return The bytes for edition.
*/
com.google.protobuf.ByteString
getEditionBytes();
/**
* string out_dir = 7;
* @return The outDir.
*/
java.lang.String getOutDir();
/**
* string out_dir = 7;
* @return The bytes for outDir.
*/
com.google.protobuf.ByteString
getOutDirBytes();
/**
* repeated string crate_features = 8;
* @return A list containing the crateFeatures.
*/
java.util.List
getCrateFeaturesList();
/**
* repeated string crate_features = 8;
* @return The count of crateFeatures.
*/
int getCrateFeaturesCount();
/**
* repeated string crate_features = 8;
* @param index The index of the element to return.
* @return The crateFeatures at the given index.
*/
java.lang.String getCrateFeatures(int index);
/**
* repeated string crate_features = 8;
* @param index The index of the value to return.
* @return The bytes of the crateFeatures at the given index.
*/
com.google.protobuf.ByteString
getCrateFeaturesBytes(int index);
/**
* repeated string dependencies_crate_ids = 9;
* @return A list containing the dependenciesCrateIds.
*/
java.util.List
getDependenciesCrateIdsList();
/**
* repeated string dependencies_crate_ids = 9;
* @return The count of dependenciesCrateIds.
*/
int getDependenciesCrateIdsCount();
/**
* repeated string dependencies_crate_ids = 9;
* @param index The index of the element to return.
* @return The dependenciesCrateIds at the given index.
*/
java.lang.String getDependenciesCrateIds(int index);
/**
* repeated string dependencies_crate_ids = 9;
* @param index The index of the value to return.
* @return The bytes of the dependenciesCrateIds at the given index.
*/
com.google.protobuf.ByteString
getDependenciesCrateIdsBytes(int index);
/**
* string crate_root = 10;
* @return The crateRoot.
*/
java.lang.String getCrateRoot();
/**
* string crate_root = 10;
* @return The bytes for crateRoot.
*/
com.google.protobuf.ByteString
getCrateRootBytes();
/**
* string version = 11;
* @return The version.
*/
java.lang.String getVersion();
/**
* string version = 11;
* @return The bytes for version.
*/
com.google.protobuf.ByteString
getVersionBytes();
/**
* repeated string proc_macro_artifacts = 12;
* @return A list containing the procMacroArtifacts.
*/
java.util.List
getProcMacroArtifactsList();
/**
* repeated string proc_macro_artifacts = 12;
* @return The count of procMacroArtifacts.
*/
int getProcMacroArtifactsCount();
/**
* repeated string proc_macro_artifacts = 12;
* @param index The index of the element to return.
* @return The procMacroArtifacts at the given index.
*/
java.lang.String getProcMacroArtifacts(int index);
/**
* repeated string proc_macro_artifacts = 12;
* @param index The index of the value to return.
* @return The bytes of the procMacroArtifacts at the given index.
*/
com.google.protobuf.ByteString
getProcMacroArtifactsBytes(int index);
}
/**
* Protobuf type {@code bazelbsp.RustCrateInfo}
*/
public static final class RustCrateInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.RustCrateInfo)
RustCrateInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
RustCrateInfo.class.getName());
}
// Use RustCrateInfo.newBuilder() to construct.
private RustCrateInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private RustCrateInfo() {
crateId_ = "";
location_ = 0;
name_ = "";
kind_ = "";
edition_ = "";
outDir_ = "";
crateFeatures_ =
com.google.protobuf.LazyStringArrayList.emptyList();
dependenciesCrateIds_ =
com.google.protobuf.LazyStringArrayList.emptyList();
crateRoot_ = "";
version_ = "";
procMacroArtifacts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_RustCrateInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_RustCrateInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo.Builder.class);
}
public static final int CRATE_ID_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object crateId_ = "";
/**
* string crate_id = 1;
* @return The crateId.
*/
@java.lang.Override
public java.lang.String getCrateId() {
java.lang.Object ref = crateId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
crateId_ = s;
return s;
}
}
/**
* string crate_id = 1;
* @return The bytes for crateId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCrateIdBytes() {
java.lang.Object ref = crateId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
crateId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOCATION_FIELD_NUMBER = 2;
private int location_ = 0;
/**
* .bazelbsp.RustCrateLocation location = 2;
* @return The enum numeric value on the wire for location.
*/
@java.lang.Override public int getLocationValue() {
return location_;
}
/**
* .bazelbsp.RustCrateLocation location = 2;
* @return The location.
*/
@java.lang.Override public org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation getLocation() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation result = org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation.forNumber(location_);
return result == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation.UNRECOGNIZED : result;
}
public static final int FROM_WORKSPACE_FIELD_NUMBER = 3;
private boolean fromWorkspace_ = false;
/**
* bool from_workspace = 3;
* @return The fromWorkspace.
*/
@java.lang.Override
public boolean getFromWorkspace() {
return fromWorkspace_;
}
public static final int NAME_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
* string name = 4;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 4;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KIND_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object kind_ = "";
/**
* string kind = 5;
* @return The kind.
*/
@java.lang.Override
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
}
}
/**
* string kind = 5;
* @return The bytes for kind.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EDITION_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private volatile java.lang.Object edition_ = "";
/**
* string edition = 6;
* @return The edition.
*/
@java.lang.Override
public java.lang.String getEdition() {
java.lang.Object ref = edition_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
edition_ = s;
return s;
}
}
/**
* string edition = 6;
* @return The bytes for edition.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getEditionBytes() {
java.lang.Object ref = edition_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
edition_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OUT_DIR_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object outDir_ = "";
/**
* string out_dir = 7;
* @return The outDir.
*/
@java.lang.Override
public java.lang.String getOutDir() {
java.lang.Object ref = outDir_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
outDir_ = s;
return s;
}
}
/**
* string out_dir = 7;
* @return The bytes for outDir.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOutDirBytes() {
java.lang.Object ref = outDir_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outDir_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CRATE_FEATURES_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList crateFeatures_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string crate_features = 8;
* @return A list containing the crateFeatures.
*/
public com.google.protobuf.ProtocolStringList
getCrateFeaturesList() {
return crateFeatures_;
}
/**
* repeated string crate_features = 8;
* @return The count of crateFeatures.
*/
public int getCrateFeaturesCount() {
return crateFeatures_.size();
}
/**
* repeated string crate_features = 8;
* @param index The index of the element to return.
* @return The crateFeatures at the given index.
*/
public java.lang.String getCrateFeatures(int index) {
return crateFeatures_.get(index);
}
/**
* repeated string crate_features = 8;
* @param index The index of the value to return.
* @return The bytes of the crateFeatures at the given index.
*/
public com.google.protobuf.ByteString
getCrateFeaturesBytes(int index) {
return crateFeatures_.getByteString(index);
}
public static final int DEPENDENCIES_CRATE_IDS_FIELD_NUMBER = 9;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList dependenciesCrateIds_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string dependencies_crate_ids = 9;
* @return A list containing the dependenciesCrateIds.
*/
public com.google.protobuf.ProtocolStringList
getDependenciesCrateIdsList() {
return dependenciesCrateIds_;
}
/**
* repeated string dependencies_crate_ids = 9;
* @return The count of dependenciesCrateIds.
*/
public int getDependenciesCrateIdsCount() {
return dependenciesCrateIds_.size();
}
/**
* repeated string dependencies_crate_ids = 9;
* @param index The index of the element to return.
* @return The dependenciesCrateIds at the given index.
*/
public java.lang.String getDependenciesCrateIds(int index) {
return dependenciesCrateIds_.get(index);
}
/**
* repeated string dependencies_crate_ids = 9;
* @param index The index of the value to return.
* @return The bytes of the dependenciesCrateIds at the given index.
*/
public com.google.protobuf.ByteString
getDependenciesCrateIdsBytes(int index) {
return dependenciesCrateIds_.getByteString(index);
}
public static final int CRATE_ROOT_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private volatile java.lang.Object crateRoot_ = "";
/**
* string crate_root = 10;
* @return The crateRoot.
*/
@java.lang.Override
public java.lang.String getCrateRoot() {
java.lang.Object ref = crateRoot_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
crateRoot_ = s;
return s;
}
}
/**
* string crate_root = 10;
* @return The bytes for crateRoot.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCrateRootBytes() {
java.lang.Object ref = crateRoot_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
crateRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VERSION_FIELD_NUMBER = 11;
@SuppressWarnings("serial")
private volatile java.lang.Object version_ = "";
/**
* string version = 11;
* @return The version.
*/
@java.lang.Override
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
}
}
/**
* string version = 11;
* @return The bytes for version.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROC_MACRO_ARTIFACTS_FIELD_NUMBER = 12;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList procMacroArtifacts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
* repeated string proc_macro_artifacts = 12;
* @return A list containing the procMacroArtifacts.
*/
public com.google.protobuf.ProtocolStringList
getProcMacroArtifactsList() {
return procMacroArtifacts_;
}
/**
* repeated string proc_macro_artifacts = 12;
* @return The count of procMacroArtifacts.
*/
public int getProcMacroArtifactsCount() {
return procMacroArtifacts_.size();
}
/**
* repeated string proc_macro_artifacts = 12;
* @param index The index of the element to return.
* @return The procMacroArtifacts at the given index.
*/
public java.lang.String getProcMacroArtifacts(int index) {
return procMacroArtifacts_.get(index);
}
/**
* repeated string proc_macro_artifacts = 12;
* @param index The index of the value to return.
* @return The bytes of the procMacroArtifacts at the given index.
*/
public com.google.protobuf.ByteString
getProcMacroArtifactsBytes(int index) {
return procMacroArtifacts_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(crateId_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, crateId_);
}
if (location_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation.WORKSPACE_DIR.getNumber()) {
output.writeEnum(2, location_);
}
if (fromWorkspace_ != false) {
output.writeBool(3, fromWorkspace_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 4, name_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(kind_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 5, kind_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(edition_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 6, edition_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(outDir_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 7, outDir_);
}
for (int i = 0; i < crateFeatures_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 8, crateFeatures_.getRaw(i));
}
for (int i = 0; i < dependenciesCrateIds_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 9, dependenciesCrateIds_.getRaw(i));
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(crateRoot_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 10, crateRoot_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(version_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 11, version_);
}
for (int i = 0; i < procMacroArtifacts_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 12, procMacroArtifacts_.getRaw(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(crateId_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, crateId_);
}
if (location_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation.WORKSPACE_DIR.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, location_);
}
if (fromWorkspace_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, fromWorkspace_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(4, name_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(kind_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(5, kind_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(edition_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(6, edition_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(outDir_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(7, outDir_);
}
{
int dataSize = 0;
for (int i = 0; i < crateFeatures_.size(); i++) {
dataSize += computeStringSizeNoTag(crateFeatures_.getRaw(i));
}
size += dataSize;
size += 1 * getCrateFeaturesList().size();
}
{
int dataSize = 0;
for (int i = 0; i < dependenciesCrateIds_.size(); i++) {
dataSize += computeStringSizeNoTag(dependenciesCrateIds_.getRaw(i));
}
size += dataSize;
size += 1 * getDependenciesCrateIdsList().size();
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(crateRoot_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(10, crateRoot_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(version_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(11, version_);
}
{
int dataSize = 0;
for (int i = 0; i < procMacroArtifacts_.size(); i++) {
dataSize += computeStringSizeNoTag(procMacroArtifacts_.getRaw(i));
}
size += dataSize;
size += 1 * getProcMacroArtifactsList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo) obj;
if (!getCrateId()
.equals(other.getCrateId())) return false;
if (location_ != other.location_) return false;
if (getFromWorkspace()
!= other.getFromWorkspace()) return false;
if (!getName()
.equals(other.getName())) return false;
if (!getKind()
.equals(other.getKind())) return false;
if (!getEdition()
.equals(other.getEdition())) return false;
if (!getOutDir()
.equals(other.getOutDir())) return false;
if (!getCrateFeaturesList()
.equals(other.getCrateFeaturesList())) return false;
if (!getDependenciesCrateIdsList()
.equals(other.getDependenciesCrateIdsList())) return false;
if (!getCrateRoot()
.equals(other.getCrateRoot())) return false;
if (!getVersion()
.equals(other.getVersion())) return false;
if (!getProcMacroArtifactsList()
.equals(other.getProcMacroArtifactsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CRATE_ID_FIELD_NUMBER;
hash = (53 * hash) + getCrateId().hashCode();
hash = (37 * hash) + LOCATION_FIELD_NUMBER;
hash = (53 * hash) + location_;
hash = (37 * hash) + FROM_WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getFromWorkspace());
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + getKind().hashCode();
hash = (37 * hash) + EDITION_FIELD_NUMBER;
hash = (53 * hash) + getEdition().hashCode();
hash = (37 * hash) + OUT_DIR_FIELD_NUMBER;
hash = (53 * hash) + getOutDir().hashCode();
if (getCrateFeaturesCount() > 0) {
hash = (37 * hash) + CRATE_FEATURES_FIELD_NUMBER;
hash = (53 * hash) + getCrateFeaturesList().hashCode();
}
if (getDependenciesCrateIdsCount() > 0) {
hash = (37 * hash) + DEPENDENCIES_CRATE_IDS_FIELD_NUMBER;
hash = (53 * hash) + getDependenciesCrateIdsList().hashCode();
}
hash = (37 * hash) + CRATE_ROOT_FIELD_NUMBER;
hash = (53 * hash) + getCrateRoot().hashCode();
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion().hashCode();
if (getProcMacroArtifactsCount() > 0) {
hash = (37 * hash) + PROC_MACRO_ARTIFACTS_FIELD_NUMBER;
hash = (53 * hash) + getProcMacroArtifactsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.RustCrateInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.RustCrateInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_RustCrateInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_RustCrateInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
crateId_ = "";
location_ = 0;
fromWorkspace_ = false;
name_ = "";
kind_ = "";
edition_ = "";
outDir_ = "";
crateFeatures_ =
com.google.protobuf.LazyStringArrayList.emptyList();
dependenciesCrateIds_ =
com.google.protobuf.LazyStringArrayList.emptyList();
crateRoot_ = "";
version_ = "";
procMacroArtifacts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_RustCrateInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.crateId_ = crateId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.location_ = location_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.fromWorkspace_ = fromWorkspace_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.name_ = name_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.kind_ = kind_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.edition_ = edition_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.outDir_ = outDir_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
crateFeatures_.makeImmutable();
result.crateFeatures_ = crateFeatures_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
dependenciesCrateIds_.makeImmutable();
result.dependenciesCrateIds_ = dependenciesCrateIds_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.crateRoot_ = crateRoot_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.version_ = version_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
procMacroArtifacts_.makeImmutable();
result.procMacroArtifacts_ = procMacroArtifacts_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo.getDefaultInstance()) return this;
if (!other.getCrateId().isEmpty()) {
crateId_ = other.crateId_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.location_ != 0) {
setLocationValue(other.getLocationValue());
}
if (other.getFromWorkspace() != false) {
setFromWorkspace(other.getFromWorkspace());
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
bitField0_ |= 0x00000008;
onChanged();
}
if (!other.getKind().isEmpty()) {
kind_ = other.kind_;
bitField0_ |= 0x00000010;
onChanged();
}
if (!other.getEdition().isEmpty()) {
edition_ = other.edition_;
bitField0_ |= 0x00000020;
onChanged();
}
if (!other.getOutDir().isEmpty()) {
outDir_ = other.outDir_;
bitField0_ |= 0x00000040;
onChanged();
}
if (!other.crateFeatures_.isEmpty()) {
if (crateFeatures_.isEmpty()) {
crateFeatures_ = other.crateFeatures_;
bitField0_ |= 0x00000080;
} else {
ensureCrateFeaturesIsMutable();
crateFeatures_.addAll(other.crateFeatures_);
}
onChanged();
}
if (!other.dependenciesCrateIds_.isEmpty()) {
if (dependenciesCrateIds_.isEmpty()) {
dependenciesCrateIds_ = other.dependenciesCrateIds_;
bitField0_ |= 0x00000100;
} else {
ensureDependenciesCrateIdsIsMutable();
dependenciesCrateIds_.addAll(other.dependenciesCrateIds_);
}
onChanged();
}
if (!other.getCrateRoot().isEmpty()) {
crateRoot_ = other.crateRoot_;
bitField0_ |= 0x00000200;
onChanged();
}
if (!other.getVersion().isEmpty()) {
version_ = other.version_;
bitField0_ |= 0x00000400;
onChanged();
}
if (!other.procMacroArtifacts_.isEmpty()) {
if (procMacroArtifacts_.isEmpty()) {
procMacroArtifacts_ = other.procMacroArtifacts_;
bitField0_ |= 0x00000800;
} else {
ensureProcMacroArtifactsIsMutable();
procMacroArtifacts_.addAll(other.procMacroArtifacts_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
crateId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
location_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
fromWorkspace_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
kind_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
edition_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 50
case 58: {
outDir_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 58
case 66: {
java.lang.String s = input.readStringRequireUtf8();
ensureCrateFeaturesIsMutable();
crateFeatures_.add(s);
break;
} // case 66
case 74: {
java.lang.String s = input.readStringRequireUtf8();
ensureDependenciesCrateIdsIsMutable();
dependenciesCrateIds_.add(s);
break;
} // case 74
case 82: {
crateRoot_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case 82
case 90: {
version_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000400;
break;
} // case 90
case 98: {
java.lang.String s = input.readStringRequireUtf8();
ensureProcMacroArtifactsIsMutable();
procMacroArtifacts_.add(s);
break;
} // case 98
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object crateId_ = "";
/**
* string crate_id = 1;
* @return The crateId.
*/
public java.lang.String getCrateId() {
java.lang.Object ref = crateId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
crateId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string crate_id = 1;
* @return The bytes for crateId.
*/
public com.google.protobuf.ByteString
getCrateIdBytes() {
java.lang.Object ref = crateId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
crateId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string crate_id = 1;
* @param value The crateId to set.
* @return This builder for chaining.
*/
public Builder setCrateId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
crateId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string crate_id = 1;
* @return This builder for chaining.
*/
public Builder clearCrateId() {
crateId_ = getDefaultInstance().getCrateId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string crate_id = 1;
* @param value The bytes for crateId to set.
* @return This builder for chaining.
*/
public Builder setCrateIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
crateId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int location_ = 0;
/**
* .bazelbsp.RustCrateLocation location = 2;
* @return The enum numeric value on the wire for location.
*/
@java.lang.Override public int getLocationValue() {
return location_;
}
/**
* .bazelbsp.RustCrateLocation location = 2;
* @param value The enum numeric value on the wire for location to set.
* @return This builder for chaining.
*/
public Builder setLocationValue(int value) {
location_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .bazelbsp.RustCrateLocation location = 2;
* @return The location.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation getLocation() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation result = org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation.forNumber(location_);
return result == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation.UNRECOGNIZED : result;
}
/**
* .bazelbsp.RustCrateLocation location = 2;
* @param value The location to set.
* @return This builder for chaining.
*/
public Builder setLocation(org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateLocation value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
location_ = value.getNumber();
onChanged();
return this;
}
/**
* .bazelbsp.RustCrateLocation location = 2;
* @return This builder for chaining.
*/
public Builder clearLocation() {
bitField0_ = (bitField0_ & ~0x00000002);
location_ = 0;
onChanged();
return this;
}
private boolean fromWorkspace_ ;
/**
* bool from_workspace = 3;
* @return The fromWorkspace.
*/
@java.lang.Override
public boolean getFromWorkspace() {
return fromWorkspace_;
}
/**
* bool from_workspace = 3;
* @param value The fromWorkspace to set.
* @return This builder for chaining.
*/
public Builder setFromWorkspace(boolean value) {
fromWorkspace_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* bool from_workspace = 3;
* @return This builder for chaining.
*/
public Builder clearFromWorkspace() {
bitField0_ = (bitField0_ & ~0x00000004);
fromWorkspace_ = false;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 4;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 4;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 4;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* string name = 4;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* string name = 4;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object kind_ = "";
/**
* string kind = 5;
* @return The kind.
*/
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string kind = 5;
* @return The bytes for kind.
*/
public com.google.protobuf.ByteString
getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string kind = 5;
* @param value The kind to set.
* @return This builder for chaining.
*/
public Builder setKind(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
kind_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* string kind = 5;
* @return This builder for chaining.
*/
public Builder clearKind() {
kind_ = getDefaultInstance().getKind();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* string kind = 5;
* @param value The bytes for kind to set.
* @return This builder for chaining.
*/
public Builder setKindBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
kind_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private java.lang.Object edition_ = "";
/**
* string edition = 6;
* @return The edition.
*/
public java.lang.String getEdition() {
java.lang.Object ref = edition_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
edition_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string edition = 6;
* @return The bytes for edition.
*/
public com.google.protobuf.ByteString
getEditionBytes() {
java.lang.Object ref = edition_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
edition_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string edition = 6;
* @param value The edition to set.
* @return This builder for chaining.
*/
public Builder setEdition(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
edition_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* string edition = 6;
* @return This builder for chaining.
*/
public Builder clearEdition() {
edition_ = getDefaultInstance().getEdition();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
* string edition = 6;
* @param value The bytes for edition to set.
* @return This builder for chaining.
*/
public Builder setEditionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
edition_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.lang.Object outDir_ = "";
/**
* string out_dir = 7;
* @return The outDir.
*/
public java.lang.String getOutDir() {
java.lang.Object ref = outDir_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
outDir_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string out_dir = 7;
* @return The bytes for outDir.
*/
public com.google.protobuf.ByteString
getOutDirBytes() {
java.lang.Object ref = outDir_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
outDir_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string out_dir = 7;
* @param value The outDir to set.
* @return This builder for chaining.
*/
public Builder setOutDir(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
outDir_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* string out_dir = 7;
* @return This builder for chaining.
*/
public Builder clearOutDir() {
outDir_ = getDefaultInstance().getOutDir();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
* string out_dir = 7;
* @param value The bytes for outDir to set.
* @return This builder for chaining.
*/
public Builder setOutDirBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
outDir_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList crateFeatures_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureCrateFeaturesIsMutable() {
if (!crateFeatures_.isModifiable()) {
crateFeatures_ = new com.google.protobuf.LazyStringArrayList(crateFeatures_);
}
bitField0_ |= 0x00000080;
}
/**
* repeated string crate_features = 8;
* @return A list containing the crateFeatures.
*/
public com.google.protobuf.ProtocolStringList
getCrateFeaturesList() {
crateFeatures_.makeImmutable();
return crateFeatures_;
}
/**
* repeated string crate_features = 8;
* @return The count of crateFeatures.
*/
public int getCrateFeaturesCount() {
return crateFeatures_.size();
}
/**
* repeated string crate_features = 8;
* @param index The index of the element to return.
* @return The crateFeatures at the given index.
*/
public java.lang.String getCrateFeatures(int index) {
return crateFeatures_.get(index);
}
/**
* repeated string crate_features = 8;
* @param index The index of the value to return.
* @return The bytes of the crateFeatures at the given index.
*/
public com.google.protobuf.ByteString
getCrateFeaturesBytes(int index) {
return crateFeatures_.getByteString(index);
}
/**
* repeated string crate_features = 8;
* @param index The index to set the value at.
* @param value The crateFeatures to set.
* @return This builder for chaining.
*/
public Builder setCrateFeatures(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureCrateFeaturesIsMutable();
crateFeatures_.set(index, value);
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
* repeated string crate_features = 8;
* @param value The crateFeatures to add.
* @return This builder for chaining.
*/
public Builder addCrateFeatures(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureCrateFeaturesIsMutable();
crateFeatures_.add(value);
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
* repeated string crate_features = 8;
* @param values The crateFeatures to add.
* @return This builder for chaining.
*/
public Builder addAllCrateFeatures(
java.lang.Iterable values) {
ensureCrateFeaturesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, crateFeatures_);
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
* repeated string crate_features = 8;
* @return This builder for chaining.
*/
public Builder clearCrateFeatures() {
crateFeatures_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);;
onChanged();
return this;
}
/**
* repeated string crate_features = 8;
* @param value The bytes of the crateFeatures to add.
* @return This builder for chaining.
*/
public Builder addCrateFeaturesBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureCrateFeaturesIsMutable();
crateFeatures_.add(value);
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList dependenciesCrateIds_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureDependenciesCrateIdsIsMutable() {
if (!dependenciesCrateIds_.isModifiable()) {
dependenciesCrateIds_ = new com.google.protobuf.LazyStringArrayList(dependenciesCrateIds_);
}
bitField0_ |= 0x00000100;
}
/**
* repeated string dependencies_crate_ids = 9;
* @return A list containing the dependenciesCrateIds.
*/
public com.google.protobuf.ProtocolStringList
getDependenciesCrateIdsList() {
dependenciesCrateIds_.makeImmutable();
return dependenciesCrateIds_;
}
/**
* repeated string dependencies_crate_ids = 9;
* @return The count of dependenciesCrateIds.
*/
public int getDependenciesCrateIdsCount() {
return dependenciesCrateIds_.size();
}
/**
* repeated string dependencies_crate_ids = 9;
* @param index The index of the element to return.
* @return The dependenciesCrateIds at the given index.
*/
public java.lang.String getDependenciesCrateIds(int index) {
return dependenciesCrateIds_.get(index);
}
/**
* repeated string dependencies_crate_ids = 9;
* @param index The index of the value to return.
* @return The bytes of the dependenciesCrateIds at the given index.
*/
public com.google.protobuf.ByteString
getDependenciesCrateIdsBytes(int index) {
return dependenciesCrateIds_.getByteString(index);
}
/**
* repeated string dependencies_crate_ids = 9;
* @param index The index to set the value at.
* @param value The dependenciesCrateIds to set.
* @return This builder for chaining.
*/
public Builder setDependenciesCrateIds(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureDependenciesCrateIdsIsMutable();
dependenciesCrateIds_.set(index, value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* repeated string dependencies_crate_ids = 9;
* @param value The dependenciesCrateIds to add.
* @return This builder for chaining.
*/
public Builder addDependenciesCrateIds(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureDependenciesCrateIdsIsMutable();
dependenciesCrateIds_.add(value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* repeated string dependencies_crate_ids = 9;
* @param values The dependenciesCrateIds to add.
* @return This builder for chaining.
*/
public Builder addAllDependenciesCrateIds(
java.lang.Iterable values) {
ensureDependenciesCrateIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, dependenciesCrateIds_);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* repeated string dependencies_crate_ids = 9;
* @return This builder for chaining.
*/
public Builder clearDependenciesCrateIds() {
dependenciesCrateIds_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);;
onChanged();
return this;
}
/**
* repeated string dependencies_crate_ids = 9;
* @param value The bytes of the dependenciesCrateIds to add.
* @return This builder for chaining.
*/
public Builder addDependenciesCrateIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureDependenciesCrateIdsIsMutable();
dependenciesCrateIds_.add(value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private java.lang.Object crateRoot_ = "";
/**
* string crate_root = 10;
* @return The crateRoot.
*/
public java.lang.String getCrateRoot() {
java.lang.Object ref = crateRoot_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
crateRoot_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string crate_root = 10;
* @return The bytes for crateRoot.
*/
public com.google.protobuf.ByteString
getCrateRootBytes() {
java.lang.Object ref = crateRoot_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
crateRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string crate_root = 10;
* @param value The crateRoot to set.
* @return This builder for chaining.
*/
public Builder setCrateRoot(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
crateRoot_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
* string crate_root = 10;
* @return This builder for chaining.
*/
public Builder clearCrateRoot() {
crateRoot_ = getDefaultInstance().getCrateRoot();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
* string crate_root = 10;
* @param value The bytes for crateRoot to set.
* @return This builder for chaining.
*/
public Builder setCrateRootBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
crateRoot_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
private java.lang.Object version_ = "";
/**
* string version = 11;
* @return The version.
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string version = 11;
* @return The bytes for version.
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string version = 11;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
version_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
* string version = 11;
* @return This builder for chaining.
*/
public Builder clearVersion() {
version_ = getDefaultInstance().getVersion();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
return this;
}
/**
* string version = 11;
* @param value The bytes for version to set.
* @return This builder for chaining.
*/
public Builder setVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
version_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList procMacroArtifacts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureProcMacroArtifactsIsMutable() {
if (!procMacroArtifacts_.isModifiable()) {
procMacroArtifacts_ = new com.google.protobuf.LazyStringArrayList(procMacroArtifacts_);
}
bitField0_ |= 0x00000800;
}
/**
* repeated string proc_macro_artifacts = 12;
* @return A list containing the procMacroArtifacts.
*/
public com.google.protobuf.ProtocolStringList
getProcMacroArtifactsList() {
procMacroArtifacts_.makeImmutable();
return procMacroArtifacts_;
}
/**
* repeated string proc_macro_artifacts = 12;
* @return The count of procMacroArtifacts.
*/
public int getProcMacroArtifactsCount() {
return procMacroArtifacts_.size();
}
/**
* repeated string proc_macro_artifacts = 12;
* @param index The index of the element to return.
* @return The procMacroArtifacts at the given index.
*/
public java.lang.String getProcMacroArtifacts(int index) {
return procMacroArtifacts_.get(index);
}
/**
* repeated string proc_macro_artifacts = 12;
* @param index The index of the value to return.
* @return The bytes of the procMacroArtifacts at the given index.
*/
public com.google.protobuf.ByteString
getProcMacroArtifactsBytes(int index) {
return procMacroArtifacts_.getByteString(index);
}
/**
* repeated string proc_macro_artifacts = 12;
* @param index The index to set the value at.
* @param value The procMacroArtifacts to set.
* @return This builder for chaining.
*/
public Builder setProcMacroArtifacts(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureProcMacroArtifactsIsMutable();
procMacroArtifacts_.set(index, value);
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
* repeated string proc_macro_artifacts = 12;
* @param value The procMacroArtifacts to add.
* @return This builder for chaining.
*/
public Builder addProcMacroArtifacts(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureProcMacroArtifactsIsMutable();
procMacroArtifacts_.add(value);
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
* repeated string proc_macro_artifacts = 12;
* @param values The procMacroArtifacts to add.
* @return This builder for chaining.
*/
public Builder addAllProcMacroArtifacts(
java.lang.Iterable values) {
ensureProcMacroArtifactsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, procMacroArtifacts_);
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
* repeated string proc_macro_artifacts = 12;
* @return This builder for chaining.
*/
public Builder clearProcMacroArtifacts() {
procMacroArtifacts_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000800);;
onChanged();
return this;
}
/**
* repeated string proc_macro_artifacts = 12;
* @param value The bytes of the procMacroArtifacts to add.
* @return This builder for chaining.
*/
public Builder addProcMacroArtifactsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureProcMacroArtifactsIsMutable();
procMacroArtifacts_.add(value);
bitField0_ |= 0x00000800;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.RustCrateInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.RustCrateInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RustCrateInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.RustCrateInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AndroidTargetInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.AndroidTargetInfo)
com.google.protobuf.MessageOrBuilder {
/**
* .bazelbsp.FileLocation android_jar = 100;
* @return Whether the androidJar field is set.
*/
boolean hasAndroidJar();
/**
* .bazelbsp.FileLocation android_jar = 100;
* @return The androidJar.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAndroidJar();
/**
* .bazelbsp.FileLocation android_jar = 100;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAndroidJarOrBuilder();
/**
* .bazelbsp.FileLocation manifest = 200;
* @return Whether the manifest field is set.
*/
boolean hasManifest();
/**
* .bazelbsp.FileLocation manifest = 200;
* @return The manifest.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getManifest();
/**
* .bazelbsp.FileLocation manifest = 200;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getManifestOrBuilder();
/**
* map<string, string> manifest_overrides = 250;
*/
int getManifestOverridesCount();
/**
* map<string, string> manifest_overrides = 250;
*/
boolean containsManifestOverrides(
java.lang.String key);
/**
* Use {@link #getManifestOverridesMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getManifestOverrides();
/**
* map<string, string> manifest_overrides = 250;
*/
java.util.Map
getManifestOverridesMap();
/**
* map<string, string> manifest_overrides = 250;
*/
/* nullable */
java.lang.String getManifestOverridesOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> manifest_overrides = 250;
*/
java.lang.String getManifestOverridesOrThrow(
java.lang.String key);
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
java.util.List
getResourceDirectoriesList();
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getResourceDirectories(int index);
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
int getResourceDirectoriesCount();
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getResourceDirectoriesOrBuilderList();
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getResourceDirectoriesOrBuilder(
int index);
/**
* string resource_java_package = 400;
* @return The resourceJavaPackage.
*/
java.lang.String getResourceJavaPackage();
/**
* string resource_java_package = 400;
* @return The bytes for resourceJavaPackage.
*/
com.google.protobuf.ByteString
getResourceJavaPackageBytes();
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
java.util.List
getAssetsDirectoriesList();
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAssetsDirectories(int index);
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
int getAssetsDirectoriesCount();
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getAssetsDirectoriesOrBuilderList();
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAssetsDirectoriesOrBuilder(
int index);
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
* @return Whether the aidlBinaryJar field is set.
*/
boolean hasAidlBinaryJar();
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
* @return The aidlBinaryJar.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAidlBinaryJar();
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAidlBinaryJarOrBuilder();
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
* @return Whether the aidlSourceJar field is set.
*/
boolean hasAidlSourceJar();
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
* @return The aidlSourceJar.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAidlSourceJar();
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAidlSourceJarOrBuilder();
/**
* .bazelbsp.FileLocation apk = 700;
* @return Whether the apk field is set.
*/
boolean hasApk();
/**
* .bazelbsp.FileLocation apk = 700;
* @return The apk.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getApk();
/**
* .bazelbsp.FileLocation apk = 700;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getApkOrBuilder();
}
/**
* Protobuf type {@code bazelbsp.AndroidTargetInfo}
*/
public static final class AndroidTargetInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.AndroidTargetInfo)
AndroidTargetInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
AndroidTargetInfo.class.getName());
}
// Use AndroidTargetInfo.newBuilder() to construct.
private AndroidTargetInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private AndroidTargetInfo() {
resourceDirectories_ = java.util.Collections.emptyList();
resourceJavaPackage_ = "";
assetsDirectories_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_AndroidTargetInfo_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 250:
return internalGetManifestOverrides();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_AndroidTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo.Builder.class);
}
private int bitField0_;
public static final int ANDROID_JAR_FIELD_NUMBER = 100;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation androidJar_;
/**
* .bazelbsp.FileLocation android_jar = 100;
* @return Whether the androidJar field is set.
*/
@java.lang.Override
public boolean hasAndroidJar() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .bazelbsp.FileLocation android_jar = 100;
* @return The androidJar.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAndroidJar() {
return androidJar_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : androidJar_;
}
/**
* .bazelbsp.FileLocation android_jar = 100;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAndroidJarOrBuilder() {
return androidJar_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : androidJar_;
}
public static final int MANIFEST_FIELD_NUMBER = 200;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation manifest_;
/**
* .bazelbsp.FileLocation manifest = 200;
* @return Whether the manifest field is set.
*/
@java.lang.Override
public boolean hasManifest() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .bazelbsp.FileLocation manifest = 200;
* @return The manifest.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getManifest() {
return manifest_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : manifest_;
}
/**
* .bazelbsp.FileLocation manifest = 200;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getManifestOrBuilder() {
return manifest_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : manifest_;
}
public static final int MANIFEST_OVERRIDES_FIELD_NUMBER = 250;
private static final class ManifestOverridesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_AndroidTargetInfo_ManifestOverridesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> manifestOverrides_;
private com.google.protobuf.MapField
internalGetManifestOverrides() {
if (manifestOverrides_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ManifestOverridesDefaultEntryHolder.defaultEntry);
}
return manifestOverrides_;
}
public int getManifestOverridesCount() {
return internalGetManifestOverrides().getMap().size();
}
/**
* map<string, string> manifest_overrides = 250;
*/
@java.lang.Override
public boolean containsManifestOverrides(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetManifestOverrides().getMap().containsKey(key);
}
/**
* Use {@link #getManifestOverridesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getManifestOverrides() {
return getManifestOverridesMap();
}
/**
* map<string, string> manifest_overrides = 250;
*/
@java.lang.Override
public java.util.Map getManifestOverridesMap() {
return internalGetManifestOverrides().getMap();
}
/**
* map<string, string> manifest_overrides = 250;
*/
@java.lang.Override
public /* nullable */
java.lang.String getManifestOverridesOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetManifestOverrides().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> manifest_overrides = 250;
*/
@java.lang.Override
public java.lang.String getManifestOverridesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetManifestOverrides().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int RESOURCE_DIRECTORIES_FIELD_NUMBER = 300;
@SuppressWarnings("serial")
private java.util.List resourceDirectories_;
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
@java.lang.Override
public java.util.List getResourceDirectoriesList() {
return resourceDirectories_;
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getResourceDirectoriesOrBuilderList() {
return resourceDirectories_;
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
@java.lang.Override
public int getResourceDirectoriesCount() {
return resourceDirectories_.size();
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getResourceDirectories(int index) {
return resourceDirectories_.get(index);
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getResourceDirectoriesOrBuilder(
int index) {
return resourceDirectories_.get(index);
}
public static final int RESOURCE_JAVA_PACKAGE_FIELD_NUMBER = 400;
@SuppressWarnings("serial")
private volatile java.lang.Object resourceJavaPackage_ = "";
/**
* string resource_java_package = 400;
* @return The resourceJavaPackage.
*/
@java.lang.Override
public java.lang.String getResourceJavaPackage() {
java.lang.Object ref = resourceJavaPackage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceJavaPackage_ = s;
return s;
}
}
/**
* string resource_java_package = 400;
* @return The bytes for resourceJavaPackage.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceJavaPackageBytes() {
java.lang.Object ref = resourceJavaPackage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceJavaPackage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ASSETS_DIRECTORIES_FIELD_NUMBER = 450;
@SuppressWarnings("serial")
private java.util.List assetsDirectories_;
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
@java.lang.Override
public java.util.List getAssetsDirectoriesList() {
return assetsDirectories_;
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getAssetsDirectoriesOrBuilderList() {
return assetsDirectories_;
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
@java.lang.Override
public int getAssetsDirectoriesCount() {
return assetsDirectories_.size();
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAssetsDirectories(int index) {
return assetsDirectories_.get(index);
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAssetsDirectoriesOrBuilder(
int index) {
return assetsDirectories_.get(index);
}
public static final int AIDL_BINARY_JAR_FIELD_NUMBER = 500;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation aidlBinaryJar_;
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
* @return Whether the aidlBinaryJar field is set.
*/
@java.lang.Override
public boolean hasAidlBinaryJar() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
* @return The aidlBinaryJar.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAidlBinaryJar() {
return aidlBinaryJar_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : aidlBinaryJar_;
}
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAidlBinaryJarOrBuilder() {
return aidlBinaryJar_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : aidlBinaryJar_;
}
public static final int AIDL_SOURCE_JAR_FIELD_NUMBER = 600;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation aidlSourceJar_;
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
* @return Whether the aidlSourceJar field is set.
*/
@java.lang.Override
public boolean hasAidlSourceJar() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
* @return The aidlSourceJar.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAidlSourceJar() {
return aidlSourceJar_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : aidlSourceJar_;
}
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAidlSourceJarOrBuilder() {
return aidlSourceJar_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : aidlSourceJar_;
}
public static final int APK_FIELD_NUMBER = 700;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation apk_;
/**
* .bazelbsp.FileLocation apk = 700;
* @return Whether the apk field is set.
*/
@java.lang.Override
public boolean hasApk() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* .bazelbsp.FileLocation apk = 700;
* @return The apk.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getApk() {
return apk_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : apk_;
}
/**
* .bazelbsp.FileLocation apk = 700;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getApkOrBuilder() {
return apk_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : apk_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(100, getAndroidJar());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(200, getManifest());
}
com.google.protobuf.GeneratedMessage
.serializeStringMapTo(
output,
internalGetManifestOverrides(),
ManifestOverridesDefaultEntryHolder.defaultEntry,
250);
for (int i = 0; i < resourceDirectories_.size(); i++) {
output.writeMessage(300, resourceDirectories_.get(i));
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(resourceJavaPackage_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 400, resourceJavaPackage_);
}
for (int i = 0; i < assetsDirectories_.size(); i++) {
output.writeMessage(450, assetsDirectories_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(500, getAidlBinaryJar());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(600, getAidlSourceJar());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(700, getApk());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(100, getAndroidJar());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(200, getManifest());
}
for (java.util.Map.Entry entry
: internalGetManifestOverrides().getMap().entrySet()) {
com.google.protobuf.MapEntry
manifestOverrides__ = ManifestOverridesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(250, manifestOverrides__);
}
for (int i = 0; i < resourceDirectories_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(300, resourceDirectories_.get(i));
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(resourceJavaPackage_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(400, resourceJavaPackage_);
}
for (int i = 0; i < assetsDirectories_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(450, assetsDirectories_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(500, getAidlBinaryJar());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(600, getAidlSourceJar());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(700, getApk());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo) obj;
if (hasAndroidJar() != other.hasAndroidJar()) return false;
if (hasAndroidJar()) {
if (!getAndroidJar()
.equals(other.getAndroidJar())) return false;
}
if (hasManifest() != other.hasManifest()) return false;
if (hasManifest()) {
if (!getManifest()
.equals(other.getManifest())) return false;
}
if (!internalGetManifestOverrides().equals(
other.internalGetManifestOverrides())) return false;
if (!getResourceDirectoriesList()
.equals(other.getResourceDirectoriesList())) return false;
if (!getResourceJavaPackage()
.equals(other.getResourceJavaPackage())) return false;
if (!getAssetsDirectoriesList()
.equals(other.getAssetsDirectoriesList())) return false;
if (hasAidlBinaryJar() != other.hasAidlBinaryJar()) return false;
if (hasAidlBinaryJar()) {
if (!getAidlBinaryJar()
.equals(other.getAidlBinaryJar())) return false;
}
if (hasAidlSourceJar() != other.hasAidlSourceJar()) return false;
if (hasAidlSourceJar()) {
if (!getAidlSourceJar()
.equals(other.getAidlSourceJar())) return false;
}
if (hasApk() != other.hasApk()) return false;
if (hasApk()) {
if (!getApk()
.equals(other.getApk())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAndroidJar()) {
hash = (37 * hash) + ANDROID_JAR_FIELD_NUMBER;
hash = (53 * hash) + getAndroidJar().hashCode();
}
if (hasManifest()) {
hash = (37 * hash) + MANIFEST_FIELD_NUMBER;
hash = (53 * hash) + getManifest().hashCode();
}
if (!internalGetManifestOverrides().getMap().isEmpty()) {
hash = (37 * hash) + MANIFEST_OVERRIDES_FIELD_NUMBER;
hash = (53 * hash) + internalGetManifestOverrides().hashCode();
}
if (getResourceDirectoriesCount() > 0) {
hash = (37 * hash) + RESOURCE_DIRECTORIES_FIELD_NUMBER;
hash = (53 * hash) + getResourceDirectoriesList().hashCode();
}
hash = (37 * hash) + RESOURCE_JAVA_PACKAGE_FIELD_NUMBER;
hash = (53 * hash) + getResourceJavaPackage().hashCode();
if (getAssetsDirectoriesCount() > 0) {
hash = (37 * hash) + ASSETS_DIRECTORIES_FIELD_NUMBER;
hash = (53 * hash) + getAssetsDirectoriesList().hashCode();
}
if (hasAidlBinaryJar()) {
hash = (37 * hash) + AIDL_BINARY_JAR_FIELD_NUMBER;
hash = (53 * hash) + getAidlBinaryJar().hashCode();
}
if (hasAidlSourceJar()) {
hash = (37 * hash) + AIDL_SOURCE_JAR_FIELD_NUMBER;
hash = (53 * hash) + getAidlSourceJar().hashCode();
}
if (hasApk()) {
hash = (37 * hash) + APK_FIELD_NUMBER;
hash = (53 * hash) + getApk().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.AndroidTargetInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.AndroidTargetInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_AndroidTargetInfo_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 250:
return internalGetManifestOverrides();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 250:
return internalGetMutableManifestOverrides();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_AndroidTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage
.alwaysUseFieldBuilders) {
getAndroidJarFieldBuilder();
getManifestFieldBuilder();
getResourceDirectoriesFieldBuilder();
getAssetsDirectoriesFieldBuilder();
getAidlBinaryJarFieldBuilder();
getAidlSourceJarFieldBuilder();
getApkFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
androidJar_ = null;
if (androidJarBuilder_ != null) {
androidJarBuilder_.dispose();
androidJarBuilder_ = null;
}
manifest_ = null;
if (manifestBuilder_ != null) {
manifestBuilder_.dispose();
manifestBuilder_ = null;
}
internalGetMutableManifestOverrides().clear();
if (resourceDirectoriesBuilder_ == null) {
resourceDirectories_ = java.util.Collections.emptyList();
} else {
resourceDirectories_ = null;
resourceDirectoriesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
resourceJavaPackage_ = "";
if (assetsDirectoriesBuilder_ == null) {
assetsDirectories_ = java.util.Collections.emptyList();
} else {
assetsDirectories_ = null;
assetsDirectoriesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
aidlBinaryJar_ = null;
if (aidlBinaryJarBuilder_ != null) {
aidlBinaryJarBuilder_.dispose();
aidlBinaryJarBuilder_ = null;
}
aidlSourceJar_ = null;
if (aidlSourceJarBuilder_ != null) {
aidlSourceJarBuilder_.dispose();
aidlSourceJarBuilder_ = null;
}
apk_ = null;
if (apkBuilder_ != null) {
apkBuilder_.dispose();
apkBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_AndroidTargetInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo result) {
if (resourceDirectoriesBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
resourceDirectories_ = java.util.Collections.unmodifiableList(resourceDirectories_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.resourceDirectories_ = resourceDirectories_;
} else {
result.resourceDirectories_ = resourceDirectoriesBuilder_.build();
}
if (assetsDirectoriesBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
assetsDirectories_ = java.util.Collections.unmodifiableList(assetsDirectories_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.assetsDirectories_ = assetsDirectories_;
} else {
result.assetsDirectories_ = assetsDirectoriesBuilder_.build();
}
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.androidJar_ = androidJarBuilder_ == null
? androidJar_
: androidJarBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.manifest_ = manifestBuilder_ == null
? manifest_
: manifestBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.manifestOverrides_ = internalGetManifestOverrides();
result.manifestOverrides_.makeImmutable();
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.resourceJavaPackage_ = resourceJavaPackage_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.aidlBinaryJar_ = aidlBinaryJarBuilder_ == null
? aidlBinaryJar_
: aidlBinaryJarBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.aidlSourceJar_ = aidlSourceJarBuilder_ == null
? aidlSourceJar_
: aidlSourceJarBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.apk_ = apkBuilder_ == null
? apk_
: apkBuilder_.build();
to_bitField0_ |= 0x00000010;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo.getDefaultInstance()) return this;
if (other.hasAndroidJar()) {
mergeAndroidJar(other.getAndroidJar());
}
if (other.hasManifest()) {
mergeManifest(other.getManifest());
}
internalGetMutableManifestOverrides().mergeFrom(
other.internalGetManifestOverrides());
bitField0_ |= 0x00000004;
if (resourceDirectoriesBuilder_ == null) {
if (!other.resourceDirectories_.isEmpty()) {
if (resourceDirectories_.isEmpty()) {
resourceDirectories_ = other.resourceDirectories_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureResourceDirectoriesIsMutable();
resourceDirectories_.addAll(other.resourceDirectories_);
}
onChanged();
}
} else {
if (!other.resourceDirectories_.isEmpty()) {
if (resourceDirectoriesBuilder_.isEmpty()) {
resourceDirectoriesBuilder_.dispose();
resourceDirectoriesBuilder_ = null;
resourceDirectories_ = other.resourceDirectories_;
bitField0_ = (bitField0_ & ~0x00000008);
resourceDirectoriesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getResourceDirectoriesFieldBuilder() : null;
} else {
resourceDirectoriesBuilder_.addAllMessages(other.resourceDirectories_);
}
}
}
if (!other.getResourceJavaPackage().isEmpty()) {
resourceJavaPackage_ = other.resourceJavaPackage_;
bitField0_ |= 0x00000010;
onChanged();
}
if (assetsDirectoriesBuilder_ == null) {
if (!other.assetsDirectories_.isEmpty()) {
if (assetsDirectories_.isEmpty()) {
assetsDirectories_ = other.assetsDirectories_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureAssetsDirectoriesIsMutable();
assetsDirectories_.addAll(other.assetsDirectories_);
}
onChanged();
}
} else {
if (!other.assetsDirectories_.isEmpty()) {
if (assetsDirectoriesBuilder_.isEmpty()) {
assetsDirectoriesBuilder_.dispose();
assetsDirectoriesBuilder_ = null;
assetsDirectories_ = other.assetsDirectories_;
bitField0_ = (bitField0_ & ~0x00000020);
assetsDirectoriesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getAssetsDirectoriesFieldBuilder() : null;
} else {
assetsDirectoriesBuilder_.addAllMessages(other.assetsDirectories_);
}
}
}
if (other.hasAidlBinaryJar()) {
mergeAidlBinaryJar(other.getAidlBinaryJar());
}
if (other.hasAidlSourceJar()) {
mergeAidlSourceJar(other.getAidlSourceJar());
}
if (other.hasApk()) {
mergeApk(other.getApk());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 802: {
input.readMessage(
getAndroidJarFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 802
case 1602: {
input.readMessage(
getManifestFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 1602
case 2002: {
com.google.protobuf.MapEntry
manifestOverrides__ = input.readMessage(
ManifestOverridesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableManifestOverrides().getMutableMap().put(
manifestOverrides__.getKey(), manifestOverrides__.getValue());
bitField0_ |= 0x00000004;
break;
} // case 2002
case 2402: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (resourceDirectoriesBuilder_ == null) {
ensureResourceDirectoriesIsMutable();
resourceDirectories_.add(m);
} else {
resourceDirectoriesBuilder_.addMessage(m);
}
break;
} // case 2402
case 3202: {
resourceJavaPackage_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 3202
case 3602: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (assetsDirectoriesBuilder_ == null) {
ensureAssetsDirectoriesIsMutable();
assetsDirectories_.add(m);
} else {
assetsDirectoriesBuilder_.addMessage(m);
}
break;
} // case 3602
case 4002: {
input.readMessage(
getAidlBinaryJarFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 4002
case 4802: {
input.readMessage(
getAidlSourceJarFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 4802
case 5602: {
input.readMessage(
getApkFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 5602
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation androidJar_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> androidJarBuilder_;
/**
* .bazelbsp.FileLocation android_jar = 100;
* @return Whether the androidJar field is set.
*/
public boolean hasAndroidJar() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .bazelbsp.FileLocation android_jar = 100;
* @return The androidJar.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAndroidJar() {
if (androidJarBuilder_ == null) {
return androidJar_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : androidJar_;
} else {
return androidJarBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation android_jar = 100;
*/
public Builder setAndroidJar(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (androidJarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
androidJar_ = value;
} else {
androidJarBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation android_jar = 100;
*/
public Builder setAndroidJar(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (androidJarBuilder_ == null) {
androidJar_ = builderForValue.build();
} else {
androidJarBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation android_jar = 100;
*/
public Builder mergeAndroidJar(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (androidJarBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
androidJar_ != null &&
androidJar_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getAndroidJarBuilder().mergeFrom(value);
} else {
androidJar_ = value;
}
} else {
androidJarBuilder_.mergeFrom(value);
}
if (androidJar_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation android_jar = 100;
*/
public Builder clearAndroidJar() {
bitField0_ = (bitField0_ & ~0x00000001);
androidJar_ = null;
if (androidJarBuilder_ != null) {
androidJarBuilder_.dispose();
androidJarBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation android_jar = 100;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getAndroidJarBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getAndroidJarFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation android_jar = 100;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAndroidJarOrBuilder() {
if (androidJarBuilder_ != null) {
return androidJarBuilder_.getMessageOrBuilder();
} else {
return androidJar_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : androidJar_;
}
}
/**
* .bazelbsp.FileLocation android_jar = 100;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getAndroidJarFieldBuilder() {
if (androidJarBuilder_ == null) {
androidJarBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getAndroidJar(),
getParentForChildren(),
isClean());
androidJar_ = null;
}
return androidJarBuilder_;
}
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation manifest_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> manifestBuilder_;
/**
* .bazelbsp.FileLocation manifest = 200;
* @return Whether the manifest field is set.
*/
public boolean hasManifest() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .bazelbsp.FileLocation manifest = 200;
* @return The manifest.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getManifest() {
if (manifestBuilder_ == null) {
return manifest_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : manifest_;
} else {
return manifestBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation manifest = 200;
*/
public Builder setManifest(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (manifestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
manifest_ = value;
} else {
manifestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation manifest = 200;
*/
public Builder setManifest(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (manifestBuilder_ == null) {
manifest_ = builderForValue.build();
} else {
manifestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation manifest = 200;
*/
public Builder mergeManifest(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (manifestBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
manifest_ != null &&
manifest_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getManifestBuilder().mergeFrom(value);
} else {
manifest_ = value;
}
} else {
manifestBuilder_.mergeFrom(value);
}
if (manifest_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation manifest = 200;
*/
public Builder clearManifest() {
bitField0_ = (bitField0_ & ~0x00000002);
manifest_ = null;
if (manifestBuilder_ != null) {
manifestBuilder_.dispose();
manifestBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation manifest = 200;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getManifestBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getManifestFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation manifest = 200;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getManifestOrBuilder() {
if (manifestBuilder_ != null) {
return manifestBuilder_.getMessageOrBuilder();
} else {
return manifest_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : manifest_;
}
}
/**
* .bazelbsp.FileLocation manifest = 200;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getManifestFieldBuilder() {
if (manifestBuilder_ == null) {
manifestBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getManifest(),
getParentForChildren(),
isClean());
manifest_ = null;
}
return manifestBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> manifestOverrides_;
private com.google.protobuf.MapField
internalGetManifestOverrides() {
if (manifestOverrides_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ManifestOverridesDefaultEntryHolder.defaultEntry);
}
return manifestOverrides_;
}
private com.google.protobuf.MapField
internalGetMutableManifestOverrides() {
if (manifestOverrides_ == null) {
manifestOverrides_ = com.google.protobuf.MapField.newMapField(
ManifestOverridesDefaultEntryHolder.defaultEntry);
}
if (!manifestOverrides_.isMutable()) {
manifestOverrides_ = manifestOverrides_.copy();
}
bitField0_ |= 0x00000004;
onChanged();
return manifestOverrides_;
}
public int getManifestOverridesCount() {
return internalGetManifestOverrides().getMap().size();
}
/**
* map<string, string> manifest_overrides = 250;
*/
@java.lang.Override
public boolean containsManifestOverrides(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetManifestOverrides().getMap().containsKey(key);
}
/**
* Use {@link #getManifestOverridesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getManifestOverrides() {
return getManifestOverridesMap();
}
/**
* map<string, string> manifest_overrides = 250;
*/
@java.lang.Override
public java.util.Map getManifestOverridesMap() {
return internalGetManifestOverrides().getMap();
}
/**
* map<string, string> manifest_overrides = 250;
*/
@java.lang.Override
public /* nullable */
java.lang.String getManifestOverridesOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetManifestOverrides().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> manifest_overrides = 250;
*/
@java.lang.Override
public java.lang.String getManifestOverridesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetManifestOverrides().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearManifestOverrides() {
bitField0_ = (bitField0_ & ~0x00000004);
internalGetMutableManifestOverrides().getMutableMap()
.clear();
return this;
}
/**
* map<string, string> manifest_overrides = 250;
*/
public Builder removeManifestOverrides(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableManifestOverrides().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableManifestOverrides() {
bitField0_ |= 0x00000004;
return internalGetMutableManifestOverrides().getMutableMap();
}
/**
* map<string, string> manifest_overrides = 250;
*/
public Builder putManifestOverrides(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableManifestOverrides().getMutableMap()
.put(key, value);
bitField0_ |= 0x00000004;
return this;
}
/**
* map<string, string> manifest_overrides = 250;
*/
public Builder putAllManifestOverrides(
java.util.Map values) {
internalGetMutableManifestOverrides().getMutableMap()
.putAll(values);
bitField0_ |= 0x00000004;
return this;
}
private java.util.List resourceDirectories_ =
java.util.Collections.emptyList();
private void ensureResourceDirectoriesIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
resourceDirectories_ = new java.util.ArrayList(resourceDirectories_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> resourceDirectoriesBuilder_;
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public java.util.List getResourceDirectoriesList() {
if (resourceDirectoriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(resourceDirectories_);
} else {
return resourceDirectoriesBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public int getResourceDirectoriesCount() {
if (resourceDirectoriesBuilder_ == null) {
return resourceDirectories_.size();
} else {
return resourceDirectoriesBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getResourceDirectories(int index) {
if (resourceDirectoriesBuilder_ == null) {
return resourceDirectories_.get(index);
} else {
return resourceDirectoriesBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public Builder setResourceDirectories(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (resourceDirectoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureResourceDirectoriesIsMutable();
resourceDirectories_.set(index, value);
onChanged();
} else {
resourceDirectoriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public Builder setResourceDirectories(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (resourceDirectoriesBuilder_ == null) {
ensureResourceDirectoriesIsMutable();
resourceDirectories_.set(index, builderForValue.build());
onChanged();
} else {
resourceDirectoriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public Builder addResourceDirectories(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (resourceDirectoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureResourceDirectoriesIsMutable();
resourceDirectories_.add(value);
onChanged();
} else {
resourceDirectoriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public Builder addResourceDirectories(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (resourceDirectoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureResourceDirectoriesIsMutable();
resourceDirectories_.add(index, value);
onChanged();
} else {
resourceDirectoriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public Builder addResourceDirectories(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (resourceDirectoriesBuilder_ == null) {
ensureResourceDirectoriesIsMutable();
resourceDirectories_.add(builderForValue.build());
onChanged();
} else {
resourceDirectoriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public Builder addResourceDirectories(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (resourceDirectoriesBuilder_ == null) {
ensureResourceDirectoriesIsMutable();
resourceDirectories_.add(index, builderForValue.build());
onChanged();
} else {
resourceDirectoriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public Builder addAllResourceDirectories(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (resourceDirectoriesBuilder_ == null) {
ensureResourceDirectoriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, resourceDirectories_);
onChanged();
} else {
resourceDirectoriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public Builder clearResourceDirectories() {
if (resourceDirectoriesBuilder_ == null) {
resourceDirectories_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
resourceDirectoriesBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public Builder removeResourceDirectories(int index) {
if (resourceDirectoriesBuilder_ == null) {
ensureResourceDirectoriesIsMutable();
resourceDirectories_.remove(index);
onChanged();
} else {
resourceDirectoriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getResourceDirectoriesBuilder(
int index) {
return getResourceDirectoriesFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getResourceDirectoriesOrBuilder(
int index) {
if (resourceDirectoriesBuilder_ == null) {
return resourceDirectories_.get(index); } else {
return resourceDirectoriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getResourceDirectoriesOrBuilderList() {
if (resourceDirectoriesBuilder_ != null) {
return resourceDirectoriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(resourceDirectories_);
}
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addResourceDirectoriesBuilder() {
return getResourceDirectoriesFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addResourceDirectoriesBuilder(
int index) {
return getResourceDirectoriesFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation resource_directories = 300;
*/
public java.util.List
getResourceDirectoriesBuilderList() {
return getResourceDirectoriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getResourceDirectoriesFieldBuilder() {
if (resourceDirectoriesBuilder_ == null) {
resourceDirectoriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
resourceDirectories_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
resourceDirectories_ = null;
}
return resourceDirectoriesBuilder_;
}
private java.lang.Object resourceJavaPackage_ = "";
/**
* string resource_java_package = 400;
* @return The resourceJavaPackage.
*/
public java.lang.String getResourceJavaPackage() {
java.lang.Object ref = resourceJavaPackage_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceJavaPackage_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string resource_java_package = 400;
* @return The bytes for resourceJavaPackage.
*/
public com.google.protobuf.ByteString
getResourceJavaPackageBytes() {
java.lang.Object ref = resourceJavaPackage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceJavaPackage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string resource_java_package = 400;
* @param value The resourceJavaPackage to set.
* @return This builder for chaining.
*/
public Builder setResourceJavaPackage(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resourceJavaPackage_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* string resource_java_package = 400;
* @return This builder for chaining.
*/
public Builder clearResourceJavaPackage() {
resourceJavaPackage_ = getDefaultInstance().getResourceJavaPackage();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* string resource_java_package = 400;
* @param value The bytes for resourceJavaPackage to set.
* @return This builder for chaining.
*/
public Builder setResourceJavaPackageBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resourceJavaPackage_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private java.util.List assetsDirectories_ =
java.util.Collections.emptyList();
private void ensureAssetsDirectoriesIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
assetsDirectories_ = new java.util.ArrayList(assetsDirectories_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> assetsDirectoriesBuilder_;
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public java.util.List getAssetsDirectoriesList() {
if (assetsDirectoriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(assetsDirectories_);
} else {
return assetsDirectoriesBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public int getAssetsDirectoriesCount() {
if (assetsDirectoriesBuilder_ == null) {
return assetsDirectories_.size();
} else {
return assetsDirectoriesBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAssetsDirectories(int index) {
if (assetsDirectoriesBuilder_ == null) {
return assetsDirectories_.get(index);
} else {
return assetsDirectoriesBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public Builder setAssetsDirectories(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (assetsDirectoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetsDirectoriesIsMutable();
assetsDirectories_.set(index, value);
onChanged();
} else {
assetsDirectoriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public Builder setAssetsDirectories(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (assetsDirectoriesBuilder_ == null) {
ensureAssetsDirectoriesIsMutable();
assetsDirectories_.set(index, builderForValue.build());
onChanged();
} else {
assetsDirectoriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public Builder addAssetsDirectories(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (assetsDirectoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetsDirectoriesIsMutable();
assetsDirectories_.add(value);
onChanged();
} else {
assetsDirectoriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public Builder addAssetsDirectories(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (assetsDirectoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAssetsDirectoriesIsMutable();
assetsDirectories_.add(index, value);
onChanged();
} else {
assetsDirectoriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public Builder addAssetsDirectories(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (assetsDirectoriesBuilder_ == null) {
ensureAssetsDirectoriesIsMutable();
assetsDirectories_.add(builderForValue.build());
onChanged();
} else {
assetsDirectoriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public Builder addAssetsDirectories(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (assetsDirectoriesBuilder_ == null) {
ensureAssetsDirectoriesIsMutable();
assetsDirectories_.add(index, builderForValue.build());
onChanged();
} else {
assetsDirectoriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public Builder addAllAssetsDirectories(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (assetsDirectoriesBuilder_ == null) {
ensureAssetsDirectoriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, assetsDirectories_);
onChanged();
} else {
assetsDirectoriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public Builder clearAssetsDirectories() {
if (assetsDirectoriesBuilder_ == null) {
assetsDirectories_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
assetsDirectoriesBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public Builder removeAssetsDirectories(int index) {
if (assetsDirectoriesBuilder_ == null) {
ensureAssetsDirectoriesIsMutable();
assetsDirectories_.remove(index);
onChanged();
} else {
assetsDirectoriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getAssetsDirectoriesBuilder(
int index) {
return getAssetsDirectoriesFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAssetsDirectoriesOrBuilder(
int index) {
if (assetsDirectoriesBuilder_ == null) {
return assetsDirectories_.get(index); } else {
return assetsDirectoriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getAssetsDirectoriesOrBuilderList() {
if (assetsDirectoriesBuilder_ != null) {
return assetsDirectoriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(assetsDirectories_);
}
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addAssetsDirectoriesBuilder() {
return getAssetsDirectoriesFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addAssetsDirectoriesBuilder(
int index) {
return getAssetsDirectoriesFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation assets_directories = 450;
*/
public java.util.List
getAssetsDirectoriesBuilderList() {
return getAssetsDirectoriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getAssetsDirectoriesFieldBuilder() {
if (assetsDirectoriesBuilder_ == null) {
assetsDirectoriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
assetsDirectories_,
((bitField0_ & 0x00000020) != 0),
getParentForChildren(),
isClean());
assetsDirectories_ = null;
}
return assetsDirectoriesBuilder_;
}
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation aidlBinaryJar_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> aidlBinaryJarBuilder_;
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
* @return Whether the aidlBinaryJar field is set.
*/
public boolean hasAidlBinaryJar() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
* @return The aidlBinaryJar.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAidlBinaryJar() {
if (aidlBinaryJarBuilder_ == null) {
return aidlBinaryJar_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : aidlBinaryJar_;
} else {
return aidlBinaryJarBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
*/
public Builder setAidlBinaryJar(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (aidlBinaryJarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
aidlBinaryJar_ = value;
} else {
aidlBinaryJarBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
*/
public Builder setAidlBinaryJar(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (aidlBinaryJarBuilder_ == null) {
aidlBinaryJar_ = builderForValue.build();
} else {
aidlBinaryJarBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
*/
public Builder mergeAidlBinaryJar(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (aidlBinaryJarBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0) &&
aidlBinaryJar_ != null &&
aidlBinaryJar_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getAidlBinaryJarBuilder().mergeFrom(value);
} else {
aidlBinaryJar_ = value;
}
} else {
aidlBinaryJarBuilder_.mergeFrom(value);
}
if (aidlBinaryJar_ != null) {
bitField0_ |= 0x00000040;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
*/
public Builder clearAidlBinaryJar() {
bitField0_ = (bitField0_ & ~0x00000040);
aidlBinaryJar_ = null;
if (aidlBinaryJarBuilder_ != null) {
aidlBinaryJarBuilder_.dispose();
aidlBinaryJarBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getAidlBinaryJarBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getAidlBinaryJarFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAidlBinaryJarOrBuilder() {
if (aidlBinaryJarBuilder_ != null) {
return aidlBinaryJarBuilder_.getMessageOrBuilder();
} else {
return aidlBinaryJar_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : aidlBinaryJar_;
}
}
/**
* .bazelbsp.FileLocation aidl_binary_jar = 500;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getAidlBinaryJarFieldBuilder() {
if (aidlBinaryJarBuilder_ == null) {
aidlBinaryJarBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getAidlBinaryJar(),
getParentForChildren(),
isClean());
aidlBinaryJar_ = null;
}
return aidlBinaryJarBuilder_;
}
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation aidlSourceJar_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> aidlSourceJarBuilder_;
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
* @return Whether the aidlSourceJar field is set.
*/
public boolean hasAidlSourceJar() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
* @return The aidlSourceJar.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getAidlSourceJar() {
if (aidlSourceJarBuilder_ == null) {
return aidlSourceJar_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : aidlSourceJar_;
} else {
return aidlSourceJarBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
*/
public Builder setAidlSourceJar(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (aidlSourceJarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
aidlSourceJar_ = value;
} else {
aidlSourceJarBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
*/
public Builder setAidlSourceJar(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (aidlSourceJarBuilder_ == null) {
aidlSourceJar_ = builderForValue.build();
} else {
aidlSourceJarBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
*/
public Builder mergeAidlSourceJar(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (aidlSourceJarBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0) &&
aidlSourceJar_ != null &&
aidlSourceJar_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getAidlSourceJarBuilder().mergeFrom(value);
} else {
aidlSourceJar_ = value;
}
} else {
aidlSourceJarBuilder_.mergeFrom(value);
}
if (aidlSourceJar_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
*/
public Builder clearAidlSourceJar() {
bitField0_ = (bitField0_ & ~0x00000080);
aidlSourceJar_ = null;
if (aidlSourceJarBuilder_ != null) {
aidlSourceJarBuilder_.dispose();
aidlSourceJarBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getAidlSourceJarBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getAidlSourceJarFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getAidlSourceJarOrBuilder() {
if (aidlSourceJarBuilder_ != null) {
return aidlSourceJarBuilder_.getMessageOrBuilder();
} else {
return aidlSourceJar_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : aidlSourceJar_;
}
}
/**
* .bazelbsp.FileLocation aidl_source_jar = 600;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getAidlSourceJarFieldBuilder() {
if (aidlSourceJarBuilder_ == null) {
aidlSourceJarBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getAidlSourceJar(),
getParentForChildren(),
isClean());
aidlSourceJar_ = null;
}
return aidlSourceJarBuilder_;
}
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation apk_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> apkBuilder_;
/**
* .bazelbsp.FileLocation apk = 700;
* @return Whether the apk field is set.
*/
public boolean hasApk() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* .bazelbsp.FileLocation apk = 700;
* @return The apk.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getApk() {
if (apkBuilder_ == null) {
return apk_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : apk_;
} else {
return apkBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation apk = 700;
*/
public Builder setApk(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (apkBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
apk_ = value;
} else {
apkBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation apk = 700;
*/
public Builder setApk(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (apkBuilder_ == null) {
apk_ = builderForValue.build();
} else {
apkBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation apk = 700;
*/
public Builder mergeApk(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (apkBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0) &&
apk_ != null &&
apk_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getApkBuilder().mergeFrom(value);
} else {
apk_ = value;
}
} else {
apkBuilder_.mergeFrom(value);
}
if (apk_ != null) {
bitField0_ |= 0x00000100;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation apk = 700;
*/
public Builder clearApk() {
bitField0_ = (bitField0_ & ~0x00000100);
apk_ = null;
if (apkBuilder_ != null) {
apkBuilder_.dispose();
apkBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation apk = 700;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getApkBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getApkFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation apk = 700;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getApkOrBuilder() {
if (apkBuilder_ != null) {
return apkBuilder_.getMessageOrBuilder();
} else {
return apk_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : apk_;
}
}
/**
* .bazelbsp.FileLocation apk = 700;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getApkFieldBuilder() {
if (apkBuilder_ == null) {
apkBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getApk(),
getParentForChildren(),
isClean());
apk_ = null;
}
return apkBuilder_;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.AndroidTargetInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.AndroidTargetInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AndroidTargetInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidTargetInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AndroidAarImportInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.AndroidAarImportInfo)
com.google.protobuf.MessageOrBuilder {
/**
* .bazelbsp.FileLocation manifest = 1;
* @return Whether the manifest field is set.
*/
boolean hasManifest();
/**
* .bazelbsp.FileLocation manifest = 1;
* @return The manifest.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getManifest();
/**
* .bazelbsp.FileLocation manifest = 1;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getManifestOrBuilder();
/**
* .bazelbsp.FileLocation resource_folder = 2;
* @return Whether the resourceFolder field is set.
*/
boolean hasResourceFolder();
/**
* .bazelbsp.FileLocation resource_folder = 2;
* @return The resourceFolder.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getResourceFolder();
/**
* .bazelbsp.FileLocation resource_folder = 2;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getResourceFolderOrBuilder();
/**
* .bazelbsp.FileLocation r_txt = 3;
* @return Whether the rTxt field is set.
*/
boolean hasRTxt();
/**
* .bazelbsp.FileLocation r_txt = 3;
* @return The rTxt.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getRTxt();
/**
* .bazelbsp.FileLocation r_txt = 3;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getRTxtOrBuilder();
}
/**
* Protobuf type {@code bazelbsp.AndroidAarImportInfo}
*/
public static final class AndroidAarImportInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.AndroidAarImportInfo)
AndroidAarImportInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
AndroidAarImportInfo.class.getName());
}
// Use AndroidAarImportInfo.newBuilder() to construct.
private AndroidAarImportInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private AndroidAarImportInfo() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_AndroidAarImportInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_AndroidAarImportInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo.Builder.class);
}
private int bitField0_;
public static final int MANIFEST_FIELD_NUMBER = 1;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation manifest_;
/**
* .bazelbsp.FileLocation manifest = 1;
* @return Whether the manifest field is set.
*/
@java.lang.Override
public boolean hasManifest() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .bazelbsp.FileLocation manifest = 1;
* @return The manifest.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getManifest() {
return manifest_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : manifest_;
}
/**
* .bazelbsp.FileLocation manifest = 1;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getManifestOrBuilder() {
return manifest_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : manifest_;
}
public static final int RESOURCE_FOLDER_FIELD_NUMBER = 2;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation resourceFolder_;
/**
* .bazelbsp.FileLocation resource_folder = 2;
* @return Whether the resourceFolder field is set.
*/
@java.lang.Override
public boolean hasResourceFolder() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .bazelbsp.FileLocation resource_folder = 2;
* @return The resourceFolder.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getResourceFolder() {
return resourceFolder_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : resourceFolder_;
}
/**
* .bazelbsp.FileLocation resource_folder = 2;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getResourceFolderOrBuilder() {
return resourceFolder_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : resourceFolder_;
}
public static final int R_TXT_FIELD_NUMBER = 3;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation rTxt_;
/**
* .bazelbsp.FileLocation r_txt = 3;
* @return Whether the rTxt field is set.
*/
@java.lang.Override
public boolean hasRTxt() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* .bazelbsp.FileLocation r_txt = 3;
* @return The rTxt.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getRTxt() {
return rTxt_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : rTxt_;
}
/**
* .bazelbsp.FileLocation r_txt = 3;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getRTxtOrBuilder() {
return rTxt_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : rTxt_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getManifest());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getResourceFolder());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(3, getRTxt());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getManifest());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getResourceFolder());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getRTxt());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo) obj;
if (hasManifest() != other.hasManifest()) return false;
if (hasManifest()) {
if (!getManifest()
.equals(other.getManifest())) return false;
}
if (hasResourceFolder() != other.hasResourceFolder()) return false;
if (hasResourceFolder()) {
if (!getResourceFolder()
.equals(other.getResourceFolder())) return false;
}
if (hasRTxt() != other.hasRTxt()) return false;
if (hasRTxt()) {
if (!getRTxt()
.equals(other.getRTxt())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasManifest()) {
hash = (37 * hash) + MANIFEST_FIELD_NUMBER;
hash = (53 * hash) + getManifest().hashCode();
}
if (hasResourceFolder()) {
hash = (37 * hash) + RESOURCE_FOLDER_FIELD_NUMBER;
hash = (53 * hash) + getResourceFolder().hashCode();
}
if (hasRTxt()) {
hash = (37 * hash) + R_TXT_FIELD_NUMBER;
hash = (53 * hash) + getRTxt().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.AndroidAarImportInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.AndroidAarImportInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_AndroidAarImportInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_AndroidAarImportInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage
.alwaysUseFieldBuilders) {
getManifestFieldBuilder();
getResourceFolderFieldBuilder();
getRTxtFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
manifest_ = null;
if (manifestBuilder_ != null) {
manifestBuilder_.dispose();
manifestBuilder_ = null;
}
resourceFolder_ = null;
if (resourceFolderBuilder_ != null) {
resourceFolderBuilder_.dispose();
resourceFolderBuilder_ = null;
}
rTxt_ = null;
if (rTxtBuilder_ != null) {
rTxtBuilder_.dispose();
rTxtBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_AndroidAarImportInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.manifest_ = manifestBuilder_ == null
? manifest_
: manifestBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.resourceFolder_ = resourceFolderBuilder_ == null
? resourceFolder_
: resourceFolderBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.rTxt_ = rTxtBuilder_ == null
? rTxt_
: rTxtBuilder_.build();
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo.getDefaultInstance()) return this;
if (other.hasManifest()) {
mergeManifest(other.getManifest());
}
if (other.hasResourceFolder()) {
mergeResourceFolder(other.getResourceFolder());
}
if (other.hasRTxt()) {
mergeRTxt(other.getRTxt());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getManifestFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getResourceFolderFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
input.readMessage(
getRTxtFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation manifest_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> manifestBuilder_;
/**
* .bazelbsp.FileLocation manifest = 1;
* @return Whether the manifest field is set.
*/
public boolean hasManifest() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .bazelbsp.FileLocation manifest = 1;
* @return The manifest.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getManifest() {
if (manifestBuilder_ == null) {
return manifest_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : manifest_;
} else {
return manifestBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation manifest = 1;
*/
public Builder setManifest(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (manifestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
manifest_ = value;
} else {
manifestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation manifest = 1;
*/
public Builder setManifest(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (manifestBuilder_ == null) {
manifest_ = builderForValue.build();
} else {
manifestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation manifest = 1;
*/
public Builder mergeManifest(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (manifestBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
manifest_ != null &&
manifest_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getManifestBuilder().mergeFrom(value);
} else {
manifest_ = value;
}
} else {
manifestBuilder_.mergeFrom(value);
}
if (manifest_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation manifest = 1;
*/
public Builder clearManifest() {
bitField0_ = (bitField0_ & ~0x00000001);
manifest_ = null;
if (manifestBuilder_ != null) {
manifestBuilder_.dispose();
manifestBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation manifest = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getManifestBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getManifestFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation manifest = 1;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getManifestOrBuilder() {
if (manifestBuilder_ != null) {
return manifestBuilder_.getMessageOrBuilder();
} else {
return manifest_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : manifest_;
}
}
/**
* .bazelbsp.FileLocation manifest = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getManifestFieldBuilder() {
if (manifestBuilder_ == null) {
manifestBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getManifest(),
getParentForChildren(),
isClean());
manifest_ = null;
}
return manifestBuilder_;
}
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation resourceFolder_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> resourceFolderBuilder_;
/**
* .bazelbsp.FileLocation resource_folder = 2;
* @return Whether the resourceFolder field is set.
*/
public boolean hasResourceFolder() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .bazelbsp.FileLocation resource_folder = 2;
* @return The resourceFolder.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getResourceFolder() {
if (resourceFolderBuilder_ == null) {
return resourceFolder_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : resourceFolder_;
} else {
return resourceFolderBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation resource_folder = 2;
*/
public Builder setResourceFolder(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (resourceFolderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resourceFolder_ = value;
} else {
resourceFolderBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation resource_folder = 2;
*/
public Builder setResourceFolder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (resourceFolderBuilder_ == null) {
resourceFolder_ = builderForValue.build();
} else {
resourceFolderBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation resource_folder = 2;
*/
public Builder mergeResourceFolder(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (resourceFolderBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
resourceFolder_ != null &&
resourceFolder_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getResourceFolderBuilder().mergeFrom(value);
} else {
resourceFolder_ = value;
}
} else {
resourceFolderBuilder_.mergeFrom(value);
}
if (resourceFolder_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation resource_folder = 2;
*/
public Builder clearResourceFolder() {
bitField0_ = (bitField0_ & ~0x00000002);
resourceFolder_ = null;
if (resourceFolderBuilder_ != null) {
resourceFolderBuilder_.dispose();
resourceFolderBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation resource_folder = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getResourceFolderBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getResourceFolderFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation resource_folder = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getResourceFolderOrBuilder() {
if (resourceFolderBuilder_ != null) {
return resourceFolderBuilder_.getMessageOrBuilder();
} else {
return resourceFolder_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : resourceFolder_;
}
}
/**
* .bazelbsp.FileLocation resource_folder = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getResourceFolderFieldBuilder() {
if (resourceFolderBuilder_ == null) {
resourceFolderBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getResourceFolder(),
getParentForChildren(),
isClean());
resourceFolder_ = null;
}
return resourceFolderBuilder_;
}
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation rTxt_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> rTxtBuilder_;
/**
* .bazelbsp.FileLocation r_txt = 3;
* @return Whether the rTxt field is set.
*/
public boolean hasRTxt() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* .bazelbsp.FileLocation r_txt = 3;
* @return The rTxt.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getRTxt() {
if (rTxtBuilder_ == null) {
return rTxt_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : rTxt_;
} else {
return rTxtBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation r_txt = 3;
*/
public Builder setRTxt(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (rTxtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rTxt_ = value;
} else {
rTxtBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation r_txt = 3;
*/
public Builder setRTxt(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (rTxtBuilder_ == null) {
rTxt_ = builderForValue.build();
} else {
rTxtBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation r_txt = 3;
*/
public Builder mergeRTxt(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (rTxtBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
rTxt_ != null &&
rTxt_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getRTxtBuilder().mergeFrom(value);
} else {
rTxt_ = value;
}
} else {
rTxtBuilder_.mergeFrom(value);
}
if (rTxt_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation r_txt = 3;
*/
public Builder clearRTxt() {
bitField0_ = (bitField0_ & ~0x00000004);
rTxt_ = null;
if (rTxtBuilder_ != null) {
rTxtBuilder_.dispose();
rTxtBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation r_txt = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getRTxtBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getRTxtFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation r_txt = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getRTxtOrBuilder() {
if (rTxtBuilder_ != null) {
return rTxtBuilder_.getMessageOrBuilder();
} else {
return rTxt_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : rTxt_;
}
}
/**
* .bazelbsp.FileLocation r_txt = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getRTxtFieldBuilder() {
if (rTxtBuilder_ == null) {
rTxtBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getRTxt(),
getParentForChildren(),
isClean());
rTxt_ = null;
}
return rTxtBuilder_;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.AndroidAarImportInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.AndroidAarImportInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AndroidAarImportInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.AndroidAarImportInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GoTargetInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.GoTargetInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string importpath = 1;
* @return The importpath.
*/
java.lang.String getImportpath();
/**
* string importpath = 1;
* @return The bytes for importpath.
*/
com.google.protobuf.ByteString
getImportpathBytes();
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
* @return Whether the sdkHomePath field is set.
*/
boolean hasSdkHomePath();
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
* @return The sdkHomePath.
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getSdkHomePath();
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getSdkHomePathOrBuilder();
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
java.util.List
getGeneratedSourcesList();
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getGeneratedSources(int index);
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
int getGeneratedSourcesCount();
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getGeneratedSourcesOrBuilderList();
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getGeneratedSourcesOrBuilder(
int index);
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
java.util.List
getGeneratedLibrariesList();
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getGeneratedLibraries(int index);
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
int getGeneratedLibrariesCount();
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getGeneratedLibrariesOrBuilderList();
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getGeneratedLibrariesOrBuilder(
int index);
}
/**
* Protobuf type {@code bazelbsp.GoTargetInfo}
*/
public static final class GoTargetInfo extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:bazelbsp.GoTargetInfo)
GoTargetInfoOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
GoTargetInfo.class.getName());
}
// Use GoTargetInfo.newBuilder() to construct.
private GoTargetInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private GoTargetInfo() {
importpath_ = "";
generatedSources_ = java.util.Collections.emptyList();
generatedLibraries_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_GoTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_GoTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo.Builder.class);
}
private int bitField0_;
public static final int IMPORTPATH_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object importpath_ = "";
/**
* string importpath = 1;
* @return The importpath.
*/
@java.lang.Override
public java.lang.String getImportpath() {
java.lang.Object ref = importpath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
importpath_ = s;
return s;
}
}
/**
* string importpath = 1;
* @return The bytes for importpath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getImportpathBytes() {
java.lang.Object ref = importpath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
importpath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SDK_HOME_PATH_FIELD_NUMBER = 2;
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation sdkHomePath_;
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
* @return Whether the sdkHomePath field is set.
*/
@java.lang.Override
public boolean hasSdkHomePath() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
* @return The sdkHomePath.
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getSdkHomePath() {
return sdkHomePath_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : sdkHomePath_;
}
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getSdkHomePathOrBuilder() {
return sdkHomePath_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : sdkHomePath_;
}
public static final int GENERATED_SOURCES_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List generatedSources_;
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
@java.lang.Override
public java.util.List getGeneratedSourcesList() {
return generatedSources_;
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getGeneratedSourcesOrBuilderList() {
return generatedSources_;
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
@java.lang.Override
public int getGeneratedSourcesCount() {
return generatedSources_.size();
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getGeneratedSources(int index) {
return generatedSources_.get(index);
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getGeneratedSourcesOrBuilder(
int index) {
return generatedSources_.get(index);
}
public static final int GENERATED_LIBRARIES_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private java.util.List generatedLibraries_;
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
@java.lang.Override
public java.util.List getGeneratedLibrariesList() {
return generatedLibraries_;
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
@java.lang.Override
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getGeneratedLibrariesOrBuilderList() {
return generatedLibraries_;
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
@java.lang.Override
public int getGeneratedLibrariesCount() {
return generatedLibraries_.size();
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getGeneratedLibraries(int index) {
return generatedLibraries_.get(index);
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getGeneratedLibrariesOrBuilder(
int index) {
return generatedLibraries_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(importpath_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, importpath_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(2, getSdkHomePath());
}
for (int i = 0; i < generatedSources_.size(); i++) {
output.writeMessage(3, generatedSources_.get(i));
}
for (int i = 0; i < generatedLibraries_.size(); i++) {
output.writeMessage(4, generatedLibraries_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(importpath_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, importpath_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getSdkHomePath());
}
for (int i = 0; i < generatedSources_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, generatedSources_.get(i));
}
for (int i = 0; i < generatedLibraries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, generatedLibraries_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo)) {
return super.equals(obj);
}
org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo other = (org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo) obj;
if (!getImportpath()
.equals(other.getImportpath())) return false;
if (hasSdkHomePath() != other.hasSdkHomePath()) return false;
if (hasSdkHomePath()) {
if (!getSdkHomePath()
.equals(other.getSdkHomePath())) return false;
}
if (!getGeneratedSourcesList()
.equals(other.getGeneratedSourcesList())) return false;
if (!getGeneratedLibrariesList()
.equals(other.getGeneratedLibrariesList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + IMPORTPATH_FIELD_NUMBER;
hash = (53 * hash) + getImportpath().hashCode();
if (hasSdkHomePath()) {
hash = (37 * hash) + SDK_HOME_PATH_FIELD_NUMBER;
hash = (53 * hash) + getSdkHomePath().hashCode();
}
if (getGeneratedSourcesCount() > 0) {
hash = (37 * hash) + GENERATED_SOURCES_FIELD_NUMBER;
hash = (53 * hash) + getGeneratedSourcesList().hashCode();
}
if (getGeneratedLibrariesCount() > 0) {
hash = (37 * hash) + GENERATED_LIBRARIES_FIELD_NUMBER;
hash = (53 * hash) + getGeneratedLibrariesList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code bazelbsp.GoTargetInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:bazelbsp.GoTargetInfo)
org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_GoTargetInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_GoTargetInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo.class, org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo.Builder.class);
}
// Construct using org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage
.alwaysUseFieldBuilders) {
getSdkHomePathFieldBuilder();
getGeneratedSourcesFieldBuilder();
getGeneratedLibrariesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
importpath_ = "";
sdkHomePath_ = null;
if (sdkHomePathBuilder_ != null) {
sdkHomePathBuilder_.dispose();
sdkHomePathBuilder_ = null;
}
if (generatedSourcesBuilder_ == null) {
generatedSources_ = java.util.Collections.emptyList();
} else {
generatedSources_ = null;
generatedSourcesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (generatedLibrariesBuilder_ == null) {
generatedLibraries_ = java.util.Collections.emptyList();
} else {
generatedLibraries_ = null;
generatedLibrariesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.internal_static_bazelbsp_GoTargetInfo_descriptor;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo getDefaultInstanceForType() {
return org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo.getDefaultInstance();
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo build() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo buildPartial() {
org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo result = new org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo result) {
if (generatedSourcesBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
generatedSources_ = java.util.Collections.unmodifiableList(generatedSources_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.generatedSources_ = generatedSources_;
} else {
result.generatedSources_ = generatedSourcesBuilder_.build();
}
if (generatedLibrariesBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
generatedLibraries_ = java.util.Collections.unmodifiableList(generatedLibraries_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.generatedLibraries_ = generatedLibraries_;
} else {
result.generatedLibraries_ = generatedLibrariesBuilder_.build();
}
}
private void buildPartial0(org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.importpath_ = importpath_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.sdkHomePath_ = sdkHomePathBuilder_ == null
? sdkHomePath_
: sdkHomePathBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo) {
return mergeFrom((org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo other) {
if (other == org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo.getDefaultInstance()) return this;
if (!other.getImportpath().isEmpty()) {
importpath_ = other.importpath_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasSdkHomePath()) {
mergeSdkHomePath(other.getSdkHomePath());
}
if (generatedSourcesBuilder_ == null) {
if (!other.generatedSources_.isEmpty()) {
if (generatedSources_.isEmpty()) {
generatedSources_ = other.generatedSources_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureGeneratedSourcesIsMutable();
generatedSources_.addAll(other.generatedSources_);
}
onChanged();
}
} else {
if (!other.generatedSources_.isEmpty()) {
if (generatedSourcesBuilder_.isEmpty()) {
generatedSourcesBuilder_.dispose();
generatedSourcesBuilder_ = null;
generatedSources_ = other.generatedSources_;
bitField0_ = (bitField0_ & ~0x00000004);
generatedSourcesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getGeneratedSourcesFieldBuilder() : null;
} else {
generatedSourcesBuilder_.addAllMessages(other.generatedSources_);
}
}
}
if (generatedLibrariesBuilder_ == null) {
if (!other.generatedLibraries_.isEmpty()) {
if (generatedLibraries_.isEmpty()) {
generatedLibraries_ = other.generatedLibraries_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureGeneratedLibrariesIsMutable();
generatedLibraries_.addAll(other.generatedLibraries_);
}
onChanged();
}
} else {
if (!other.generatedLibraries_.isEmpty()) {
if (generatedLibrariesBuilder_.isEmpty()) {
generatedLibrariesBuilder_.dispose();
generatedLibrariesBuilder_ = null;
generatedLibraries_ = other.generatedLibraries_;
bitField0_ = (bitField0_ & ~0x00000008);
generatedLibrariesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getGeneratedLibrariesFieldBuilder() : null;
} else {
generatedLibrariesBuilder_.addAllMessages(other.generatedLibraries_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
importpath_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getSdkHomePathFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (generatedSourcesBuilder_ == null) {
ensureGeneratedSourcesIsMutable();
generatedSources_.add(m);
} else {
generatedSourcesBuilder_.addMessage(m);
}
break;
} // case 26
case 34: {
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation m =
input.readMessage(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.parser(),
extensionRegistry);
if (generatedLibrariesBuilder_ == null) {
ensureGeneratedLibrariesIsMutable();
generatedLibraries_.add(m);
} else {
generatedLibrariesBuilder_.addMessage(m);
}
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object importpath_ = "";
/**
* string importpath = 1;
* @return The importpath.
*/
public java.lang.String getImportpath() {
java.lang.Object ref = importpath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
importpath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string importpath = 1;
* @return The bytes for importpath.
*/
public com.google.protobuf.ByteString
getImportpathBytes() {
java.lang.Object ref = importpath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
importpath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string importpath = 1;
* @param value The importpath to set.
* @return This builder for chaining.
*/
public Builder setImportpath(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
importpath_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string importpath = 1;
* @return This builder for chaining.
*/
public Builder clearImportpath() {
importpath_ = getDefaultInstance().getImportpath();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string importpath = 1;
* @param value The bytes for importpath to set.
* @return This builder for chaining.
*/
public Builder setImportpathBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
importpath_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation sdkHomePath_;
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> sdkHomePathBuilder_;
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
* @return Whether the sdkHomePath field is set.
*/
public boolean hasSdkHomePath() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
* @return The sdkHomePath.
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getSdkHomePath() {
if (sdkHomePathBuilder_ == null) {
return sdkHomePath_ == null ? org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : sdkHomePath_;
} else {
return sdkHomePathBuilder_.getMessage();
}
}
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
*/
public Builder setSdkHomePath(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (sdkHomePathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sdkHomePath_ = value;
} else {
sdkHomePathBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
*/
public Builder setSdkHomePath(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (sdkHomePathBuilder_ == null) {
sdkHomePath_ = builderForValue.build();
} else {
sdkHomePathBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
*/
public Builder mergeSdkHomePath(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (sdkHomePathBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
sdkHomePath_ != null &&
sdkHomePath_ != org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance()) {
getSdkHomePathBuilder().mergeFrom(value);
} else {
sdkHomePath_ = value;
}
} else {
sdkHomePathBuilder_.mergeFrom(value);
}
if (sdkHomePath_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
*/
public Builder clearSdkHomePath() {
bitField0_ = (bitField0_ & ~0x00000002);
sdkHomePath_ = null;
if (sdkHomePathBuilder_ != null) {
sdkHomePathBuilder_.dispose();
sdkHomePathBuilder_ = null;
}
onChanged();
return this;
}
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getSdkHomePathBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getSdkHomePathFieldBuilder().getBuilder();
}
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getSdkHomePathOrBuilder() {
if (sdkHomePathBuilder_ != null) {
return sdkHomePathBuilder_.getMessageOrBuilder();
} else {
return sdkHomePath_ == null ?
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance() : sdkHomePath_;
}
}
/**
* .bazelbsp.FileLocation sdk_home_path = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getSdkHomePathFieldBuilder() {
if (sdkHomePathBuilder_ == null) {
sdkHomePathBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
getSdkHomePath(),
getParentForChildren(),
isClean());
sdkHomePath_ = null;
}
return sdkHomePathBuilder_;
}
private java.util.List generatedSources_ =
java.util.Collections.emptyList();
private void ensureGeneratedSourcesIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
generatedSources_ = new java.util.ArrayList(generatedSources_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> generatedSourcesBuilder_;
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public java.util.List getGeneratedSourcesList() {
if (generatedSourcesBuilder_ == null) {
return java.util.Collections.unmodifiableList(generatedSources_);
} else {
return generatedSourcesBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public int getGeneratedSourcesCount() {
if (generatedSourcesBuilder_ == null) {
return generatedSources_.size();
} else {
return generatedSourcesBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getGeneratedSources(int index) {
if (generatedSourcesBuilder_ == null) {
return generatedSources_.get(index);
} else {
return generatedSourcesBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public Builder setGeneratedSources(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (generatedSourcesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneratedSourcesIsMutable();
generatedSources_.set(index, value);
onChanged();
} else {
generatedSourcesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public Builder setGeneratedSources(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (generatedSourcesBuilder_ == null) {
ensureGeneratedSourcesIsMutable();
generatedSources_.set(index, builderForValue.build());
onChanged();
} else {
generatedSourcesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public Builder addGeneratedSources(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (generatedSourcesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneratedSourcesIsMutable();
generatedSources_.add(value);
onChanged();
} else {
generatedSourcesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public Builder addGeneratedSources(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (generatedSourcesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneratedSourcesIsMutable();
generatedSources_.add(index, value);
onChanged();
} else {
generatedSourcesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public Builder addGeneratedSources(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (generatedSourcesBuilder_ == null) {
ensureGeneratedSourcesIsMutable();
generatedSources_.add(builderForValue.build());
onChanged();
} else {
generatedSourcesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public Builder addGeneratedSources(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (generatedSourcesBuilder_ == null) {
ensureGeneratedSourcesIsMutable();
generatedSources_.add(index, builderForValue.build());
onChanged();
} else {
generatedSourcesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public Builder addAllGeneratedSources(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (generatedSourcesBuilder_ == null) {
ensureGeneratedSourcesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, generatedSources_);
onChanged();
} else {
generatedSourcesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public Builder clearGeneratedSources() {
if (generatedSourcesBuilder_ == null) {
generatedSources_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
generatedSourcesBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public Builder removeGeneratedSources(int index) {
if (generatedSourcesBuilder_ == null) {
ensureGeneratedSourcesIsMutable();
generatedSources_.remove(index);
onChanged();
} else {
generatedSourcesBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getGeneratedSourcesBuilder(
int index) {
return getGeneratedSourcesFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getGeneratedSourcesOrBuilder(
int index) {
if (generatedSourcesBuilder_ == null) {
return generatedSources_.get(index); } else {
return generatedSourcesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getGeneratedSourcesOrBuilderList() {
if (generatedSourcesBuilder_ != null) {
return generatedSourcesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(generatedSources_);
}
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addGeneratedSourcesBuilder() {
return getGeneratedSourcesFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addGeneratedSourcesBuilder(
int index) {
return getGeneratedSourcesFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation generated_sources = 3;
*/
public java.util.List
getGeneratedSourcesBuilderList() {
return getGeneratedSourcesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getGeneratedSourcesFieldBuilder() {
if (generatedSourcesBuilder_ == null) {
generatedSourcesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
generatedSources_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
generatedSources_ = null;
}
return generatedSourcesBuilder_;
}
private java.util.List generatedLibraries_ =
java.util.Collections.emptyList();
private void ensureGeneratedLibrariesIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
generatedLibraries_ = new java.util.ArrayList(generatedLibraries_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder> generatedLibrariesBuilder_;
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public java.util.List getGeneratedLibrariesList() {
if (generatedLibrariesBuilder_ == null) {
return java.util.Collections.unmodifiableList(generatedLibraries_);
} else {
return generatedLibrariesBuilder_.getMessageList();
}
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public int getGeneratedLibrariesCount() {
if (generatedLibrariesBuilder_ == null) {
return generatedLibraries_.size();
} else {
return generatedLibrariesBuilder_.getCount();
}
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getGeneratedLibraries(int index) {
if (generatedLibrariesBuilder_ == null) {
return generatedLibraries_.get(index);
} else {
return generatedLibrariesBuilder_.getMessage(index);
}
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public Builder setGeneratedLibraries(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (generatedLibrariesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneratedLibrariesIsMutable();
generatedLibraries_.set(index, value);
onChanged();
} else {
generatedLibrariesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public Builder setGeneratedLibraries(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (generatedLibrariesBuilder_ == null) {
ensureGeneratedLibrariesIsMutable();
generatedLibraries_.set(index, builderForValue.build());
onChanged();
} else {
generatedLibrariesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public Builder addGeneratedLibraries(org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (generatedLibrariesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneratedLibrariesIsMutable();
generatedLibraries_.add(value);
onChanged();
} else {
generatedLibrariesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public Builder addGeneratedLibraries(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation value) {
if (generatedLibrariesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneratedLibrariesIsMutable();
generatedLibraries_.add(index, value);
onChanged();
} else {
generatedLibrariesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public Builder addGeneratedLibraries(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (generatedLibrariesBuilder_ == null) {
ensureGeneratedLibrariesIsMutable();
generatedLibraries_.add(builderForValue.build());
onChanged();
} else {
generatedLibrariesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public Builder addGeneratedLibraries(
int index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder builderForValue) {
if (generatedLibrariesBuilder_ == null) {
ensureGeneratedLibrariesIsMutable();
generatedLibraries_.add(index, builderForValue.build());
onChanged();
} else {
generatedLibrariesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public Builder addAllGeneratedLibraries(
java.lang.Iterable extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation> values) {
if (generatedLibrariesBuilder_ == null) {
ensureGeneratedLibrariesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, generatedLibraries_);
onChanged();
} else {
generatedLibrariesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public Builder clearGeneratedLibraries() {
if (generatedLibrariesBuilder_ == null) {
generatedLibraries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
generatedLibrariesBuilder_.clear();
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public Builder removeGeneratedLibraries(int index) {
if (generatedLibrariesBuilder_ == null) {
ensureGeneratedLibrariesIsMutable();
generatedLibraries_.remove(index);
onChanged();
} else {
generatedLibrariesBuilder_.remove(index);
}
return this;
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder getGeneratedLibrariesBuilder(
int index) {
return getGeneratedLibrariesFieldBuilder().getBuilder(index);
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getGeneratedLibrariesOrBuilder(
int index) {
if (generatedLibrariesBuilder_ == null) {
return generatedLibraries_.get(index); } else {
return generatedLibrariesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getGeneratedLibrariesOrBuilderList() {
if (generatedLibrariesBuilder_ != null) {
return generatedLibrariesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(generatedLibraries_);
}
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addGeneratedLibrariesBuilder() {
return getGeneratedLibrariesFieldBuilder().addBuilder(
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder addGeneratedLibrariesBuilder(
int index) {
return getGeneratedLibrariesFieldBuilder().addBuilder(
index, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.getDefaultInstance());
}
/**
* repeated .bazelbsp.FileLocation generated_libraries = 4;
*/
public java.util.List
getGeneratedLibrariesBuilderList() {
return getGeneratedLibrariesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getGeneratedLibrariesFieldBuilder() {
if (generatedLibrariesBuilder_ == null) {
generatedLibrariesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation.Builder, org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>(
generatedLibraries_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
generatedLibraries_ = null;
}
return generatedLibrariesBuilder_;
}
// @@protoc_insertion_point(builder_scope:bazelbsp.GoTargetInfo)
}
// @@protoc_insertion_point(class_scope:bazelbsp.GoTargetInfo)
private static final org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo();
}
public static org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GoTargetInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.jetbrains.bsp.bazel.info.BspTargetInfo.GoTargetInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TargetInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:bazelbsp.TargetInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string id = 10;
* @return The id.
*/
java.lang.String getId();
/**
* string id = 10;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* string kind = 20;
* @return The kind.
*/
java.lang.String getKind();
/**
* string kind = 20;
* @return The bytes for kind.
*/
com.google.protobuf.ByteString
getKindBytes();
/**
* repeated string tags = 30;
* @return A list containing the tags.
*/
java.util.List
getTagsList();
/**
* repeated string tags = 30;
* @return The count of tags.
*/
int getTagsCount();
/**
* repeated string tags = 30;
* @param index The index of the element to return.
* @return The tags at the given index.
*/
java.lang.String getTags(int index);
/**
* repeated string tags = 30;
* @param index The index of the value to return.
* @return The bytes of the tags at the given index.
*/
com.google.protobuf.ByteString
getTagsBytes(int index);
/**
* repeated .bazelbsp.Dependency dependencies = 40;
*/
java.util.List
getDependenciesList();
/**
* repeated .bazelbsp.Dependency dependencies = 40;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.Dependency getDependencies(int index);
/**
* repeated .bazelbsp.Dependency dependencies = 40;
*/
int getDependenciesCount();
/**
* repeated .bazelbsp.Dependency dependencies = 40;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.DependencyOrBuilder>
getDependenciesOrBuilderList();
/**
* repeated .bazelbsp.Dependency dependencies = 40;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.DependencyOrBuilder getDependenciesOrBuilder(
int index);
/**
* repeated .bazelbsp.FileLocation sources = 50;
*/
java.util.List
getSourcesList();
/**
* repeated .bazelbsp.FileLocation sources = 50;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocation getSources(int index);
/**
* repeated .bazelbsp.FileLocation sources = 50;
*/
int getSourcesCount();
/**
* repeated .bazelbsp.FileLocation sources = 50;
*/
java.util.List extends org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder>
getSourcesOrBuilderList();
/**
* repeated .bazelbsp.FileLocation sources = 50;
*/
org.jetbrains.bsp.bazel.info.BspTargetInfo.FileLocationOrBuilder getSourcesOrBuilder(
int index);
/**
* repeated .bazelbsp.FileLocation generated_sources = 51;
*/
java.util.List
getGeneratedSourcesList();
/**
*