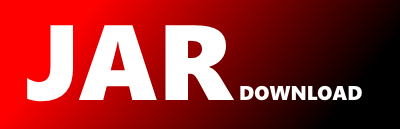
org.jetbrains.exposed.sql.kotlin.datetime.KotlinDateFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of exposed-kotlin-datetime Show documentation
Show all versions of exposed-kotlin-datetime Show documentation
Exposed, an ORM framework for Kotlin
@file:Suppress("FunctionName")
package org.jetbrains.exposed.sql.kotlin.datetime
import kotlinx.datetime.Instant
import kotlinx.datetime.LocalDate
import kotlinx.datetime.LocalDateTime
import kotlinx.datetime.LocalTime
import org.jetbrains.exposed.sql.*
import org.jetbrains.exposed.sql.Function
import org.jetbrains.exposed.sql.vendors.H2Dialect
import org.jetbrains.exposed.sql.vendors.MysqlDialect
import org.jetbrains.exposed.sql.vendors.SQLServerDialect
import org.jetbrains.exposed.sql.vendors.currentDialect
import org.jetbrains.exposed.sql.vendors.h2Mode
import java.time.OffsetDateTime
import kotlin.time.Duration
internal class DateInternal(val expr: Expression<*>) : Function(KotlinLocalDateColumnType.INSTANCE) {
override fun toQueryBuilder(queryBuilder: QueryBuilder) = queryBuilder { append("DATE(", expr, ")") }
}
/** Represents an SQL function that extracts the date part from a given [expr]. */
@JvmName("LocalDateDateFunction")
fun Date(expr: Expression): Function = DateInternal(expr)
/** Represents an SQL function that extracts the date part from a given datetime [expr]. */
@JvmName("LocalDateTimeDateFunction")
fun Date(expr: Expression): Function = DateInternal(expr)
/** Represents an SQL function that extracts the date part from a given timestamp [expr]. */
@JvmName("InstantDateFunction")
fun Date(expr: Expression): Function = DateInternal(expr)
internal class TimeFunction(val expr: Expression<*>) : Function(KotlinLocalTimeColumnType.INSTANCE) {
override fun toQueryBuilder(queryBuilder: QueryBuilder) = queryBuilder { append("Time(", expr, ")") }
}
/** Represents an SQL function that extracts the time part from a given [expr]. */
@JvmName("LocalDateTimeFunction")
fun Time(expr: Expression): Function = TimeFunction(expr)
/** Represents an SQL function that extracts the time part from a given datetime [expr]. */
@JvmName("LocalDateTimeTimeFunction")
fun Time(expr: Expression): Function = TimeFunction(expr)
/** Represents an SQL function that extracts the time part from a given timestamp [expr]. */
@JvmName("InstantTimeFunction")
fun Time(expr: Expression): Function = TimeFunction(expr)
/**
* Represents an SQL function that returns the current date and time, as [LocalDateTime].
*
* @sample org.jetbrains.exposed.DefaultsTest.testConsistentSchemeWithFunctionAsDefaultExpression
*/
object CurrentDateTime : Function(KotlinLocalDateTimeColumnType.INSTANCE) {
override fun toQueryBuilder(queryBuilder: QueryBuilder) = queryBuilder {
+when {
(currentDialect as? MysqlDialect)?.isFractionDateTimeSupported() == true -> "CURRENT_TIMESTAMP(6)"
else -> "CURRENT_TIMESTAMP"
}
}
}
/**
* Represents an SQL function that returns the current date, as [LocalDate].
*
* @sample org.jetbrains.exposed.DefaultsTest.testConsistentSchemeWithFunctionAsDefaultExpression
*/
object CurrentDate : Function(KotlinLocalDateColumnType.INSTANCE) {
override fun toQueryBuilder(queryBuilder: QueryBuilder) = queryBuilder {
+when (currentDialect) {
is MysqlDialect -> "CURRENT_DATE()"
is SQLServerDialect -> "GETDATE()"
else -> "CURRENT_DATE"
}
}
}
/**
* Represents an SQL function that returns the current date and time.
*
* @sample org.jetbrains.exposed.DefaultsTest.testConsistentSchemeWithFunctionAsDefaultExpression
*/
class CurrentTimestamp : Function(KotlinInstantColumnType.INSTANCE) {
override fun toQueryBuilder(queryBuilder: QueryBuilder) = queryBuilder {
+when {
(currentDialect as? MysqlDialect)?.isFractionDateTimeSupported() == true -> "CURRENT_TIMESTAMP(6)"
else -> "CURRENT_TIMESTAMP"
}
}
}
class YearInternal(val expr: Expression<*>) : Function(IntegerColumnType()) {
override fun toQueryBuilder(queryBuilder: QueryBuilder) = queryBuilder {
val dialect = currentDialect
val functionProvider = when (dialect.h2Mode) {
H2Dialect.H2CompatibilityMode.SQLServer -> (dialect as H2Dialect).originalFunctionProvider
else -> dialect.functionProvider
}
functionProvider.year(expr, queryBuilder)
}
}
/** Represents an SQL function that extracts the year field from a given date [expr]. */
@JvmName("LocalDateYearFunction")
fun Year(expr: Expression): Function = YearInternal(expr)
/** Represents an SQL function that extracts the year field from a given datetime [expr]. */
@JvmName("LocalDateTimeYearFunction")
fun Year(expr: Expression): Function = YearInternal(expr)
/** Represents an SQL function that extracts the year field from a given timestamp [expr]. */
@JvmName("InstantYearFunction")
fun Year(expr: Expression): Function = YearInternal(expr)
internal class MonthInternal(val expr: Expression<*>) : Function(IntegerColumnType()) {
override fun toQueryBuilder(queryBuilder: QueryBuilder) = queryBuilder {
val dialect = currentDialect
val functionProvider = when (dialect.h2Mode) {
H2Dialect.H2CompatibilityMode.SQLServer -> (dialect as H2Dialect).originalFunctionProvider
else -> dialect.functionProvider
}
functionProvider.month(expr, queryBuilder)
}
}
/** Represents an SQL function that extracts the month field from a given date [expr]. */
@JvmName("LocalDateMonthFunction")
fun Month(expr: Expression): Function = MonthInternal(expr)
/** Represents an SQL function that extracts the month field from a given datetime [expr]. */
@JvmName("LocalDateTimeMonthFunction")
fun Month(expr: Expression): Function = MonthInternal(expr)
/** Represents an SQL function that extracts the month field from a given timestamp [expr]. */
@JvmName("InstantMonthFunction")
fun Month(expr: Expression): Function = MonthInternal(expr)
internal class DayInternal(val expr: Expression<*>) : Function(IntegerColumnType()) {
override fun toQueryBuilder(queryBuilder: QueryBuilder) = queryBuilder {
val dialect = currentDialect
val functionProvider = when (dialect.h2Mode) {
H2Dialect.H2CompatibilityMode.SQLServer -> (dialect as H2Dialect).originalFunctionProvider
else -> dialect.functionProvider
}
functionProvider.day(expr, queryBuilder)
}
}
/** Represents an SQL function that extracts the day field from a given date [expr]. */
@JvmName("LocalDateDayFunction")
fun Day(expr: Expression): Function = DayInternal(expr)
/** Represents an SQL function that extracts the day field from a given datetime [expr]. */
@JvmName("LocalDateTimeDayFunction")
fun Day(expr: Expression): Function = DayInternal(expr)
/** Represents an SQL function that extracts the day field from a given timestamp [expr]. */
@JvmName("InstantDayFunction")
fun Day(expr: Expression): Function = DayInternal(expr)
internal class HourInternal(val expr: Expression<*>) : Function(IntegerColumnType()) {
override fun toQueryBuilder(queryBuilder: QueryBuilder) = queryBuilder {
val dialect = currentDialect
val functionProvider = when (dialect.h2Mode) {
H2Dialect.H2CompatibilityMode.SQLServer -> (dialect as H2Dialect).originalFunctionProvider
else -> dialect.functionProvider
}
functionProvider.hour(expr, queryBuilder)
}
}
/** Represents an SQL function that extracts the hour field from a given [expr]. */
@JvmName("LocalDateHourFunction")
fun Hour(expr: Expression): Function = HourInternal(expr)
/** Represents an SQL function that extracts the hour field from a given datetime [expr]. */
@JvmName("LocalDateTimeHourFunction")
fun Hour(expr: Expression): Function = HourInternal(expr)
/** Represents an SQL function that extracts the hour field from a given timestamp [expr]. */
@JvmName("InstantHourFunction")
fun Hour(expr: Expression): Function = HourInternal(expr)
internal class MinuteInternal(val expr: Expression<*>) : Function(IntegerColumnType()) {
override fun toQueryBuilder(queryBuilder: QueryBuilder) = queryBuilder {
val dialect = currentDialect
val functionProvider = when (dialect.h2Mode) {
H2Dialect.H2CompatibilityMode.SQLServer -> (dialect as H2Dialect).originalFunctionProvider
else -> dialect.functionProvider
}
functionProvider.minute(expr, queryBuilder)
}
}
/** Represents an SQL function that extracts the minute field from a given [expr]. */
@JvmName("LocalDateMinuteFunction")
fun Minute(expr: Expression): Function = MinuteInternal(expr)
/** Represents an SQL function that extracts the minute field from a given datetime [expr]. */
@JvmName("LocalDateTimeMinuteFunction")
fun Minute(expr: Expression): Function = MinuteInternal(expr)
/** Represents an SQL function that extracts the minute field from a given timestamp [expr]. */
@JvmName("InstantMinuteFunction")
fun Minute(expr: Expression): Function = MinuteInternal(expr)
internal class SecondInternal(val expr: Expression<*>) : Function(IntegerColumnType()) {
override fun toQueryBuilder(queryBuilder: QueryBuilder) = queryBuilder {
val dialect = currentDialect
val functionProvider = when (dialect.h2Mode) {
H2Dialect.H2CompatibilityMode.SQLServer -> (dialect as H2Dialect).originalFunctionProvider
else -> dialect.functionProvider
}
functionProvider.second(expr, queryBuilder)
}
}
/** Represents an SQL function that extracts the second field from a given [expr]. */
@JvmName("LocalDateSecondFunction")
fun Second(expr: Expression): Function = SecondInternal(expr)
/** Represents an SQL function that extracts the second field from a given datetime [expr]. */
@JvmName("LocalDateTimeSecondFunction")
fun Second(expr: Expression): Function = SecondInternal(expr)
/** Represents an SQL function that extracts the second field from a given timestamp [expr]. */
@JvmName("InstantSecondFunction")
fun Second(expr: Expression): Function = SecondInternal(expr)
// Extension functions
/** Returns the date from this expression. */
@JvmName("LocalDateDateExt")
fun Expression.date() = Date(this)
/** Returns the date from this datetime expression. */
@JvmName("LocalDateTimeDateExt")
fun Expression.date() = Date(this)
/** Returns the date from this timestamp expression. */
@JvmName("InstantDateExt")
fun Expression.date() = Date(this)
/** Returns the year from this date expression, as an integer. */
@JvmName("LocalDateYearExt")
fun Expression.year() = Year(this)
/** Returns the year from this datetime expression, as an integer. */
@JvmName("LocalDateTimeYearExt")
fun Expression.year() = Year(this)
/** Returns the year from this timestamp expression, as an integer. */
@JvmName("InstantYearExt")
fun Expression.year() = Year(this)
/** Returns the month from this date expression, as an integer between 1 and 12 inclusive. */
@JvmName("LocalDateMonthExt")
fun Expression.month() = Month(this)
/** Returns the month from this datetime expression, as an integer between 1 and 12 inclusive. */
@JvmName("LocalDateTimeMonthExt")
fun Expression.month() = Month(this)
/** Returns the month from this timestamp expression, as an integer between 1 and 12 inclusive. */
@JvmName("InstantMonthExt")
fun Expression.month() = Month(this)
/** Returns the day from this date expression, as an integer between 1 and 31 inclusive. */
@JvmName("LocalDateDayExt")
fun Expression.day() = Day(this)
/** Returns the day from this datetime expression, as an integer between 1 and 31 inclusive. */
@JvmName("LocalDateTimeDayExt")
fun Expression.day() = Day(this)
/** Returns the day from this timestamp expression, as an integer between 1 and 31 inclusive. */
@JvmName("InstantDayExt")
fun Expression.day() = Day(this)
/** Returns the hour from this expression, as an integer between 0 and 23 inclusive. */
@JvmName("LocalDateHourExt")
fun Expression.hour() = Hour(this)
/** Returns the hour from this datetime expression, as an integer between 0 and 23 inclusive. */
@JvmName("LocalDateTimeHourExt")
fun Expression.hour() = Hour(this)
/** Returns the hour from this timestamp expression, as an integer between 0 and 23 inclusive. */
@JvmName("InstantHourExt")
fun Expression.hour() = Hour(this)
/** Returns the minute from this expression, as an integer between 0 and 59 inclusive. */
@JvmName("LocalDateMinuteExt")
fun Expression.minute() = Minute(this)
/** Returns the minute from this datetime expression, as an integer between 0 and 59 inclusive. */
@JvmName("LocalDateTimeMinuteExt")
fun Expression.minute() = Minute(this)
/** Returns the minute from this timestamp expression, as an integer between 0 and 59 inclusive. */
@JvmName("InstantMinuteExt")
fun Expression.minute() = Minute(this)
/** Returns the second from this expression, as an integer between 0 and 59 inclusive. */
@JvmName("LocalDateSecondExt")
fun Expression.second() = Second(this)
/** Returns the second from this datetime expression, as an integer between 0 and 59 inclusive. */
@JvmName("LocalDateTimeSecondExt")
fun Expression.second() = Second(this)
/** Returns the second from this timestamp expression, as an integer between 0 and 59 inclusive. */
@JvmName("InstantSecondExt")
fun Expression.second() = Second(this)
/** Returns the specified [value] as a date query parameter. */
fun dateParam(value: LocalDate): Expression = QueryParameter(value, KotlinLocalDateColumnType.INSTANCE)
/** Returns the specified [value] as a time query parameter. */
fun timeParam(value: LocalTime): Expression = QueryParameter(value, KotlinLocalTimeColumnType.INSTANCE)
/** Returns the specified [value] as a date with time query parameter. */
fun dateTimeParam(value: LocalDateTime): Expression = QueryParameter(value, KotlinLocalDateTimeColumnType.INSTANCE)
/** Returns the specified [value] as a timestamp query parameter. */
fun timestampParam(value: Instant): Expression = QueryParameter(value, KotlinInstantColumnType.INSTANCE)
/** Returns the specified [value] as a date with time and time zone query parameter. */
fun timestampWithTimeZoneParam(value: OffsetDateTime): Expression =
QueryParameter(value, KotlinOffsetDateTimeColumnType.INSTANCE)
/** Returns the specified [value] as a duration query parameter. */
fun durationParam(value: Duration): Expression = QueryParameter(value, KotlinDurationColumnType.INSTANCE)
/** Returns the specified [value] as a date literal. */
fun dateLiteral(value: LocalDate): LiteralOp = LiteralOp(KotlinLocalDateColumnType.INSTANCE, value)
/** Returns the specified [value] as a time literal. */
fun timeLiteral(value: LocalTime): LiteralOp = LiteralOp(KotlinLocalTimeColumnType.INSTANCE, value)
/** Returns the specified [value] as a date with time literal. */
fun dateTimeLiteral(value: LocalDateTime): LiteralOp = LiteralOp(KotlinLocalDateTimeColumnType.INSTANCE, value)
/** Returns the specified [value] as a timestamp literal. */
fun timestampLiteral(value: Instant): LiteralOp = LiteralOp(KotlinInstantColumnType.INSTANCE, value)
/** Returns the specified [value] as a date with time and time zone literal. */
fun timestampWithTimeZoneLiteral(value: OffsetDateTime): LiteralOp =
LiteralOp(KotlinOffsetDateTimeColumnType.INSTANCE, value)
/** Returns the specified [value] as a duration literal. */
fun durationLiteral(value: Duration): LiteralOp = LiteralOp(KotlinDurationColumnType.INSTANCE, value)
/**
* Calls a custom SQL function with the specified [functionName], that returns a date only,
* and passing [params] as its arguments.
*/
fun CustomDateFunction(functionName: String, vararg params: Expression<*>): CustomFunction =
CustomFunction(functionName, KotlinLocalDateColumnType.INSTANCE, *params)
/**
* Calls a custom SQL function with the specified [functionName], that returns a time only,
* and passing [params] as its arguments.
*/
fun CustomTimeFunction(functionName: String, vararg params: Expression<*>): CustomFunction =
CustomFunction(functionName, KotlinLocalTimeColumnType.INSTANCE, *params)
/**
* Calls a custom SQL function with the specified [functionName], that returns both a date and a time,
* and passing [params] as its arguments.
*/
fun CustomDateTimeFunction(functionName: String, vararg params: Expression<*>): CustomFunction =
CustomFunction(functionName, KotlinLocalDateTimeColumnType.INSTANCE, *params)
/**
* Calls a custom SQL function with the specified [functionName], that returns a timestamp,
* and passing [params] as its arguments.
*/
fun CustomTimeStampFunction(functionName: String, vararg params: Expression<*>): CustomFunction =
CustomFunction(functionName, KotlinInstantColumnType.INSTANCE, *params)
/**
* Calls a custom SQL function with the specified [functionName], that returns both a date and a time with time zone,
* and passing [params] as its arguments.
*/
@Suppress("FunctionName")
fun CustomTimestampWithTimeZoneFunction(
functionName: String,
vararg params: Expression<*>
): CustomFunction = CustomFunction(functionName, KotlinOffsetDateTimeColumnType.INSTANCE, *params)
/**
* Calls a custom SQL function with the specified [functionName], that returns a duration,
* and passing [params] as its arguments.
*/
fun CustomDurationFunction(functionName: String, vararg params: Expression<*>): CustomFunction =
CustomFunction(functionName, KotlinDurationColumnType.INSTANCE, *params)
© 2015 - 2024 Weber Informatics LLC | Privacy Policy