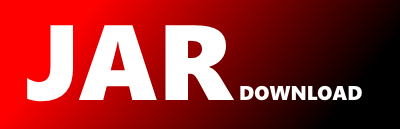
generated._ArraysToPrimitiveArrays.kt Maven / Gradle / Ivy
package kotlin
//
// NOTE THIS FILE IS AUTO-GENERATED by the GenerateStandardLib.kt
// See: https://github.com/JetBrains/kotlin/tree/master/libraries/stdlib
//
import kotlin.platform.*
import java.util.*
import java.util.Collections // TODO: it's temporary while we have java.util.Collections in js
/**
* Returns an array of Boolean containing all of the elements of this generic array.
*/
public fun Array.toBooleanArray(): BooleanArray {
val result = BooleanArray(size())
for (index in indices)
result[index] = this[index]
return result
}
/**
* Returns an array of Boolean containing all of the elements of this collection.
*/
public fun Collection.toBooleanArray(): BooleanArray {
val result = BooleanArray(size())
var index = 0
for (element in this)
result[index++] = element
return result
}
/**
* Returns an array of Byte containing all of the elements of this generic array.
*/
public fun Array.toByteArray(): ByteArray {
val result = ByteArray(size())
for (index in indices)
result[index] = this[index]
return result
}
/**
* Returns an array of Byte containing all of the elements of this collection.
*/
public fun Collection.toByteArray(): ByteArray {
val result = ByteArray(size())
var index = 0
for (element in this)
result[index++] = element
return result
}
/**
* Returns an array of Char containing all of the elements of this generic array.
*/
public fun Array.toCharArray(): CharArray {
val result = CharArray(size())
for (index in indices)
result[index] = this[index]
return result
}
/**
* Returns an array of Char containing all of the elements of this collection.
*/
public fun Collection.toCharArray(): CharArray {
val result = CharArray(size())
var index = 0
for (element in this)
result[index++] = element
return result
}
/**
* Returns an array of Double containing all of the elements of this generic array.
*/
public fun Array.toDoubleArray(): DoubleArray {
val result = DoubleArray(size())
for (index in indices)
result[index] = this[index]
return result
}
/**
* Returns an array of Double containing all of the elements of this collection.
*/
public fun Collection.toDoubleArray(): DoubleArray {
val result = DoubleArray(size())
var index = 0
for (element in this)
result[index++] = element
return result
}
/**
* Returns an array of Float containing all of the elements of this generic array.
*/
public fun Array.toFloatArray(): FloatArray {
val result = FloatArray(size())
for (index in indices)
result[index] = this[index]
return result
}
/**
* Returns an array of Float containing all of the elements of this collection.
*/
public fun Collection.toFloatArray(): FloatArray {
val result = FloatArray(size())
var index = 0
for (element in this)
result[index++] = element
return result
}
/**
* Returns an array of Int containing all of the elements of this generic array.
*/
public fun Array.toIntArray(): IntArray {
val result = IntArray(size())
for (index in indices)
result[index] = this[index]
return result
}
/**
* Returns an array of Int containing all of the elements of this collection.
*/
public fun Collection.toIntArray(): IntArray {
val result = IntArray(size())
var index = 0
for (element in this)
result[index++] = element
return result
}
/**
* Returns an array of Long containing all of the elements of this generic array.
*/
public fun Array.toLongArray(): LongArray {
val result = LongArray(size())
for (index in indices)
result[index] = this[index]
return result
}
/**
* Returns an array of Long containing all of the elements of this collection.
*/
public fun Collection.toLongArray(): LongArray {
val result = LongArray(size())
var index = 0
for (element in this)
result[index++] = element
return result
}
/**
* Returns an array of Short containing all of the elements of this generic array.
*/
public fun Array.toShortArray(): ShortArray {
val result = ShortArray(size())
for (index in indices)
result[index] = this[index]
return result
}
/**
* Returns an array of Short containing all of the elements of this collection.
*/
public fun Collection.toShortArray(): ShortArray {
val result = ShortArray(size())
var index = 0
for (element in this)
result[index++] = element
return result
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy