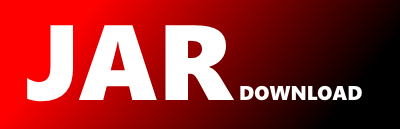
jvmMain.kotlinx.rpc.krpc.test.KRPCTestService.kt Maven / Gradle / Ivy
/*
* Copyright 2023-2024 JetBrains s.r.o and contributors. Use of this source code is governed by the Apache 2.0 license.
*/
package kotlinx.rpc.krpc.test
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.SharedFlow
import kotlinx.coroutines.flow.StateFlow
import kotlinx.rpc.RemoteService
import kotlinx.rpc.annotations.Rpc
@Suppress("detekt.TooManyFunctions")
@Rpc
interface KRPCTestService : RemoteService {
suspend fun empty()
suspend fun returnType(): String
suspend fun simpleWithParams(name: String): String
suspend fun genericReturnType(): List
suspend fun doubleGenericReturnType(): List>
suspend fun paramsSingle(arg1: String)
suspend fun paramsDouble(arg1: String, arg2: String)
suspend fun varargParams(arg1: String, vararg arg2: String)
suspend fun genericParams(arg1: List)
suspend fun doubleGenericParams(arg1: List>)
suspend fun mapParams(arg1: Map>>)
suspend fun customType(arg1: TestClass): TestClass
suspend fun nullable(arg1: String?): TestClass?
suspend fun variance(arg2: TestList, arg3: TestList2<*>): TestList?
suspend fun incomingStreamSyncCollect(arg1: Flow): Int
suspend fun incomingStreamAsyncCollect(arg1: Flow): Int
suspend fun outgoingStream(): Flow
suspend fun bidirectionalStream(arg1: Flow): Flow
suspend fun echoStream(arg1: Flow): Flow
suspend fun streamInDataClass(payloadWithStream: PayloadWithStream): Int
suspend fun streamInStream(payloadWithStream: Flow): Int
suspend fun streamOutDataClass(): PayloadWithStream
suspend fun streamOfStreamsInReturn(): Flow>
suspend fun streamOfPayloadsInReturn(): Flow
suspend fun streamInDataClassWithStream(payloadWithPayload: PayloadWithPayload): Int
suspend fun streamInStreamWithStream(payloadWithPayload: Flow): Int
suspend fun returnPayloadWithPayload(): PayloadWithPayload
suspend fun returnFlowPayloadWithPayload(): Flow
suspend fun bidirectionalFlowOfPayloadWithPayload(
payloadWithPayload: Flow
): Flow
suspend fun getNInts(n: Int): Flow
suspend fun getNIntsBatched(n: Int): Flow>
suspend fun bytes(byteArray: ByteArray)
suspend fun nullableBytes(byteArray: ByteArray?)
suspend fun throwsIllegalArgument(message: String)
suspend fun throwsSerializableWithMessageAndCause(message: String)
suspend fun throwsThrowable(message: String)
suspend fun throwsUNSTOPPABLEThrowable(message: String)
suspend fun nullableInt(v: Int?): Int?
suspend fun nullableList(v: List?): List?
suspend fun delayForever(): Flow
suspend fun answerToAnything(arg: String): Int
val plainFlowOfInts : Flow
val plainFlowOfFlowsOfInts : Flow>
val plainFlowOfFlowsOfFlowsOfInts : Flow>>
val sharedFlowOfInts : SharedFlow
val sharedFlowOfFlowsOfInts : SharedFlow>
val sharedFlowOfFlowsOfFlowsOfInts : SharedFlow>>
val stateFlowOfInts : StateFlow
val stateFlowOfFlowsOfInts : StateFlow>
val stateFlowOfFlowsOfFlowsOfInts : StateFlow>>
suspend fun emitNextForStateFlowOfInts(value: Int)
suspend fun emitNextForStateFlowOfFlowsOfInts(value: Int)
suspend fun emitNextForStateFlowOfFlowsOfFlowsOfInts(value: Int)
suspend fun sharedFlowInFunction(sharedFlow: SharedFlow): StateFlow
suspend fun stateFlowInFunction(stateFlow: StateFlow): StateFlow
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy