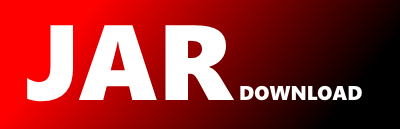
commonMain.org.jetbrains.compose.resources.LoadState.kt Maven / Gradle / Ivy
package org.jetbrains.compose.resources
import androidx.compose.runtime.Composable
import androidx.compose.runtime.LaunchedEffect
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
/**
* Represents the load state of [T].
*/
sealed class LoadState {
class Loading : LoadState()
data class Success(val value: T) : LoadState()
data class Error(val exception: Exception) : LoadState()
}
/**
* Load an item with type [T] asynchronously, and notify the caller about the load state.
* Whenever the load state changes (for example it succeeds or fails), the caller will be recomposed with the new state.
* The load will be cancelled when the [load] leaves the composition.
*/
@Composable
fun load(load: suspend () -> T): LoadState {
return load(Unit, load)
}
/**
* Load an item with type [T] asynchronously. Returns null while loading or if the load has failed.
* Whenever the result changes, the caller will be recomposed with the new value.
* The load will be cancelled when the [loadOrNull] leaves the composition.
*/
@Composable
fun loadOrNull(load: suspend () -> T): T? {
return loadOrNull(Unit, load)
}
/**
* Load an item with type [T] asynchronously, and notify the caller about the load state.
* Whenever the load state changes (for example it succeeds or fails), the caller will be recomposed with the new state.
* The load will be cancelled and re-launched when [load] is recomposed with a different [key1].
* The load will be cancelled when the [load] leaves the composition.
*/
@Composable
fun load(key1: Any?, load: suspend () -> T): LoadState {
return load(key1, Unit, load)
}
/**
* Load an item with type [T] asynchronously. Returns null while loading or if the load has failed.
* Whenever the result changes, the caller will be recomposed with the new value.
* The load will be cancelled and re-launched when [loadOrNull] is recomposed with a different [key1].
* The load will be cancelled when the [loadOrNull] leaves the composition.
*/
@Composable
fun loadOrNull(key1: Any?, load: suspend () -> T): T? {
return loadOrNull(key1, Unit, load)
}
/**
* Load an item with type [T] asynchronously, and notify the caller about the load state.
* Whenever the load state changes (for example it succeeds or fails), the caller will be recomposed with the new state.
* The load will be cancelled and re-launched when [load] is recomposed with a different [key1] or [key2].
* The load will be cancelled when the [load] leaves the composition.
*/
@Composable
fun load(key1: Any?, key2: Any?, load: suspend () -> T): LoadState {
return load(key1, key2, Unit, load)
}
/**
* Load an item with type [T] asynchronously. Returns null while loading or if the load has failed.
* Whenever the result changes, the caller will be recomposed with the new value.
* The load will be cancelled and re-launched when [loadOrNull] is recomposed with a different [key1] or [key2].
* The load will be cancelled when the [loadOrNull] leaves the composition.
*/
@Composable
fun loadOrNull(key1: Any?, key2: Any?, load: suspend () -> T): T? {
return loadOrNull(key1, key2, Unit, load)
}
/**
* Load an item with type [T] asynchronously, and notify the caller about the load state.
* Whenever the load state changes (for example it succeeds or fails), the caller will be recomposed with the new state.
* The load will be cancelled and re-launched when [load] is recomposed with a different [key1], [key2] or [key3].
* The load will be cancelled when the [load] leaves the composition.
*/
@Composable
fun load(key1: Any?, key2: Any?, key3: Any?, load: suspend () -> T): LoadState {
var state: LoadState by remember(key1, key2, key3) { mutableStateOf(LoadState.Loading()) }
LaunchedEffect(key1, key2, key3) {
state = try {
LoadState.Success(load())
} catch (e: Exception) {
LoadState.Error(e)
}
}
return state
}
/**
* Load an item with type [T] asynchronously. Returns null while loading or if the load has failed..
* Whenever the result changes, the caller will be recomposed with the new value.
* The load will be cancelled and re-launched when [loadOrNull] is recomposed with a different [key1], [key2] or [key3].
* The load will be cancelled when the [loadOrNull] leaves the composition.
*/
@Composable
fun loadOrNull(key1: Any?, key2: Any?, key3: Any?, load: suspend () -> T): T? {
val state = load(key1, key2, key3, load)
return (state as? LoadState.Success)?.value
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy