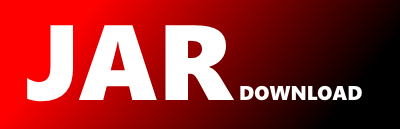
kotlinx.reflect.lite.KCallable.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlinx.reflect.lite Show documentation
Show all versions of kotlinx.reflect.lite Show documentation
Experimental lightweight library that replaces existing 'kotlin-reflect' implementation
The newest version!
/*
* Copyright 2016-2022 JetBrains s.r.o. Use of this source code is governed by the Apache 2.0 license.
*/
package kotlinx.reflect.lite
/**
* Represents a callable entity, such as a function or a property.
*
* @param R return type of the callable.
*/
public interface KCallable : KAnnotatedElement {
/**
* The name of this callable as it was declared in the source code.
* If the callable has no name, a special invented name is created.
* Nameless callables include:
* - constructors have the name "",
* - property accessors: the getter for a property named "foo" will have the name "",
* the setter, similarly, will have the name "".
*/
public val name: String
/**
* Parameters required to make a call to this callable.
* If this callable requires a `this` instance or an extension receiver parameter,
* they come first in the list in that order.
*/
public val parameters: List
/**
* The type of values returned by this callable.
*/
public val returnType: KType
/**
* The list of type parameters of this callable.
*/
@SinceKotlin("1.1")
public val typeParameters: List
/**
* Calls this callable with the specified list of arguments and returns the result.
* Throws an exception if the number of specified arguments is not equal to the size of [parameters],
* or if their types do not match the types of the parameters.
*/
public fun call(vararg args: Any?): R
/**
* Calls this callable with the specified mapping of parameters to arguments and returns the result.
* If a parameter is not found in the mapping and is not optional (as per [KParameter.isOptional]),
* or its type does not match the type of the provided value, an exception is thrown.
*/
public fun callBy(args: Map): R
/**
* Visibility of this callable, or `null` if its visibility cannot be represented in Kotlin.
*/
@SinceKotlin("1.1")
public val visibility: KVisibility?
/**
* `true` if this callable is `final`.
*/
@SinceKotlin("1.1")
public val isFinal: Boolean
/**
* `true` if this callable is `open`.
*/
@SinceKotlin("1.1")
public val isOpen: Boolean
/**
* `true` if this callable is `abstract`.
*/
@SinceKotlin("1.1")
public val isAbstract: Boolean
/**
* `true` if this is a suspending function.
*/
@SinceKotlin("1.3")
public val isSuspend: Boolean
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy