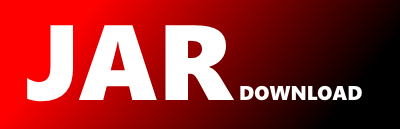
generated._Ordering.kt Maven / Gradle / Ivy
package kotlin
//
// NOTE THIS FILE IS AUTO-GENERATED by the GenerateStandardLib.kt
// See: https://github.com/JetBrains/kotlin/tree/master/libraries/stdlib
//
import kotlin.platform.*
import java.util.*
import java.util.Collections // TODO: it's temporary while we have java.util.Collections in js
/**
* Returns a list with elements in reversed order.
*/
deprecated("reverse will change its behavior soon. Use reversed() instead.", ReplaceWith("reversed()"))
public fun Array.reverse(): List {
return reversed()
}
/**
* Returns a list with elements in reversed order.
*/
deprecated("reverse will change its behavior soon. Use reversed() instead.", ReplaceWith("reversed()"))
public fun BooleanArray.reverse(): List {
return reversed()
}
/**
* Returns a list with elements in reversed order.
*/
deprecated("reverse will change its behavior soon. Use reversed() instead.", ReplaceWith("reversed()"))
public fun ByteArray.reverse(): List {
return reversed()
}
/**
* Returns a list with elements in reversed order.
*/
deprecated("reverse will change its behavior soon. Use reversed() instead.", ReplaceWith("reversed()"))
public fun CharArray.reverse(): List {
return reversed()
}
/**
* Returns a list with elements in reversed order.
*/
deprecated("reverse will change its behavior soon. Use reversed() instead.", ReplaceWith("reversed()"))
public fun DoubleArray.reverse(): List {
return reversed()
}
/**
* Returns a list with elements in reversed order.
*/
deprecated("reverse will change its behavior soon. Use reversed() instead.", ReplaceWith("reversed()"))
public fun FloatArray.reverse(): List {
return reversed()
}
/**
* Returns a list with elements in reversed order.
*/
deprecated("reverse will change its behavior soon. Use reversed() instead.", ReplaceWith("reversed()"))
public fun IntArray.reverse(): List {
return reversed()
}
/**
* Returns a list with elements in reversed order.
*/
deprecated("reverse will change its behavior soon. Use reversed() instead.", ReplaceWith("reversed()"))
public fun LongArray.reverse(): List {
return reversed()
}
/**
* Returns a list with elements in reversed order.
*/
deprecated("reverse will change its behavior soon. Use reversed() instead.", ReplaceWith("reversed()"))
public fun ShortArray.reverse(): List {
return reversed()
}
/**
* Returns a list with elements in reversed order.
*/
deprecated("reverse will change its behavior soon. Use reversed() instead.", ReplaceWith("reversed()"))
public fun Iterable.reverse(): List {
return reversed()
}
/**
* Returns a list with elements in reversed order.
*/
deprecated("reverse will change its behavior soon. Use reversed() instead.", ReplaceWith("reversed()"))
public fun String.reverse(): String {
return reversed()
}
/**
* Returns a list with elements in reversed order.
*/
public fun Array.reversed(): List {
if (isEmpty()) return emptyList()
val list = toArrayList()
Collections.reverse(list)
return list
}
/**
* Returns a list with elements in reversed order.
*/
public fun BooleanArray.reversed(): List {
if (isEmpty()) return emptyList()
val list = toArrayList()
Collections.reverse(list)
return list
}
/**
* Returns a list with elements in reversed order.
*/
public fun ByteArray.reversed(): List {
if (isEmpty()) return emptyList()
val list = toArrayList()
Collections.reverse(list)
return list
}
/**
* Returns a list with elements in reversed order.
*/
public fun CharArray.reversed(): List {
if (isEmpty()) return emptyList()
val list = toArrayList()
Collections.reverse(list)
return list
}
/**
* Returns a list with elements in reversed order.
*/
public fun DoubleArray.reversed(): List {
if (isEmpty()) return emptyList()
val list = toArrayList()
Collections.reverse(list)
return list
}
/**
* Returns a list with elements in reversed order.
*/
public fun FloatArray.reversed(): List {
if (isEmpty()) return emptyList()
val list = toArrayList()
Collections.reverse(list)
return list
}
/**
* Returns a list with elements in reversed order.
*/
public fun IntArray.reversed(): List {
if (isEmpty()) return emptyList()
val list = toArrayList()
Collections.reverse(list)
return list
}
/**
* Returns a list with elements in reversed order.
*/
public fun LongArray.reversed(): List {
if (isEmpty()) return emptyList()
val list = toArrayList()
Collections.reverse(list)
return list
}
/**
* Returns a list with elements in reversed order.
*/
public fun ShortArray.reversed(): List {
if (isEmpty()) return emptyList()
val list = toArrayList()
Collections.reverse(list)
return list
}
/**
* Returns a list with elements in reversed order.
*/
public fun Iterable.reversed(): List {
if (this is Collection && isEmpty()) return emptyList()
val list = toArrayList()
Collections.reverse(list)
return list
}
/**
* Returns a string with characters in reversed order.
*/
public fun String.reversed(): String {
return StringBuilder().append(this).reverse().toString()
}
/**
* Returns an array with elements of this array in reversed order.
*/
public fun Array.reversedArray(): Array {
if (isEmpty()) return this
val result = arrayOfNulls(this, size()) as Array
val lastIndex = lastIndex
for (i in 0..lastIndex)
result[lastIndex - i] = this[i]
return result
}
/**
* Returns an array with elements of this array in reversed order.
*/
public fun BooleanArray.reversedArray(): BooleanArray {
if (isEmpty()) return this
val result = BooleanArray(size())
val lastIndex = lastIndex
for (i in 0..lastIndex)
result[lastIndex - i] = this[i]
return result
}
/**
* Returns an array with elements of this array in reversed order.
*/
public fun ByteArray.reversedArray(): ByteArray {
if (isEmpty()) return this
val result = ByteArray(size())
val lastIndex = lastIndex
for (i in 0..lastIndex)
result[lastIndex - i] = this[i]
return result
}
/**
* Returns an array with elements of this array in reversed order.
*/
public fun CharArray.reversedArray(): CharArray {
if (isEmpty()) return this
val result = CharArray(size())
val lastIndex = lastIndex
for (i in 0..lastIndex)
result[lastIndex - i] = this[i]
return result
}
/**
* Returns an array with elements of this array in reversed order.
*/
public fun DoubleArray.reversedArray(): DoubleArray {
if (isEmpty()) return this
val result = DoubleArray(size())
val lastIndex = lastIndex
for (i in 0..lastIndex)
result[lastIndex - i] = this[i]
return result
}
/**
* Returns an array with elements of this array in reversed order.
*/
public fun FloatArray.reversedArray(): FloatArray {
if (isEmpty()) return this
val result = FloatArray(size())
val lastIndex = lastIndex
for (i in 0..lastIndex)
result[lastIndex - i] = this[i]
return result
}
/**
* Returns an array with elements of this array in reversed order.
*/
public fun IntArray.reversedArray(): IntArray {
if (isEmpty()) return this
val result = IntArray(size())
val lastIndex = lastIndex
for (i in 0..lastIndex)
result[lastIndex - i] = this[i]
return result
}
/**
* Returns an array with elements of this array in reversed order.
*/
public fun LongArray.reversedArray(): LongArray {
if (isEmpty()) return this
val result = LongArray(size())
val lastIndex = lastIndex
for (i in 0..lastIndex)
result[lastIndex - i] = this[i]
return result
}
/**
* Returns an array with elements of this array in reversed order.
*/
public fun ShortArray.reversedArray(): ShortArray {
if (isEmpty()) return this
val result = ShortArray(size())
val lastIndex = lastIndex
for (i in 0..lastIndex)
result[lastIndex - i] = this[i]
return result
}
/**
* Returns a sorted list of all elements.
*/
deprecated("This method may change its behavior soon. Use sorted() instead.", ReplaceWith("sorted()"))
public fun > Iterable.sort(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a list of all elements, sorted by the specified [comparator].
*/
deprecated("This method may change its behavior soon. Use sortedWith() instead.", ReplaceWith("sortedWith(comparator)"))
public fun Array.sortBy(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a list of all elements, sorted by the specified [comparator].
*/
deprecated("This method may change its behavior soon. Use sortedWith() instead.", ReplaceWith("sortedWith(comparator)"))
public fun Iterable.sortBy(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("This method may change its behavior soon. Use sortedBy() instead.", ReplaceWith("sortedBy(order)"))
public inline fun > Array.sortBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) order: (T) -> R): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("This method may change its behavior soon. Use sortedBy() instead.", ReplaceWith("sortedBy(order)"))
public inline fun > Iterable.sortBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) order: (T) -> R): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, in descending order.
*/
deprecated("This method may change its behavior soon. Use sortedDescending() instead.", ReplaceWith("sortedDescending()"))
public fun > Iterable.sortDescending(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator { x, y -> y.compareTo(x) })
return sortedList
}
/**
* Returns a sorted list of all elements, in descending order by results of specified [order] function.
*/
deprecated("This method may change its behavior soon. Use sortedByDescending() instead.", ReplaceWith("sortedByDescending(order)"))
public inline fun > Array.sortDescendingBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) order: (T) -> R): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareByDescending(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, in descending order by results of specified [order] function.
*/
deprecated("This method may change its behavior soon. Use sortedByDescending() instead.", ReplaceWith("sortedByDescending(order)"))
public inline fun > Iterable.sortDescendingBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) order: (T) -> R): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareByDescending(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a list of all elements sorted according to their natural sort order.
*/
public fun > Array.sorted(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a list of all elements sorted according to their natural sort order.
*/
public fun ByteArray.sorted(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a list of all elements sorted according to their natural sort order.
*/
public fun CharArray.sorted(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a list of all elements sorted according to their natural sort order.
*/
public fun DoubleArray.sorted(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a list of all elements sorted according to their natural sort order.
*/
public fun FloatArray.sorted(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a list of all elements sorted according to their natural sort order.
*/
public fun IntArray.sorted(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a list of all elements sorted according to their natural sort order.
*/
public fun LongArray.sorted(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a list of all elements sorted according to their natural sort order.
*/
public fun ShortArray.sorted(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a list of all elements sorted according to their natural sort order.
*/
public fun > Iterable.sorted(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sequence that yields elements of this sequence sorted according to their natural sort order.
*/
public fun > Sequence.sorted(): Sequence {
return object : Sequence {
override fun iterator(): Iterator {
val sortedList = [email protected]()
java.util.Collections.sort(sortedList)
return sortedList.iterator()
}
}
}
/**
* Returns an array with all elements of this array sorted according to their natural sort order.
*/
public fun > Array.sortedArray(): Array {
if (isEmpty()) return this
return this.copyOf().apply { sort() }
}
/**
* Returns an array with all elements of this array sorted according to their natural sort order.
*/
public fun ByteArray.sortedArray(): ByteArray {
if (isEmpty()) return this
return this.copyOf().apply { sort() }
}
/**
* Returns an array with all elements of this array sorted according to their natural sort order.
*/
public fun CharArray.sortedArray(): CharArray {
if (isEmpty()) return this
return this.copyOf().apply { sort() }
}
/**
* Returns an array with all elements of this array sorted according to their natural sort order.
*/
public fun DoubleArray.sortedArray(): DoubleArray {
if (isEmpty()) return this
return this.copyOf().apply { sort() }
}
/**
* Returns an array with all elements of this array sorted according to their natural sort order.
*/
public fun FloatArray.sortedArray(): FloatArray {
if (isEmpty()) return this
return this.copyOf().apply { sort() }
}
/**
* Returns an array with all elements of this array sorted according to their natural sort order.
*/
public fun IntArray.sortedArray(): IntArray {
if (isEmpty()) return this
return this.copyOf().apply { sort() }
}
/**
* Returns an array with all elements of this array sorted according to their natural sort order.
*/
public fun LongArray.sortedArray(): LongArray {
if (isEmpty()) return this
return this.copyOf().apply { sort() }
}
/**
* Returns an array with all elements of this array sorted according to their natural sort order.
*/
public fun ShortArray.sortedArray(): ShortArray {
if (isEmpty()) return this
return this.copyOf().apply { sort() }
}
/**
* Returns an array with all elements of this array sorted descending according to their natural sort order.
*/
public fun > Array.sortedArrayDescending(): Array {
if (isEmpty()) return this
// TODO: Use reverseOrder()
return this.copyOf().apply { sortWith(comparator { a, b -> b.compareTo(a) }) }
}
/**
* Returns an array with all elements of this array sorted descending according to their natural sort order.
*/
public fun ByteArray.sortedArrayDescending(): ByteArray {
if (isEmpty()) return this
// TODO: Use in-place reverse
return this.copyOf().apply { sort() }.reversedArray()
}
/**
* Returns an array with all elements of this array sorted descending according to their natural sort order.
*/
public fun CharArray.sortedArrayDescending(): CharArray {
if (isEmpty()) return this
// TODO: Use in-place reverse
return this.copyOf().apply { sort() }.reversedArray()
}
/**
* Returns an array with all elements of this array sorted descending according to their natural sort order.
*/
public fun DoubleArray.sortedArrayDescending(): DoubleArray {
if (isEmpty()) return this
// TODO: Use in-place reverse
return this.copyOf().apply { sort() }.reversedArray()
}
/**
* Returns an array with all elements of this array sorted descending according to their natural sort order.
*/
public fun FloatArray.sortedArrayDescending(): FloatArray {
if (isEmpty()) return this
// TODO: Use in-place reverse
return this.copyOf().apply { sort() }.reversedArray()
}
/**
* Returns an array with all elements of this array sorted descending according to their natural sort order.
*/
public fun IntArray.sortedArrayDescending(): IntArray {
if (isEmpty()) return this
// TODO: Use in-place reverse
return this.copyOf().apply { sort() }.reversedArray()
}
/**
* Returns an array with all elements of this array sorted descending according to their natural sort order.
*/
public fun LongArray.sortedArrayDescending(): LongArray {
if (isEmpty()) return this
// TODO: Use in-place reverse
return this.copyOf().apply { sort() }.reversedArray()
}
/**
* Returns an array with all elements of this array sorted descending according to their natural sort order.
*/
public fun ShortArray.sortedArrayDescending(): ShortArray {
if (isEmpty()) return this
// TODO: Use in-place reverse
return this.copyOf().apply { sort() }.reversedArray()
}
/**
* Returns an array with all elements of this array sorted according the specified [comparator].
*/
public fun Array.sortedArrayWith(comparator: Comparator): Array {
if (isEmpty()) return this
return this.copyOf().apply { sortWith(comparator) }
}
/**
* Returns a list of all elements sorted according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > Array.sortedBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (T) -> R?): List {
return sortedWith(compareBy(selector))
}
/**
* Returns a list of all elements sorted according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > BooleanArray.sortedBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Boolean) -> R?): List {
return sortedWith(compareBy(selector))
}
/**
* Returns a list of all elements sorted according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > ByteArray.sortedBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Byte) -> R?): List {
return sortedWith(compareBy(selector))
}
/**
* Returns a list of all elements sorted according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > CharArray.sortedBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Char) -> R?): List {
return sortedWith(compareBy(selector))
}
/**
* Returns a list of all elements sorted according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > DoubleArray.sortedBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Double) -> R?): List {
return sortedWith(compareBy(selector))
}
/**
* Returns a list of all elements sorted according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > FloatArray.sortedBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Float) -> R?): List {
return sortedWith(compareBy(selector))
}
/**
* Returns a list of all elements sorted according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > IntArray.sortedBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Int) -> R?): List {
return sortedWith(compareBy(selector))
}
/**
* Returns a list of all elements sorted according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > LongArray.sortedBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Long) -> R?): List {
return sortedWith(compareBy(selector))
}
/**
* Returns a list of all elements sorted according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > ShortArray.sortedBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Short) -> R?): List {
return sortedWith(compareBy(selector))
}
/**
* Returns a list of all elements sorted according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > Iterable.sortedBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (T) -> R?): List {
return sortedWith(compareBy(selector))
}
/**
* Returns a sequence that yields elements of this sequence sorted according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > Sequence.sortedBy(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (T) -> R?): Sequence {
return sortedWith(compareBy(selector))
}
/**
* Returns a list of all elements sorted descending according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > Array.sortedByDescending(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (T) -> R?): List {
return sortedWith(compareByDescending(selector))
}
/**
* Returns a list of all elements sorted descending according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > BooleanArray.sortedByDescending(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Boolean) -> R?): List {
return sortedWith(compareByDescending(selector))
}
/**
* Returns a list of all elements sorted descending according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > ByteArray.sortedByDescending(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Byte) -> R?): List {
return sortedWith(compareByDescending(selector))
