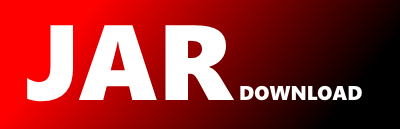
org.jetbrains.kotlinx.spark.api.Conversions.kt Maven / Gradle / Ivy
/*-
* =LICENSE=
* Kotlin Spark API: API for Spark 3.0+ (Scala 2.12)
* ----------
* Copyright (C) 2019 - 2021 JetBrains
* ----------
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* =LICENSEEND=
*/
/**
* This files contains conversions of Iterators, Collections, Tuples, etc. between the Scala-
* and Kotlin/Java variants.
*/
@file:Suppress("NOTHING_TO_INLINE", "RemoveExplicitTypeArguments", "unused")
package org.jetbrains.kotlinx.spark.api
import org.apache.spark.api.java.Optional
import scala.*
import scala.collection.JavaConverters
import java.util.*
import java.util.Enumeration
import java.util.concurrent.ConcurrentMap
import scala.collection.Iterable as ScalaIterable
import scala.collection.Iterator as ScalaIterator
import scala.collection.Map as ScalaMap
import scala.collection.Seq as ScalaSeq
import scala.collection.Set as ScalaSet
import scala.collection.concurrent.Map as ScalaConcurrentMap
import scala.collection.mutable.Buffer as ScalaMutableBuffer
import scala.collection.mutable.Map as ScalaMutableMap
import scala.collection.mutable.Seq as ScalaMutableSeq
import scala.collection.mutable.Set as ScalaMutableSet
import org.apache.spark.streaming.State
/** Returns state value if it exists, else `null`. */
fun State.getOrNull(): T? = if (exists()) get() else null
/** Returns state value if it exists, else [other]. */
fun State.getOrElse(other: T): T = if (exists()) get() else other
/** Converts Scala [Option] to Kotlin nullable. */
fun Option.getOrNull(): T? = getOrElse(null)
/** Get if available else [other]. */
fun Option.getOrElse(other: T): T = getOrElse { other }
/** Converts nullable value to Scala [Option]. */
fun T?.toOption(): Option = Option.apply(this)
/** Converts Scala [Option] to Java [Optional]. */
fun Option.toOptional(): Optional = Optional.ofNullable(getOrNull())
/** Converts [Optional] to Kotlin nullable. */
fun Optional.getOrNull(): T? = orNull()
/** Get if available else [other]. */
fun Optional.getOrElse(other: T): T = orElse(other)
/** Converts nullable value to [Optional]. */
fun T?.toOptional(): Optional = Optional.ofNullable(this)
/** Converts Java [Optional] to Scala [Option]. */
fun Optional.toOption(): Option = Option.apply(getOrNull())
/**
* @see JavaConverters.asScalaIterator for more information.
*/
fun Iterator.asScalaIterator(): ScalaIterator = JavaConverters.asScalaIterator(this)
/**
* @see JavaConverters.enumerationAsScalaIterator for more information.
*/
fun Enumeration.asScalaIterator(): ScalaIterator = JavaConverters.enumerationAsScalaIterator(this)
/**
* @see JavaConverters.iterableAsScalaIterable for more information.
*/
fun Iterable.asScalaIterable(): ScalaIterable = JavaConverters.iterableAsScalaIterable(this)
/**
* @see JavaConverters.collectionAsScalaIterable for more information.
*/
fun Collection.asScalaIterable(): ScalaIterable = JavaConverters.collectionAsScalaIterable(this)
/**
* @see JavaConverters.asScalaBuffer for more information.
*/
fun MutableList.asScalaMutableBuffer(): ScalaMutableBuffer = JavaConverters.asScalaBuffer(this)
/**
* @see JavaConverters.asScalaSet for more information.
*/
fun MutableSet.asScalaMutableSet(): ScalaMutableSet = JavaConverters.asScalaSet(this)
/**
* @see JavaConverters.mapAsScalaMap for more information.
*/
fun MutableMap.asScalaMutableMap(): ScalaMutableMap = JavaConverters.mapAsScalaMap(this)
/**
* @see JavaConverters.dictionaryAsScalaMap for more information.
*/
fun Map.asScalaMap(): ScalaMap = JavaConverters.mapAsScalaMap(this)
/**
* @see JavaConverters.mapAsScalaConcurrentMap for more information.
*/
fun ConcurrentMap.asScalaConcurrentMap(): ScalaConcurrentMap =
JavaConverters.mapAsScalaConcurrentMap(this)
/**
* @see JavaConverters.dictionaryAsScalaMap for more information.
*/
fun Dictionary.asScalaMap(): ScalaMutableMap = JavaConverters.dictionaryAsScalaMap(this)
/**
* @see JavaConverters.propertiesAsScalaMap for more information.
*/
fun Properties.asScalaMap(): ScalaMutableMap = JavaConverters.propertiesAsScalaMap(this)
/**
* @see JavaConverters.asJavaIterator for more information.
*/
fun ScalaIterator.asKotlinIterator(): Iterator = JavaConverters.asJavaIterator(this)
/**
* @see JavaConverters.asJavaEnumeration for more information.
*/
fun ScalaIterator.asKotlinEnumeration(): Enumeration = JavaConverters.asJavaEnumeration(this)
/**
* @see JavaConverters.asJavaIterable for more information.
*/
fun ScalaIterable.asKotlinIterable(): Iterable = JavaConverters.asJavaIterable(this)
/**
* @see JavaConverters.asJavaCollection for more information.
*/
fun ScalaIterable.asKotlinCollection(): Collection = JavaConverters.asJavaCollection(this)
/**
* @see JavaConverters.bufferAsJavaList for more information.
*/
fun ScalaMutableBuffer.asKotlinMutableList(): MutableList = JavaConverters.bufferAsJavaList(this)
/**
* @see JavaConverters.mutableSeqAsJavaList for more information.
*/
fun ScalaMutableSeq.asKotlinMutableList(): MutableList = JavaConverters.mutableSeqAsJavaList(this)
/**
* @see JavaConverters.seqAsJavaList for more information.
*/
fun ScalaSeq.asKotlinList(): List = JavaConverters.seqAsJavaList(this)
/**
* @see JavaConverters.mutableSetAsJavaSet for more information.
*/
fun ScalaMutableSet.asKotlinMutableSet(): MutableSet = JavaConverters.mutableSetAsJavaSet(this)
/**
* @see JavaConverters.setAsJavaSet for more information.
*/
fun ScalaSet.asKotlinSet(): Set = JavaConverters.setAsJavaSet(this)
/**
* @see JavaConverters.mutableMapAsJavaMap for more information.
*/
fun ScalaMutableMap.asKotlinMutableMap(): MutableMap = JavaConverters.mutableMapAsJavaMap(this)
/**
* @see JavaConverters.asJavaDictionary for more information.
*/
fun ScalaMutableMap.asKotlinDictionary(): Dictionary = JavaConverters.asJavaDictionary(this)
/**
* @see JavaConverters.mapAsJavaMap for more information.
*/
fun ScalaMap.asKotlinMap(): Map = JavaConverters.mapAsJavaMap(this)
/**
* @see JavaConverters.mapAsJavaConcurrentMap for more information.
*/
fun ScalaConcurrentMap.asKotlinConcurrentMap(): ConcurrentMap =
JavaConverters.mapAsJavaConcurrentMap(this)
/**
* Returns a new [Arity2] based on the arguments in the current [Pair].
*/
@Deprecated("Use Scala tuples instead.", ReplaceWith("this.toTuple()", "scala.Tuple2"))
fun Pair.toArity(): Arity2 = Arity2(first, second)
/**
* Returns a new [Pair] based on the arguments in the current [Arity2].
*/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity2.toPair(): Pair = Pair(_1, _2)
/**
* Returns a new [Arity3] based on the arguments in the current [Triple].
*/
@Deprecated("Use Scala tuples instead.", ReplaceWith("this.toTuple()", "scala.Tuple3"))
fun Triple.toArity(): Arity3 = Arity3(first, second, third)
/**
* Returns a new [Triple] based on the arguments in the current [Arity3].
*/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity3.toTriple(): Triple = Triple(_1, _2, _3)
/**
* Returns a new Arity1 based on this Tuple1.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple1.toArity(): Arity1 = Arity1(this._1())
/**
* Returns a new Arity2 based on this Tuple2.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple2.toArity(): Arity2 = Arity2(this._1(), this._2())
/**
* Returns a new Arity3 based on this Tuple3.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple3.toArity(): Arity3 = Arity3(this._1(), this._2(), this._3())
/**
* Returns a new Arity4 based on this Tuple4.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple4.toArity(): Arity4 = Arity4(this._1(), this._2(), this._3(), this._4())
/**
* Returns a new Arity5 based on this Tuple5.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple5.toArity(): Arity5 = Arity5(this._1(), this._2(), this._3(), this._4(), this._5())
/**
* Returns a new Arity6 based on this Tuple6.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple6.toArity(): Arity6 = Arity6(this._1(), this._2(), this._3(), this._4(), this._5(), this._6())
/**
* Returns a new Arity7 based on this Tuple7.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple7.toArity(): Arity7 = Arity7(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7())
/**
* Returns a new Arity8 based on this Tuple8.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple8.toArity(): Arity8 = Arity8(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8())
/**
* Returns a new Arity9 based on this Tuple9.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple9.toArity(): Arity9 = Arity9(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9())
/**
* Returns a new Arity10 based on this Tuple10.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple10.toArity(): Arity10 = Arity10(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10())
/**
* Returns a new Arity11 based on this Tuple11.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple11.toArity(): Arity11 = Arity11(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11())
/**
* Returns a new Arity12 based on this Tuple12.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple12.toArity(): Arity12 = Arity12(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11(), this._12())
/**
* Returns a new Arity13 based on this Tuple13.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple13.toArity(): Arity13 = Arity13(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11(), this._12(), this._13())
/**
* Returns a new Arity14 based on this Tuple14.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple14.toArity(): Arity14 = Arity14(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11(), this._12(), this._13(), this._14())
/**
* Returns a new Arity15 based on this Tuple15.
**/@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple15.toArity(): Arity15 = Arity15(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11(), this._12(), this._13(), this._14(), this._15())
/**
* Returns a new Arity16 based on this Tuple16.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple16.toArity(): Arity16 = Arity16(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11(), this._12(), this._13(), this._14(), this._15(), this._16())
/**
* Returns a new Arity17 based on this Tuple17.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple17.toArity(): Arity17 = Arity17(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11(), this._12(), this._13(), this._14(), this._15(), this._16(), this._17())
/**
* Returns a new Arity18 based on this Tuple18.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple18.toArity(): Arity18 = Arity18(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11(), this._12(), this._13(), this._14(), this._15(), this._16(), this._17(), this._18())
/**
* Returns a new Arity19 based on this Tuple19.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple19.toArity(): Arity19 = Arity19(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11(), this._12(), this._13(), this._14(), this._15(), this._16(), this._17(), this._18(), this._19())
/**
* Returns a new Arity20 based on this Tuple20.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple20.toArity(): Arity20 = Arity20(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11(), this._12(), this._13(), this._14(), this._15(), this._16(), this._17(), this._18(), this._19(), this._20())
/**
* Returns a new Arity21 based on this Tuple21.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple21.toArity(): Arity21 = Arity21(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11(), this._12(), this._13(), this._14(), this._15(), this._16(), this._17(), this._18(), this._19(), this._20(), this._21())
/**
* Returns a new Arity22 based on this Tuple22.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Tuple22.toArity(): Arity22 = Arity22(this._1(), this._2(), this._3(), this._4(), this._5(), this._6(), this._7(), this._8(), this._9(), this._10(), this._11(), this._12(), this._13(), this._14(), this._15(), this._16(), this._17(), this._18(), this._19(), this._20(), this._21(), this._22())
/**
* Returns a new Tuple1 based on this Arity1.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity1.toTuple(): Tuple1 = Tuple1(this._1)
/**
* Returns a new Tuple2 based on this Arity2.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity2.toTuple(): Tuple2 = Tuple2(this._1, this._2)
/**
* Returns a new Tuple3 based on this Arity3.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity3.toTuple(): Tuple3 = Tuple3(this._1, this._2, this._3)
/**
* Returns a new Tuple4 based on this Arity4.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity4.toTuple(): Tuple4 = Tuple4(this._1, this._2, this._3, this._4)
/**
* Returns a new Tuple5 based on this Arity5.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity5.toTuple(): Tuple5 = Tuple5(this._1, this._2, this._3, this._4, this._5)
/**
* Returns a new Tuple6 based on this Arity6.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity6.toTuple(): Tuple6 = Tuple6(this._1, this._2, this._3, this._4, this._5, this._6)
/**
* Returns a new Tuple7 based on this Arity7.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity7.toTuple(): Tuple7 = Tuple7(this._1, this._2, this._3, this._4, this._5, this._6, this._7)
/**
* Returns a new Tuple8 based on this Arity8.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity8.toTuple(): Tuple8 = Tuple8(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8)
/**
* Returns a new Tuple9 based on this Arity9.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity9.toTuple(): Tuple9 = Tuple9(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9)
/**
* Returns a new Tuple10 based on this Arity10.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity10.toTuple(): Tuple10 = Tuple10(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10)
/**
* Returns a new Tuple11 based on this Arity11.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity11.toTuple(): Tuple11 = Tuple11(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11)
/**
* Returns a new Tuple12 based on this Arity12.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity12.toTuple(): Tuple12 = Tuple12(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11, this._12)
/**
* Returns a new Tuple13 based on this Arity13.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity13.toTuple(): Tuple13 = Tuple13(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11, this._12, this._13)
/**
* Returns a new Tuple14 based on this Arity14.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity14.toTuple(): Tuple14 = Tuple14(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11, this._12, this._13, this._14)
/**
* Returns a new Tuple15 based on this Arity15.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity15.toTuple(): Tuple15 = Tuple15(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11, this._12, this._13, this._14, this._15)
/**
* Returns a new Tuple16 based on this Arity16.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity16.toTuple(): Tuple16 = Tuple16(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11, this._12, this._13, this._14, this._15, this._16)
/**
* Returns a new Tuple17 based on this Arity17.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity17.toTuple(): Tuple17 = Tuple17(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11, this._12, this._13, this._14, this._15, this._16, this._17)
/**
* Returns a new Tuple18 based on this Arity18.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity18.toTuple(): Tuple18 = Tuple18(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11, this._12, this._13, this._14, this._15, this._16, this._17, this._18)
/**
* Returns a new Tuple19 based on this Arity19.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity19.toTuple(): Tuple19 = Tuple19(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11, this._12, this._13, this._14, this._15, this._16, this._17, this._18, this._19)
/**
* Returns a new Tuple20 based on this Arity20.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity20.toTuple(): Tuple20 = Tuple20(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11, this._12, this._13, this._14, this._15, this._16, this._17, this._18, this._19, this._20)
/**
* Returns a new Tuple21 based on this Arity21.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity21.toTuple(): Tuple21 = Tuple21(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11, this._12, this._13, this._14, this._15, this._16, this._17, this._18, this._19, this._20, this._21)
/**
* Returns a new Tuple22 based on this Arity22.
**/
@Deprecated("Use Scala tuples instead.", ReplaceWith(""))
fun Arity22.toTuple(): Tuple22 = Tuple22(this._1, this._2, this._3, this._4, this._5, this._6, this._7, this._8, this._9, this._10, this._11, this._12, this._13, this._14, this._15, this._16, this._17, this._18, this._19, this._20, this._21, this._22)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy