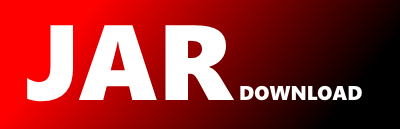
kotlin.metadata.internal.FlagDelegatesImpl.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlin-metadata-jvm Show documentation
Show all versions of kotlin-metadata-jvm Show documentation
Kotlin JVM metadata manipulation library
The newest version!
/*
* Copyright 2010-2023 JetBrains s.r.o. and Kotlin Programming Language contributors.
* Use of this source code is governed by the Apache 2.0 license that can be found in the license/LICENSE.txt file.
*/
package kotlin.metadata.internal
import kotlin.metadata.*
import kotlin.metadata.internal.FlagImpl as Flag
import kotlin.enums.EnumEntries
import kotlin.reflect.KMutableProperty1
import kotlin.reflect.KProperty
import org.jetbrains.kotlin.metadata.deserialization.Flags.FlagField as ProtoFlagSet
import org.jetbrains.kotlin.metadata.deserialization.Flags as ProtoFlags
import org.jetbrains.kotlin.protobuf.Internal.EnumLite as ProtoEnumLite
internal class EnumFlagDelegate>(
val flags: KMutableProperty1,
private val protoSet: ProtoFlagSet,
private val entries: EnumEntries,
private val flagValues: List
) {
// Pre-built permutation ProtoEnum <> E to allow reordering of enum entries?
// Concern: if new enum values are added to metadata proto, everything (including existing flags) will break
operator fun getValue(thisRef: Node, property: KProperty<*>): E = entries[protoSet.get(flags.get(thisRef)).number]
operator fun setValue(thisRef: Node, property: KProperty<*>, value: E) {
flags.set(thisRef, flagValues[value.ordinal] + flags.get(thisRef))
}
}
// Public in internal package - for reuse in JvmFlags
public class BooleanFlagDelegate(private val flags: KMutableProperty1, private val flag: Flag) {
private val mask: Int
init {
require(flag.bitWidth == 1 && flag.value == 1) { "BooleanFlagDelegate can work only with boolean flags (bitWidth = 1 and value = 1), but $flag was passed" }
mask = 1 shl flag.offset
}
public operator fun getValue(thisRef: Node, property: KProperty<*>): Boolean = flag(flags.get(thisRef))
public operator fun setValue(thisRef: Node, property: KProperty<*>, value: Boolean) {
val newValue = if (value) flags.get(thisRef) or mask else flags.get(thisRef) and mask.inv()
flags.set(thisRef, newValue)
}
}
internal fun visibilityDelegate(flags: KMutableProperty1) =
EnumFlagDelegate(flags, ProtoFlags.VISIBILITY, Visibility.entries, Visibility.entries.map { it.flag })
internal fun modalityDelegate(flags: KMutableProperty1) =
EnumFlagDelegate(flags, ProtoFlags.MODALITY, Modality.entries, Modality.entries.map { it.flag })
internal fun memberKindDelegate(flags: KMutableProperty1) =
EnumFlagDelegate(flags, ProtoFlags.MEMBER_KIND, MemberKind.entries, MemberKind.entries.map { it.flag })
internal fun classBooleanFlag(flag: Flag) = BooleanFlagDelegate(KmClass::flags, flag)
internal fun functionBooleanFlag(flag: Flag) = BooleanFlagDelegate(KmFunction::flags, flag)
internal fun constructorBooleanFlag(flag: Flag) = BooleanFlagDelegate(KmConstructor::flags, flag)
internal fun propertyBooleanFlag(flag: Flag) = BooleanFlagDelegate(KmProperty::flags, flag)
internal fun propertyAccessorBooleanFlag(flag: Flag) = BooleanFlagDelegate(KmPropertyAccessorAttributes::flags, flag)
internal fun typeBooleanFlag(flag: Flag) = BooleanFlagDelegate(KmType::flags, flag)
internal fun valueParameterBooleanFlag(flag: Flag) = BooleanFlagDelegate(KmValueParameter::flags, flag)
internal fun annotationsOn(flags: KMutableProperty1) = BooleanFlagDelegate(flags, Flag(ProtoFlags.HAS_ANNOTATIONS))
// Used for kotlin-metadata-jvm tests:
public fun _flagAccess(kmClass: KmClass): Int = kmClass.flags
public fun _flagAccess(kmFunc: KmFunction): Int = kmFunc.flags
public fun _flagAccess(kmType: KmType): Int = kmType.flags
public fun _flagAccess(kmConstr: KmConstructor): Int = kmConstr.flags
© 2015 - 2024 Weber Informatics LLC | Privacy Policy