}
/**
* Returns a list of all elements sorted descending according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > CharArray.sortedByDescending(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Char) -> R?): List {
return sortedWith(compareByDescending(selector))
}
/**
* Returns a list of all elements sorted descending according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > DoubleArray.sortedByDescending(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Double) -> R?): List {
return sortedWith(compareByDescending(selector))
}
/**
* Returns a list of all elements sorted descending according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > FloatArray.sortedByDescending(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Float) -> R?): List {
return sortedWith(compareByDescending(selector))
}
/**
* Returns a list of all elements sorted descending according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > IntArray.sortedByDescending(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Int) -> R?): List {
return sortedWith(compareByDescending(selector))
}
/**
* Returns a list of all elements sorted descending according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > LongArray.sortedByDescending(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Long) -> R?): List {
return sortedWith(compareByDescending(selector))
}
/**
* Returns a list of all elements sorted descending according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > ShortArray.sortedByDescending(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (Short) -> R?): List {
return sortedWith(compareByDescending(selector))
}
/**
* Returns a list of all elements sorted descending according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > Iterable.sortedByDescending(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (T) -> R?): List {
return sortedWith(compareByDescending(selector))
}
/**
* Returns a sequence that yields elements of this sequence sorted descending according to natural sort order of the value returned by specified [selector] function.
*/
public inline fun > Sequence.sortedByDescending(inlineOptions(InlineOption.ONLY_LOCAL_RETURN) selector: (T) -> R?): Sequence {
return sortedWith(compareByDescending(selector))
}
/**
* Returns a list of all elements sorted descending according to their natural sort order.
*/
public fun > Array.sortedDescending(): List {
return sortedWith(comparator { x, y -> y.compareTo(x) })
}
/**
* Returns a list of all elements sorted descending according to their natural sort order.
*/
public fun ByteArray.sortedDescending(): List {
return copyOf().apply { sort() }.reversed()
}
/**
* Returns a list of all elements sorted descending according to their natural sort order.
*/
public fun CharArray.sortedDescending(): List {
return copyOf().apply { sort() }.reversed()
}
/**
* Returns a list of all elements sorted descending according to their natural sort order.
*/
public fun DoubleArray.sortedDescending(): List {
return copyOf().apply { sort() }.reversed()
}
/**
* Returns a list of all elements sorted descending according to their natural sort order.
*/
public fun FloatArray.sortedDescending(): List {
return copyOf().apply { sort() }.reversed()
}
/**
* Returns a list of all elements sorted descending according to their natural sort order.
*/
public fun IntArray.sortedDescending(): List {
return copyOf().apply { sort() }.reversed()
}
/**
* Returns a list of all elements sorted descending according to their natural sort order.
*/
public fun LongArray.sortedDescending(): List {
return copyOf().apply { sort() }.reversed()
}
/**
* Returns a list of all elements sorted descending according to their natural sort order.
*/
public fun ShortArray.sortedDescending(): List {
return copyOf().apply { sort() }.reversed()
}
/**
* Returns a list of all elements sorted descending according to their natural sort order.
*/
public fun > Iterable.sortedDescending(): List {
return sortedWith(comparator { x, y -> y.compareTo(x) })
}
/**
* Returns a sequence that yields elements of this sequence sorted descending according to their natural sort order.
*/
public fun > Sequence.sortedDescending(): Sequence {
return sortedWith(comparator { x, y -> y.compareTo(x) })
}
/**
* Returns a list of all elements sorted according to the specified [comparator].
*/
public fun Array.sortedWith(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a list of all elements sorted according to the specified [comparator].
*/
public fun BooleanArray.sortedWith(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a list of all elements sorted according to the specified [comparator].
*/
public fun ByteArray.sortedWith(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a list of all elements sorted according to the specified [comparator].
*/
public fun CharArray.sortedWith(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a list of all elements sorted according to the specified [comparator].
*/
public fun DoubleArray.sortedWith(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a list of all elements sorted according to the specified [comparator].
*/
public fun FloatArray.sortedWith(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a list of all elements sorted according to the specified [comparator].
*/
public fun IntArray.sortedWith(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a list of all elements sorted according to the specified [comparator].
*/
public fun LongArray.sortedWith(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a list of all elements sorted according to the specified [comparator].
*/
public fun ShortArray.sortedWith(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a list of all elements sorted according to the specified [comparator].
*/
public fun Iterable.sortedWith(comparator: Comparator): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList, comparator)
return sortedList
}
/**
* Returns a sequence that yields elements of this sequence sorted according to the specified [comparator].
*/
public fun Sequence.sortedWith(comparator: Comparator): Sequence {
return object : Sequence {
override fun iterator(): Iterator {
val sortedList = [email protected]()
java.util.Collections.sort(sortedList, comparator)
return sortedList.iterator()
}
}
}
/**
* Returns a sorted list of all elements.
*/
deprecated("Use sorted() instead.", ReplaceWith("sorted()"))
public fun > Array.toSortedList(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sorted list of all elements.
*/
deprecated("Use sorted() instead.", ReplaceWith("sorted()"))
public fun BooleanArray.toSortedList(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sorted list of all elements.
*/
deprecated("Use sorted() instead.", ReplaceWith("sorted()"))
public fun ByteArray.toSortedList(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sorted list of all elements.
*/
deprecated("Use sorted() instead.", ReplaceWith("sorted()"))
public fun CharArray.toSortedList(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sorted list of all elements.
*/
deprecated("Use sorted() instead.", ReplaceWith("sorted()"))
public fun DoubleArray.toSortedList(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sorted list of all elements.
*/
deprecated("Use sorted() instead.", ReplaceWith("sorted()"))
public fun FloatArray.toSortedList(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sorted list of all elements.
*/
deprecated("Use sorted() instead.", ReplaceWith("sorted()"))
public fun IntArray.toSortedList(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sorted list of all elements.
*/
deprecated("Use sorted() instead.", ReplaceWith("sorted()"))
public fun LongArray.toSortedList(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sorted list of all elements.
*/
deprecated("Use sorted() instead.", ReplaceWith("sorted()"))
public fun ShortArray.toSortedList(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sorted list of all elements.
*/
deprecated("Use sorted() instead.", ReplaceWith("sorted()"))
public fun > Iterable.toSortedList(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sorted list of all elements.
*/
deprecated("Use asIterable().sorted() instead.", ReplaceWith("asIterable().sorted()"))
public fun > Sequence.toSortedList(): List {
val sortedList = toArrayList()
java.util.Collections.sort(sortedList)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("Use sortedBy(order) instead.", ReplaceWith("sortedBy(order)"))
public fun > Array.toSortedListBy(order: (T) -> V): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("Use sortedBy(order) instead.", ReplaceWith("sortedBy(order)"))
public fun > BooleanArray.toSortedListBy(order: (Boolean) -> V): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("Use sortedBy(order) instead.", ReplaceWith("sortedBy(order)"))
public fun > ByteArray.toSortedListBy(order: (Byte) -> V): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("Use sortedBy(order) instead.", ReplaceWith("sortedBy(order)"))
public fun > CharArray.toSortedListBy(order: (Char) -> V): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("Use sortedBy(order) instead.", ReplaceWith("sortedBy(order)"))
public fun > DoubleArray.toSortedListBy(order: (Double) -> V): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("Use sortedBy(order) instead.", ReplaceWith("sortedBy(order)"))
public fun > FloatArray.toSortedListBy(order: (Float) -> V): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("Use sortedBy(order) instead.", ReplaceWith("sortedBy(order)"))
public fun > IntArray.toSortedListBy(order: (Int) -> V): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("Use sortedBy(order) instead.", ReplaceWith("sortedBy(order)"))
public fun > LongArray.toSortedListBy(order: (Long) -> V): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("Use sortedBy(order) instead.", ReplaceWith("sortedBy(order)"))
public fun > ShortArray.toSortedListBy(order: (Short) -> V): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("Use sortedBy(order) instead.", ReplaceWith("sortedBy(order)"))
public fun > Iterable.toSortedListBy(order: (T) -> V): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
/**
* Returns a sorted list of all elements, ordered by results of specified [order] function.
*/
deprecated("Use asIterable().sortedBy(order) instead.", ReplaceWith("asIterable().sortedBy(order)"))
public fun > Sequence.toSortedListBy(order: (T) -> V): List {
val sortedList = toArrayList()
val sortBy: Comparator = compareBy(order)
java.util.Collections.sort(sortedList, sortBy)
return sortedList
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy