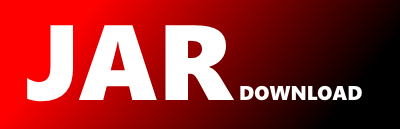
org.jetbrains.kotlinx.ggdsl.dsl.bindings.kt Maven / Gradle / Ivy
package org.jetbrains.kotlinx.ggdsl.dsl
import org.jetbrains.kotlinx.ggdsl.ir.aes.*
import org.jetbrains.kotlinx.ggdsl.ir.bindings.*
import org.jetbrains.kotlinx.ggdsl.ir.data.DataSource
import kotlin.reflect.KProperty
import kotlin.reflect.typeOf
/**
* Sets the given constant value to this non-positional aesthetic attribute
*
* @param value the assigned value.
*/
operator fun NonPositionalAes.invoke(value: T) {
context.bindingCollector.settings[this.name] = NonPositionalSetting(this.name, value)
}
// TODO
operator fun PositionalAes.invoke(value: T) {
context.bindingCollector.settings[this.name] = PositionalSetting(this.name, value)
}
/**
* Maps the given [DataSource] to this non-scalable ("sub-positional") aesthetic attribute.
*
* @param source the mapped raw data source.
*/
inline operator fun NonScalablePositionalAes.invoke(
source: DataSource
) {
context.bindingCollector.mappings[this.name] =
NonScalablePositionalMapping(this.name, source, typeOf())
}
/**
* Maps the given [Iterable] to this non-scalable ("sub-positional") aesthetic attribute.
*
* @param data the mapped raw data.
*/
inline operator fun NonScalablePositionalAes.invoke(
data: Iterable
) {
context.bindingCollector.mappings[this.name] =
NonScalablePositionalMapping(this.name, with(context) { data.toDataSource() }, typeOf())
}
/**
* Maps the given property to this non-scalable ("sub-positional") aesthetic attribute.
*
* @param property the mapped [KProperty].
*/
inline operator fun NonScalablePositionalAes.invoke(
property: KProperty
) {
context.bindingCollector.mappings[this.name] =
NonScalablePositionalMapping(this.name, property.toDataSource(), typeOf())
}
/**
* Maps the given [DataSource] to this positional aesthetic attribute with default scale.
*
* @param source the mapped raw data source.
*/
inline operator fun ScalablePositionalAes.invoke(
source: DataSource
): ScaledUnspecifiedDefaultPositionalMapping {
val mapping = ScaledUnspecifiedDefaultPositionalMapping(
this.name,
source.scaled(),
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given [Iterable] to this positional aesthetic attribute with default scale.
*
* @param data the mapped raw data.
*/
inline operator fun ScalablePositionalAes.invoke(
data: Iterable
): ScaledUnspecifiedDefaultPositionalMapping {
val mapping = ScaledUnspecifiedDefaultPositionalMapping(
this.name,
with(context) { data.toDataSource() }.scaled(),
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given property to this positional aesthetic attribute with default scale.
*
* @param property the mapped [KProperty].
*/
inline operator fun ScalablePositionalAes.invoke(
property: KProperty
): ScaledUnspecifiedDefaultPositionalMapping {
val mapping = ScaledUnspecifiedDefaultPositionalMapping(
this.name,
property.toDataSource().scaled(),
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given [DataSource] to this non-positional aesthetic attribute with default scale.
*
* @param source the mapped raw data source.
*/
inline operator fun MappableNonPositionalAes.invoke(
source: DataSource
): ScaledUnspecifiedDefaultNonPositionalMapping {
val mapping = ScaledUnspecifiedDefaultNonPositionalMapping(
this.name,
source.scaled(),
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given [Iterable] to this non-positional aesthetic attribute with default scale.
*
* @param data the mapped raw data.
*/
inline operator fun MappableNonPositionalAes.invoke(
data: Iterable
): ScaledUnspecifiedDefaultNonPositionalMapping {
val mapping = ScaledUnspecifiedDefaultNonPositionalMapping(
this.name,
with(context) { data.toDataSource() }.scaled(),
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given property to this non-positional aesthetic attribute with default scale.
*
* @param property the mapped [KProperty].
*/
inline operator fun MappableNonPositionalAes.invoke(
property: KProperty
): ScaledUnspecifiedDefaultNonPositionalMapping {
val mapping = ScaledUnspecifiedDefaultNonPositionalMapping(
this.name,
property.toDataSource().scaled(),
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled source to this positional aesthetic attribute with unspecified scale.
*
* @param sourceScaledDefault the mapped source scaled default.
*/
inline operator fun ScalablePositionalAes.invoke(
sourceScaledDefault: SourceScaledUnspecifiedDefault
): ScaledUnspecifiedDefaultPositionalMapping {
val mapping = ScaledUnspecifiedDefaultPositionalMapping(
this.name,
sourceScaledDefault,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled source to this non-positional aesthetic attribute with unspecified scale.
*
* @param sourceScaledDefault the mapped source scaled default.
*/
inline operator fun MappableNonPositionalAes.invoke(
sourceScaledDefault: SourceScaledUnspecifiedDefault
): ScaledUnspecifiedDefaultNonPositionalMapping {
val mapping = ScaledUnspecifiedDefaultNonPositionalMapping(
this.name,
sourceScaledDefault,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled source to this positional aesthetic attribute with default scale.
*
* @param sourceScaledDefault the mapped source scaled unspecified positional.
*/
inline operator fun ScalablePositionalAes.invoke(
sourceScaledDefault: SourceScaledPositionalUnspecified
): ScaledPositionalDefaultMapping {
val mapping = ScaledPositionalDefaultMapping(
this.name,
sourceScaledDefault,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled source to this non-positional aesthetic attribute with unspecified scale.
*
* @param sourceScaledDefault the mapped source scaled unspecified non-positional.
*/
inline operator fun MappableNonPositionalAes.invoke(
sourceScaledDefault: SourceScaledNonPositionalUnspecified
): ScaledNonPositionalDefaultMapping {
val mapping = ScaledNonPositionalDefaultMapping(
this.name,
sourceScaledDefault,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled source to this positional aesthetic attribute.
*
* @param sourceScaledPositional the mapped source scaled positional.
*/
inline operator fun ScalablePositionalAes.invoke(
sourceScaledPositional: SourceScaledPositional
): ScaledPositionalMapping {
val mapping = ScaledPositionalMapping(
this.name,
sourceScaledPositional,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled source to this non-positional aesthetic attribute.
*
* @param sourceScaledNonPositional the mapped source scaled non-positional.
*/
inline operator fun
MappableNonPositionalAes.invoke(
sourceScaledNonPositional: SourceScaledNonPositional
): ScaledNonPositionalMapping {
val mapping = ScaledNonPositionalMapping(
this.name,
sourceScaledNonPositional,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy