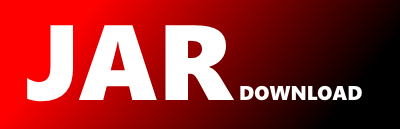
intrinsics.Undispatched.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlinx-coroutines-core-common Show documentation
Show all versions of kotlinx-coroutines-core-common Show documentation
Coroutines support libraries for Kotlin
/*
* Copyright 2016-2018 JetBrains s.r.o. Use of this source code is governed by the Apache 2.0 license.
*/
package kotlinx.coroutines.intrinsics
import kotlinx.coroutines.*
import kotlin.coroutines.*
import kotlin.coroutines.intrinsics.*
/**
* Use this function to restart coroutine directly from inside of [suspendCoroutine],
* when the code is already in the context of this coroutine.
* It does not use [ContinuationInterceptor] and does not update context of the current thread.
*/
public fun (suspend () -> T).startCoroutineUnintercepted(completion: Continuation) {
startDirect(completion) {
startCoroutineUninterceptedOrReturn(completion)
}
}
/**
* Use this function to restart coroutine directly from inside of [suspendCoroutine],
* when the code is already in the context of this coroutine.
* It does not use [ContinuationInterceptor] and does not update context of the current thread.
*/
public fun (suspend (R) -> T).startCoroutineUnintercepted(receiver: R, completion: Continuation) {
startDirect(completion) {
startCoroutineUninterceptedOrReturn(receiver, completion)
}
}
/**
* Use this function to start new coroutine in [CoroutineStart.UNDISPATCHED] mode —
* immediately execute coroutine in the current thread until next suspension.
* It does not use [ContinuationInterceptor], but updates the context of the current thread for the new coroutine.
*/
public fun (suspend () -> T).startCoroutineUndispatched(completion: Continuation) {
startDirect(completion) {
withCoroutineContext(completion.context) {
startCoroutineUninterceptedOrReturn(completion)
}
}
}
/**
* Use this function to start new coroutine in [CoroutineStart.UNDISPATCHED] mode —
* immediately execute coroutine in the current thread until next suspension.
* It does not use [ContinuationInterceptor], but updates the context of the current thread for the new coroutine.
*/
public fun (suspend (R) -> T).startCoroutineUndispatched(receiver: R, completion: Continuation) {
startDirect(completion) {
withCoroutineContext(completion.context) {
startCoroutineUninterceptedOrReturn(receiver, completion)
}
}
}
private inline fun startDirect(completion: Continuation, block: () -> Any?) {
val value = try {
block()
} catch (e: Throwable) {
completion.resumeWithException(e)
return
}
if (value !== COROUTINE_SUSPENDED) {
@Suppress("UNCHECKED_CAST")
completion.resume(value as T)
}
}
/**
* Starts this coroutine with the given code [block] in the same context and returns result when it
* completes without suspension.
* This function shall be invoked at most once on this coroutine.
*
* First, this function initializes parent job from the `parentContext` of this coroutine that was passed to it
* during construction. Second, it starts the coroutine using [startCoroutineUninterceptedOrReturn].
*/
public fun AbstractCoroutine.startUndispatchedOrReturn(block: suspend () -> T): Any? {
initParentJob()
return undispatchedResult { block.startCoroutineUninterceptedOrReturn(this) }
}
/**
* Starts this coroutine with the given code [block] in the same context and returns result when it
* completes without suspension.
* This function shall be invoked at most once on this coroutine.
*
* First, this function initializes parent job from the `parentContext` of this coroutine that was passed to it
* during construction. Second, it starts the coroutine using [startCoroutineUninterceptedOrReturn].
*/
public fun AbstractCoroutine.startUndispatchedOrReturn(receiver: R, block: suspend R.() -> T): Any? {
initParentJob()
return undispatchedResult { block.startCoroutineUninterceptedOrReturn(receiver, this) }
}
private inline fun AbstractCoroutine.undispatchedResult(startBlock: () -> Any?): Any? {
val result = try {
startBlock()
} catch (e: Throwable) {
CompletedExceptionally(e)
}
return when {
result === COROUTINE_SUSPENDED -> COROUTINE_SUSPENDED
makeCompletingOnce(result, MODE_IGNORE) -> {
if (result is CompletedExceptionally) throw result.cause else result
}
else -> COROUTINE_SUSPENDED
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy