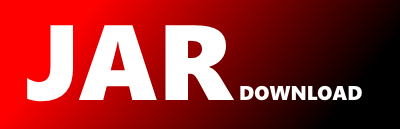
org.jetbrains.kotlinx.ggdsl.ir.bindings.mapping.kt Maven / Gradle / Ivy
package org.jetbrains.kotlinx.ggdsl.ir.bindings
import org.jetbrains.kotlinx.ggdsl.ir.aes.*
import org.jetbrains.kotlinx.ggdsl.ir.data.DataSource
import org.jetbrains.kotlinx.ggdsl.ir.scale.ScaleParameters
import kotlin.reflect.KType
/**
* Mapping base interface
*
* @property aes the aesthetic attribute to be mapped to.
*/
sealed interface Mapping {
val aes: AesName
}
/**
* Mapping with an implicit scale.
*
* @property aes the aesthetic attribute to be mapped to
* @property source the source to which the mapping is applied
* @property domainType the type of domain
*/
data class NonScalablePositionalMapping(
override val aes: AesName,
val source: DataSource,
val domainType: KType
) : Mapping
/**
* Mapping interface with an explicit scale.
*
* @property aes the aesthetic attribute to be mapped to
* @property sourceScaled the scaled source to which the mapping is applied
* @property domainType the type of domain
*/
sealed interface ScaledMapping : Mapping {
override val aes: AesName
val sourceScaled: SourceScaled
val domainType: KType
var scaleParameters: ScaleParameters?
}
sealed interface BaseScaledPositionalMapping : ScaledMapping {
override val aes: AesName
override val sourceScaled: SourceScaled
override val domainType: KType
override var scaleParameters: ScaleParameters?
}
sealed interface BaseScaledNonPositionalMapping : ScaledMapping {
override val aes: AesName
override val sourceScaled: SourceScaled
override val domainType: KType
override var scaleParameters: ScaleParameters?
}
/**
* Mapping with an unspecified default scale.
*
* @property aes the aesthetic attribute to be mapped to
* @property sourceScaled the scaled default unspecified source to which the mapping is applied
* @property domainType type of domain
*/
sealed interface ScaledUnspecifiedDefaultMapping : ScaledMapping {
override val aes: AesName
override val sourceScaled: SourceScaledUnspecifiedDefault
override val domainType: KType
override var scaleParameters: ScaleParameters?
}
data class ScaledUnspecifiedDefaultPositionalMapping(
override val aes: AesName,
override val sourceScaled: SourceScaledUnspecifiedDefault,
override val domainType: KType
) : BaseScaledPositionalMapping, ScaledUnspecifiedDefaultMapping {
override var scaleParameters: ScaleParameters? = null
}
data class ScaledUnspecifiedDefaultNonPositionalMapping(
override val aes: AesName,
override val sourceScaled: SourceScaledUnspecifiedDefault,
override val domainType: KType
) : BaseScaledNonPositionalMapping, ScaledUnspecifiedDefaultMapping {
override var scaleParameters: ScaleParameters? = null
}
/**
* Mapping with a positional default scale.
*
* @property aes the aesthetic attribute to be mapped to
* @property sourceScaled the scaled default positional source to which the mapping is applied
* @property domainType type of domain
*/
data class ScaledPositionalDefaultMapping(
override val aes: AesName,
override val sourceScaled: SourceScaledPositionalUnspecified,
override val domainType: KType
) : BaseScaledPositionalMapping {
override var scaleParameters: ScaleParameters? = null
}
/**
* Mapping with a non-positional default scale.
*
* @property aes the aesthetic attribute to be mapped to
* @property sourceScaled the scaled default non-positional source to which the mapping is applied
* @property domainType type of domain
*/
data class ScaledNonPositionalDefaultMapping(
override val aes: AesName,
override val sourceScaled: SourceScaledNonPositionalUnspecified,
override val domainType: KType
) : BaseScaledNonPositionalMapping {
override var scaleParameters: ScaleParameters? = null
}
/**
* Mapping with a positional scale.
*
* @property aes the aesthetic attribute to be mapped to
* @property sourceScaled the scaled positional source to which the mapping is applied
* @property domainType type of domain
*/
data class ScaledPositionalMapping(
override val aes: AesName,
override val sourceScaled: SourceScaledPositional,
override val domainType: KType
) : BaseScaledPositionalMapping {
override var scaleParameters: ScaleParameters? = null
}
/**
* Mapping with a non-positional scale.
*
* @property aes the aesthetic attribute to be mapped to
* @property sourceScaled the scaled non-positional source to which the mapping is applied
* @property domainType type of domain
*/
data class ScaledNonPositionalMapping(
override val aes: AesName,
override val sourceScaled: SourceScaledNonPositional,
override val domainType: KType,
) : BaseScaledNonPositionalMapping {
override var scaleParameters: ScaleParameters? = null
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy