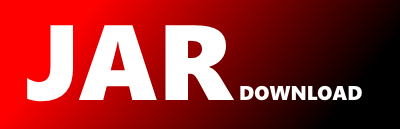
org.jetbrains.kotlin.android.synthetic.res.androidResources.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlin-android-extensions Show documentation
Show all versions of kotlin-android-extensions Show documentation
Kotlin Android Extensions Compiler
/*
* Copyright 2010-2015 JetBrains s.r.o.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jetbrains.kotlin.android.synthetic.res
import org.jetbrains.kotlin.com.intellij.openapi.project.Project
import org.jetbrains.kotlin.com.intellij.psi.PsiElement
import org.jetbrains.kotlin.com.intellij.psi.util.CachedValue
import org.jetbrains.kotlin.com.intellij.psi.util.CachedValueProvider
import org.jetbrains.kotlin.com.intellij.psi.util.CachedValuesManager
import org.jetbrains.kotlin.android.synthetic.AndroidConst
import org.jetbrains.kotlin.descriptors.ClassDescriptor
import org.jetbrains.kotlin.descriptors.ModuleDescriptor
import org.jetbrains.kotlin.name.ClassId
import org.jetbrains.kotlin.name.FqName
import org.jetbrains.kotlin.name.isValidJavaFqName
import org.jetbrains.kotlin.serialization.deserialization.findClassAcrossModuleDependencies
class AndroidVariant(val name: String, val resDirectories: List) {
val packageName: String = name
val isMainVariant: Boolean
get() = name == "main"
companion object {
fun createMainVariant(resDirectories: List) = AndroidVariant("main", resDirectories)
}
}
class AndroidModule(val applicationPackage: String, val variants: List) {
override fun equals(other: Any?) = other is AndroidModule && applicationPackage == other.applicationPackage
override fun hashCode() = applicationPackage.hashCode()
}
class ResourceIdentifier(val name: String, val packageName: String?) {
// Without packageName
override fun equals(other: Any?): Boolean {
if (this === other) return true
if (other?.javaClass != javaClass) return false
other as ResourceIdentifier
if (name != other.name) return false
return true
}
override fun hashCode(): Int {
return name.hashCode()
}
}
sealed class AndroidResource(val id: ResourceIdentifier, val sourceElement: PsiElement?) {
open fun sameClass(other: AndroidResource): Boolean = false
class Widget(
id: ResourceIdentifier,
val xmlType: String,
sourceElement: PsiElement?
) : AndroidResource(id, sourceElement) {
override fun sameClass(other: AndroidResource) = other is Widget
}
class Fragment(id: ResourceIdentifier, sourceElement: PsiElement?) : AndroidResource(id, sourceElement) {
override fun sameClass(other: AndroidResource) = other is Fragment
}
}
fun cachedValue(project: Project, result: () -> CachedValueProvider.Result): CachedValue {
return CachedValuesManager.getManager(project).createCachedValue(result, false)
}
class ResolvedWidget(val widget: AndroidResource.Widget, val viewClassDescriptor: ClassDescriptor?) {
val isErrorType: Boolean
get() = viewClassDescriptor == null
val errorType: String?
get() = if (isErrorType) widget.xmlType else null
}
fun AndroidResource.Widget.resolve(module: ModuleDescriptor): ResolvedWidget? {
fun resolve(fqName: String): ClassDescriptor? {
if (!isValidJavaFqName(fqName)) return null
return module.findClassAcrossModuleDependencies(ClassId.topLevel(FqName(fqName)))
}
if (id.packageName != null && resolve(id.packageName + ".R") == null) {
return null
}
if ('.' in xmlType) {
return ResolvedWidget(this, resolve(xmlType))
}
for (packageName in AndroidConst.FQNAME_RESOLVE_PACKAGES) {
val classDescriptor = resolve("$packageName.$xmlType")
if (classDescriptor != null) {
return ResolvedWidget(this, classDescriptor)
}
}
return ResolvedWidget(this, null)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy