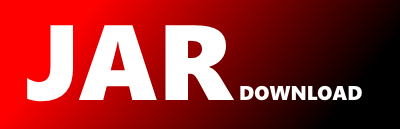
model.Documentable.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dokka-core Show documentation
Show all versions of dokka-core Show documentation
Dokka is an API documentation engine for Kotlin and Java, performing the same function as Javadoc for Java
package org.jetbrains.dokka.model
import org.jetbrains.dokka.DokkaConfiguration.DokkaSourceSet
import org.jetbrains.dokka.links.DRI
import org.jetbrains.dokka.model.doc.DocumentationNode
import org.jetbrains.dokka.model.properties.PropertyContainer
import org.jetbrains.dokka.model.properties.WithExtraProperties
interface AnnotationTarget
abstract class Documentable : WithChildren,
AnnotationTarget {
abstract val name: String?
abstract val dri: DRI
abstract val documentation: SourceSetDependent
abstract val sourceSets: Set
abstract val expectPresentInSet: DokkaSourceSet?
abstract override val children: List
override fun toString(): String =
"${javaClass.simpleName}($dri)"
override fun equals(other: Any?) =
other is Documentable && this.dri == other.dri // TODO: https://github.com/Kotlin/dokka/pull/667#discussion_r382555806
override fun hashCode() = dri.hashCode()
}
typealias SourceSetDependent = Map
interface WithSources {
val sources: SourceSetDependent
}
interface WithScope {
val functions: List
val properties: List
val classlikes: List
}
interface WithVisibility {
val visibility: SourceSetDependent
}
interface WithType {
val type: Bound
}
interface WithAbstraction {
val modifier: SourceSetDependent
}
sealed class Modifier(val name: String)
sealed class KotlinModifier(name: String) : Modifier(name) {
object Abstract : KotlinModifier("abstract")
object Open : KotlinModifier("open")
object Final : KotlinModifier("final")
object Sealed : KotlinModifier("sealed")
object Empty : KotlinModifier("")
}
sealed class JavaModifier(name: String) : Modifier(name) {
object Abstract : JavaModifier("abstract")
object Final : JavaModifier("final")
object Empty : JavaModifier("")
}
interface WithCompanion {
val companion: DObject?
}
interface WithConstructors {
val constructors: List
}
interface WithGenerics {
val generics: List
}
interface WithSupertypes {
val supertypes: SourceSetDependent>
}
interface WithIsExpectActual {
val isExpectActual: Boolean
}
interface Callable : WithVisibility, WithType, WithAbstraction, WithSources, WithIsExpectActual {
val receiver: DParameter?
}
sealed class DClasslike : Documentable(), WithScope, WithVisibility, WithSources, WithIsExpectActual
data class DModule(
override val name: String,
val packages: List,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet? = null,
override val sourceSets: Set,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Documentable(), WithExtraProperties {
override val dri: DRI = DRI.topLevel
override val children: List
get() = packages
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
data class DPackage(
override val dri: DRI,
override val functions: List,
override val properties: List,
override val classlikes: List,
val typealiases: List,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet? = null,
override val sourceSets: Set,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Documentable(), WithScope, WithExtraProperties {
val packageName: String = dri.packageName.orEmpty()
/**
* !!! WARNING !!!
* This name is not guaranteed to be a be a canonical/real package name.
* e.g. this will return a human readable version for root packages.
* Use [packageName] or `dri.packageName` instead to obtain the real packageName
*/
override val name: String = packageName.ifBlank { "[root]" }
override val children: List = properties + functions + classlikes + typealiases
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
data class DClass(
override val dri: DRI,
override val name: String,
override val constructors: List,
override val functions: List,
override val properties: List,
override val classlikes: List,
override val sources: SourceSetDependent,
override val visibility: SourceSetDependent,
override val companion: DObject?,
override val generics: List,
override val supertypes: SourceSetDependent>,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet?,
override val modifier: SourceSetDependent,
override val sourceSets: Set,
override val isExpectActual: Boolean,
override val extra: PropertyContainer = PropertyContainer.empty()
) : DClasslike(), WithAbstraction, WithCompanion, WithConstructors, WithGenerics, WithSupertypes,
WithExtraProperties {
override val children: List
get() = (functions + properties + classlikes + constructors)
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
data class DEnum(
override val dri: DRI,
override val name: String,
val entries: List,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet?,
override val sources: SourceSetDependent,
override val functions: List,
override val properties: List,
override val classlikes: List,
override val visibility: SourceSetDependent,
override val companion: DObject?,
override val constructors: List,
override val supertypes: SourceSetDependent>,
override val sourceSets: Set,
override val isExpectActual: Boolean,
override val extra: PropertyContainer = PropertyContainer.empty()
) : DClasslike(), WithCompanion, WithConstructors, WithSupertypes, WithExtraProperties {
override val children: List
get() = (entries + functions + properties + classlikes + constructors)
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
data class DEnumEntry(
override val dri: DRI,
override val name: String,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet?,
override val functions: List,
override val properties: List,
override val classlikes: List,
override val sourceSets: Set,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Documentable(), WithScope, WithExtraProperties {
override val children: List
get() = (functions + properties + classlikes)
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
data class DFunction(
override val dri: DRI,
override val name: String,
val isConstructor: Boolean,
val parameters: List,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet?,
override val sources: SourceSetDependent,
override val visibility: SourceSetDependent,
override val type: Bound,
override val generics: List,
override val receiver: DParameter?,
override val modifier: SourceSetDependent,
override val sourceSets: Set,
override val isExpectActual: Boolean,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Documentable(), Callable, WithGenerics, WithExtraProperties {
override val children: List
get() = parameters
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
data class DInterface(
override val dri: DRI,
override val name: String,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet?,
override val sources: SourceSetDependent,
override val functions: List,
override val properties: List,
override val classlikes: List,
override val visibility: SourceSetDependent,
override val companion: DObject?,
override val generics: List,
override val supertypes: SourceSetDependent>,
override val sourceSets: Set,
override val isExpectActual: Boolean,
override val extra: PropertyContainer = PropertyContainer.empty()
) : DClasslike(), WithCompanion, WithGenerics, WithSupertypes, WithExtraProperties {
override val children: List
get() = (functions + properties + classlikes)
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
data class DObject(
override val name: String?,
override val dri: DRI,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet?,
override val sources: SourceSetDependent,
override val functions: List,
override val properties: List,
override val classlikes: List,
override val visibility: SourceSetDependent,
override val supertypes: SourceSetDependent>,
override val sourceSets: Set,
override val isExpectActual: Boolean,
override val extra: PropertyContainer = PropertyContainer.empty()
) : DClasslike(), WithSupertypes, WithExtraProperties {
override val children: List
get() = (functions + properties + classlikes)
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
data class DAnnotation(
override val name: String,
override val dri: DRI,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet?,
override val sources: SourceSetDependent,
override val functions: List,
override val properties: List,
override val classlikes: List,
override val visibility: SourceSetDependent,
override val companion: DObject?,
override val constructors: List,
override val generics: List,
override val sourceSets: Set,
override val isExpectActual: Boolean,
override val extra: PropertyContainer = PropertyContainer.empty()
) : DClasslike(), WithCompanion, WithConstructors, WithExtraProperties, WithGenerics {
override val children: List
get() = (functions + properties + classlikes + constructors)
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
data class DProperty(
override val dri: DRI,
override val name: String,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet?,
override val sources: SourceSetDependent,
override val visibility: SourceSetDependent,
override val type: Bound,
override val receiver: DParameter?,
val setter: DFunction?,
val getter: DFunction?,
override val modifier: SourceSetDependent,
override val sourceSets: Set,
override val generics: List,
override val isExpectActual: Boolean,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Documentable(), Callable, WithExtraProperties, WithGenerics {
override val children: List
get() = emptyList()
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
// TODO: treat named Parameters and receivers differently
data class DParameter(
override val dri: DRI,
override val name: String?,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet?,
override val type: Bound,
override val sourceSets: Set,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Documentable(), WithExtraProperties, WithType {
override val children: List
get() = emptyList()
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
data class DTypeParameter(
val variantTypeParameter: Variance,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet?,
val bounds: List,
override val sourceSets: Set,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Documentable(), WithExtraProperties {
constructor(
dri: DRI,
name: String,
presentableName: String?,
documentation: SourceSetDependent,
expectPresentInSet: DokkaSourceSet?,
bounds: List,
sourceSets: Set,
extra: PropertyContainer = PropertyContainer.empty()
) : this(
Invariance(TypeParameter(dri, name, presentableName)),
documentation,
expectPresentInSet,
bounds,
sourceSets,
extra
)
override val dri: DRI by variantTypeParameter.inner::dri
override val name: String by variantTypeParameter.inner::name
override val children: List
get() = emptyList()
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
data class DTypeAlias(
override val dri: DRI,
override val name: String,
override val type: Bound,
val underlyingType: SourceSetDependent,
override val visibility: SourceSetDependent,
override val documentation: SourceSetDependent,
override val expectPresentInSet: DokkaSourceSet?,
override val sourceSets: Set,
override val generics: List,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Documentable(), WithType, WithVisibility, WithExtraProperties, WithGenerics {
override val children: List
get() = emptyList()
override fun withNewExtras(newExtras: PropertyContainer) = copy(extra = newExtras)
}
sealed class Projection
sealed class Bound : Projection()
data class TypeParameter(
val dri: DRI,
val name: String,
val presentableName: String? = null,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Bound(), AnnotationTarget, WithExtraProperties {
override fun withNewExtras(newExtras: PropertyContainer): TypeParameter =
copy(extra = extra)
}
sealed class TypeConstructor : Bound(), AnnotationTarget {
abstract val dri: DRI
abstract val projections: List
abstract val presentableName: String?
}
data class GenericTypeConstructor(
override val dri: DRI,
override val projections: List,
override val presentableName: String? = null,
override val extra: PropertyContainer = PropertyContainer.empty()
) : TypeConstructor(), WithExtraProperties {
override fun withNewExtras(newExtras: PropertyContainer): GenericTypeConstructor =
copy(extra = newExtras)
}
data class FunctionalTypeConstructor(
override val dri: DRI,
override val projections: List,
val isExtensionFunction: Boolean = false,
val isSuspendable: Boolean = false,
override val presentableName: String? = null,
override val extra: PropertyContainer = PropertyContainer.empty(),
) : TypeConstructor(), WithExtraProperties {
override fun withNewExtras(newExtras: PropertyContainer): FunctionalTypeConstructor =
copy(extra = newExtras)
}
// kotlin.annotation.AnnotationTarget.TYPEALIAS
data class TypeAliased(
val typeAlias: Bound,
val inner: Bound,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Bound(), AnnotationTarget, WithExtraProperties {
override fun withNewExtras(newExtras: PropertyContainer): TypeAliased =
copy(extra = newExtras)
}
data class PrimitiveJavaType(
val name: String,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Bound(), AnnotationTarget, WithExtraProperties {
override fun withNewExtras(newExtras: PropertyContainer): PrimitiveJavaType =
copy(extra = newExtras)
}
data class JavaObject(override val extra: PropertyContainer = PropertyContainer.empty()) :
Bound(), AnnotationTarget, WithExtraProperties {
override fun withNewExtras(newExtras: PropertyContainer): JavaObject =
copy(extra = newExtras)
}
data class UnresolvedBound(
val name: String,
override val extra: PropertyContainer = PropertyContainer.empty()
) : Bound(), AnnotationTarget, WithExtraProperties {
override fun withNewExtras(newExtras: PropertyContainer): UnresolvedBound =
copy(extra = newExtras)
}
// The following Projections are not AnnotationTargets; they cannot be annotated.
data class Nullable(val inner: Bound) : Bound()
/**
* It introduces [definitely non-nullable types](https://github.com/Kotlin/KEEP/blob/c72601cf35c1e95a541bb4b230edb474a6d1d1a8/proposals/definitely-non-nullable-types.md)
*/
data class DefinitelyNonNullable(val inner: Bound) : Bound()
sealed class Variance : Projection() {
abstract val inner: T
}
data class Covariance(override val inner: T) : Variance() {
override fun toString() = "out"
}
data class Contravariance(override val inner: T) : Variance() {
override fun toString() = "in"
}
data class Invariance(override val inner: T) : Variance() {
override fun toString() = ""
}
object Star : Projection()
object Void : Bound()
object Dynamic : Bound()
fun Variance.withDri(dri: DRI) = when (this) {
is Contravariance -> Contravariance(TypeParameter(dri, inner.name, inner.presentableName))
is Covariance -> Covariance(TypeParameter(dri, inner.name, inner.presentableName))
is Invariance -> Invariance(TypeParameter(dri, inner.name, inner.presentableName))
}
fun Documentable.dfs(predicate: (Documentable) -> Boolean): Documentable? =
if (predicate(this)) {
this
} else {
this.children.asSequence().mapNotNull { it.dfs(predicate) }.firstOrNull()
}
sealed class Visibility(val name: String)
sealed class KotlinVisibility(name: String) : Visibility(name) {
object Public : KotlinVisibility("public")
object Private : KotlinVisibility("private")
object Protected : KotlinVisibility("protected")
object Internal : KotlinVisibility("internal")
}
sealed class JavaVisibility(name: String) : Visibility(name) {
object Public : JavaVisibility("public")
object Private : JavaVisibility("private")
object Protected : JavaVisibility("protected")
object Default : JavaVisibility("")
}
fun SourceSetDependent?.orEmpty(): SourceSetDependent = this ?: emptyMap()
interface DocumentableSource {
val path: String
}
data class TypeConstructorWithKind(val typeConstructor: TypeConstructor, val kind: ClassKind)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy