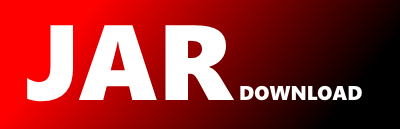
generated._Aggregates.kt Maven / Gradle / Ivy
package kotlin
//
// NOTE THIS FILE IS AUTO-GENERATED by the GenerateStandardLib.kt
// See: https://github.com/JetBrains/kotlin/tree/master/libraries/stdlib
//
import kotlin.platform.*
import java.util.*
import java.util.Collections // TODO: it's temporary while we have java.util.Collections in js
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun Array.all(predicate: (T) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun BooleanArray.all(predicate: (Boolean) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun ByteArray.all(predicate: (Byte) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun CharArray.all(predicate: (Char) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun DoubleArray.all(predicate: (Double) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun FloatArray.all(predicate: (Float) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun IntArray.all(predicate: (Int) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun LongArray.all(predicate: (Long) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun ShortArray.all(predicate: (Short) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun Iterable.all(predicate: (T) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun Map.all(predicate: (Map.Entry) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun Sequence.all(predicate: (T) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if all elements match the given [predicate].
*/
public inline fun String.all(predicate: (Char) -> Boolean): Boolean {
for (element in this) if (!predicate(element)) return false
return true
}
/**
* Returns `true` if collection has at least one element.
*/
public fun Array.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun BooleanArray.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun ByteArray.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun CharArray.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun DoubleArray.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun FloatArray.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun IntArray.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun LongArray.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun ShortArray.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun Iterable.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun Map.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun Sequence.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if collection has at least one element.
*/
public fun String.any(): Boolean {
for (element in this) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun Array.any(predicate: (T) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun BooleanArray.any(predicate: (Boolean) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun ByteArray.any(predicate: (Byte) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun CharArray.any(predicate: (Char) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun DoubleArray.any(predicate: (Double) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun FloatArray.any(predicate: (Float) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun IntArray.any(predicate: (Int) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun LongArray.any(predicate: (Long) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun ShortArray.any(predicate: (Short) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun Iterable.any(predicate: (T) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun Map.any(predicate: (Map.Entry) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun Sequence.any(predicate: (T) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns `true` if at least one element matches the given [predicate].
*/
public inline fun String.any(predicate: (Char) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return true
return false
}
/**
* Returns the number of elements in this collection.
*/
public fun Array.count(): Int {
return size()
}
/**
* Returns the number of elements in this collection.
*/
public fun BooleanArray.count(): Int {
return size()
}
/**
* Returns the number of elements in this collection.
*/
public fun ByteArray.count(): Int {
return size()
}
/**
* Returns the number of elements in this collection.
*/
public fun CharArray.count(): Int {
return size()
}
/**
* Returns the number of elements in this collection.
*/
public fun DoubleArray.count(): Int {
return size()
}
/**
* Returns the number of elements in this collection.
*/
public fun FloatArray.count(): Int {
return size()
}
/**
* Returns the number of elements in this collection.
*/
public fun IntArray.count(): Int {
return size()
}
/**
* Returns the number of elements in this collection.
*/
public fun LongArray.count(): Int {
return size()
}
/**
* Returns the number of elements in this collection.
*/
public fun ShortArray.count(): Int {
return size()
}
/**
* Returns the number of elements in this collection.
*/
public fun Collection.count(): Int {
return size()
}
/**
* Returns the number of elements in this collection.
*/
public fun Iterable.count(): Int {
var count = 0
for (element in this) count++
return count
}
/**
* Returns the number of elements in this collection.
*/
public fun Map.count(): Int {
return size()
}
/**
* Returns the number of elements in this collection.
*/
public fun Sequence.count(): Int {
var count = 0
for (element in this) count++
return count
}
/**
* Returns the length of this string.
*/
public fun String.count(): Int {
return length()
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun Array.count(predicate: (T) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun BooleanArray.count(predicate: (Boolean) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun ByteArray.count(predicate: (Byte) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun CharArray.count(predicate: (Char) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun DoubleArray.count(predicate: (Double) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun FloatArray.count(predicate: (Float) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun IntArray.count(predicate: (Int) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun LongArray.count(predicate: (Long) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun ShortArray.count(predicate: (Short) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun Iterable.count(predicate: (T) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun Map.count(predicate: (Map.Entry) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun Sequence.count(predicate: (T) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Returns the number of elements matching the given [predicate].
*/
public inline fun String.count(predicate: (Char) -> Boolean): Int {
var count = 0
for (element in this) if (predicate(element)) count++
return count
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun Array.fold(initial: R, operation: (R, T) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun BooleanArray.fold(initial: R, operation: (R, Boolean) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun ByteArray.fold(initial: R, operation: (R, Byte) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun CharArray.fold(initial: R, operation: (R, Char) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun DoubleArray.fold(initial: R, operation: (R, Double) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun FloatArray.fold(initial: R, operation: (R, Float) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun IntArray.fold(initial: R, operation: (R, Int) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun LongArray.fold(initial: R, operation: (R, Long) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun ShortArray.fold(initial: R, operation: (R, Short) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun Iterable.fold(initial: R, operation: (R, T) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun Sequence.fold(initial: R, operation: (R, T) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun String.fold(initial: R, operation: (R, Char) -> R): R {
var accumulator = initial
for (element in this) accumulator = operation(accumulator, element)
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun Array.foldRight(initial: R, operation: (T, R) -> R): R {
var index = lastIndex
var accumulator = initial
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun BooleanArray.foldRight(initial: R, operation: (Boolean, R) -> R): R {
var index = lastIndex
var accumulator = initial
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun ByteArray.foldRight(initial: R, operation: (Byte, R) -> R): R {
var index = lastIndex
var accumulator = initial
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun CharArray.foldRight(initial: R, operation: (Char, R) -> R): R {
var index = lastIndex
var accumulator = initial
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun DoubleArray.foldRight(initial: R, operation: (Double, R) -> R): R {
var index = lastIndex
var accumulator = initial
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun FloatArray.foldRight(initial: R, operation: (Float, R) -> R): R {
var index = lastIndex
var accumulator = initial
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun IntArray.foldRight(initial: R, operation: (Int, R) -> R): R {
var index = lastIndex
var accumulator = initial
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun LongArray.foldRight(initial: R, operation: (Long, R) -> R): R {
var index = lastIndex
var accumulator = initial
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun ShortArray.foldRight(initial: R, operation: (Short, R) -> R): R {
var index = lastIndex
var accumulator = initial
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun List.foldRight(initial: R, operation: (T, R) -> R): R {
var index = lastIndex
var accumulator = initial
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with [initial] value and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun String.foldRight(initial: R, operation: (Char, R) -> R): R {
var index = lastIndex
var accumulator = initial
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Performs the given [operation] on each element.
*/
public inline fun Array.forEach(operation: (T) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun BooleanArray.forEach(operation: (Boolean) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun ByteArray.forEach(operation: (Byte) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun CharArray.forEach(operation: (Char) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun DoubleArray.forEach(operation: (Double) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun FloatArray.forEach(operation: (Float) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun IntArray.forEach(operation: (Int) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun LongArray.forEach(operation: (Long) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun ShortArray.forEach(operation: (Short) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun Iterable.forEach(operation: (T) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun Map.forEach(operation: (Map.Entry) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun Sequence.forEach(operation: (T) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element.
*/
public inline fun String.forEach(operation: (Char) -> Unit): Unit {
for (element in this) operation(element)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun Array.forEachIndexed(operation: (Int, T) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun BooleanArray.forEachIndexed(operation: (Int, Boolean) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun ByteArray.forEachIndexed(operation: (Int, Byte) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun CharArray.forEachIndexed(operation: (Int, Char) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun DoubleArray.forEachIndexed(operation: (Int, Double) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun FloatArray.forEachIndexed(operation: (Int, Float) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun IntArray.forEachIndexed(operation: (Int, Int) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun LongArray.forEachIndexed(operation: (Int, Long) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun ShortArray.forEachIndexed(operation: (Int, Short) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun Iterable.forEachIndexed(operation: (Int, T) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun Sequence.forEachIndexed(operation: (Int, T) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Performs the given [operation] on each element, providing sequential index with the element.
*/
public inline fun String.forEachIndexed(operation: (Int, Char) -> Unit): Unit {
var index = 0
for (item in this) operation(index++, item)
}
/**
* Returns the largest element or `null` if there are no elements.
*/
public fun > Array.max(): T? {
if (isEmpty()) return null
var max = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (max < e) max = e
}
return max
}
/**
* Returns the largest element or `null` if there are no elements.
*/
public fun ByteArray.max(): Byte? {
if (isEmpty()) return null
var max = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (max < e) max = e
}
return max
}
/**
* Returns the largest element or `null` if there are no elements.
*/
public fun CharArray.max(): Char? {
if (isEmpty()) return null
var max = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (max < e) max = e
}
return max
}
/**
* Returns the largest element or `null` if there are no elements.
*/
public fun DoubleArray.max(): Double? {
if (isEmpty()) return null
var max = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (max < e) max = e
}
return max
}
/**
* Returns the largest element or `null` if there are no elements.
*/
public fun FloatArray.max(): Float? {
if (isEmpty()) return null
var max = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (max < e) max = e
}
return max
}
/**
* Returns the largest element or `null` if there are no elements.
*/
public fun IntArray.max(): Int? {
if (isEmpty()) return null
var max = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (max < e) max = e
}
return max
}
/**
* Returns the largest element or `null` if there are no elements.
*/
public fun LongArray.max(): Long? {
if (isEmpty()) return null
var max = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (max < e) max = e
}
return max
}
/**
* Returns the largest element or `null` if there are no elements.
*/
public fun ShortArray.max(): Short? {
if (isEmpty()) return null
var max = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (max < e) max = e
}
return max
}
/**
* Returns the largest element or `null` if there are no elements.
*/
public fun > Iterable.max(): T? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var max = iterator.next()
while (iterator.hasNext()) {
val e = iterator.next()
if (max < e) max = e
}
return max
}
/**
* Returns the largest element or `null` if there are no elements.
*/
public fun > Sequence.max(): T? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var max = iterator.next()
while (iterator.hasNext()) {
val e = iterator.next()
if (max < e) max = e
}
return max
}
/**
* Returns the largest element or `null` if there are no elements.
*/
public fun String.max(): Char? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var max = iterator.next()
while (iterator.hasNext()) {
val e = iterator.next()
if (max < e) max = e
}
return max
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun , T : Any> Array.maxBy(f: (T) -> R): T? {
if (isEmpty()) return null
var maxElem = this[0]
var maxValue = f(maxElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun > BooleanArray.maxBy(f: (Boolean) -> R): Boolean? {
if (isEmpty()) return null
var maxElem = this[0]
var maxValue = f(maxElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun > ByteArray.maxBy(f: (Byte) -> R): Byte? {
if (isEmpty()) return null
var maxElem = this[0]
var maxValue = f(maxElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun > CharArray.maxBy(f: (Char) -> R): Char? {
if (isEmpty()) return null
var maxElem = this[0]
var maxValue = f(maxElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun > DoubleArray.maxBy(f: (Double) -> R): Double? {
if (isEmpty()) return null
var maxElem = this[0]
var maxValue = f(maxElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun > FloatArray.maxBy(f: (Float) -> R): Float? {
if (isEmpty()) return null
var maxElem = this[0]
var maxValue = f(maxElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun > IntArray.maxBy(f: (Int) -> R): Int? {
if (isEmpty()) return null
var maxElem = this[0]
var maxValue = f(maxElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun > LongArray.maxBy(f: (Long) -> R): Long? {
if (isEmpty()) return null
var maxElem = this[0]
var maxValue = f(maxElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun > ShortArray.maxBy(f: (Short) -> R): Short? {
if (isEmpty()) return null
var maxElem = this[0]
var maxValue = f(maxElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun , T : Any> Iterable.maxBy(f: (T) -> R): T? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var maxElem = iterator.next()
var maxValue = f(maxElem)
while (iterator.hasNext()) {
val e = iterator.next()
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun , T : Any> Sequence.maxBy(f: (T) -> R): T? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var maxElem = iterator.next()
var maxValue = f(maxElem)
while (iterator.hasNext()) {
val e = iterator.next()
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun > String.maxBy(f: (Char) -> R): Char? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var maxElem = iterator.next()
var maxValue = f(maxElem)
while (iterator.hasNext()) {
val e = iterator.next()
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the first element yielding the largest value of the given function or `null` if there are no elements.
*/
public inline fun > Map.maxBy(f: (Map.Entry) -> R): Map.Entry? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var maxElem = iterator.next()
var maxValue = f(maxElem)
while (iterator.hasNext()) {
val e = iterator.next()
val v = f(e)
if (maxValue < v) {
maxElem = e
maxValue = v
}
}
return maxElem
}
/**
* Returns the smallest element or `null` if there are no elements.
*/
public fun > Array.min(): T? {
if (isEmpty()) return null
var min = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (min > e) min = e
}
return min
}
/**
* Returns the smallest element or `null` if there are no elements.
*/
public fun ByteArray.min(): Byte? {
if (isEmpty()) return null
var min = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (min > e) min = e
}
return min
}
/**
* Returns the smallest element or `null` if there are no elements.
*/
public fun CharArray.min(): Char? {
if (isEmpty()) return null
var min = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (min > e) min = e
}
return min
}
/**
* Returns the smallest element or `null` if there are no elements.
*/
public fun DoubleArray.min(): Double? {
if (isEmpty()) return null
var min = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (min > e) min = e
}
return min
}
/**
* Returns the smallest element or `null` if there are no elements.
*/
public fun FloatArray.min(): Float? {
if (isEmpty()) return null
var min = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (min > e) min = e
}
return min
}
/**
* Returns the smallest element or `null` if there are no elements.
*/
public fun IntArray.min(): Int? {
if (isEmpty()) return null
var min = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (min > e) min = e
}
return min
}
/**
* Returns the smallest element or `null` if there are no elements.
*/
public fun LongArray.min(): Long? {
if (isEmpty()) return null
var min = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (min > e) min = e
}
return min
}
/**
* Returns the smallest element or `null` if there are no elements.
*/
public fun ShortArray.min(): Short? {
if (isEmpty()) return null
var min = this[0]
for (i in 1..lastIndex) {
val e = this[i]
if (min > e) min = e
}
return min
}
/**
* Returns the smallest element or `null` if there are no elements.
*/
public fun > Iterable.min(): T? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var min = iterator.next()
while (iterator.hasNext()) {
val e = iterator.next()
if (min > e) min = e
}
return min
}
/**
* Returns the smallest element or `null` if there are no elements.
*/
public fun > Sequence.min(): T? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var min = iterator.next()
while (iterator.hasNext()) {
val e = iterator.next()
if (min > e) min = e
}
return min
}
/**
* Returns the smallest element or `null` if there are no elements.
*/
public fun String.min(): Char? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var min = iterator.next()
while (iterator.hasNext()) {
val e = iterator.next()
if (min > e) min = e
}
return min
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun , T : Any> Array.minBy(f: (T) -> R): T? {
if (size() == 0) return null
var minElem = this[0]
var minValue = f(minElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun > BooleanArray.minBy(f: (Boolean) -> R): Boolean? {
if (size() == 0) return null
var minElem = this[0]
var minValue = f(minElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun > ByteArray.minBy(f: (Byte) -> R): Byte? {
if (size() == 0) return null
var minElem = this[0]
var minValue = f(minElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun > CharArray.minBy(f: (Char) -> R): Char? {
if (size() == 0) return null
var minElem = this[0]
var minValue = f(minElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun > DoubleArray.minBy(f: (Double) -> R): Double? {
if (size() == 0) return null
var minElem = this[0]
var minValue = f(minElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun > FloatArray.minBy(f: (Float) -> R): Float? {
if (size() == 0) return null
var minElem = this[0]
var minValue = f(minElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun > IntArray.minBy(f: (Int) -> R): Int? {
if (size() == 0) return null
var minElem = this[0]
var minValue = f(minElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun > LongArray.minBy(f: (Long) -> R): Long? {
if (size() == 0) return null
var minElem = this[0]
var minValue = f(minElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun > ShortArray.minBy(f: (Short) -> R): Short? {
if (size() == 0) return null
var minElem = this[0]
var minValue = f(minElem)
for (i in 1..lastIndex) {
val e = this[i]
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun , T : Any> Iterable.minBy(f: (T) -> R): T? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var minElem = iterator.next()
var minValue = f(minElem)
while (iterator.hasNext()) {
val e = iterator.next()
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun , T : Any> Sequence.minBy(f: (T) -> R): T? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var minElem = iterator.next()
var minValue = f(minElem)
while (iterator.hasNext()) {
val e = iterator.next()
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun > String.minBy(f: (Char) -> R): Char? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var minElem = iterator.next()
var minValue = f(minElem)
while (iterator.hasNext()) {
val e = iterator.next()
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns the first element yielding the smallest value of the given function or `null` if there are no elements.
*/
public inline fun > Map.minBy(f: (Map.Entry) -> R): Map.Entry? {
val iterator = iterator()
if (!iterator.hasNext()) return null
var minElem = iterator.next()
var minValue = f(minElem)
while (iterator.hasNext()) {
val e = iterator.next()
val v = f(e)
if (minValue > v) {
minElem = e
minValue = v
}
}
return minElem
}
/**
* Returns `true` if collection has no elements.
*/
public fun Array.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun BooleanArray.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun ByteArray.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun CharArray.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun DoubleArray.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun FloatArray.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun IntArray.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun LongArray.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun ShortArray.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun Iterable.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun Map.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun Sequence.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if collection has no elements.
*/
public fun String.none(): Boolean {
for (element in this) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun Array.none(predicate: (T) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun BooleanArray.none(predicate: (Boolean) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun ByteArray.none(predicate: (Byte) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun CharArray.none(predicate: (Char) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun DoubleArray.none(predicate: (Double) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun FloatArray.none(predicate: (Float) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun IntArray.none(predicate: (Int) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun LongArray.none(predicate: (Long) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun ShortArray.none(predicate: (Short) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun Iterable.none(predicate: (T) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun Map.none(predicate: (Map.Entry) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun Sequence.none(predicate: (T) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Returns `true` if no elements match the given [predicate].
*/
public inline fun String.none(predicate: (Char) -> Boolean): Boolean {
for (element in this) if (predicate(element)) return false
return true
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun Array.reduce(operation: (S, T) -> S): S {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator: S = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun Iterable.reduce(operation: (S, T) -> S): S {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator: S = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun Sequence.reduce(operation: (S, T) -> S): S {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator: S = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun BooleanArray.reduce(operation: (Boolean, Boolean) -> Boolean): Boolean {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun ByteArray.reduce(operation: (Byte, Byte) -> Byte): Byte {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun CharArray.reduce(operation: (Char, Char) -> Char): Char {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun DoubleArray.reduce(operation: (Double, Double) -> Double): Double {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun FloatArray.reduce(operation: (Float, Float) -> Float): Float {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun IntArray.reduce(operation: (Int, Int) -> Int): Int {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun LongArray.reduce(operation: (Long, Long) -> Long): Long {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun ShortArray.reduce(operation: (Short, Short) -> Short): Short {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with the first element and applying [operation] from left to right to current accumulator value and each element.
*/
public inline fun String.reduce(operation: (Char, Char) -> Char): Char {
val iterator = this.iterator()
if (!iterator.hasNext()) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = iterator.next()
while (iterator.hasNext()) {
accumulator = operation(accumulator, iterator.next())
}
return accumulator
}
/**
* Accumulates value starting with last element and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun Array.reduceRight(operation: (T, S) -> S): S {
var index = lastIndex
if (index < 0) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator: S = get(index--)
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with last element and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun List.reduceRight(operation: (T, S) -> S): S {
var index = lastIndex
if (index < 0) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator: S = get(index--)
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with last element and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun BooleanArray.reduceRight(operation: (Boolean, Boolean) -> Boolean): Boolean {
var index = lastIndex
if (index < 0) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = get(index--)
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with last element and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun ByteArray.reduceRight(operation: (Byte, Byte) -> Byte): Byte {
var index = lastIndex
if (index < 0) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = get(index--)
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with last element and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun CharArray.reduceRight(operation: (Char, Char) -> Char): Char {
var index = lastIndex
if (index < 0) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = get(index--)
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with last element and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun DoubleArray.reduceRight(operation: (Double, Double) -> Double): Double {
var index = lastIndex
if (index < 0) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = get(index--)
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with last element and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun FloatArray.reduceRight(operation: (Float, Float) -> Float): Float {
var index = lastIndex
if (index < 0) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = get(index--)
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with last element and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun IntArray.reduceRight(operation: (Int, Int) -> Int): Int {
var index = lastIndex
if (index < 0) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = get(index--)
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with last element and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun LongArray.reduceRight(operation: (Long, Long) -> Long): Long {
var index = lastIndex
if (index < 0) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = get(index--)
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with last element and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun ShortArray.reduceRight(operation: (Short, Short) -> Short): Short {
var index = lastIndex
if (index < 0) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = get(index--)
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Accumulates value starting with last element and applying [operation] from right to left to each element and current accumulator value.
*/
public inline fun String.reduceRight(operation: (Char, Char) -> Char): Char {
var index = lastIndex
if (index < 0) throw UnsupportedOperationException("Empty iterable can't be reduced.")
var accumulator = get(index--)
while (index >= 0) {
accumulator = operation(get(index--), accumulator)
}
return accumulator
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun Array.sumBy(transform: (T) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun BooleanArray.sumBy(transform: (Boolean) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun ByteArray.sumBy(transform: (Byte) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun CharArray.sumBy(transform: (Char) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun DoubleArray.sumBy(transform: (Double) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun FloatArray.sumBy(transform: (Float) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun IntArray.sumBy(transform: (Int) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun LongArray.sumBy(transform: (Long) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun ShortArray.sumBy(transform: (Short) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun Iterable.sumBy(transform: (T) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun Sequence.sumBy(transform: (T) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from characters in the string.
*/
public inline fun String.sumBy(transform: (Char) -> Int): Int {
var sum: Int = 0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun Array.sumByDouble(transform: (T) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun BooleanArray.sumByDouble(transform: (Boolean) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun ByteArray.sumByDouble(transform: (Byte) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun CharArray.sumByDouble(transform: (Char) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun DoubleArray.sumByDouble(transform: (Double) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun FloatArray.sumByDouble(transform: (Float) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun IntArray.sumByDouble(transform: (Int) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun LongArray.sumByDouble(transform: (Long) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun ShortArray.sumByDouble(transform: (Short) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun Iterable.sumByDouble(transform: (T) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from elements in the collection.
*/
public inline fun Sequence.sumByDouble(transform: (T) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
/**
* Returns the sum of all values produced by [transform] function from characters in the string.
*/
public inline fun String.sumByDouble(transform: (Char) -> Double): Double {
var sum: Double = 0.0
for (element in this) {
sum += transform(element)
}
return sum
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy