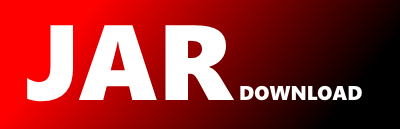
generated._Sets.kt Maven / Gradle / Ivy
package kotlin
//
// NOTE THIS FILE IS AUTO-GENERATED by the GenerateStandardLib.kt
// See: https://github.com/JetBrains/kotlin/tree/master/libraries/stdlib
//
import kotlin.platform.*
import java.util.*
import java.util.Collections // TODO: it's temporary while we have java.util.Collections in js
/**
* Returns a list containing only distinct elements from the given collection.
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public fun Array.distinct(): List {
return this.toMutableSet().toList()
}
/**
* Returns a list containing only distinct elements from the given collection.
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public fun BooleanArray.distinct(): List {
return this.toMutableSet().toList()
}
/**
* Returns a list containing only distinct elements from the given collection.
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public fun ByteArray.distinct(): List {
return this.toMutableSet().toList()
}
/**
* Returns a list containing only distinct elements from the given collection.
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public fun CharArray.distinct(): List {
return this.toMutableSet().toList()
}
/**
* Returns a list containing only distinct elements from the given collection.
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public fun DoubleArray.distinct(): List {
return this.toMutableSet().toList()
}
/**
* Returns a list containing only distinct elements from the given collection.
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public fun FloatArray.distinct(): List {
return this.toMutableSet().toList()
}
/**
* Returns a list containing only distinct elements from the given collection.
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public fun IntArray.distinct(): List {
return this.toMutableSet().toList()
}
/**
* Returns a list containing only distinct elements from the given collection.
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public fun LongArray.distinct(): List {
return this.toMutableSet().toList()
}
/**
* Returns a list containing only distinct elements from the given collection.
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public fun ShortArray.distinct(): List {
return this.toMutableSet().toList()
}
/**
* Returns a list containing only distinct elements from the given collection.
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public fun Iterable.distinct(): List {
return this.toMutableSet().toList()
}
/**
* Returns a sequence containing only distinct elements from the given sequence.
* The elements in the resulting sequence are in the same order as they were in the source sequence.
*/
public fun Sequence.distinct(): Sequence {
return this.distinctBy { it }
}
/**
* Returns a list containing only distinct elements from the given collection according to the [keySelector].
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public inline fun Array.distinctBy(keySelector: (T) -> K): List {
val set = HashSet()
val list = ArrayList()
for (e in this) {
val key = keySelector(e)
if (set.add(key))
list.add(e)
}
return list
}
/**
* Returns a list containing only distinct elements from the given collection according to the [keySelector].
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public inline fun BooleanArray.distinctBy(keySelector: (Boolean) -> K): List {
val set = HashSet()
val list = ArrayList()
for (e in this) {
val key = keySelector(e)
if (set.add(key))
list.add(e)
}
return list
}
/**
* Returns a list containing only distinct elements from the given collection according to the [keySelector].
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public inline fun ByteArray.distinctBy(keySelector: (Byte) -> K): List {
val set = HashSet()
val list = ArrayList()
for (e in this) {
val key = keySelector(e)
if (set.add(key))
list.add(e)
}
return list
}
/**
* Returns a list containing only distinct elements from the given collection according to the [keySelector].
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public inline fun CharArray.distinctBy(keySelector: (Char) -> K): List {
val set = HashSet()
val list = ArrayList()
for (e in this) {
val key = keySelector(e)
if (set.add(key))
list.add(e)
}
return list
}
/**
* Returns a list containing only distinct elements from the given collection according to the [keySelector].
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public inline fun DoubleArray.distinctBy(keySelector: (Double) -> K): List {
val set = HashSet()
val list = ArrayList()
for (e in this) {
val key = keySelector(e)
if (set.add(key))
list.add(e)
}
return list
}
/**
* Returns a list containing only distinct elements from the given collection according to the [keySelector].
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public inline fun FloatArray.distinctBy(keySelector: (Float) -> K): List {
val set = HashSet()
val list = ArrayList()
for (e in this) {
val key = keySelector(e)
if (set.add(key))
list.add(e)
}
return list
}
/**
* Returns a list containing only distinct elements from the given collection according to the [keySelector].
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public inline fun IntArray.distinctBy(keySelector: (Int) -> K): List {
val set = HashSet()
val list = ArrayList()
for (e in this) {
val key = keySelector(e)
if (set.add(key))
list.add(e)
}
return list
}
/**
* Returns a list containing only distinct elements from the given collection according to the [keySelector].
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public inline fun LongArray.distinctBy(keySelector: (Long) -> K): List {
val set = HashSet()
val list = ArrayList()
for (e in this) {
val key = keySelector(e)
if (set.add(key))
list.add(e)
}
return list
}
/**
* Returns a list containing only distinct elements from the given collection according to the [keySelector].
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public inline fun ShortArray.distinctBy(keySelector: (Short) -> K): List {
val set = HashSet()
val list = ArrayList()
for (e in this) {
val key = keySelector(e)
if (set.add(key))
list.add(e)
}
return list
}
/**
* Returns a list containing only distinct elements from the given collection according to the [keySelector].
* The elements in the resulting list are in the same order as they were in the source collection.
*/
public inline fun Iterable.distinctBy(keySelector: (T) -> K): List {
val set = HashSet()
val list = ArrayList()
for (e in this) {
val key = keySelector(e)
if (set.add(key))
list.add(e)
}
return list
}
/**
* Returns a sequence containing only distinct elements from the given sequence according to the [keySelector].
* The elements in the resulting sequence are in the same order as they were in the source sequence.
*/
public fun Sequence.distinctBy(keySelector: (T) -> K): Sequence {
return DistinctSequence(this, keySelector)
}
/**
* Returns a set containing all elements that are contained by both this set and the specified collection.
*/
public fun Array.intersect(other: Iterable): Set {
val set = this.toMutableSet()
set.retainAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by both this set and the specified collection.
*/
public fun BooleanArray.intersect(other: Iterable): Set {
val set = this.toMutableSet()
set.retainAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by both this set and the specified collection.
*/
public fun ByteArray.intersect(other: Iterable): Set {
val set = this.toMutableSet()
set.retainAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by both this set and the specified collection.
*/
public fun CharArray.intersect(other: Iterable): Set {
val set = this.toMutableSet()
set.retainAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by both this set and the specified collection.
*/
public fun DoubleArray.intersect(other: Iterable): Set {
val set = this.toMutableSet()
set.retainAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by both this set and the specified collection.
*/
public fun FloatArray.intersect(other: Iterable): Set {
val set = this.toMutableSet()
set.retainAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by both this set and the specified collection.
*/
public fun IntArray.intersect(other: Iterable): Set {
val set = this.toMutableSet()
set.retainAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by both this set and the specified collection.
*/
public fun LongArray.intersect(other: Iterable): Set {
val set = this.toMutableSet()
set.retainAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by both this set and the specified collection.
*/
public fun ShortArray.intersect(other: Iterable): Set {
val set = this.toMutableSet()
set.retainAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by both this set and the specified collection.
*/
public fun Iterable.intersect(other: Iterable): Set {
val set = this.toMutableSet()
set.retainAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by this set and not contained by the specified collection.
*/
public fun Array.subtract(other: Iterable): Set {
val set = this.toMutableSet()
set.removeAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by this set and not contained by the specified collection.
*/
public fun BooleanArray.subtract(other: Iterable): Set {
val set = this.toMutableSet()
set.removeAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by this set and not contained by the specified collection.
*/
public fun ByteArray.subtract(other: Iterable): Set {
val set = this.toMutableSet()
set.removeAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by this set and not contained by the specified collection.
*/
public fun CharArray.subtract(other: Iterable): Set {
val set = this.toMutableSet()
set.removeAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by this set and not contained by the specified collection.
*/
public fun DoubleArray.subtract(other: Iterable): Set {
val set = this.toMutableSet()
set.removeAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by this set and not contained by the specified collection.
*/
public fun FloatArray.subtract(other: Iterable): Set {
val set = this.toMutableSet()
set.removeAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by this set and not contained by the specified collection.
*/
public fun IntArray.subtract(other: Iterable): Set {
val set = this.toMutableSet()
set.removeAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by this set and not contained by the specified collection.
*/
public fun LongArray.subtract(other: Iterable): Set {
val set = this.toMutableSet()
set.removeAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by this set and not contained by the specified collection.
*/
public fun ShortArray.subtract(other: Iterable): Set {
val set = this.toMutableSet()
set.removeAll(other)
return set
}
/**
* Returns a set containing all elements that are contained by this set and not contained by the specified collection.
*/
public fun Iterable.subtract(other: Iterable): Set {
val set = this.toMutableSet()
set.removeAll(other)
return set
}
/**
* Returns a mutable set containing all distinct elements from the given collection.
*/
public fun Array.toMutableSet(): MutableSet {
val set = LinkedHashSet(mapCapacity(size()))
for (item in this) set.add(item)
return set
}
/**
* Returns a mutable set containing all distinct elements from the given collection.
*/
public fun BooleanArray.toMutableSet(): MutableSet {
val set = LinkedHashSet(mapCapacity(size()))
for (item in this) set.add(item)
return set
}
/**
* Returns a mutable set containing all distinct elements from the given collection.
*/
public fun ByteArray.toMutableSet(): MutableSet {
val set = LinkedHashSet(mapCapacity(size()))
for (item in this) set.add(item)
return set
}
/**
* Returns a mutable set containing all distinct elements from the given collection.
*/
public fun CharArray.toMutableSet(): MutableSet {
val set = LinkedHashSet(mapCapacity(size()))
for (item in this) set.add(item)
return set
}
/**
* Returns a mutable set containing all distinct elements from the given collection.
*/
public fun DoubleArray.toMutableSet(): MutableSet {
val set = LinkedHashSet(mapCapacity(size()))
for (item in this) set.add(item)
return set
}
/**
* Returns a mutable set containing all distinct elements from the given collection.
*/
public fun FloatArray.toMutableSet(): MutableSet {
val set = LinkedHashSet(mapCapacity(size()))
for (item in this) set.add(item)
return set
}
/**
* Returns a mutable set containing all distinct elements from the given collection.
*/
public fun IntArray.toMutableSet(): MutableSet {
val set = LinkedHashSet(mapCapacity(size()))
for (item in this) set.add(item)
return set
}
/**
* Returns a mutable set containing all distinct elements from the given collection.
*/
public fun LongArray.toMutableSet(): MutableSet {
val set = LinkedHashSet(mapCapacity(size()))
for (item in this) set.add(item)
return set
}
/**
* Returns a mutable set containing all distinct elements from the given collection.
*/
public fun ShortArray.toMutableSet(): MutableSet {
val set = LinkedHashSet(mapCapacity(size()))
for (item in this) set.add(item)
return set
}
/**
* Returns a mutable set containing all distinct elements from the given collection.
*/
public fun Iterable.toMutableSet(): MutableSet {
return when (this) {
is Collection -> LinkedHashSet(this)
else -> toCollection(LinkedHashSet())
}
}
/**
* Returns a mutable set containing all distinct elements from the given sequence.
*/
public fun Sequence.toMutableSet(): MutableSet {
val set = LinkedHashSet()
for (item in this) set.add(item)
return set
}
/**
* Returns a set containing all distinct elements from both collections.
*/
public fun Array.union(other: Iterable): Set {
val set = this.toMutableSet()
set.addAll(other)
return set
}
/**
* Returns a set containing all distinct elements from both collections.
*/
public fun BooleanArray.union(other: Iterable): Set {
val set = this.toMutableSet()
set.addAll(other)
return set
}
/**
* Returns a set containing all distinct elements from both collections.
*/
public fun ByteArray.union(other: Iterable): Set {
val set = this.toMutableSet()
set.addAll(other)
return set
}
/**
* Returns a set containing all distinct elements from both collections.
*/
public fun CharArray.union(other: Iterable): Set {
val set = this.toMutableSet()
set.addAll(other)
return set
}
/**
* Returns a set containing all distinct elements from both collections.
*/
public fun DoubleArray.union(other: Iterable): Set {
val set = this.toMutableSet()
set.addAll(other)
return set
}
/**
* Returns a set containing all distinct elements from both collections.
*/
public fun FloatArray.union(other: Iterable): Set {
val set = this.toMutableSet()
set.addAll(other)
return set
}
/**
* Returns a set containing all distinct elements from both collections.
*/
public fun IntArray.union(other: Iterable): Set {
val set = this.toMutableSet()
set.addAll(other)
return set
}
/**
* Returns a set containing all distinct elements from both collections.
*/
public fun LongArray.union(other: Iterable): Set {
val set = this.toMutableSet()
set.addAll(other)
return set
}
/**
* Returns a set containing all distinct elements from both collections.
*/
public fun ShortArray.union(other: Iterable): Set {
val set = this.toMutableSet()
set.addAll(other)
return set
}
/**
* Returns a set containing all distinct elements from both collections.
*/
public fun Iterable.union(other: Iterable): Set {
val set = this.toMutableSet()
set.addAll(other)
return set
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy