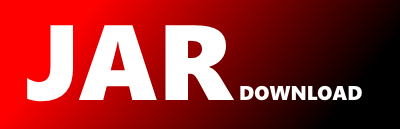
org.jetbrains.kotlinx.ggdsl.dsl.mappings.kt Maven / Gradle / Ivy
/*
* Copyright 2020-2022 JetBrains s.r.o. Use of this column code is governed by the Apache 2.0 license.
*/
package org.jetbrains.kotlinx.ggdsl.dsl
import org.jetbrains.kotlinx.ggdsl.ir.bindings.*
import org.jetbrains.kotlinx.ggdsl.ir.data.ColumnPointer
import kotlin.reflect.typeOf
/**
* Maps the given [ColumnPointer] to this non-scalable positional ("sub-positional") aesthetic attribute.
*
* @param column the mapped raw data column.
*/
public inline operator fun NonScalablePositionalAes.invoke(
column: ColumnPointer
) {
context.bindingCollector.mappings[this.name] =
NonScalablePositionalMapping(this.name, column, typeOf())
}
/**
* Maps the given [ColumnPointer] to this non-scalable non-positional aesthetic attribute.
*
* @param column the mapped raw data column.
*/
public inline operator fun NonScalableNonPositionalAes.invoke(
column: ColumnPointer
) {
context.bindingCollector.mappings[this.name] =
NonScalableNonPositionalMapping(this.name, column, typeOf())
}
/**
* Maps the given [ColumnPointer] to this positional aesthetic attribute with an unspecified
* (i.e. without specifying the type and parameters; they will be defined automatically) scale.
*
* @param column the mapped raw data column.
*/
public inline operator fun ScalablePositionalAes.invoke(
column: ColumnPointer
): ScaledUnspecifiedDefaultPositionalMapping {
val mapping = ScaledUnspecifiedDefaultPositionalMapping(
this.name,
column.scaled(),
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given [ColumnPointer] to this non-positional aesthetic attribute with an unspecified
* (i.e. without specifying the type and parameters; they will be defined automatically) scale.
*
* @param column the mapped raw data column.
*/
public inline operator fun ScalableNonPositionalAes.invoke(
column: ColumnPointer
): ScaledUnspecifiedDefaultNonPositionalMapping {
val mapping = ScaledUnspecifiedDefaultNonPositionalMapping(
this.name,
column.scaled(),
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled column to this positional aesthetic attribute with an unspecified
* (i.e. without specifying the type and parameters; they will be defined automatically) scale.
*
* @param columnScaledDefault the mapped column scaled default.
*/
public inline operator fun ScalablePositionalAes.invoke(
columnScaledDefault: ColumnScaledUnspecifiedDefault
): ScaledUnspecifiedDefaultPositionalMapping {
val mapping = ScaledUnspecifiedDefaultPositionalMapping(
this.name,
columnScaledDefault,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled column to this non-positional aesthetic attribute with an unspecified
* (i.e. without specifying the type and parameters; they will be defined automatically) scale.
*
* @param columnScaledDefault the mapped column scaled default.
*/
public inline operator fun ScalableNonPositionalAes.invoke(
columnScaledDefault: ColumnScaledUnspecifiedDefault
): ScaledUnspecifiedDefaultNonPositionalMapping {
val mapping = ScaledUnspecifiedDefaultNonPositionalMapping(
this.name,
columnScaledDefault,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled column to this positional aesthetic attribute with a default
* (i.e. without specifying the type and parameters; they will be defined automatically) scale.
*
* @param columnScaledDefault the mapped column scaled unspecified positional.
*/
public inline operator fun ScalablePositionalAes.invoke(
columnScaledDefault: ColumnScaledPositionalUnspecified
): ScaledPositionalUnspecifiedMapping {
val mapping = ScaledPositionalUnspecifiedMapping(
this.name,
columnScaledDefault,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled column to this non-positional aesthetic attribute with an unspecified
* (i.e. without specifying the type and parameters; they will be defined automatically) scale.
*
* @param columnScaledDefault the mapped column scaled unspecified non-positional.
*/
public inline operator fun ScalableNonPositionalAes.invoke(
columnScaledDefault: ColumnScaledNonPositionalUnspecified
): ScaledNonPositionalUnspecifiedMapping {
val mapping = ScaledNonPositionalUnspecifiedMapping(
this.name,
columnScaledDefault,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled column to this positional aesthetic attribute.
*
* @param columnScaledPositional the mapped column scaled positional.
*/
public inline operator fun ScalablePositionalAes.invoke(
columnScaledPositional: ColumnScaledPositional
): ScaledPositionalMapping {
val mapping = ScaledPositionalMapping(
this.name,
columnScaledPositional,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
/**
* Maps the given scaled column to this non-positional aesthetic attribute.
*
* @param columnScaledNonPositional the mapped column scaled non-positional.
*/
public inline operator fun
ScalableNonPositionalAes.invoke(
columnScaledNonPositional: ColumnScaledNonPositional
): ScaledNonPositionalMapping {
val mapping = ScaledNonPositionalMapping(
this.name,
columnScaledNonPositional,
typeOf()
)
context.bindingCollector.mappings[this.name] = mapping
return mapping
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy