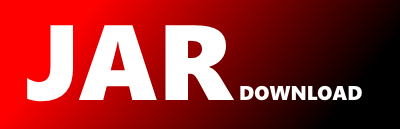
kotlin.coroutines.experimental.CoroutinesLibrary.kt Maven / Gradle / Ivy
/*
* Copyright 2010-2017 JetBrains s.r.o.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
@file:kotlin.jvm.JvmName("CoroutinesKt")
package kotlin.coroutines.experimental
import kotlin.coroutines.experimental.intrinsics.COROUTINE_SUSPENDED
import kotlin.coroutines.experimental.intrinsics.suspendCoroutineOrReturn
import kotlin.coroutines.experimental.intrinsics.createCoroutineUnchecked
/**
* Starts coroutine with receiver type [R] and result type [T].
* This function creates and start a new, fresh instance of suspendable computation every time it is invoked.
* The [completion] continuation is invoked when coroutine completes with result or exception.
*/
@SinceKotlin("1.1")
@Suppress("UNCHECKED_CAST")
public fun (suspend R.() -> T).startCoroutine(
receiver: R,
completion: Continuation
) {
createCoroutineUnchecked(receiver, completion).resume(Unit)
}
/**
* Starts coroutine without receiver and with result type [T].
* This function creates and start a new, fresh instance of suspendable computation every time it is invoked.
* The [completion] continuation is invoked when coroutine completes with result or exception.
*/
@SinceKotlin("1.1")
@Suppress("UNCHECKED_CAST")
public fun (suspend () -> T).startCoroutine(
completion: Continuation
) {
createCoroutineUnchecked(completion).resume(Unit)
}
/**
* Creates coroutine with receiver type [R] and result type [T].
* This function creates a new, fresh instance of suspendable computation every time it is invoked.
* To start executing the created coroutine, invoke `resume(Unit)` on the returned [Continuation] instance.
* The [completion] continuation is invoked when coroutine completes with result or exception.
*/
@SinceKotlin("1.1")
@Suppress("UNCHECKED_CAST")
public fun (suspend R.() -> T).createCoroutine(
receiver: R,
completion: Continuation
): Continuation = SafeContinuation(createCoroutineUnchecked(receiver, completion), COROUTINE_SUSPENDED)
/**
* Creates coroutine without receiver and with result type [T].
* This function creates a new, fresh instance of suspendable computation every time it is invoked.
* To start executing the created coroutine, invoke `resume(Unit)` on the returned [Continuation] instance.
* The [completion] continuation is invoked when coroutine completes with result or exception.
*/
@SinceKotlin("1.1")
@Suppress("UNCHECKED_CAST")
public fun (suspend () -> T).createCoroutine(
completion: Continuation
): Continuation = SafeContinuation(createCoroutineUnchecked(completion), COROUTINE_SUSPENDED)
/**
* Obtains the current continuation instance inside suspend functions and suspends
* currently running coroutine.
*
* In this function both [Continuation.resume] and [Continuation.resumeWithException] can be used either synchronously in
* the same stack-frame where suspension function is run or asynchronously later in the same thread or
* from a different thread of execution. Repeated invocation of any resume function produces [IllegalStateException].
*/
@SinceKotlin("1.1")
public inline suspend fun suspendCoroutine(crossinline block: (Continuation) -> Unit): T =
suspendCoroutineOrReturn { c: Continuation ->
val safe = SafeContinuation(c)
block(safe)
safe.getResult()
}
// INTERNAL DECLARATIONS
@kotlin.internal.InlineOnly
internal inline fun processBareContinuationResume(completion: Continuation<*>, block: () -> Any?) {
try {
val result = block()
if (result !== COROUTINE_SUSPENDED) {
(completion as Continuation).resume(result)
}
} catch (t: Throwable) {
completion.resumeWithException(t)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy